CSE-2102 Final Exam Review PDF
Document Details
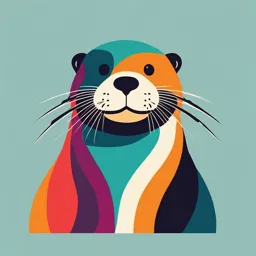
Uploaded by CostSavingDjinn
Jonathan Clark
Tags
Summary
This document is a review for a computer science final exam. It covers topics like databases, Python, and containers. Key concepts like Docker and JSON Web Tokens are also included, emphasizing material from modules 5, 7 and 8.
Full Transcript
CSE-2102 Final Exam Review Prof. Jonathan Clark INFORMATION Project grades posted by EOW Focus was on major components: (1) Pets list, (2) adoption, (3) approval, (4) users and roles If you didn’t work on it a...
CSE-2102 Final Exam Review Prof. Jonathan Clark INFORMATION Project grades posted by EOW Focus was on major components: (1) Pets list, (2) adoption, (3) approval, (4) users and roles If you didn’t work on it after Milestone 7, you will get a zero Some individuals will get higher grade than their teammates, some will get a lower grade SET surveys Dates Extra Credit options – Due Wed, 12/4 (graded by 12/8) Honors Conversion – Due Fri, 12/6 (graded by 12/10) Final Exam – Mon, 12/9 @1pm, ITE-C80 2 EXAM GOALS Conceptual understand of the material Does not cover the lecture modules already covered by the midterm. One Exception: DOES cover Databases (module 5)! And some concepts transcend the entire year. (E.g. everything you did in the group project) Goal: if you worked hard on the Group Project and were attentive during lecture, you’d ace the exam 3 LOGISTICS The Test 40 multiple choice ~90 min “Select all that apply” will have multiple answers Study: Lecture slides, labs, homeworks, group project code Practice exam in HCT – gives feel for types of questions, but not exhaustive Logistics Bring your ID Come 10-15 min early Spread out in the room – at least one seat between 4 MODULE 5 Databases Group Project work 5 PYTHON & SQLITE import sqlite3 def query(): conn = sqlite3.connect(‘your-db-name.db') conn.row_factory = sqlite3.Row result = conn.execute('SELECT * FROM _____').fetchall() conn.close() 6 CRUD SQL = Structure Query Language Create – inserting new records/values INSERT INTO (FIELD1, FILED2,…) VALUES (1, 2,…) Read – searching for records SELECT field1, field2, …FROM [WHERE ] Update – modifying existing records UPDATE SET field1=value1, field2=value2,… [WHERE ] Delete – removing existing records DELETE FROM [WHERE ] MODULE 7A Containers Lab 8 – Dockerize a simple python flask app Requirements.txt, pip freeze, Dockerfile commands, Docker build/run commands, Docker cleanup commands HW3 – Docker Different base images, exposing different ports 8 HYPERVISOR 9 “Bare Metal / Native” “Embedded / Hosted” CONTAINERS Virtual Machines = virtualize the hardware Hypervisor = program used to run/manage virtual machines Containers = Operating system virtualization Lighter weight than VMs (no hypervisor) Package up: code, dependencies, and OS itself Portability Docker – build, test, deploy containerized applications 10 CONTAINER V. T2 HYPERVISOR Hypervisor, VMs Container technology Abstraction of hardware Abstraction of the OS/app layer Usually abstract a single server Used to run multiple copies of application Virtualize the hardware of the Virtualize the OS of the host host machine to create multiple machine to create multiple VMs containers Gigabytes Megabytes Start/Stop in minutes Start/Stop in seconds 11 DOCKER IMAGES 12 https://www.linkedin.com/pulse/understanding-docker-layers-efficient-image-building-majid-sheikh/ DOCKER IMAGES 13 https://www.linkedin.com/pulse/understanding-docker-layers-efficient-image-building-majid-sheikh/ DOCKER IMAGES Layered – Start with a base image and build on top So organize so top layers are things that change more often Each layer increases size of image Solves software dependency issues Portable across clouds Simplifies DevOps 14 DOCKERFILE Dockerfile - text file (no file extension); contains a script of instructions The “blueprint”: FROM Specify the base image (starting point) WORKDIR Sets the working directory in the image for subsequent commands COPY Copy the file/folders to the image ADD Download files from HTTP/HTTPS destinations RUN < Command + ARGS> Executes any commands in a new layer on top of the current image and commits the result ENTRYPOINT [command + args] Command/script to be called when container starts CMD < Command > Lets you define the default program that is run once you start the container based on this image 15 DOCKER COMMANDS docker build – builds the image docker run – start the container (-p for port mapping) docker stop – stop the container docker rm – delete the container docker images – list all images docker ps – list running docker images docker exec – execute cmd in running container 16 DOCKER CLEANUP docker stop – stop the container docker container prune – remove all stopped containers docker image prune – remove all images w/o associated container docker rm – delete the container docker image rm – remove docker system prune – remove all unused containers, networks, images (both dangling and unused), and optionally, volumes 17 MODULE 7B Auth 18 TERMS Identity Who are you? Registration Provide documentation to establish digital identity Authentication (AuthN) Proof of identity Are you who you say you are? Authorization (AuthZ) Rights, privileges What are you allowed to do? 19 MFA Multi-factor Authentication (MFA) Something you know and something you have Two or more factors (2FA) Adaptive Authentication – authN rigor based on risk level of requested action Identity Provider (IdP) - Stores and manages digital identities Single Sign On (SSO) – one login controls access to disparate resources/apps 20 JWT JSON Web Token (JWT)… pronounced like “Jot” Compact and secure way of exchanging information JSON format, base64 encoded (NOT encrypted!) Contains “claims” of truth Digitally signed… so if someone changes the data inside, the key signature will no longer match Contains the address for verification of the signature 21 FLOW SUMMARY OpenID Connect (OIDC) Flow Types: 1. Client Credentials Flow – machine-to-machine 2. Implicit Flow – user login fetches token 3. Authorization Code Flow – user login, code for token 4. PKCE – Auth Code Flow + (code verifier, code challenge) 22 3 TOKENS Identity Token – For the client to get authenticated user info Access Token – For the server to protect resources Refresh Token – How the client obtains new Identity and Access Tokens after they expire MODULE 8 Frontend, React Lab 9 – npm commands to build/run locally, package.json, Lab 10 – React components, calling async functions Group Project work 24 FILE TYPES Three basic file types for a web page HTML – structure (e.g. paragraphs, tables, etc) CSS – style (e.g. text size, borders, drop shadows, animation, etc.) “Cascading Style Sheets” JS – dynamic functionality (e.g. show, hide, fetch data, etc) Javascript 25 https://scandiweb.com/blog/basics-of-website-development/ DOM Document Object Model (DOM) The API/interface into the webpage OO model/representation of a web page Independent of any programming language Has properties and methods Window – represents a window containing a DOM document Document – represents the webpage 26 MPA V. SPA Multi-Page Application Each link click loads a new page from the server (Browsers can cache to reduce number of server calls) User sees blank screen as new page loads Single-Page Application (SPA) Dynamically updates content on a single web page without requiring a full page reload from the server JS updates the DOM to update the screen as needed 27 ASYNC / AWAIT async keyword = function returns a Promise await keyword = execution stops/waits until Promise is resolved and returns a result You can also use the thenables.then() syntax let response = await fetch(url); let response = fetch(url) let value = await response.json();.then(response => response.json()) console.log(value);.then(json => { console.log(json); }).catch(err => console.error(err)); 28 AXIOS OR FETCH? Axios – has better backward compatibility. Perhaps easier access to the data. const axios = require('axios’); axios.get(url).then(response => { console.log(response.data); }).catch(error => { console.error(error); }); 29 JSX JavaScript XML (JSX) – syntactic extension for JS Allows the writing of HTML in our JS/TS React files const name = ‘UConn Huskies’; const element = The {name}; 30 REACT COMPONENTS Components – modular and reusable //Welcome.tsx State – internal values function Welcome({first, last}) { Props – R/O values return Hello {first} {last} passed between } components export default Welcome; Note: Use names with capital letter Use parens ( ) if your //Home.tsx JSX spans multiple lines 31 STATE MANAGEMENT useState hook Allows app to maintain state within a component E.g. fetch date from an API and display on screen asynchronously useContext hook Allows our app to share state w/o having to pass Props 32 MODULE 9 Cloud 33 DEPLOYMENT On-Premise… (aka. “On-prem”) = your own data center Public Cloud – managed by another company in their data center Private Cloud – virtualized environments managed by your company in your data center Operational Terms: High-Availability (HA) – multiple data centers, fail-over Disaster-Recovery (DR) – spread the data centers out 34 AS-A-SERVICE Infrastructure-as-a-Service (IaaS) Servers in the cloud You manage the scaling Platform-as-a-Service (PaaS) “Managed Service” – let the cloud provider handle scaling Serverless You just focus on writing business logic 35 AUTO SCALING Auto scaling = adjusting quantity of resources to match load Load balancing = distributing traffic across available resources 36 CLOUD TERMS Cloud Native = Software designed and built specifically for deployment to a modern cloud platform Cloud Computing = WHERE apps are deployed Cloud Native = HOW apps are built Edge Computing – Bring storage and compute closer to the consumer/client Polyglot – Mix of tools/languages (Right tool for the job) Infrastructure as Code (IAC) – Using code to create hardware and software resources 37 MODULE 10 Security, Privacy 38 INJECTION ATTACKS Input of malicious code or database commands (into an input field) Malicious instructions executed and damage the system E.g. Cross-site Scripting (XSS), SQL Injection 39 ATTACK TYPES DOS – Anything that causes the system to be unavailable DDOS – Distributed DOS (Lots and computers doing a coordination DOS) Brute Force – Trial-and-error… Try all possibilities Buffer Overflow – Overflowing the stack / heap Man-in-the-Middle – Intercept user traffic Supply Chain attack – Attack the third-party vendor software 40 COUNTER MEASURES Encryption of data ”at rest” and “in transit” Multi-factor authentication (MFA) Short timeouts of sessions Input Validation – Testing input entered by a user Client-side – helpful to users Server-side – prevent nefarious users Regular expressions – sequence of characters that specifies the exact pattern. E.g.: a,b,c{2,5} = ab followed by 2-5 c’s Prevents: XSS, Injection Attacks, buffer overflow Role-base Access Controls (RBAC) – assign users to roles instead of directly granting users access 41 PRIVACY Personally Identifiable Information (PII) Name, address, phone SSN, passport number, drivers license number DOB, email address Protected Health Information (PHI) Any information that relates to an individual’s health status, medical history, or treatment Doctors’ visits, prescription medication, lab tests, insurance information, Note: All PHI is also PII (but obviously not all PII is PHI) 42 DESIGN PATTERNS API Gateway Adapter Aggregator Circuit Breaker Decorator BFF Async Facade Strangler Messaging Health Check Factory CQRS Saga Singleton Sidecar 43 SAGA PATTERN Saga Pattern – manage data consistency across microservices in distributed transaction scenarios Orchestration – a controller (orchestrator) handles the order of interactions, conditional flow Choreography – services work independently using shared events 44 PATTERNS API Gateway – single point of entry Circuit breaker – fail fast Saga pattern – manage distributed transactions Health check – simple ping to check for service availability Asynchronous messaging – queue 45 PATTERNS Adapter – different interfaces but similar behavior Decorator – dynamically attach new behaviors to objects (not classes) without changing existing implementation Façade – simpler interface to complex system Factory – create concrete implementations of a common interface Singleton – single instance of a object 46 PATTERNS Aggregator – collect data from multiple microservices, rather than require client to make multiple calls Backend for Frontend (BFF) – unique API for each specific client Strangler Pattern – replace the monolith piece by piece; operate in parallel until monolith can be replaced CQRS – (Command Query Responsibility Segregation) Separate DB reads from writes Sidecar – Deploy part of your application into a separate process/container for isolation/encapsulation 47 MODULE 11 Reliable Programming 48 TECHNIQUES Simple techniques for reliability improvement: 1. Fault avoidance – Code in such a way that you avoid introducing faults into your program. 2. Input validation – Define the expected format for user inputs and validate that all inputs conforms 3. Failure management – Code so that failures have minimal impact 49 CODE SMELL EXAMPLES Large classes – single responsibility principle violated? Long methods/functions – Function doing more than one thing? Duplicated code – Changes have to be made everywhere the code is duplicated Meaningless names – a sign of programmer haste. Code harder to understand. Unused code – Increases reading complexity 50 Guard Clause = a check that immediately exits the function GUARD CLAUSES def agecheck2(age, experience): if age