java.docx
Document Details
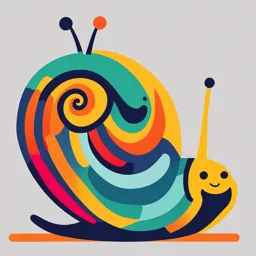
Uploaded by ImpressedMoldavite2157
Tags
Related
- Topic 1.2 Objects_ CS 002-CS21S1 - Advanced Object-Oriented Programming.pdf
- Introduction to Java Programming and Data Structures (2019) by Y. Daniel Liang - PDF
- Object-Oriented Programming using Java PDF
- Object-Oriented Programming Concepts - Chapter 10 Abstraction (PDF)
- CP213 Lesson 7: Object-Oriented Programming Concepts (PDF)
- Unit-1 Introduction to Object Oriented Programming and Basic Concepts PDF
Full Transcript
Java is a class-based object-oriented programming (OOP) language that is built around the concept of objects. OOP concepts (OOP) intend to improve code readability and reusability by defining how to structure a Java program efficiently. The main principles of object-oriented programming are: 1. [A...
Java is a class-based object-oriented programming (OOP) language that is built around the concept of objects. OOP concepts (OOP) intend to improve code readability and reusability by defining how to structure a Java program efficiently. The main principles of object-oriented programming are: 1. [Abstraction](https://raygun.com/blog/oop-concepts-java/#abstraction) 2. [Encapsulation](https://raygun.com/blog/oop-concepts-java/#encapsulation) 3. [Inheritance](https://raygun.com/blog/oop-concepts-java/#inheritance) 4. [Polymorphism](https://raygun.com/blog/oop-concepts-java/#polymorphism) 5. Class 6. object Java comes with specific code structures for each OOP principle. For example, the extends keyword for inheritance or getter and setter methods for encapsulation. **What are OOP concepts in Java?** OOP concepts allow us to create specific interactions between Java objects. They make it possible to reuse code without creating security risks or making a Java program less readable. Here are the four main principles in more detail. **Abstraction** Abstraction aims to hide complexity from the users and show them only the relevant information. For example, if you want to drive a car, you don't need to know about its internal workings. The same is true of Java classes. You can hide internal implementation details by using abstract classes or interfaces. On the abstract level, you only need to define the method signatures (name and parameter list) and let each class implement them in their own way. **Abstraction in Java:** - Hides the underlying complexity of data - Helps avoid repetitive code - Presents only the signature of internal functionality - Gives flexibility to programmers to change the implementation of the abstract behaviour - Partial abstraction (0-100%) can be achieved with abstract classes - Total abstraction (100%) can be achieved with interfaces **Encapsulation** Encapsulation allows us to protect the data stored in a class from system-wide access. As its name suggests, it safeguards the internal contents of a class like a real-life capsule. You can implement encapsulation in Java by keeping the fields (class variables) private and providing public getter and setter methods to each of them. Java Beans are examples of fully encapsulated classes. **Encapsulation in Java:** - Restricts direct access to data members (fields) of a class. - Fields are set to private - Each field has a getter and setter method - Getter methods return the field - Setter methods let us change the value of the field **Polymorphism** [**[Polymorphism]**](https://en.wikipedia.org/wiki/Polymorphism) refers to the ability to perform a certain action in different ways. In Java, polymorphism can take two forms: method overloading and method overriding. Method overloading happens when various methods with the same name are present in a class. When they are called they are differentiated by the number, order, and types of their parameters. Method overriding occurs when the child class overrides a method of its parent. **Polymorphism in Java:** - The same method name is used several times. - Different methods of the same name can be called from the object. - All Java objects can be considered polymorphic (at the minimum, they are of their own type and instances of the Object class). - Example of static polymorphism in Java is method overloading. - Example of dynamic polymorphism in Java is method overriding. **Inheritance** [**[Inheritance]**](https://raygun.com/blog/oop-concepts-java/www.linkedin.com/pulse/types-relationships-object-oriented-programming-oop-sarah-el-dawody/) makes it possible to create a child class that inherits the fields and methods of the parent class. The child class can override the values and methods of the parent class, however it's not necessary. It can also add new data and functionality to its parent. Parent classes are also called superclasses or base classes, while child classes are known as subclasses or derived classes as well. Java uses the extends keyword to implement the principle of inheritance in code. **Inheritance in Java:** - A class (child class) can extend another class (parent class) by inheriting its features. - Implements the DRY (Don't Repeat Yourself) programming principle. - Improves code reusability. - Multilevel inheritance is allowed in Java (a child class can have its own child class as well). - Multiple inheritances are not allowed in Java (a class can't extend more than one class). Features of Java ================ The primary objective of [Java programming](https://www.javatpoint.com/java-tutorial) language creation was to make it portable, simple and secure programming language. Apart from this, there are also some excellent features which play an important role in the popularity of this language. The features of Java are also known as Java buzzwords. 1. [Simple](https://www.javatpoint.com/features-of-java#Simple) 2. [Object-Oriented](https://www.javatpoint.com/features-of-java#Object-Oriented) 3. [Portable](https://www.javatpoint.com/features-of-java#Portable) 4. [Platform independent](https://www.javatpoint.com/features-of-java#Platform-independent) 5. [Secured](https://www.javatpoint.com/features-of-java#Secured) 6. [Robust](https://www.javatpoint.com/features-of-java#Robust) 7. [Architecture neutral](https://www.javatpoint.com/features-of-java#Architecture-neutral) 8. [Interpreted](https://www.javatpoint.com/features-of-java#Interpreted) 9. [High Performance](https://www.javatpoint.com/features-of-java#High-Performance) 10. [Multithreaded](https://www.javatpoint.com/features-of-java#Multithreaded) 11. [Distributed](https://www.javatpoint.com/features-of-java#Distributed) 12. [Dynamic](https://www.javatpoint.com/features-of-java#Dynamic) Java Features **[Simple]** Java is very easy to learn, and its syntax is simple, clean and easy to understand. According to Sun Microsystem, Java language is a simple programming language because: - Java syntax is based on C++ (so easier for programmers to learn it after C++). - Java has removed many complicated and rarely-used features, for example, explicit pointers, operator overloading, etc. - There is no need to remove unreferenced objects because there is an Automatic Garbage Collection in Java. ### [Object-oriented] Java is an [object-oriented](https://www.javatpoint.com/java-oops-concepts) programming language. Everything in Java is an object. Object-oriented means we organize our software as a combination of different types of objects that incorporate both data and behavior. Object-oriented programming (OOPs) is a methodology that simplifies software development and maintenance by providing some rules. Basic concepts of OOPs are: 1. [Object](https://www.javatpoint.com/object-and-class-in-java) 2. [Class](https://www.javatpoint.com/object-and-class-in-java#class) 3. [Inheritance](https://www.javatpoint.com/inheritance-in-java) 4. [Polymorphism](https://www.javatpoint.com/runtime-polymorphism-in-java) 5. [Abstraction](https://www.javatpoint.com/abstract-class-in-java) 6. [Encapsulation](https://www.javatpoint.com/encapsulation) ### [Platform Independent]  Java is platform independent because it is different from other languages like [C](https://www.javatpoint.com/c-programming-language-tutorial), [C++](https://www.javatpoint.com/cpp-tutorial), etc. which are compiled into platform specific machines while Java is a write once, run anywhere language. A platform is the hardware or software environment in which a program runs. There are two types of platforms software-based and hardware-based. Java provides a software-based platform. The Java platform differs from most other platforms in the sense that it is a software-based platform that runs on top of other hardware-based platforms. It has two components: 1. Runtime Environment 2. API(Application Programming Interface) Java code can be executed on multiple platforms, for example, Windows, Linux, Sun Solaris, Mac/OS, etc. Java code is compiled by the compiler and converted into bytecode. This bytecode is a platform-independent code because it can be run on multiple platforms, i.e., Write Once and Run Anywhere (WORA). **[Secured]** Java is best known for its security. With Java, we can develop virus-free systems. Java is secured because: - **No explicit pointer** - **Java Programs run inside a virtual machine sandbox** how Java is secured - **Classloader:** Classloader in Java is a part of the Java Runtime Environment (JRE) which is used to load Java classes into the Java Virtual Machine dynamically. It adds security by separating the package for the classes of the local file system from those that are imported from network sources. - **Bytecode Verifier:** It checks the code fragments for illegal code that can violate access rights to objects. - **Security Manager:** It determines what resources a class can access such as reading and writing to the local disk. Java language provides these securities by default. Some security can also be provided by an application developer explicitly through SSL, JAAS, Cryptography, etc. **[Robust]** The English mining of Robust is strong. Java is robust because: - It uses strong memory management. - There is a lack of pointers that avoids security problems. - Java provides automatic garbage collection which runs on the Java Virtual Machine to get rid of objects which are not being used by a Java application anymore. - There are exception handling and the type checking mechanism in Java. All these points make Java robust. **[Architecture-neutral]** Java is architecture neutral because there are no implementation dependent features, for example, the size of primitive types is fixed. In C programming, int data type occupies 2 bytes of memory for 32-bit architecture and 4 bytes of memory for 64-bit architecture. However, it occupies 4 bytes of memory for both 32 and 64-bit architectures in Java. **[Portable]** Java is portable because it facilitates you to carry the Java bytecode to any platform. It doesn\'t require any implementation. **[High-performance]** Java is faster than other traditional interpreted programming languages because Java bytecode is \"close\" to native code. It is still a little bit slower than a compiled language (e.g., C++). Java is an interpreted language that is why it is slower than compiled languages, e.g., C, C++, etc. **[Distributed]** Java is distributed because it facilitates users to create distributed applications in Java. RMI and EJB are used for creating distributed applications. This feature of Java makes us able to access files by calling the methods from any machine on the internet. **[Multi-threaded]** A thread is like a separate program, executing concurrently. We can write Java programs that deal with many tasks at once by defining multiple threads. The main advantage of multi-threading is that it doesn\'t occupy memory for each thread. It shares a common memory area. Threads are important for multi-media, Web applications, etc. **[Dynamic]** Java is a dynamic language. It supports the dynamic loading of classes. It means classes are loaded on demand. It also supports functions from its native languages, i.e., C and C++. [Java Essentials] If you are planning to learn Java, but not sure where to begin and which technologies to focus on, this post is for you. If you are a beginner who has started out in the field of programming and you are in the phase of developing your programming skills, there are certain essentials that you must be aware of. Learning any programming language is a tedious process requiring a great level of focus and determination otherwise it is easy to give up. Here we have covered the essentials of Java programming that you must be familiar with as a beginner. [Java virtual machine] JVM (Java Virtual Machine) is an abstract machine. It is a specification that provides runtime environment in which java bytecode can be executed. JVMs are available for many hardware and software platforms (i.e. JVM is platform dependent). [What is JVM] It is: 1. **A specification** where working of Java Virtual Machine is specified. But implementation provider is independent to choose the algorithm. Its implementation has been provided by Oracle and other companies. 2. **An implementation** Its implementation is known as JRE (Java Runtime Environment). 3. **Runtime Instance** Whenever you write java command on the command prompt to run the java class, an instance of JVM is created. [What it does] The JVM performs following operation:514 - Loads code - Verifies code - Executes code - Provides runtime environment JVM provides definitions for the: - Memory area - Class file format - Register set - Garbage-collected heap - Fatal error reporting etc. [JVM Architecture] Let\'s understand the internal architecture of JVM. It contains classloader, memory area, execution engine etc.  [1) Classloader] Classloader is a subsystem of JVM which is used to load class files. Whenever we run the java program, it is loaded first by the classloader. There are three built-in classloaders in Java. 1. **Bootstrap ClassLoader**: This is the first classloader which is the super class of Extension classloader. It loads the *rt.jar* file which contains all class files of Java Standard Edition like java.lang package classes, java.net package classes, java.util package classes, java.io package classes, java.sql package classes etc. 2. **Extension ClassLoader**: This is the child classloader of Bootstrap and parent classloader of System classloader. It loades the jar files located inside *\$JAVA\_HOME/jre/lib/ext* directory. 3. **System/Application ClassLoader**: This is the child classloader of Extension classloader. It loads the classfiles from classpath. By default, classpath is set to current directory. You can change the classpath using \"-cp\" or \"-classpath\" switch. It is also known as Application classloader. 4. These are the internal classloaders provided by Java. If you want to create your own classloader, you need to extend the ClassLoader class. 2\) Class(Method) Area Class(Method) Area stores per-class structures such as the runtime constant pool, field and method data, the code for methods. 3\) Heap It is the runtime data area in which objects are allocated. 4\) Stack Java Stack stores frames. It holds local variables and partial results, and plays a part in method invocation and return. Each thread has a private JVM stack, created at the same time as thread. A new frame is created each time a method is invoked. A frame is destroyed when its method invocation completes. 5\) Program Counter Register PC (program counter) register contains the address of the Java virtual machine instruction currently being executed. 6\) Native Method Stack It contains all the native methods used in the application. 7\) Execution Engine It contains: 1. **A virtual processor** 2. **Interpreter:** Read bytecode stream then execute the instructions. 3. **Just-In-Time(JIT) compiler:** It is used to improve the performance. JIT compiles parts of the byte code that have similar functionality at the same time, and hence reduces the amount of time needed for compilation. Here, the term \"compiler\" refers to a translator from the instruction set of a Java virtual machine (JVM) to the instruction set of a specific CPU. 8\) Java Native Interface Java Native Interface (JNI) is a framework which provides an interface to communicate with another application written in another language like C, C++, Assembly etc. Java uses JNI framework to send output to the Console or interact with OS libraries. Structure of Java Program ========================= Java is an [object-oriented programming](https://www.javatpoint.com/java-oops-concepts), **platform-independent,** and **secure** programming language that makes it popular. Using the Java programming language, we can develop a wide variety of applications. So, before diving in depth, it is necessary to understand the **basic structure of Java program** in detail. In this section, we have discussed the basic **structure of a Java program**. At the end of this section, you will able to develop the [Hello world Java program](https://www.javatpoint.com/simple-program-of-java), easily. Structure of Java Program Let\'s see which elements are included in the structure of a [Java program](https://www.javatpoint.com/java-programs). A typical structure of a [Java](https://www.javatpoint.com/java-tutorial) program contains the following elements: - Documentation Section - Package Declaration - Import Statements - Interface Section - Class Definition - Class Variables and Variables - Main Method Class - Methods and Behaviors C++ vs Java =========== There are many differences and similarities between the [C++ programming](https://www.javatpoint.com/cpp-tutorial) language and [Java](https://www.javatpoint.com/java-tutorial). A list of top differences between C++ and Java are given below: **Comparison Index** **C++** **Java** ----------------------------------------- ----------------------------------------------------------------------------------------------------------------------------------------------------------------------------- ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- **Platform-independent** C++ is platform-dependent. Java is platform-independent. **Mainly used for** C++ is mainly used for system programming. Java is mainly used for application programming. It is widely used in Windows-based, web-based, enterprise, and mobile applications. **Design Goal** C++ was designed for systems and applications programming. It was an extension of the [C programming language](https://www.javatpoint.com/c-programming-language-tutorial). Java was designed and created as an interpreter for printing systems but later extended as a support network computing. It was designed to be easy to use and accessible to a broader audience. **Goto** C++ supports the [goto](https://www.javatpoint.com/cpp-goto-statement) statement. Java doesn\'t support the goto statement. **Multiple inheritance** C++ supports multiple inheritance. Java doesn\'t support multiple inheritance through class. It can be achieved by using [interfaces in java](https://www.javatpoint.com/interface-in-java). **Operator Overloading** C++ supports [operator overloading](https://www.javatpoint.com/cpp-overloading). Java doesn\'t support operator overloading. **Pointers** C++ supports [pointers](https://www.javatpoint.com/cpp-pointers). You can write a pointer program in C++. Java supports pointer internally. However, you can\'t write the pointer program in java. It means java has restricted pointer support in java. **Compiler and Interpreter** C++ uses compiler only. C++ is compiled and run using the compiler which converts source code into machine code so, C++ is platform dependent. Java uses both compiler and interpreter. Java source code is converted into bytecode at compilation time. The interpreter executes this bytecode at runtime and produces output. Java is interpreted that is why it is platform-independent. **Call by Value and Call by reference** C++ supports both call by value and call by reference. Java supports call by value only. There is no call by reference in java. **Structure and Union** C++ supports structures and unions. Java doesn\'t support structures and unions. **Thread Support** C++ doesn\'t have built-in support for threads. It relies on third-party libraries for thread support. Java has built-in [thread](https://www.javatpoint.com/multithreading-in-java) support. **Documentation comment** C++ doesn\'t support documentation comments. Java supports documentation comment (/\*\* \... \*/) to create documentation for java source code. **Virtual Keyword** C++ supports virtual keyword so that we can decide whether or not to override a function. Java has no virtual keyword. We can override all non-static methods by default. In other words, non-static methods are virtual by default. **unsigned right shift \>\>\>** C++ doesn\'t support \>\>\> operator. Java supports unsigned right shift \>\>\> operator that fills zero at the top for the negative numbers. For positive numbers, it works same like \>\> operator. **Inheritance Tree** C++ always creates a new inheritance tree. Java always uses a single inheritance tree because all classes are the child of the Object class in Java. The Object class is the root of the [inheritance](https://www.javatpoint.com/inheritance-in-java) tree in java. **Hardware** C++ is nearer to hardware. Java is not so interactive with hardware. **Object-oriented** C++ is an object-oriented language. However, in the C language, a single root hierarchy is not possible. Java is also an [object-oriented](https://www.javatpoint.com/java-oops-concepts) language. However, everything (except fundamental types) is an object in Java. It is a single root hierarchy as everything gets derived from java.lang.Object. **Note** - Java doesn\'t support default arguments like C++. - Java does not support header files like C++. Java uses the import keyword to include different classes and methods. [JavaFX Environment] ================================ We have to set the JavaFX environment on the system in order to run JavaFX Applications. All the versions of Java after JDK 1.8, supports JavaFX therefore we must have JDK 1.8 or later installed on our system. There are various IDE such as Net-beans or Eclipse also supports JavaFX Library. In this chapter, we will discuss the various ways of executing JavaFX applications. [Install Java] -------------------------- ### Step 1: Verify that it is already installed or not Check whether Java is already installed on the system or not. In my case, it is not installed therefore I need to install JDK 1.8 on my computer.  ### Step 2: Download JDK Click the below link to download jdk 1.8 for you windows 64 bit system. **Download JDK For Windows** There are available releases for Linux and mac operating systems. You can visit the official link for JDK distributions i.e. Step 3: Install JDK Open the executable file which you have just downloaded and follow the steps. JavaFX Install JDK Click **Next** to continue  Just Choose Development Tools and click Next. JavaFX Install JDK 2 Set up is being ready.  Choose the Destination folder in which you want to install JDK. Click Next to continue with the installation. JavaFX Install JDK 4 Set up is installing Java to the computer.  We have successfully installed Java SE development kit 8. Close the installation set up. Step 4 : Set the Permanent Path To execute Java applications from command line, we need to set Java Path. To set the path, follow the following steps. JavaFX Install Java Set the Permanent Path Right click on \"this PC\". It can be named as \"My Computer\" in some systems. Choose \"properties\" from the options.  The screen look alike the above image will open. Click on \"Advanced system settings\" to continue. JavaFX Install Java Set the Permanent Path 2 Above window will open. Click on \"Environment Variables\" to continue.  Enter \"path\" in variable name and enter the path to the bin folder inside your JDK in the variable value. Click OK. Now Java Path has been set up. Open the Command prompt and type **\"javac\"** In case you have already open up the command prompt, I suggest you to close the existing window and reopen it again. We will get javac executed as shown in the image below. JavaFX Install Java Set the Permanent Path 4 The Java has been installed on our system. Now, we need to configure IDEs like NetBeans or Eclipse in order to execute JavaFX applications. **[Integrated Development Ebvironment]** Java IDE (**Integrated Development Environment**) is a software application that enables users to **write** and **debug** Java programs more easily. Most IDEs have features such as syntax highlighting and code completion that helps users to code more easily. Usually, Java IDEs include a **code editor, a compiler, a debugger**, and **an interpreter** that the developer may access via a single graphical user interface. Java IDEs also provide language-specific elements such as **Maven, Ant building tools, Junit**, and **TestNG for testing**. The [Java](https://www.javatpoint.com/java-tutorial) IDE or Integrated Development Environment provides considerable support for the application development process. Through using them, we can save time and effort and set up a standard development process for the team or company. **Eclipse, NetBeans, [IntelliJ IDEA](https://www.javatpoint.com/intellij-idea-tutorial)**, and many other IDE\'s are most popular in the Java IDE\'s that can be used according to our requirements. In this topic, we will discuss the best Java IDE\'s that are used by the users. The following are the best Java IDEs that are mostly used in the world: - [Eclipse](https://www.javatpoint.com/java-ides#Eclipse) - [NetBeans](https://www.javatpoint.com/java-ides#NetBeans) - [IntelliJ IDEA](https://www.javatpoint.com/java-ides#IntelliJ-IDEA) - [BlueJ](https://www.javatpoint.com/java-ides#BlueJ) - [JCreator](https://www.javatpoint.com/java-ides#JCreator) - [JDeveloper](https://www.javatpoint.com/java-ides#JDeveloper) - [MyEclipse](https://www.javatpoint.com/java-ides#MyEclipse) - [Greenfoot](https://www.javatpoint.com/java-ides#Greenfoot) - [DrJava](https://www.javatpoint.com/java-ides#DrJava) - [Xcode](https://www.javatpoint.com/java-ides#Xcode) - [Codenvy](https://www.javatpoint.com/java-ides#Codenvy) ### Eclipse It is a Java-based open-source platform that enables us to create highly customized IDEs from [Eclipse](https://www.javatpoint.com/javafx-how-to-install-eclipse) member\'s plug-in components. This platform is also suitable for beginners to create user-friendly and more sophisticated applications. It contains many plugins that enable developers to develop and test code written in different languages. Some of Eclipse\'s features are as follows: 1. Eclipse provides powerful tools for different software development processes, such as charting, reporting, checking, etc. so that Java developers can build the application as quickly as possible. 2. Eclipse can be used on platforms such as MacOS, Linux, Windows, and Solaris. 3. Eclipse could also make several mathematical documents with LaTeX using both the TeXlipse plug-in and Mathematica software packages. ### NetBeans NetBeans is a Java-based IDE and basic application platform framework. Besides Java, [JavaScript](https://www.javatpoint.com/javascript-tutorial) and [JavaFX](https://www.javatpoint.com/javafx-tutorial), NetBeans supports [PHP](https://www.javatpoint.com/php-tutorial), [C](https://www.javatpoint.com/c-programming-language-tutorial)/[C++](https://www.javatpoint.com/cpp-tutorial), [Groovy](https://www.javatpoint.com/groovy), and [HTML5](https://www.javatpoint.com/html5-tutorial) languages. It is a free and open-source Java IDE that enables Java developers to develop various applications using different module sets. Some of the following features of [NetBeans](https://www.javatpoint.com/how-to-install-netbeans-on-centos) are as follows: 1. NetBeans is available for various operating systems, such as Linux, MacOS, Windows, Solaris, etc. 2. Although NetBeans is mainly a Java IDE, it has extensions to operate in many other programming languages, such as C, PHP, C++, JavaScript, HTML5, etc. 3. NetBeans may be used on different systems such as MacOS, Windows, Solaris and Linux. IntelliJ IDEA It is a free and open-source commercial Java IDE. It has two versions that are a paid-up Ultimate edition and a free and open-source community edition. Some of the features of the IDEA IntelliJ are as follows: 1. IntelliJ IDEA has several features to make programming easier, like code completion, debugging, XML editing support, code refactoring, code checks, TestNG, unit testing, etc. 2. Both versions of IntelliJ IDEA support different programming languages like Java, Kotlin, Groovy, Scala, etc. BlueJ It is a Java IDE that is widely used by the world\'s Java programmers. However, it was mainly designed for educational purposes, but also useful for software development. Some of BlueJ\'s features are as follows: 1. The BlueJ main screen displays the current development application\'s class structure, which is simple to access and change objects. 2. BlueJ\'s basic design is distinct from other IDE\'s because it was specifically designed to teach OOPS to beginners. ### JCreator It is another lightweight Java IDE developed by Xerox Software. It has a similar graphical interface to Microsoft\'s Visual Studio. It is available in three different versions: Lite Edition, Pro Edition and Life-Pro Edition. Unlike Java IDEs, which are developed using Java, JCreator is developed completely in C++. In addition, it does not require a [JRE](https://www.javatpoint.com/difference-between-jdk-jre-and-jvm#jre) for Java code execution. The developer community believes that JCreator is quicker than most traditional Java IDEs for the unique purpose. On the other hand, it\'s small and easy, making it perfect for newbies starting with Java. The following are the features of JCreator IDE: 1. JCreator\'s paid version has different features similar to other Java IDEs like code wizards, debugger Ant support, and project management. However, it does not have advanced features such as automatic refactoring, support for popular frameworks, etc. 2. Since JCreator is a Java IDE program in C++, there is no need for a JRE to execute Java code. 3. Despite some limitations, JCreator is a perfect Java IDE for beginners due to its compact size and higher pace. 4. JCreator can be used on various platforms like Linux and Windows. ### JDeveloper JDeveloper is a free and open-source Java IDE supported by Oracle Corporation. It can be used to build applications in different languages other than Java, like [PL/SQL](https://www.javatpoint.com/pl-sql-tutorial), PHP, [XML](https://www.javatpoint.com/xml-tutorial), [HTML](https://www.javatpoint.com/html-tutorial), JavaScript, [SQL](https://www.javatpoint.com/sql-tutorial), etc. The following are the features of JDeveloper IDE: 1. JDeveloper also simplifies the application development process by offering a wide range of visual application development tools and all the tools required to build an advanced coding environment. 2. JDeveloper may implement the Oracle Application Development Framework, which is an end-to-end Java EE-based framework. It means that the production of the application process becomes much simpler. 3. It offers several features for the entire application\'s life cycle that is coding, debugging, designing, deploying, optimizing, etc. ### MyEclipse It is a unique Java IDE built on top of the Eclipse platform that is created and maintained by Genuitec. Many tools and techniques available in MyEclipse could be used to develop Java apps and web development. It also offers support for JavaScript, HTML, [Angular](https://www.javatpoint.com/angularjs-tutorial), and [TypeScript](https://www.javatpoint.com/typescript-tutorial) and provides support for database connectors and application server connectors. It has five editions: the Professional Edition, the Regular Edition, the Blue Edition, the Spring Edition and the Bling Edition. Some of MyEclipse\'s features are as follows: 1. MyEclipse offers support to web programming languages like HTML, JavaScript, Angular, TypeScript, etc. 2. Various tools are available in MyEclipse, like visual web designers, spring tools database tools, persistence tools, etc. that may be used to develop Java apps and web development. 3. MyEclipse IDE provides various features, including Spring Tooling, WebSphere Connectors, Maven Project Management, Database Connectors, and Swing GUI Design Support etc. Greenfoot It is an IDE that has been developed to provide high school and undergraduate education. Greenfoot is free and open-source software that is maintained with the help of Oracle. Some of Greenfoot\'s characteristics are as follows: 1. Greenfoot has the ability to show various OOPS features like class & entity relationships, processes, object interactions, parameters, etc. 2. Two-dimensional graphical applications could be easily built using Greenfoot; prime examples are simulations, immersive games, etc. 3. Also, as it was intended to be a learning medium, it has easy access to animation and sound. 1. Unlike other Java IDEs, DrJava has a consistent presence on various platforms. It\'s because it was designed using the Swing toolkit of Sun Microsystems. 2. There are various features available for expert Java Developers in DrJava, including automatic indentation, commenting autocompletion, syntax coloring, brace matching, etc. 3. It will interactively test the Java code from the console and display the result in the same console. ### Xcode It is a Java IDE developed by Apple Inc. for MacOS. It can be accessed free of charge. This IDE comes with several software development tools for the development of TVOS, MacOS, and iOS software and allows Java programming. Some of the main highlights of Xcode are the built-in debugger, GUI designer, and autocomplete profile. AppleScript, Objective-C, C, Objective-C++, C++, [Ruby](https://www.javatpoint.com/ruby-tutorial), [Python](https://www.javatpoint.com/python-tutorial), and [Swift](https://www.javatpoint.com/swift-tutorial) are provided by Xcode, in addition to Java. There are the following features of Xcode as follows: 1. Split Window View and Coding Assistant 2. Code Filter and User Interface Prototyping 3. Version Editor and Source Control 4. Testing and Configurations 5. Quick Access and Schemes Management ### Codenvy It is a commercial Java IDE built on Eclipse Che. Developers can select the IDE from the three Java IDE variants, which is the creator, enterprise, and team. It is a workspace for cloud-based and on-demand developers. In addition to writing Java code using a browser-based editor, java developer may take advantage of the additional features, including refactoring, syntax highlighting, and code completion. By providing an easy way to create, debug, edit, and run different projects, the Codenvy editor also makes things better for programmers. Codenvy\'s team version is targeted at teamwork. In addition to Java, it offers various programming languages for both interpreted and compiled, including [C\#](https://www.javatpoint.com/c-sharp-tutorial), JavaScript, C++, and PHP. The following are the features of the Codenvy IDE: 1. Act with an adaptable and well-paced IDE on-prem or in the cloud with 2. With extensions and assemblies from Eclipse Che, customize the IDE. 3. Start a project, from any device, in seconds. 4. Act online with CLI push, pull, and clone, or offline. 5. Start the create, run, and deploy parallel processes. Java Variables ============== A variable is a container which holds the value while the [Java program](https://www.javatpoint.com/simple-program-of-java) is executed. A variable is assigned with a data type. Variable is a name of memory location. There are three types of variables in java: local, instance and static. There are two types of [data types in Java](https://www.javatpoint.com/java-data-types): primitive and non-primitive. Variable A variable is the name of a reserved area allocated in memory. In other words, it is a name of the memory location. It is a combination of \"vary + able\" which means its value can be changed. ### Types of Variables There are three types of variables in [Java](https://www.javatpoint.com/java-tutorial): - local variable - instance variable - static variable  #### 1) Local Variable A variable declared inside the body of the method is called local variable. You can use this variable only within that method and the other methods in the class aren\'t even aware that the variable exists. A local variable cannot be defined with \"static\" keyword. #### 2) Instance Variable A variable declared inside the class but outside the body of the method, is called an instance variable. It is not declared as [static](https://www.javatpoint.com/static-keyword-in-java). It is called an instance variable because its value is instance-specific and is not shared among instances. #### 3) Static variable A variable that is declared as static is called a static variable. It cannot be local. You can create a single copy of the static variable and share it among all the instances of the class. Memory allocation for static variables happens only once when the class is loaded in the memory. Primitive Data Types in Java ============================ Primitive data types in Java are predefined by the Java language and named as the reserved keywords. A primitive data type does not share a state with other primitive values. [Java programming language](https://www.javatpoint.com/java-tutorial) supports the following eight primitive data types. 1. Boolean data type 2. byte data type 3. int data type 4. long data type 5. float data type 6. double data type 7. char data type 8. short data type in this section, we will discuss all the Primitive data types in detail. ### Primitive Number Types Generally, the primitive number types are classified into two categories: **Whole numbers:** The whole numbers hold the complete number, positive and negative, for example, 170, 225, -170, -225, etc. For these numbers, the valid data types are **byte, short, int**, and **long**. It depends on the number that which data type would be preferred. 10 Sec C++ vs Java **Floating Numbers:** The floating numbers are the numbers with a fraction part. These numbers have one or more decimal values, for example, 10.25, 15.25, etc. For these numbers, the valid data types are **float** and **double**. From the all above data types, the int, double, and float are the most widely used data types. Before understanding the Primitive data types, let\'s discuss [data types in Java](https://www.javatpoint.com/java-data-types): ### Data Types in Java As its name specifies, [data types](https://www.javatpoint.com/java-data-types) are used to specify the type of data to store inside the variables. Java is a statically-typed language, which means all the variables should be declared before use. So, we have to specify the variable\'s type and name. A variable is declared as follows: The above statement acknowledges your program that a file \'a\' exists and holds integer type data with value 1. A variables data type specifies the type of value it contains. Data types in Java categories into two categories: - Primitive - Non-primitive A non-primitive data type can be a class, interface, and Array. Let\'s back to our main topic, primitive data type; discuss each primitive data type in detail: ### 1) Boolean Data Type A [boolean data type](https://www.javatpoint.com/boolean-keyword-in-java) can have two types of values, which are **true** and **false.** It is used to add a simple flag that displays true/false conditions. It represents one bit of information. It\'s is not a specific size data type. So, we can not precisely define its size. **Example:** ### 2) Byte Data Type It is an 8-bit signed [2\'s complement](https://www.javatpoint.com/2s-complement-in-digital-electronics) integer. It can have a value of (-128) to 127 ( inclusive). Below are the benefits of using the [byte data type](https://www.javatpoint.com/byte-keyword-in-java): - It is useful for saving memory in large Arrays. - It can be used instead of int to clarify our code using its limits. - It saves memory, too, because it is 4 times smaller than an integer. **Example:** ### 3) Int Data Type The int stands for Integer; it is a 32-bit signed two\'s complement integer. It\'s value can be from -2\^31 to (2\^31-1), which is -32,768 to 32,767 (inclusive). Its default value is zero. It represents an unsigned 32-bit integer, which has a value range from 0 to 32,767. If memory saving is not our primary goal, then the [int data type](https://www.javatpoint.com/int-keyword-in-java) is used to define the integer value. **Example:** ### 4) Long Data Type It is a 64-bit 2\'s complement integer with a value range of (-2\^63) to (2\^63 -1) inclusive. It is used for the higher values that can not be handled by the int data type. **Example:** ### 5) Float Data Type The [Float data type](https://www.javatpoint.com/float-keyword-in-java) is used to declare the floating values ( fractional numbers). It is a single-precision 32-bit IEEE 754 floating-point data type. Its value range is infinite. While declaring the floating, we must end the value with an f. It is useful for saving memory in large arrays of floating-point numbers. It is recommended to use float data type instead of double while saving the floating numbers in large arrays, and not use it with precise numbers such as currency. **Example:** ### 6) Double Data Type The [double data type](https://www.javatpoint.com/double-keyword-in-java) is also used for the floating-point ( Fractional values) number. It is much similar to the float data type. But, generally, it is used for decimal values. Like the float data type, its value range is infinite and also can not be used for precise values such as currency. The default value of the double data type is 0.0d. While declaring the double type values, we must end the value with a d. **Example:** ### 7) Char Data Type The [char data type](https://www.javatpoint.com/char-keyword-in-java) is also an essential primitive data type in Java. It is used to declare the character values. It is a single 16-bit Unicode Character with a value range of 0 to 65,535 (inclusive). While declaring a character variable, its value must be surrounded by a single quote (\'\'). **Example:** **char** myChar= \'H\';  System.out.println(myChar);  ### 8) Short Data Type The [short data type](https://www.javatpoint.com/short-keyword-in-java) is also used to store the integer values. It is a 16-bit signed 2\'s complement integer with a value range of -32,768 to 32,767 (inclusive). It is also used to save memory, just like the byte data type. It is recommended to use the short data type in a large array when memory saving is essential. System.out.println(num);  Here, we have discussed all the primitive data types in Java. Other data types such as Strings, Classes, Interfaces, and Arrays are non-primitive data types in Java. However, Java provides support for character strings using the **String** class of Java.lang package. String class has some special support from the Java Programming language, so, technically it is a primitive data type. While using String class, a character string will automatically create a new String Object. For example, String s= \" JavaTpoint is the best portal to learn Java\"; Learn more about the [String class in Java](https://www.javatpoint.com/java-string). ### Default Values of Primitive data type In Java In Java, it is not necessary to assign values while declaring. Every data type has some default values. If we do not assign a value to a data type, it will be initialized to the default values by the compiler. Mostly, these values are null or 0 (Zero), depending on the data type. However, it is not recommended to leave the variables to their default values. If you have declared a variable, then initialize it with some value. Otherwise, do not declare it unnecessarily. The below table is representing the data types with their default values: **Data type** **Default Value** --------------- ------------------- boolean false Byte 0 Int 0 Long 0L float 0.0f double 0.0d Char \'\\u0000\' short 0 Identifiers in Java =================== Identifiers in Java are symbolic names used for identification. They can be a class name, variable name, method name, package name, constant name, and more. However, In [Java](https://www.javatpoint.com/java-tutorial), There are some reserved words that can not be used as an identifier. For every identifier there are some conventions that should be used before declaring them. Let\'s understand it with a simple Java program: 1. **public** **class** HelloJava {  2.     **public** **static** **void** main(String\[\] args) {  3.         System.out.println(\"Hello JavaTpoint\");  4.     }  5. }  Rules for Identifiers in Java There are some rules and conventions for declaring the identifiers in Java. If the identifiers are not properly declared, we may get a compile-time error. Following are some rules and conventions for declaring identifiers: - A valid identifier must have characters \[A-Z\] or \[a-z\] or numbers \[0-9\], and underscore(\_) or a dollar sign (\$). for example, \@javatpoint is not a valid identifier because it contains a special character which is @. - There should not be any space in an identifier. For example, java tpoint is an invalid identifier. - An identifier should not contain a number at the starting. For example, 123javatpoint is an invalid identifier. - An identifier should be of length 4-15 letters only. However, there is no limit on its length. But, it is good to follow the standard conventions. - We can\'t use the Java reserved keywords as an identifier such as int, float, double, char, etc. For example, int double is an invalid identifier in Java. - An identifier should not be any query language keywords such as SELECT, FROM, COUNT, DELETE, etc. Java Reserved Keywords Java reserved keywords are predefined words, which are reserved for any functionality or meaning. We can not use these keywords as our identifier names, such as class name or method name. These keywords are used by the syntax of Java for some functionality. If we use a reserved word as our variable name, it will throw an error. In Java, every reserved word has a unique meaning and functionality. Consider the below syntax: 1. **double** marks;  in the above statement, double is a reserved word while marks is a valid identifier. Below is the list of reserved keywords in Java: abstract continue for protected transient ---------- ---------- ------------ -------------- ----------- Assert Default Goto public Try Boolean Do If Static throws break double implements strictfp Package byte else import super Private case enum Interface Short switch Catch Extends instanceof return void Char Final Int synchronized volatile class finally long throw Date const float Native This while Although the const and goto are not part of the Java language; But, they are also considered keywords. Example of Valid and Invalid Identifiers **Valid identifiers:** Following are some examples of valid identifiers in Java: - TestVariable - testvariable - a - i - Test\_Variable - \_testvariable - \$testvariable - sum\_of\_array - TESTVARIABLE - jtp123 - JavaTpoint - Javatpoint123 **Invalid identifiers:** Below are some examples of invalid identifiers: - Test Variable ( We can not include a space in an identifier) - 123javatpoint ( The identifier should not begin with numbers) - java+tpoint ( The plus (+) symbol cannot be used) - a-javatpoint ( Hyphen symbol is not allowed) - java\_&\_Tpoint ( ampersand symbol is not allowed) - Java\'tpoint (we can not use an apostrophe symbol in an identifier) We should follow some naming convention while declaring an identifier. However, these conventions are not forced to follow by the Java programming language. That\'s why it is called conventions, not rules. But it is good to follow them. These are some industry standards and recommended by Java communities such as Oracle and Netscape. If we do not follow these conventions, it may generate confusion or erroneous code. Literals in Java ================ In [Java](https://www.javatpoint.com/java-tutorial), **literal** is a notation that represents a fixed value in the source code. In lexical analysis, literals of a given type are generally known as [**tokens**](https://www.javatpoint.com/java-tokens). In this section, we will discuss the term **literals in Java**. Literals -------- In Java, **literals** are the constant values that appear directly in the program. It can be assigned directly to a variable. Java has various types of literals. The following figure represents a literal. Literals in Java Types of Literals in Java ------------------------- There are the majorly **four** types of literals in Java: 1. Integer Literal 2. Character Literal 3. Boolean Literal 4. String Literal  Integer Literals Integer literals are sequences of digits. There are three types of integer literals: - **Decimal Integer:** These are the set of numbers that consist of digits from 0 to 9. It may have a positive (**+)** or negative (**-**) Note that between numbers commas and non-digit characters are not permitted. For example, **5678, +657, -89,** etc. - **Octal Integer:** It is a combination of number have digits from 0 to 7 with a leading 0. For example, **045, 026,** - **Hexa-Decimal:** The sequence of digits preceded by **0x** or **0X** is considered as hexadecimal integers. It may also include a character from **a** to **f** or **A** to **F** that represents numbers from **10** to **15**, respectively. For example, **0xd, 0xf,** - **Binary Integer:** Base 2, whose digits consists of the numbers 0 and 1 (you can create binary literals in Java SE 7 and later). Prefix 0b represents the Binary system. For example, 0b11010. Real Literals The numbers that contain fractional parts are known as real literals. We can also represent real literals in exponent form. For example, **879.90, 99E-3,** etc. Backslash Literals Java supports some special backslash character literals known as backslash literals. They are used in formatted output. For example: **\\n:** It is used for a new line **\\t:** It is used for horizontal tab **\\b:** It is used for blank space **\\v:** It is used for vertical tab **\\a:** It is used for a small beep **\\r:** It is used for carriage return **\\\':** It is used for a single quote **\\\":** It is used for double quotes Character Literals A character literal is expressed as a character or an escape sequence, enclosed in a **single** quote (**\'\'**) mark. It is always a type of char. For example, **\'a\', \'%\', \'\\u000d\',** etc. String Literals String literal is a sequence of characters that is enclosed between **double** quotes (\"\") marks. It may be alphabet, numbers, special characters, blank space, etc. For example, \"**Jack\", \"12345\", \"\\n\",** etc. Floating Point Literals The vales that contain decimal are floating literals. In Java, float and double primitive types fall into floating-point literals. Keep in mind while dealing with floating-point literals. - Floating-point literals for float type end with F or f. For example, **6f, 8.354F**, etc. It is a **32**-bit float literal. - Floating-point literals for double type end with D or d. It is optional to write D or d. For example, **6d, 8.354D,** etc. It is a **64**-bit double literal. - It can also be represented in the form of the **exponent**. **Floating:** **Decimal:** **Decimal in Exponent form:** Boolean Literals Boolean literals are the value that is either true or false. It may also have values 0 and 1. For example, **true, 0,** etc. **boolean** isEven = **true**;  Null Literals **Null** literal is often used in programs as a marker to indicate that reference type object is unavailable. The value **null** may be assigned to any variable, except variables of primitive types. String stuName = **null**;  Student age = **null**;  Class Literals **Class literal** formed by taking a type name and appending **.class** extension. For example, **Scanner.class**. It refers to the object (of type Class) that represents the type itself. **class** classType = Scanner.**class**;  Invalid Literals There is some invalid declaration of literals. **float** g = 6\_.674f;  **float** g = 6.\_674F;  **long** phoneNumber = 99\_00\_99\_00\_99\_L;  **int** x = 77\_;  **int** y = 0\_x76;  **int** z = 0X\_12;   **int** z = 0X12\_;   Restrictions to Use Underscore (\_) - It can be used at the beginning, at the end, and in-between of a number. - It can be adjacent to a decimal point in a floating-point literal. - Also, can be used prior to an F or L suffix. - In positions where a string of digits is expected. Why use literals? To avoid defining the constant somewhere and making up a label for it. Instead, to write the value of a constant operand as a part of the instruction. Operators in Java ================= **Operator** in [Java](https://www.javatpoint.com/java-tutorial) is a symbol that is used to perform operations. For example: +, -, \*, / etc. There are many types of operators in Java which are given below: - Unary Operator, - Arithmetic Operator, - Shift Operator, - Relational Operator, - Bitwise Operator, - Logical Operator, - Ternary Operator and - Assignment Operator. Java Operator Precedence ------------------------ **Operator Type** **Category** **Precedence** ------------------- ---------------------- -------------------------------------------------- Unary Postfix *expr*++ *expr*\-- Prefix ++*expr* \--*expr* +*expr* -*expr* \~ ! Arithmetic multiplicative \* / % Additive \+ - Shift Shift \\> \>\>\> Relational comparison \< \> \= instanceof Equality == != Bitwise bitwise AND & bitwise exclusive OR \^ bitwise inclusive OR \| Logical logical AND && logical OR \|\| Ternary Ternary ? : Assignment assignment = += -= \*= /= %= &= \^= \|= \= \>\>\>= ### Java Unary Operator The Java unary operators require only one operand. Unary operators are used to perform various operations i.e.:HTML Tutorial - incrementing/decrementing a value by one - negating an expression - inverting the value of a boolean Highest precedence in Java ========================== When we talk about precedence in Java, the operator comes first in mind. There are certain rules defined in Java to specify the order in which the operators in an expression are evaluated. **Operator precedence** is a concept of determining the group of terms in an expression. The operator precedence is responsible for evaluating the expressions. In [Java](https://www.javatpoint.com/java-tutorial), **parentheses()** and **Array subscript\[\]** have the highest precedence in Java. For example, Addition and Subtraction have higher precedence than the Left shift and Right shift operators. Highest precedence in Java Below is a table defined in which the lowest precedence operator show at the top of it. ---------------------------------------------------------------------------------------------- **Precedence** **Operator** **Type** **Associativity** ---------------- -------------- ------------------------------------------ ------------------- **1)** =\ Assignment\ Right to left +=\ Addition assignment\ -=\ Subtraction assignment\ \*=\ Multiplication assignment\ /=\ Division assignment\ %= Modulus assignment **2)** ? : Ternary conditional Right to left **3)** \|\| Logical OR Left to right **4)** && Logical AND Left to right **5)** \| Bitwise inclusive OR Left to right **6)** \^ Bitwise exclusive OR Left to right **7)** & Bitwise AND Left to right **8)** !=\ Relational is not equal to\ Left to right == Relational is equal to **9)** \=\ Relational greater than or equal\ instanceof Type comparison (objects only) **10)** \>\>\ Bitwise right shift with sign extension\ Left to right \\> Bitwise right shift with zero extension **11)** -\ Subtraction\ Left to right + Addition **12)** \*\ Multiplication\ Left to right /\ Division\ % Modulus **13)** -\ Unary minus\ Right to left +\ Unary plus\ \~\ Unary bitwise complement\ !\ Unary logical negation\ ( type) Unary typecast **14)** ++\ Unary post-increment\ Right to left \-- Unary post-decrement **15)** ·\ Dot operator\ Left to Right ()\ Parentheses\ \[\] Array subscript ---------------------------------------------------------------------------------------------- ### Precedence order When two operators share a single operand, the operator having the highest precedence goes first. For example, x + y \* z is treated as x + (y \* z), whereas x \* y + z is treated as (x \* y) + z because \* operator has highest precedence in comparison of + operator. ### Associativity Associative is a concept related to the operators applied when two operators with the same precedence come in an expression. The associativity concept is very helpful to goes from that situation. Suppose we have an expression a + b - c **(+ and - operators have the same priority)**, and this expression will be treated as **(a + (b - c))** because these operators are right to left-associative. On the other hand, a+++\--b+c++ will be treated as **((a++)+((\--b)+(c++)))** because the unary post-increment and decrement operators are right to left-associative. An example is defined below to understand how an expression is evaluated using precedence order and associativity? **Expression: x = 4 / 2 + 8 \* 4 - ( 5+ 2 ) % 3** **Solution:** 1\) In the above expression, the highest precedence operator is **()**. So, the parenthesis goes first and calculates first. **x = 4 / 2 + 8 \* 4 - 7 % 3** 2\) Now, **/, \*** and **%** operators have the same precedence and highest from the **+** and **-** Here, we use the associativity concept to solve them. The associative of these operators are from left to right. So, **/** operator goes first and then **\*** and **%** simultaneously. **x = 2 + 8 \* 4 - 7 % 3** **x = 2 + 32 - 7 % 3** **x = 2 + 32 - 1** 3\) Now, **+** and **-** operators both also have the same precedence, and the associativity of these operators lest to the right. So, **+** operator will go first, and then **-** will go. **x = 34 - 1** **x = 33** Type Casting in Java ==================== In Java, **type casting** is a method or process that converts a data type into another data type in both ways manually and automatically. The automatic conversion is done by the compiler and manual conversion performed by the programmer. In this section, we will discuss **type casting** and **its types** with proper examples.  Type casting Convert a value from one data type to another data type is known as **type casting**. Types of Type Casting There are two types of type casting: - Widening Type Casting - Narrowing Type Casting Widening Type Casting Converting a lower data type into a higher one is called **widening** type casting. It is also known as **implicit conversion** or **casting down**. It is done automatically. It is safe because there is no chance to lose data. It takes place when: - Both data types must be compatible with each other. - The target type must be larger than the source type. 1. **byte** -\> **short** -\> **char** -\> **int** -\> **long** -\> **float** -\> **double**  For example, the conversion between numeric data type to char or Boolean is not done automatically. Also, the char and Boolean data types are not compatible with each other. Let\'s see an example. Narrowing Type Casting Converting a higher data type into a lower one is called **narrowing** type casting. It is also known as **explicit conversion** or **casting up**. It is done manually by the programmer. If we do not perform casting then the compiler reports a compile-time error. 1. **double** -\> **float** -\> **long** -\> **int** -\> **char** -\> **short** -\> **byte**  Let\'s see an example of narrowing type casting. In the following example, we have performed the narrowing type casting two times. First, we have converted the double type into long data type after that long data type is converted into int type. Java Control Statements \| Control Flow in Java =============================================== Java compiler executes the code from top to bottom. The statements in the code are executed according to the order in which they appear. However, [Java](https://www.javatpoint.com/java-tutorial)provides statements that can be used to control the flow of Java code. Such statements are called control flow statements. It is one of the fundamental features of Java, which provides a smooth flow of program. Java provides three types of control flow statements. 1. Decision Making statements - if statements - switch statement 2. Loop statements - do while loop - while loop - for loop - for-each loop 3. Jump statements - break statement - continue statement ### Decision-Making statements: As the name suggests, decision-making statements decide which statement to execute and when. Decision-making statements evaluate the Boolean expression and control the program flow depending upon the result of the condition provided. There are two types of decision-making statements in Java, i.e., If statement and switch statement. ### 1) If Statement: In Java, the \"if\" statement is used to evaluate a condition. The control of the program is diverted depending upon the specific condition. The condition of the If statement gives a Boolean value, either true or false. In Java, there are four types of if-statements given below. 1. Simple if statement 2. if-else statement 3. if-else-if ladder 4. Nested if-statement Let\'s understand the if-statements one by one. ### 1) Simple if statement: It is the most basic statement among all control flow statements in Java. It evaluates a Boolean expression and enables the program to enter a block of code if the expression evaluates to true. Syntax of if statement is given below. **if**(condition) {    statement 1; //executes when condition is true   }    Consider the following example in which we have used the **if** statement in the java code. Student.java **Student.java** **public** **class** Student {    **public** **static** **void** main(String\[\] args) {    **int** x = 10;    **int** y = 12;    **if**(x+y \> 20) {    System.out.println(\"x + y is greater than 20\");    }    }      }     **Output:** x + y is greater than 20 ### 2) if-else statement The [if-else statement](https://www.javatpoint.com/java-if-else)is an extension to the if-statement, which uses another block of code, i.e., else block. The else block is executed if the condition of the if-block is evaluated as false. **Syntax:** **if**(condition) {    statement 1; //executes when condition is true   }  **else**{  statement 2; //executes when condition is false   }  Consider the following example. **Student.java** **public** **class** Student {  **public** **static** **void** main(String\[\] args) {  **int** x = 10;  **int** y = 12;  **if**(x+y \