Object-Oriented Programming Concepts - Chapter 10 Abstraction (PDF)
Document Details
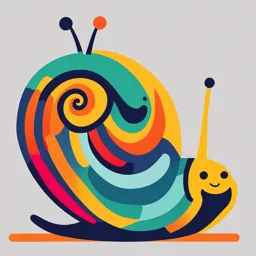
Uploaded by PrudentPsaltery3984
2024
Abdalbasit M. Qadir
Tags
Summary
This document is a chapter on abstraction in object-oriented programming. It covers the concept of abstraction in the context of object-oriented programs, including abstract classes and methods, and gives examples implemented in java. It may be lecture notes or classwork material for a programming course.
Full Transcript
Object Oriented Programming Concepts Chapter 10 — Abstraction — Abdalbasit M. Qadir 2024-2025 December 3, 2024 Abstraction 1 ...
Object Oriented Programming Concepts Chapter 10 — Abstraction — Abdalbasit M. Qadir 2024-2025 December 3, 2024 Abstraction 1 OOP Concepts Revisited December 3, 2024 Abstraction 2 What is an Abstraction? » Data abstraction is the process of hiding certain details and showing only essential information to the user. Abstraction can be achieved with either abstract classes or interfaces » In other words, the user will have the information on what the object does instead of how it does it. The abstract keyword is a non-access modifier, used for classes and methods: » Abstract class: is a restricted class that cannot be used to create objects (to access it, it must be inherited from another class). » Abstract method: can only be used in an abstract class, and it does not have a body. The body is provided by the subclass (inherited from). » An abstract class can have both abstract and regular methods: 3 Class Abstraction ◼ Class abstraction means to separate class implementation from the use of the class. The creator of the class provides a description of the class and let the user know how the class can be used. The user of the class does not need to know how the class is implemented. The detail of implementation is encapsulated and hidden from the user. Class implementation Class Contract is like a black box (Signatures of Clients use the hidden from the clients Class public methods and class through the public constants) contract of the class 4 Syntax of Abstraction? Abstraction is the quality of dealing with ideas rather than events. Syntax abstract class abstract class class_name{ …. } Syntax abstract method abstract return_type function_name(); // No Definition 5 Abstract class ◼ A class that contains the abstract keyword in its declaration is known as the abstract class. ◼ Abstract class may or may not contain abstract methods, i.e., methods without a body ( public void get(); ) ◼ But, if a class has at least one abstract method, then the class must be declared abstract. ◼ If a class is declared abstract, it cannot be instantiated. ◼ To use an abstract class, you have to inherit it from another class, provide implementations to the abstract methods in it. ◼ If you inherit an abstract class, you have to provide implementations to all the abstract methods in it or be abstract itself ◼ If your class doesn’t have any abstract behavior (behavior that changes between different types) then it’s a good candidate for being a concrete class rather than abstract. 6 Abstract Methods » If you want a class to contain a particular method but you want the actual implementation of that method to be determined by child classes, you can declare the method in the parent class as an abstract. A. Abstract keyword is used to declare the method as abstract. B. You have to place the abstract keyword before the method name in the method declaration. C. An abstract method contains a method signature, but no method body. D. Instead of curly braces, an abstract method will have a semi colon (;) at the end. Example: Abstract void absMethod(); 7 abstract method in abstract class ◼ An abstract method cannot be contained in a nonabstract class. If a subclass of an abstract superclass does not implement all the abstract methods, the subclass must be defined abstract. In other words, in a nonabstract subclass extended from an abstract class, all the abstract methods must be implemented, even if they are not used in the subclass. object cannot be created from abstract class: ◼ An abstract class cannot be instantiated using the new operator, but you can still define its constructors, which are invoked in the constructors of its subclasses. For instance, the constructors of GeometricObject are invoked in the Circle class and the Rectangle class. 8 Example 9 abstract class without abstract method ◼ A class that contains abstract methods must be abstract. However, it is possible to define an abstract class that contains no abstract methods. In this case, you cannot create instances of the class using the new operator. This class is used as a base class for defining a new subclass. superclass of abstract class may be concrete: ◼ A subclass can be abstract even if its superclass is concrete. For example, the Object class is concrete, but its subclasses, such as GeometricObject, may be abstract. 10 Concrete VS Abstract ◼ In Java, a concrete class is a class that can be instantiated on its own and can provide implementations for all of its methods, including any inherited abstract methods. ◼ A concrete class can also have its own instance variables and methods. Abstract Class Concrete Class Concrete Class December 3, 2024 Abstraction 11 If class A is parent and class B is child. ◼ The difference between A obj = new B(); and B obj = new B(); Aspect A obj = new B(); B obj = new B(); Reference Type A (Superclass) B (Actual Class) Object Type B B Accessible Methods Only those defined in A All methods in A and B Polymorphism and Full access to B-specific Use Case generalization behavior December 3, 2024 Abstraction 12 Abstract Class Concrete Class An abstract class is declared using abstract A concrete class is not declared using abstract modifier. modifier. An abstract class cannot be directly instantiated A concrete class can be directly instantiated using the new keyword. using the new keyword. An abstract class may or may not contain A concrete class cannot contain an abstract abstract methods. method. An abstract class cannot be declared as final. A concrete class can be declared as final. Implement an interface is possible by not providing implementations of all of the Easy implementation of all of the methods in the interface’s methods. For this a child class is interface. needed.. December 3, 2024 Abstraction 13 14 3 0 15 Example 16 16 What is the Output of the following example? 17 17 When to use Abstract Methods & Abstract Class? ◼ Abstract methods are usually declared where two or more subclasses are expected to do a similar thing in different ways through different implementations. ◼ These subclasses extend the same Abstract class and provide different implementations for the abstract methods. ◼ Abstract class in Java; Always remember that anything abstract (e.g. abstract class or abstract method) is not complete in Java, and you must extend the abstract class and override the abstract method to make use of that class. Remember, Abstraction is very important to design flexible systems. 18 Declaring a method as abstract has two consequences ◼ The class containing it must be declared as abstract. ◼ Any class inheriting the current class must either override the abstract method or declare itself as abstract. 19 Output? 20 21 Differences between Abstraction & Encapsulation Output? 22 Advantages of Abstraction ◼ It reduces the complexity of viewing the things. ◼ Avoids code duplication and increases reusability. ◼ Helps to increase the security of an application or program as only important details are provided to the user. ◼ One advantage of this approach is that we can change the implementation anytime without changing the behavior that is exposed to the user. 23 In Class Exercise ◼ Write a Java program to create an abstract class Animal with an abstract method called sound(). ◼ Create subclasses Lion and Tiger that extend the Animal class and implement the sound() method to make a specific sound for each animal. December 3, 2024 Abstraction 24 In Class Exercise 2 ◼ Write a Java program to create an abstract class Shape with abstract methods calculateArea() and calculatePerimeter(). ◼ Create subclasses Circle and Triangle that extend the Shape class and implement the respective methods to calculate the area and perimeter of each shape. December 3, 2024 Abstraction 25 Abstract Class Product: Create an abstract class Product to represent the common properties and behaviors of all products. It should include the following: Fields: String name, double price. Constructor: A constructor to initialize the name and price. Abstract Methods: double calculateDiscount(): This method will calculate the discount based on the product category. void displayInfo(): This method will display the details of the product, including the discount and final price. Optional: You may add common behaviors, like void applyDiscount() if needed. Subclasses: Create two subclasses, Electronics and Clothing, to represent specific categories of products. Class: Electronics Add an additional field: double warrantyYears to store the warranty period for electronic products. The calculateDiscount() method should implement a 10% discount rule for electronics. The displayInfo() method should display: Name, price, warranty period, discount amount, and final price (price after discount). Class: Clothing Add an additional field: String size to represent the clothing size (e.g., S, M, L). The calculateDiscount() method should implement a 20% discount rule for clothing. The displayInfo() method should display: Name, price, size, discount amount, and final price (price after discount). Main Method: In the main() method: Create at least one object of each subclass (Electronics and Clothing). Call the displayInfo() method for each object to show the details of the product and its discount calculation. December 3, 2024 Abstraction 26