Chapter 7 - IoT Physical Devices and Endpoints PDF
Document Details
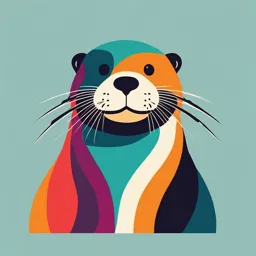
Uploaded by WholesomeNephrite2213
Tags
Related
- IoT Application Areas and Devices PDF
- Chapter 13 - 01 - Understand IoT Devices, Application Areas, and Communication Models - 03_ocred.pdf
- IoT Architecture PDF
- Chapter 13 - 01 - Understand IoT Devices, Application Areas, and Communication Models - 05_ocred.pdf
- Unit-II IoT PDF
- Chapter 7: IoT Physical Devices & Endpoints
Summary
This document provides an overview of IoT physical devices and endpoints, including their basic building blocks, examples like Raspberry Pi, and various components. It serves as an introduction to this technology.
Full Transcript
# Chapter 7 - IoT Physical Devices and Endpoints ## Outline - Basic building blocks of an IoT Device - Exemplary Device: Raspberry Pi - Raspberry Pi interfaces - Programming Raspberry Pi with Python - Other IoT devices ## What is an IoT Device - A “Thing” in Internet of Things (IoT) can be any o...
# Chapter 7 - IoT Physical Devices and Endpoints ## Outline - Basic building blocks of an IoT Device - Exemplary Device: Raspberry Pi - Raspberry Pi interfaces - Programming Raspberry Pi with Python - Other IoT devices ## What is an IoT Device - A “Thing” in Internet of Things (IoT) can be any object that has a unique identifier and which can send/receive data (including user data) over a network. Examples of these objects include: smart phone, smart TV, computer, refrigerator, car etc. - Iot Devices are connected to the internet and send information about themselves or about their surroundings. Examples of this include information sensed by connected sensors over a network or allowing actuation upon the physical entities/ environment around them remotely. ## IoT Device Examples - A home automation device that allows remotely monitoring the status of appliances and controlling the appliances. - An industrial machine which sends information abouts its operation and health monitoring data to a server. - A car which sends information about its location to a cloud-based service. - A wireless-enabled wearable device that measures data about a person such as the number of steps walked and sends the data to a cloud-based service. ## Basic Building Blocks Of An IoT Device - **Sensing:** <br> Sensors can be either on-board the IoT device or attached to the device. - **Actuation:** <br> IoT devices can have various types of actuators attached that allow taking actions upon the physical entities in the vicinity of the device. - **Communication:** <br> Communication modules are responsible for sending collected data to other devices or cloud-based servers/storage and receiving data from other devices and commands from remote applications. - **Analysis and Processing:** <br> Analysis and processing modules are responsible for making sense of the collected data. ## Block Diagram Of An IoT Device - **Connectivity:** * USB Host * RJ45/Ethernet - **Processor:** * CPU - **Graphics:** * GPU - **Interconnect:** - **Audio/Video:** * HDMI * 3.5mm audio * RCA video - **Interfaces:** * UART * SPI * I2C * CAN - **Storage Interfaces:** * SD * MMC * SDIO - **Memory Interfaces:** * NAND/NOR * DDR1/DDR2/DDR3 ## Exemplary Device: Raspberry Pi - Raspberry Pi is a low-cost mini-computer with the physical size of a credit card. - Raspberry Pi runs various flavors of Linux and can perform almost all tasks that a normal desktop computer can do. - Raspberry Pi also allows interfacing sensors and actuators through the general purpose I/O pins. - Since Raspberry Pi runs Linux operating system, it supports Python “out of the box”. >The phrase “out of the box” typically refers to something that is ready to use or functional immediately after initial setup or installation, without requiring additional configuration, modifications, or adjustments. - Raspberry Pi OS is based on the Debian Linux distribution and offers a user-friendly desktop environment, a variety of pre-installed applications, and access to a vast software repository. It is available in different editions, including a full desktop version with the Pixel desktop environment and a Lite version without the graphical desktop environment. ## Raspberry Pi Components - **System-on-a-Chip (SoC):** The SoC is the main processor of the Raspberry Pi board. It integrates the CPU (Central Processing Unit), GPU (Graphics Processing Unit), and other components onto a single chip. The most commonly used SoCs in Raspberry Pi models are from the Broadcom BCM2xxx series. - **CPU:** The CPU is responsible for executing instructions and performing general-purpose computing tasks. In Raspberry Pi boards, the CPU is typically an ARM-based processor, which provides low power consumption and efficient performance. - **GPU:** The GPU is a specialized processor designed to handle graphics-related tasks. It offloads graphic processing from the CPU and is particularly useful for multimedia applications and graphical user interfaces. - **RAM:** Random Access Memory (RAM) provides temporary storage for data and instructions that are actively used by the CPU. Raspberry Pi boards have varying amounts of RAM, typically ranging from 1GB to 8GB, depending on the model. - **Storage:** Raspberry Pi boards do not have built-in storage like traditional hard drives. Instead, they use microSD cards for storage, where the operating system, applications, and user data are stored. Some models also support booting from USB storage devices. - **GPIO Pins:** General Purpose Input/Output (GPIO) pins are a set of pins on the Raspberry Pi board that can be used to interact with external devices and components. These pins allow you to interface with sensors, motors, LEDs, and other electronic components. - **USB Ports:** Raspberry Pi boards feature multiple USB ports that can be used to connect peripherals such as keyboards, mice, USB storage devices, Wi-Fi dongles, and other USB-enabled devices. - **Video and Audio Outputs:** Raspberry Pi boards typically have HDMI or composite video outputs that allow you to connect them to monitors or TVs. They also have audio jacks or HDMI audio output for connecting speakers or headphones. - **Ethernet and Wireless Connectivity:** Raspberry Pi boards often come with Ethernet ports for wired network connections. Additionally, models like the Raspberry Pi 3 and later have built-in Wi-Fi and Bluetooth capabilities, allowing wireless connectivity. - **Power Supply:** Raspberry Pi boards require a power supply to operate. They typically use a micro USB or USB-C port for power input. The power requirements vary depending on the model and connected peripherals. ## Linux on Raspberry Pi - **Raspbian:** <br> Raspbian Linux is a Debian Wheezy port optimized for Raspberry Pi. - **Arch:** <br> Arch is an Arch Linux port for AMD devices. - **Pidora:** <br> Pidora Linux is a Fedora Linux optimized for Raspberry Pi. - **RaspBMC:** <br> RaspBMC is an XBMC media-center distribution for Raspberry Pi. - **OpenELEC:** <br> OpenELEC is a fast and user-friendly XBMC media-center distribution. - **RISC OS:** <br> RISC OS is a very fast and compact operating system. ## Raspberry Pi GPIO - 3V3 - 5V - GPIO 2 (I2C SDA) - 5V - GPIO 3 (I2C SDL) - GROUND - GPIO 4 - GPIO 14 (UART TXD) - GROUND - GPIO 15 (UART RxD) - GPIO 17 - GPIO 18 - GPIO 27 - GROUND - GPIO 22 - GPIO 23 - 3V3 - GPIO 24 - GPIO 10 (SPIO MOSI) - Ground - GPIO 9 (SPIO MISO) - GPIO 25 - GPIO 11 (SPIO SCLK) - GPIO 8 (SPIO CEO N) - GROUND - GPIO 7 (SPIO CE1 N) ## Raspberry Pi Interfaces - **Serial:** <br> The serial interface on Raspberry Pi has receive (Rx) and transmit (Tx) pins for communication with serial peripherals. - **SPI:** <br> Serial Peripheral Interface (SPI) is a synchronous serial data protocol used for communicating with one or more peripheral devices. - **I2C:** <br> The I2C interface pins on Raspberry Pi allow you to connect hardware modules. I2C interface allows synchronous data transfer with just two pins - SDA (data line) and SCL (clock line). >SDA stands for Serial Data Line and SCL stands for Serial Clock Line. These two lines are used in the Inter-Integrated Circuit (I2C) communication protocol, which is a low-speed bus that allows multiple devices to be connected on the same two-wire bus, each with a unique 7-bits address (up to 128 devices). The SDA line is used for sending and receiving data between the master and slave devices, while the SCL line carries the clock signal that synchronizes the data transfer between the devices. ## Raspberry Pi Example: Interfacing LED and Switch with Raspberry Pi ```python from time import sleep import RPI.GPIO as GPIO GPIO.setmode(GPIO.BCM) #Switch Pin GPIO.setup(25, GPIO.IN) #LED Pin GPIO.setup(18, GPIO.OUT) state=false def toggleLED(pin): state = not state GPIO.output(pin, state) while True: try: if (GPIO.input(25) == True): toggleLED(pin) sleep(.01) except KeyboardInterrupt: exit() ``` ## Other Devices - **pcDuino** - **BeagleBone Black** - **Cubieboard** ## pcDuino pcDuino is a single-board computer that is similar in concept to the Raspberry Pi. It is designed to provide a platform for learning, prototyping, and developing various projects. The pcDuino board combines the functionality of a microcontroller and a mini-PC, offering a wide range of features and connectivity options. Here are some key features and components of pcDuino: 1. **Processor**: pcDuino boards are typically powered by ARM-based processors 2. **RAM**: pcDuino boards come with varying amounts of onboard RAM, typically ranging from 512MB to 1GB or more. 3. **Storage**: Similar to Raspberry Pi 4. **GPIO Pins**: similar to the GPIO functionality on Raspberry Pi. 5. **Video and Audio Outputs**: pcDuino boards typically have HDMI or VGA video outputs, allowing you to connect them to monitors or displays. They also have audio jacks for connecting speakers or headphones. 6. **USB Ports**: pcDuino boards come with USB ports that can be used to connect peripherals such as keyboards, mice, USB storage devices, and other USB-enabled devices. 7. **Ethernet and Wireless Connectivity**: pcDuino boards often feature Ethernet ports for wired network connections. Some models also have built-in Wi-Fi and Bluetooth capabilities for wireless connectivity. 8. **Operating System**: pcDuino boards can run various operating systems, including Linux distributions such as Ubuntu and Android. ## BeagleBone Black BeagleBone Black is a single-board computer that is designed for embedded computing, prototyping, and development projects. It is produced by BeagleBoard.org and offers a range of features and connectivity options. Here are some key features and components of the BeagleBone Black: 1. **Processor**: BeagleBone Black is powered by a Texas Instruments Sitara AM335x ARM Cortex-A8 processor, which provides a balance between performance and power efficiency. The processor runs at a clock speed of 1 GHz. 2. **RAM**: The board has 512MB of onboard DDR3 RAM, which provides temporary storage for data and instructions during program execution. 3. **Storage**: Similar to other single-board computers, BeagleBone Black uses a microSD card for storage. The operating system, applications, and user data are stored on the microSD card. 4. **GPIO Pins**: BeagleBone Black features a large number of GPIO pins, allowing users to interface with external devices and components. It has 92 configurable I/O pins, including digital I/O, analog inputs, PWM (Pulse Width Modulation) outputs, I2C, SPI, and UART interfaces. 5. **Video and Audio Outputs**: BeagleBone Black has an HDMI output for connecting to monitors or displays. It also has an audio jack for connecting speakers or headphones. 6. **USB Ports**: The board offers a USB host port and a USB client port. The USB host port can be used to connect peripherals such as keyboards, mice, USB storage devices, and other USB-enabled devices. The USB client port allows the board to be connected to a computer for programming and debugging. 7. **Ethernet and Wireless Connectivity**: BeagleBone Black has a built-in Ethernet port for wired network connections. Additionally, it supports USB Wi-Fi adapters for wireless connectivity. 8. **Operating System**: BeagleBone Black is compatible with various operating systems, including Linux distributions such as Debian and Ubuntu. These operating systems provide a familiar environment for software development and offer a wide range of software libraries and tools. ## Cubieboard Cubieboard is a series of single-board computers that are designed for embedded computing, development, and DIY projects. They are developed by CubieTech Limited and offer a range of features and connectivity options. Here are some key features and components of the Cubieboard: 1. **Processor**: Cubieboard models typically use Allwinner ARM processors. The specific processor model can vary between different Cubieboard versions. For example, the Cubieboard 2 is powered by an Allwinner A20 dual-core Cortex-A7 processor. 2. **RAM**: Cubieboard models come with varying amounts of onboard RAM. The amount of RAM can range from 512MB to 2GB or more. 3. **Storage**: Cubieboard boards use a microSD card for storage, similar to other single-board computers. The microSD card holds the operating system, applications, and user data. 4. **GPIO Pins**: Cubieboard boards feature GPIO pins that allow users to interface with external devices and components. These pins can be used for digital input/output, analog input, and various other purposes. 5. **Video and Audio Outputs**: Cubieboard boards typically have HDMI or VGA video outputs, allowing you to connect them to monitors or displays. They also have audio jacks for connecting speakers or headphones. 6. **USB Ports**: Cubieboard boards come with USB ports that can be used to connect peripherals such as keyboards, mice, USB storage devices, and other USB-enabled devices. 7. **Ethernet and Wireless Connectivity**: Most Cubieboard models have built-in Ethernet ports for wired network connections. Some models also support USB Wi-Fi adapters for wireless connectivity. 8. **Operating System**: Cubieboard boards are compatible with various operating systems, including Linux distributions such as Ubuntu and Android. ## Thanks