Javascript III - Introduction to Document Object Model PDF
Document Details
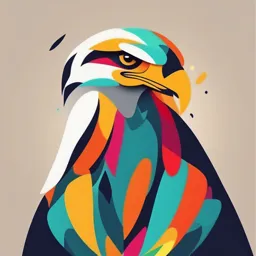
Uploaded by RockStarGraph3453
MSA University
Tags
Summary
This document provides an introduction to JavaScript's Document Object Model (DOM) and arrays. It explains how to work with arrays in JavaScript, including common array methods. This document may be for learning purposes, in an educational setting.
Full Transcript
Lecture06 Javascript III Introduction to Document Object Model Defining An Array ❑ const cars = [“Opel", “BMW", “Mercedes"]; OR can span multiple lines like const cars = [ "Saab", "Volvo", "BMW“]; ❑Code Examples: https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_new Defin...
Lecture06 Javascript III Introduction to Document Object Model Defining An Array ❑ const cars = [“Opel", “BMW", “Mercedes"]; OR can span multiple lines like const cars = [ "Saab", "Volvo", "BMW“]; ❑Code Examples: https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_new Defining An Array ❑ const cars = new Array("Saab", "Volvo", "BMW"); ❑ Is the same as ❑ const cars = []; cars= “Opel"; cars= “BMW"; cars= "Mercedes "; // for accessing the array element myArray let car= cars; = Date.now; myArray // for changing= the element value myFunction; myArray Cars=“Volvo”= myCars; ❑Code Examples: https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_new Accessing Array Elements const cars = [“Opel", “BMW", “Mercedes"]; cars = "Opel"; Retrieve the entire array: document.getElementById("demo").innerHTML = cars; Code Example: https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_full Defining an Array ❑It is a common practice to declare arrays as const ❑Arrays are Not Constants. ❑Const does NOT define a constant array. ❑It defines a constant reference to an array ❑Arrays CanNOT be reassigned. ❑Code Example: ❑https://www.w3schools.com/js/tryit.asp?filename=tryjs_const_array_assign const cars = ["Saab", "Volvo", "BMW"]; cars = ["Toyota", "Volvo", "Audi"]; // ERROR JavaScript has a built-in array constructor new Array(). JavaScript new Array() These two different statements both create a new empty array named points: const points = new Array(); const points = []; These two different statements both create a new array containing 6 numbers: const points = new Array(40, 100, 1, 5, 25, 10); const points = [40, 100, 1, 5, 25, 10]; A Common Error const points = ; //Create an array with one element: is not the same as: const points = new Array(40); // Create an array with 40 undefined elements: Converting Array to Strings const fruits = ["Banana", "Orange", "Apple", "Mango"]; document.getElementById("demo").innerHTML = fruits.toString(); OUTPUT → Banana,Orange,Apple,Mango const fruits = ["Banana", "Orange", "Apple", "Mango"]; document.getElementById("demo").innerHTML = fruits.join(" * "); OUTPUT: Banana * Orange * Apple * Mango Code Example: https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_join Array Length Property const fruits = ["Banana", "Orange", "Apple", "Mango"]; let length = fruits.length; let fruit = fruits; let fruit = fruits[fruits.length - 1]; C ode Example: https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_last Looping on Arrays using For const fruits = ["Banana", "Orange", "Apple", "Mango"]; let fLen = fruits.length; let text = ""; for (let i = 0; i < fLen; i++) { text += "" + fruits[i] + ""; } text += ""; C ode Example: https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_loop Looping on Arrays using foreach const fruits = ["Banana", "Orange", "Apple", "Mango"]; let text = ""; fruits.forEach(myFunction); text += ""; function myFunction(value) { text += "" + value + ""; } C ode Example: https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_loop_foreach How to Recognize an Array const fruits = ["Banana", "Orange", "Apple"]; let type = typeof fruits; //The typeof operator returns object because a JavaScript array is an object. Solution : Array.isArray(fruits); Or (fruits instanceof Array); Code example https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_isarray_method Nested Arrays and Objects Values in objects can be arrays, and values in arrays can be objects: const myObj = { name: "John", age: 30, cars: [ {name:"Ford", models:["Fiesta", "Focus", "Mustang"]}, {name:"BMW", models:["320", "X3", "X5"]}, {name:"Fiat", models:["500", "Panda"]} ] } Nested Arrays and Objects To access arrays inside arrays, use a for-in loop for each array: for (let i in myObj.cars) { x += "" + myObj.cars[i].name + ""; for (let j in myObj.cars[i].models) { x += myObj.cars[i].models[j]; } } Code Example https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_nested Push() and Pop() ! Push () → appends (at the end) an element to the array. Pop() → pops the last element in the array. const fruits = ["Banana", "Orange", "Apple"]; fruits.push("Lemon"); //Same as: const fruits = ["Banana", "Orange", "Apple"]; fruits[fruits.length] = "Lemon"; // Adds "Lemon" to fruits const fruits = ["Banana", "Orange", "Apple", "Mango"]; let fruit = fruits.pop(); Code Example: https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_add_push Shift and unshift shift () → removes the first array element and "shifts" all other elements to a lower index. unshift () → adds a new element to an array (at the beginning), and "unshifts" older elements const fruits = ["Banana", "Orange", "Apple"]; let fruit = fruits.shift(); const fruits = ["Banana", "Orange", "Apple", "Mango"]; Fruits.unshift(“lemon”); https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_u nshift_return Adding elements to array Adding elements with high indexes can create undefined "holes" in an array: const fruits = ["Banana", "Orange", "Apple"]; fruits = "Lemon"; // Creates undefined "holes" in fruits //the output Banana Orange Apple undefined undefined undefined Lemon JavaScript Array concat() The concat() method creates a new array by merging (concatenating) existing arrays: const myGirls = ["Cecilie", "Lone"]; const myBoys = ["Emil", "Tobias", "Linus"]; const myChildren = myGirls.concat(myBoys); //The concat() method does not change the existing arrays. It always returns a new array. //The concat() method can take any number of array arguments. The splice() method can be used to add new items to an array: JavaScript Array splice() const fruits = ["Banana", "Orange", "Apple", "Mango"]; fruits.splice(2, 0, "Lemon", "Kiwi"); //The first parameter (2) defines the position where new elements should be added (spliced in). //The second parameter (0) defines how many elements should be removed. //The rest of the parameters ("Lemon" , "Kiwi") define the new elements to be added. //The splice() method returns an array with the deleted items: you can use splice() to remove elements without leaving "holes" in the array: const fruits = ["Banana", "Orange", "Apple", "Mango"]; fruits.splice(2, 1); JavaScript Array slice() The slice() method slices out a piece of an array into a new array: const fruits = ["Banana", "Orange", "Lemon", "Apple", "Mango"]; const citrus = fruits.slice(1); //returns Orange, Lemon, Apple, Mango The slice() method creates a new array. The slice() method does not remove any elements from the source array. JavaScript Array flat() Flattening is useful when you want to convert a multi-dimensional array into a one- dimensional array. The flat() method creates a new array with sub-array elements concatenated to a specified depth. const myArr = [[1,2],[3,4],[5,6]]; const newArr = myArr.flat(); Code Example https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_flat JavaScript Array indexOf() and lastIndexOf() const fruits = ["Apple", "Orange", "Apple", "Mango"]; let position = fruits.indexOf("Apple") + 1; //If the item is present more than once, it returns the position of the first occurrence. const fruits = ["Apple", "Orange", "Apple", "Mango"]; let position = fruits.lastIndexOf("Apple") + 1; JavaScript Array find() and findIndex() The find() method returns the value of the first array element that passes a test function. const numbers = [4, 9, 16, 25, 29]; let first = numbers.find(myFunction); function myFunction(value, index, array) { return value > 18; } The findIndex() method returns the index of the first array element that passes a test function. const numbers = [4, 9, 16, 25, 29]; let first = numbers.findIndex(myFunction); function myFunction(value, index, array) { return value > 18; } Sorting an Array of strings const fruits = ["Banana", "Orange", "Apple", "Mango"]; fruits.sort(); fruits.reverse(); Another two methods toSorted() and toReversed() methods can be used to keep the original array unchanged const months = ["Jan", "Feb", "Mar", "Apr"]; const sorted = months.toSorted(); C ode Example: https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_sort_reverse Sorting a Numerical Array using (Compare function) ❑the sort() function sorts values as strings const points = [50, 900, 21, 35, 200, 10]; points.sort(function(a, b){return a - b}); points.sort(function(a, b){return b - a}); ❑C ode Example: https://www.w3schools.com/js/tryit.asp?filename=tryjs_array_sort_alpha Using Math.min() and Math.max on an Array const points = [40, 100, 1, 5, 25, 10]; function myArrayMin(arr) { return Math.min.apply(null, arr); } function myArrayMax(arr) { return Math.max.apply(null, arr); } JavaScript For Loop JavaScript supports different kinds of loops: for - loops through a block of code a number of times for/in - loops through the properties of an object for/of - loops through the values of an iterable object while - loops through a block of code while a specified condition is true do/while - also loops through a block of code while a specified condition is true JavaScript For Loop Syntax: for (expression 1; expression 2; expression 3) { // code block to be executed } for (let i = 0; i < 5; i++) { text += "The number is " + i + ""; } Expression 1 and 3 are optional let i = 0; let len = cars.length; let text = ""; for (; i < len; ) { text += cars[i] + ""; i++; } JavaScript For Loop Loop scope: var i = 5; for (var i = 0; i < 10; i++) { // some code } // Here i is 10 let i = 5; for (let i = 0; i < 10; i++) { // some code } // Here i is 5 JavaScript For Loop- For In The JavaScript for in statement loops through the properties of an Object: const person = {fname:"John", lname:"Doe", age:25}; let text = ""; for (let x in person) { text += person[x]; } The JavaScript for in statement can also loop over the properties of an Array: const numbers = [45, 4, 9, 16, 25]; let txt = ""; for (let x in numbers) { txt += numbers[x]; } JavaScript For Loop- For of The JavaScript for of statement loops through the values of an iterable const cars = ["BMW", "Volvo", "Mini"]; let text = ""; for (let x of cars) { text += x; } JavaScript while Loop Syntax while (condition) { // code block to be executed } while (i < 10) { text += "The number is " + i; i++; } JavaScript do-while Loop do { // code block to be executed } while (condition); do { text += "The number is " + i; i++; } while (i < 10); JavaScript Regular Expressions A regular expression is a sequence of characters that forms a search pattern. The search pattern can be used for text search and text replace operations. Syntax /pattern/modifiers; example /w3schools/i; /w3schools/i is a regular expression. w3schools is a pattern (to be used in a search). i is a modifier (modifies the search to be case-insensitive). JavaScript Regular Expressions //Use a regular expression to do a case-insensitive search for "w3schools" in a string: let text = "Visit W3Schools"; let n = text.search(/w3schools/i); // n =6 //Use a case insensitive regular expression to replace Microsoft with W3Schools in a string: let text = "Visit Microsoft!"; let result = text.replace(/microsoft/i, "W3Schools"); Regular Expression Patterns Brackets are used to find a range of characters: Expression Description Try it [abc] Find any of the characters between the brackets Try it » [0-9] Find any of the digits between the brackets Try it » (x|y) Find any of the alternatives separated with | Try it » Regular Expression Patterns Metacharacters are characters with a special meaning: Metacharacter Description Try it \d Find a digit Try it » \s Find a whitespace character Try it » \b Find a match at the beginning Try it » of a word like this: \bWORD, or Try it » at the end of a word like this: WORD\b \uxxxx Find the Unicode character specified by the hexadecimal number xxxx Regular Expression Patterns Quantifiers define quantities: Quantifier Description Try it n+ Matches any string that Try it » contains at least one n n* Matches any string that Try it » contains zero or more occurrences of n n? Matches any string that Try it » contains zero or one occurrences of n