Comp 1028 Programming And Algorithms Past Paper 2018-19 PDF
Document Details
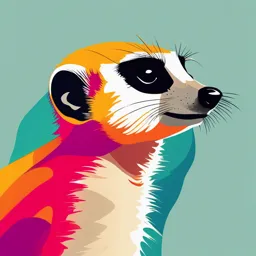
Uploaded by ClearedFoil
2019
The University of Nottingham
Tags
Related
- PGCET 2024-2025 Computer Stream Exam Paper PDF
- Fundamentals of Programming - Algorithms and Flowcharts PDF
- Computer Science Algorithms and Problem Solving 2015 PDF
- Computer Programming Concepts PDF
- National University of Computer & Emerging Sciences Final Exam CS302 PDF
- Introduction to Computer and Internet PDF for Engineers Exam
Summary
This is a past paper for a programming and algorithms course (COMP 1028) from the University of Nottingham, Malaysia Campus. It covers topics like program control, recursion, pseudocode, pointers, linked lists, scope, and function-related concepts for a first-level module in the autumn semester of 2018-2019. Several programming exercises and questions are included in the document related to these concepts.
Full Transcript
COMP 1028 The University of Nottingham Malaysia Campus SCHOOL OF COMPUTER SCIENCE A LEVEL 1 MODULE, AUTUMN SEMESTER 2018-19 PROGRAMMING AND ALGORITHMS (COMP 1028)...
COMP 1028 The University of Nottingham Malaysia Campus SCHOOL OF COMPUTER SCIENCE A LEVEL 1 MODULE, AUTUMN SEMESTER 2018-19 PROGRAMMING AND ALGORITHMS (COMP 1028) Time allowed ONE hour Candidates must NOT start writing their answers until told to do so Answer ALL questions No calculators are permitted in this examination. Dictionaries are not allowed with one exception. Those whose first language is not English may use a standard translation dictionary to translate between that language and English provided that neither language is the subject of this examination. Subject specific translation dictionaries are not permitted. No electronic devices capable of storing and retrieving text, including electronic dictionaries, may be used. DO NOT turn examination paper over until instructed to do so INFORMATION FOR INVIGILATORS: Collect both the exam papers and the answer booklets at the end of exam. COMP 1028 Turn Over 2 COMP 1028 1. Topics: Program Control, Recursion, Pseudocode a) Convert the following while loop into_a_ for loop. #include < stdio.h > int main() { int sum = 0, i = 3; while (i < 10) { i++; if (i % 3 == 0) sum += i; } return 0; } [3 marks] b) i) What reserved word can be used to_end_an execution of a loop? [2 marks] ii) A sequence of six tests, all scored out of 100, are to be given different weightings in determining a final mark. The following incomplete C program should compute the appropriate weighted score for one test. The fragment should first read values of testNumber and score. Complete the program below by using switch statement, to compute and print the appropriate value of weightedScore using the weightings given in the following table. Test Number Weight 1 10% 2 20% 3 20% 4 15% 5 15% 6 20% For example, input of 3 and 27 should produce the following output: A score of 27 on test 3 gives a weighted score of 5.40. continue on next page COMP 1028 Turn Over 3 COMP 1028 #include < stdio.h > int main() { int testNumber, score; float weight = 0.0; printf("Please enter the test number and the score: "); scanf("%d%d", & testNumber, & score); //complete your switch statement here printf("A score of %d on test %d give a weighted score of %.2 f\n", score, testNumber, weight); return 0; } [5 marks] iii) Write a function that solves the same problem described in part ii) using only conditional expressions. The whole body of the function should be written using only one statement. The following C program will display the following output for an input of 3 and 27: A score of 27 on test 3 gives a weighted score of 5.4. continue on next page COMP 1028 Turn Over 4 COMP 1028 #include < stdio.h > float result(int testNumber, int score); //function prototype int main() { int testNumber, score; float weight = 0.0; printf("Please enter the test number and the score: "); scanf("%d%d", & testNumber, & score); weight = result(testNumber, score); printf("A score of %d on test %d give a weighted score of %.2f\n", score, testNumber, weight); return 0; } float result(int testNumber, int score) { //write your function } [5 marks] COMP 1028 Turn Over 5 COMP 1028 c) Based on the following program with TWO functions: 1: #include 2: 3: int inclusion(int n1, int n2); 4: int mystery(int n); 5: 6: int main (){ 7: int n; 8: 9: printf("%d", mystery(n)); 10: return 0; 11:} 12: 13:int inclusion(int n1, int n2){ 14: if (n1 == 0) 15: return 0; 16: else 17: return n2 + inclusion(n1 - 1, n2); 18:} 19: 20:int mystery(int n){ 21: if (n == 0) 22: return 1; 23: else 24: return inclusion(2, mystery(n - 1)); 25:} What is the output if n = 3 in line 9? [4 marks] COMP 1028 Turn Over 6 COMP 1028 d) Translate the following flowchart to pseudocode. [6 marks] COMP 1028 Turn Over 7 COMP 1028 2. Topics: Pointers, Linked-List, Scope, C-Function a) The following C program compiles, links without errors and should print “COMP1028”, but it produces segmentation faults when we run it. 1: #include 2: #include 3: #include 4: 5: // linked list node for a list of c-strings 6: 7: typedef struct node { 8: char *data; //string data in this node 9: struct node *next; //next node or NULL if none 10: } Node; 11: 12: // print strings in list that starts at head 13: void print(Node *head); //function prototype print 14: 15: // add x to front of strlist and return pointer to new list head 16: Node *push_node(Node x, Node *strlist); //function prototype push_node 17: 18: // link two nodes together as a list and then print the list 19: int main () { 20: Node n1; 21: Node n2; 22: Node *list = NULL; //head of linked list of NULL if empty 23: 24: // copy "1028" to first node and push onto front of list 25: strcpy(n1.data, "1028"); 26: list = push_node(n1, list); 27: 28: // copy "COMP" to second node and push onto front of list 29: strcpy(n2.data, "COMP"); 30: list = push_node(n2, list); 31: 32: // print list 33: print(list); 34: return 0; 35: } 36: 37: // print strings in list that starts at head 38: void print(Node *head) { 39: Node *p = head; 40: 41: while (p != NULL) { 42: printf("%s", p->data); 43: p = p->next; 44: } 45: } COMP 1028 Turn Over 8 COMP 1028 46: 47: // add x to front of strlist and return pointer to new list head 48: Node *push_node(Node x, Node *strlist) { 49: x.next= strlist; 50: return &x; 51: } Identify the TWO errors in the code and suggest minimal changes that would allow the program to execute successfully. Your changes should not alter the general structure of the program, i.e., it should still link the two nodes in the main program into a linked list and print it. Indicate the line of code of each error, describe the error and give the correct code. [12 marks] b) What does the following function do? [3 marks] int mysteryFunction(char *x){ char *marker; int count = 0; for(marker = x; *marker; ++marker) ++ count; return count; } COMP 1028 Turn Over 9 COMP 1028 c) Based on the following program: #include char *username = "UNMC"; double average( int *x, int n); int main(){ int z; int i; for(i=0;i