JavaScript Arrays and Callback Functions PDF
Document Details
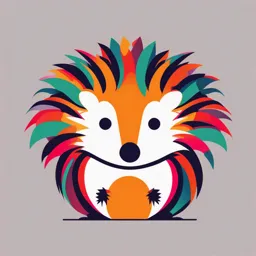
Uploaded by GlimmeringRational392
Université Ferhat Abbas de Sétif
Dr. AISSAOUA HABIB
Tags
Summary
This document provides a tutorial on JavaScript arrays and callback functions. It includes examples of different array methods and explains how callback functions can be used with arrays in programming.
Full Transcript
Callback Function A callback function is a function that is passed as an argument to another function and is executed after the completion of some operations. Dr. AISSAOUA HABIB University Setif -1- Callback Func...
Callback Function A callback function is a function that is passed as an argument to another function and is executed after the completion of some operations. Dr. AISSAOUA HABIB University Setif -1- Callback Function A callback's primary purpose is to execute code in response to an event. With a callback, you may instruct your application to "execute this code every time the user clicks a key on the keyboard." Dr. AISSAOUA HABIB University Setif -1- JavaScript arrays A JavaScript array has the following characteristics: 1. First, an array can hold values of mixed types 2. Second, the size of an array is dynamic. JavaScript provides you with two ways to create an array. The first one is to use the Array constructor as follows: let scores = new Array(9,10,8,7,6); scores is an array that has five elements. JavaScript allows you to omit the new operator Dr. AISSAOUA HABIB University Setif -1- JavaScript arrays The more preferred way to create an array is to use the array literal notation: let arrayName = [element1, element2, element3,...]; To access an element in an array, you specify an index in the square brackets [ ]: The length property of an array returns the number of elements. let mountains = ['Everest', 'Fuji', 'Nanga Parbat']; console.log(mountains.length); // 3 Dr. AISSAOUA HABIB University Setif -1- Some basic operations on arrays To add an element to the end of an array, you use the push() method: let seas = ['Black Sea', 'Caribbean Sea', 'North Sea', 'Baltic Sea']; seas.push('Red Sea'); console.log(seas); output: [ 'Black Sea', 'Caribbean Sea', 'North Sea', 'Baltic Sea', 'Red Sea' ] To add an element to the beginning of an array, you use the unshift() method: let seas = ['Black Sea', 'Caribbean Sea', 'North Sea', 'Baltic Sea']; seas.unshift('Red Sea'); console.log(seas); Output:[ 'Red Sea', 'Black Sea', 'Caribbean Dr. AISSAOUA HABIB Sea',Setif University 'North -1- Sea', 'Baltic Sea' ] Some basic operations on arrays To remove an element from the end of an array, you use the pop() method: let seas = ['Black Sea', 'Caribbean Sea', 'North Sea', 'Baltic Sea']; const lastElement = seas.pop(); console.log(lastElement); // Baltic Sea To remove an element from the beginning of an array, you use the shift() method: let seas = ['Black Sea', 'Caribbean Sea', 'North Sea', 'Baltic Sea']; const firstElement = seas.shift(); console.log(firstElement); // Black Sea Dr. AISSAOUA HABIB University Setif -1- Some basic operations on arrays The forEach() method executes a provided function once for each array element. Example: let students = ['John', 'Sara', 'Jack']; students.forEach(myFunction); function myFunction(item) { console.log(item); } Output: John Sara Jack Dr. AISSAOUA HABIB University Setif -1- Some basic operations on arrays The syntax of the forEach() method is: array.forEach(function(currentValue, index, arr)) Here, 1.function(currentValue, index, arr) - a function to be run for each element of an array 2. CurrentValue - the value of the current element 3. index (optional) - the index of the current element 4. arr (optional) - the array of the current elements Dr. AISSAOUA HABIB University Setif -1- Some basic operations on arrays Example: let students = ['John', 'Sara', 'Jack']; students.forEach(myFunction); function myFunction(item, index, arr) { arr[index] = 'Hello ' + item; } console.log(students); The output: ["Hello John", "Hello Sara", "Hello Jack"] Dr. AISSAOUA HABIB University Setif -1- Some basic operations on arrays The map method runs every item in the array through a function and returns a new array with the values returned by the function. Example: function double(number) { return number * 2; } let numbers = [1, 2, 3]; let numbersDoubled = numbers.map(double); The map() method runs the function we provided (double) on each item in the array and uses the return values to create a new array. In the example numbersDoubled is a new array containing [2, 4, 6] Dr. AISSAOUA HABIB University Setif -1- Some basic operations on arrays Filter() runs every item in the array through a condition that we set, and returns a new array with the values that match the condition. Example: let testScores = [90, 50, 100, 66, 25, 80, 81]; function isHighScore(score) { return score > 80; } let highTestScores = testScores.filter(isHighScore); console.log(highTestScores); // logs [90, 100, 81] Dr. AISSAOUA HABIB University Setif -1- Some basic operations on arrays reduce() method executes a reducer function on each element of the array and returns a single output value. It takes two parameters: an accumulator and the current element of an array. The accumulator is initialized to the first element of an array. The callback function performs some operation on the accumulator and the current element, and returns the result, which becomes the new value of the accumulator for the next iteration. The syntax is: let value = arr.reduce(function(accumulator,currentValue) { //... }, [initial]); Dr. AISSAOUA HABIB University Setif -1- Some basic operations on arrays Example: let arr = [10, 20, 30, 40, 50, 60]; // Callback function for reduce method function sumofArray(sum, i) { return sum + i; } // Display output console.log(arr.reduce(sumofArray)); const getMax = (a, b) => Math.max(a, b); let x=[1, 150,100].reduce(getMax, 500); console.log(x); Dr. AISSAOUA HABIB University Setif -1-