Graphics Programming I Optimizations PDF
Document Details
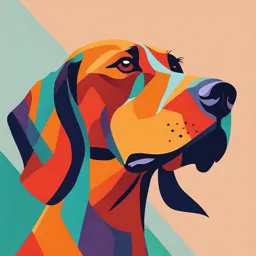
Uploaded by PrincipledSugilite4815
Howest
Tags
Summary
This document discusses optimizations for ray tracing, including techniques like intersection speed improvements, concurrency vs parallelism, acceleration structures (AABB), and multithreading. The focus is on practical examples and implementation of these concepts in graphics programming.
Full Transcript
GRAPHICS PROGRAMMING I OPTIMIZATIONS Graphics Programming 1 – Optimizations Ray Tracing| Optimizations Increase the intersection speed Reduce the number of rays More performant hit algorithms Moller-T...
GRAPHICS PROGRAMMING I OPTIMIZATIONS Graphics Programming 1 – Optimizations Ray Tracing| Optimizations Increase the intersection speed Reduce the number of rays More performant hit algorithms Moller-Trumbore (Triangle Intersection) Sphere HitTest (Geometric vs Analytic)... Data vs Object Oriented framework Reduce the number of intersections Acceleration Structures Axis-Aligned Bounding Boxes (AABB) - SlabTest Use parallel algorithms Uniform Grid Algorithm Multithreading Bounding Volume Hierarchies (BVH) Octree Algorithm Thread / ThreadPools Binary Space Partitioning (BSP) Tree Algorithm Parallel For KD-Tree Algorithm Async... Graphics Programming 1 – Optimizations Ray Tracing| Multithreading A software implementation allowing different threads to be executed concurrently. A multithreaded program appears to be doing several things at the same time even when it’s running on a single-core machine (concurrent). Concurrency vs Parallelism Concurrency Executing multiple tasks at the same time but not necessarily simultaneously. On a single-core machine it’s an illusion of multiple tasks running in parallel because of a very fast switching between threads/tasks by the CPU. Parallelism When the tasks are actually being executed in parallel on multiple cores. Multi-core machines will of course use a combination of concurrency and parallelism Graphics Programming 1 – Optimizations Ray Tracing| Hands-On 1. Restructure ‘renderer’ > Function to render a single pixel (task) 2. Synchronous Execution (default) 3. Parallel Execution (std::execution) Graphics Programming 1 – Optimizations Ray Tracing| Hands-On (MultiThreading) Create function to process a single pixel (Renderer::RenderPixel) Renderer.h Renderer.cpp Graphics Programming 1 – Optimizations Ray Tracing| Hands-On (MultiThreading) Renderer::Render Renderer.cpp The majority of the code can be removed (double for-loop) Data that every pixel needs for processing can be prefetched here CameraToWorld matrix and Camera Origin Scene pointer Aspect Ratio Field of View Calculate the total number of pixels (width * height) Make use of ‘preprocessor directives’ to switch between implementations Renderer.cpp (top) Graphics Programming 1 – Optimizations Ray Tracing| Hands-On (MultiThreading) Synchronous Logic (No Threading) Simple for-loop that loops amountOfPixels amount of times Renderer::Render Graphics Programming 1 – Optimizations Ray Tracing| Hands-On (MultiThreading) Parallel Logic We can easily parallelize our for-loop by making use of the "execution" library, part of the STD 'algorithms' starting from C++17 By adding an execution policy to a parallelizable algorithm, the task will be split up and multithread for you. We will use the std::for_each algorithm to loop over each pixel index and call RenderPixel for each. Requires the include of 'execution' header. Renderer.cpp Renderer::Render Graphics Programming 1 – Optimizations Ray Tracing| Acceleration Structure An acceleration structure is super useful to prevent unnecessary calculations (= reducing the number of intersection test per frame) There are lots of acceleration techniques available For this hands-on we are going to implement a very simple one The Slab-Test → AABB bases pre-hit check for our TriangleMeshes Every triangle mesh has an AABB which we test first. If no intersection is found, we don’t need to check the triangles. Graphics Programming 1 – Optimizations Ray Tracing| Acceleration Structure Axis-Aligned Bounding Box (AABB) is a box defined with the axis of our Cartesian coordinate system (X, Y, Z). AABB defined by two points which defined the maximum extent. Some systems have an additional origin point and have the extents being relative to the origin, but the memory overhead is often not desired! How can we perform Ray-AABB intersection tests? Ray / Line equation: 𝒑 𝑡 = 𝒐𝒓𝒂𝒚 + 𝑡𝒅 𝒐𝒑𝒍𝒂𝒏𝒆 − 𝒐𝒓𝒂𝒚 ⋅ 𝒏 𝒕= Volume bounds define a set of lines parallel to each axis. 𝒅⋅𝒏 We can express the volume’s minimum coordinate as: 𝒑 = 𝑩𝟎𝒙 To find intersection we can combine both: 𝑩𝟎𝒙 = 𝒐𝒓𝒂𝒚 + 𝑡𝒅 Reordering leads to: 𝑩𝟎𝒙 − 𝒐𝒓𝒂𝒚 𝒕𝟎𝒙 = 𝒅 This can also be done for each component/slab and for the volume’s maximum coordinate. Note that negative values means the intersection is behind the ray. Graphics Programming 1 – Optimizations Ray Tracing| Acceleration Structure Once we know all 𝒕 values, we need to determine if ray actually intersects our AABB. If our ray is parallel to an axis, it won’t be considered an intersection, similar to our ray-plane intersection. Per slab, find which of the two values lies on the cube by comparing values. Easy to do by knowing both max and min value of these values and check if max is larger than 0 and larger or equal to min. tmin = std::max(t0x, t0y) tmax = std::min(t1x, t1y) if(t0x > t1y || t0y > t1x) return false Graphics Programming 1 – Optimizations Ray Tracing| Acceleration Structure We can change this to 3D and we can make comparison easier by just tracking the min and max for each component while calculating the values. Graphics Programming 1 – Optimizations Ray Tracing| Acceleration Structure Update Vector3 class Vector3::Min > Get smallest components of 2 vectors Vector3::Max > Get biggest components of 2 vectors Vector3.h Vector3.cpp Vector3.cpp Graphics Programming 1 – Optimizations Ray Tracing| Acceleration Structure Update TriangleMesh Find AABB for (object-space) vertices (AABB is defined by a Min & Max position) Calculate the AABB after transforming the vertices (world-space)! While we don’t transform the AABB itself, if vertices change, we need to recalculate the min and max bounds (in world-space) DataTypes.h (TriangleMesh) Vector3.cpp Graphics Programming 1 – Optimizations Ray Tracing| Acceleration Structure Update TriangleMesh Function to calculate the object-space AABB (TriangleMesh::UpdateAABB) Function to calculate the word-space AABB (TriangleMesh::UpdateTransformedAABB) (closest fitting AABB after transformations) DataTypes.h (TriangleMesh) Vector3.cpp Graphics Programming 1 – Optimizations Ray Tracing| Acceleration Structure TriangleMesh::UpdateAABB DataTypes.h (TriangleMesh) Graphics Programming 1 – Optimizations Ray Tracing| Acceleration Structure DataTypes.h (TriangleMesh) TriangleMesh::UpdateAABB Make sure to Update the AABB every time the TriangleMesh::UpdateTransforms is called TriangleMesh::UpdateTransforms Graphics Programming 1 – Optimizations Ray Tracing| Acceleration Structure Utils::SlabTest_TriangleMesh Utils.h Graphics Programming 1 – Optimizations Ray Tracing| Acceleration Structure Perform SlabTest before testing the entire TriangleMesh Utils.h (HitTest_TriangleMesh) Graphics Programming 1 – Optimizations Ray Tracing| Acceleration Structure Use in your scenes! Scene_W4_Bunny::Initialize Graphics Programming 1 – Optimizations