C++ Classes Lecture Notes PDF
Document Details
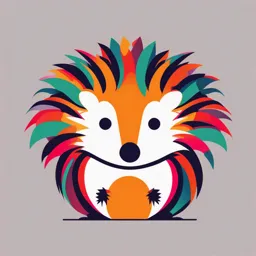
Uploaded by RicherChrysanthemum210
Durham University
Stuart James
Tags
Related
Summary
These lecture notes provide an introduction to C++ classes, covering topics such as data members, member functions, constructors, and destructors. The notes also include examples and discussion on object-oriented programming concepts within C++.
Full Transcript
Systems Programming Lecture 12: C++ Classes Stuart James [email protected] Summative Submission Point Available Test 01. Find Executable Test 02. Find Library Test 03. Find Report PDF in Root Test 04. Makefile Rules Test 05. Code Quality Test 0...
Systems Programming Lecture 12: C++ Classes Stuart James [email protected] Summative Submission Point Available Test 01. Find Executable Test 02. Find Library Test 03. Find Report PDF in Root Test 04. Makefile Rules Test 05. Code Quality Test 06. Generate Output File Test 07. Reverse Example Result ( Includes visualisation ) Test 08. Basic Example Result Timing Test 09. Basic Example Result Memory (Includes memory profile graph ) Test 10. Check All Options Run Through Recap https://PollEv.com/stuartjames Recall: Differences to C Text input and output supported by the iostream library Use three streams for I/O: cin , cout and cerr The > (get from) operator retrieves data from a stream Note: in the #include , although file is iostream.h we drop the.h here New Scoping rule: using namespace std; Global variables/functions can be in spaces. Normally you'd do: std::function() Recall: The new and delete operators Recall: Dynamic memory management allows the programmer to control when memory is allocated and deallocated. The memory is allocated on the heap, while local variables are allocated on the stack C uses malloc() and calloc() for dynamic memory allocation and free() to deallocate it In C++ this has been simplified: new creates space - no need to specify size delete frees up the space Object Classes in C++ A C++ class has data members and member functions Very similar to Java Java fields = C++ data members Java methods = C++ member functions (Remember C++ no garbage collector unlike Java) Object Classes in C++ Typically the class is declared in a header (.h ) file The member functions are then defined in a source file Common file extensions for C++ source:.cpp,.cxx,.cc,.C Object Classes in C++ Remember C had struct which could only have data struct Rectangle{ int width; int height; } In Object Oriented Programming (OOP) we want to operate on "objects", we should be able to encapsulate the data and the methods that operate on it not easily possible with a struct Q: Thinking about Object Oriented Programming what could we want our Rectangle to be able to do? Object Classes in C++ Remember C had struct which could only have data struct Rectangle{ int width; int height; } In Object Oriented Programming (OOP) we want to operate on "objects", we should be able to encapsulate the data and the methods that operate on it not easily possible with a struct Q: Thinking about Object Oriented Programming what could we want our Rectangle to be able to do? Calculate its Area? Example of a C++ class declaration Example of a C++ class declaration In [ ]: 1 #include 2 using namespace std; 3 4 class Rectangle { 5 public: 6 Rectangle () {}; // Default Constructor 7 int Area(); // Example function of area method (Not defined for the moment) 8 private: 9 int width; // Private member variable 10 int height; // Private member variable 11 }; // remember to have this! 12 13 int main(){} Example of a C++ class declaration All lines after public: are public until we get to private / protected public members can be accessed from outside the class private members cannot Example of a C++ class declaration Example of a C++ class declaration In : 1 #include 2 using namespace std; 3 4 class Rectangle { 5 public: 6 Rectangle () {}; // Default Constructor 7 8 private: 9 int width; // Private member variable 10 int height; // Private member variable 11 }; // remember to have this! 12 13 int main(){ 14 Rectangle r; 15 r.width = 10; // No! You cant access private variables 16 r.height = 5; 17 cout