CFE2_ch02_condensed.pdf
Document Details
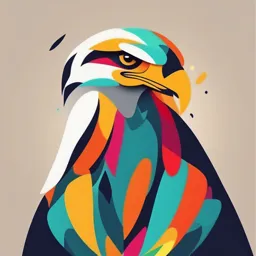
Uploaded by PunctualFoil
Universiti Malaysia Sabah
Full Transcript
Chapter Two: Fundamental Data Types C++ for Everyone by Cay Horstmann Slides by Evan Gallagher Copyright © 2012 by John Wiley & Sons. All rights reserved Variable Definitions The following statement def...
Chapter Two: Fundamental Data Types C++ for Everyone by Cay Horstmann Slides by Evan Gallagher Copyright © 2012 by John Wiley & Sons. All rights reserved Variable Definitions The following statement defines a variable. cans_per_pack is the variable’s name. int cans_per_pack = 6; int indicates that the variable cans_per_pack will be used to hold integers. = 6 indicates that the variable cans_per_pack will initially contain the value 6. C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved Variable Definitions Must obey the rules for valid names C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved Number Types A number written by a programmer is called a number literal. There are rules for writing literal values: C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved Number Types C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved Variable Names When you define a variable, you should pick a name that explains its purpose. For example, it is better to use a descriptive name, such as can_volume, than a terse name, such as cv. C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved Variable Names 1. Variable names must start with a letter or the underscore (_) character, and the remaining characters must be letters numbers, or underscores. 2. You cannot use other symbols such as $ or %. Spaces are not permitted inside names; you can use an underscore instead, as in can_volume. 3. Variable names are case-sensitive, that is, can_volume and can_Volume are different names. For that reason, it is a good idea to use only lowercase letters in variable names. 4. You cannot use reserved words such as double or return as names; these words are reserved exclusively for their special C++ meanings. C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved Variable Names C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved The Assignment Statement The contents in variables can “vary” over time (hence the name!). Variables can be changed by – assigning to them The assignment statement – using the increment or decrement operator – inputting into them The input statement C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved The Assignment Statement An assignment statement stores a new value in a variable, replacing the previously stored value. C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved The Assignment Statement cans_per_pack = 8; This assignment statement changes the value stored in cans_per_pack to be 8. The previous value is replaced. C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved The Assignment Statement C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved The Assignment Statement There is an important difference between a variable definition and an assignment statement: int cans_per_pack = 6; // Variable definition... cans_per_pack = 8; // Assignment statement The first statement is the definition of cans_per_pack. The second statement is an assignment statement. An existing variable’s contents are replaced. C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved The Assignment Statement The = in an assignment does not mean the left hand side is equal to the right hand side as it does in math. = is an instruction to do something: copy the value of the expression on the right into the variable on the left. Consider what it would mean, mathematically, to state: counter = counter + 2; counter EQUALS counter + 1 ? C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved The Assignment Statement counter = 11; // set counter to 11 counter = counter + 2; // increment C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved The Assignment Statement counter = 11; // set counter to 11 counter = counter + 2; // increment 1. Look up what is currently in counter (11) C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved The Assignment Statement counter = 11; // set counter to 11 counter = counter + 2; // increment 1. Look up what is currently in counter (11) 2. Add 2 to that value (13) C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved The Assignment Statement counter = 11; // set counter to 11 counter = counter + 2; // increment 1. Look up what is currently in counter (11) 2. Add 2 to that value (13) 3. copy the result of the addition expression into the variable on the left, changing counter cout > s3; double average = (s1 + s2 + s3) / 3; cout cans; The user can supply both inputs on the same line: Enter the number of bottles and cans: 2 6 C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved Input Statement C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved Formatted Output When you print an amount in dollars and cents, you usually want it to be rounded to two significant digits. You learned how to actually round off and store a value but, for output, we want to round off only for display. A manipulator is something that is sent to cout to specify how values should be formatted. To use manipulators, you must include the iomanip header in your program: #include and using namespace std; is also needed C++ for Everyone by Cay Horstmann Copyright © 2012 by John Wiley & Sons. All rights reserved Formatted Output You can combine manipulators and values to be displayed into a single statement: price_per_liter = 1.21997; cout