Restaurant Management App Data Flow PDF
Document Details
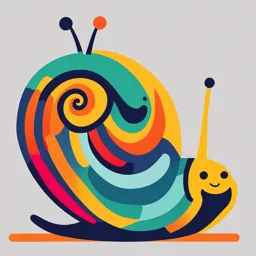
Uploaded by DecisiveGreatWallOfChina1467
Tags
Summary
This document details the end-to-end data flow for a secure authentication system in a restaurant management application. It covers user authentication, token-based authorization, and secure JWT token handling. The document also highlights the differences between synchronous and asynchronous approaches for accessing backend data.
Full Transcript
⭐ 01. End-to-End Data Flow for a Restaurant ** Management App ** ** Over...
⭐ 01. End-to-End Data Flow for a Restaurant ** Management App ** ** Overview ** ** This document outlines the detailed data flow for implementing a secure and efficient ** ** ** ** ** ** authentication system for a restaurant management app. It focuses on the end-to-end process of ** * * ** ** * user authentication, token-based authorization, and interaction with backend services, ensuring both * * * * * ** performance and security. Key components include preliminary checks for existing authentication ** ** ** ** ** * * ** tokens, database or third-party service credential validation, and secure JWT token generation and ** * * ** handling. ** The flow begins with the user initiating a login request, where credentials are securely transmitted ** ** ** ** * * over HTTPS. If an Authorization header is present, the system attempts to validate the provided ** ** ** ** ** ** ** token to avoid unnecessary database queries. In the absence of a valid token, the system queries ** * * ** ** ** the database or a third-party authentication provider to validate the user’s credentials. Upon ** * * ** ** ** successful authentication, a signed JWT is issued, enabling stateless and scalable user session ** ** ** ** ** ** management. ** ** Subsequent API requests use the JWT for authentication, which is verified by backend ** ** ** ** middleware. User roles and permissions are seamlessly incorporated into the token to streamline ** ** ** ** ** ** access control. For secure handling, best practices such as encrypted storage of secrets, HTTPS ** ** ** ** ** * * ** enforcement, and token expiration policies are integrated into the design. The document also ** ** ** ** ** provides appendices detailing the database design (for relational and NoSQL systems), the process ** ** ** ** * * * * ** of shared secret management for JWT signing, and key terminology to ensure clarity and ** ** ** ** ** ** ** ** implementation efficiency. This holistic approach ensures the system is robust, scalable, and ** ** ** ** ** ** ** ** aligned with modern security standards. ** High-Level Data Flow Diagram Synchronous vs. Asynchronous Data Flow ** Data Flow ** ** Step 1: User Initiates Login ** ** Flow Description: ** 1. The user navigates to the login page (web or mobile app). * * 2. The user inputs their credentials (username/email and password). ** ** * * * * 3. These credentials are securely sent to the backend using HTTPS. ** ** ** Key Details: ** Communication is encrypted using SSL/TLS to ensure data confidentiality and integrity. ** ** * * * * Backend receives and validates the request. ** Developer Responsibilities: ** Set up HTTPS by configuring the server with SSL/TLS. ** ** ** ** Redirect all HTTP traffic to HTTPS. ** ** ** ** Implement a frontend that: ** ** Collects user input securely. ** ** Sends the data to the backend via a POST request to an endpoint like /login. ` ` ` ` Validate frontend inputs (e.g., length checks) before sending to the backend. ** ** * * Step 2: Backend Authentication (with Preliminary ** Authorization Check) ** ** Flow Description: ** 1. The backend checks the incoming request for an Authorization header. ** ** ** ** If an Authorization header exists: ** ** * Validate the JWT immediately (see Step 5 for token validation). * ** ** If the token is valid, skip the database query and authorize the user. ** ** * * If the token is invalid or expired, return a 401 Unauthorized response. ** ** ** ` ` ** If no Authorization header exists: ** ** Query the database or third-party authentication service to validate the ** ** ** ** provided credentials. 2. If credentials are valid: ** ** Generate a JWT token with user-specific claims (e.g., user_id , role , and exp ). ** ** ` ` ` ` ` ` ** Sign the token using a private key or shared secret. ** * * * * Return the token securely to the client. 3. If credentials are invalid: ** ** Return an error response (e.g., 401 Unauthorized ). ** ** ` ` ** Key Details: ** The preliminary check optimizes performance by skipping unnecessary database queries if ** ** the user is already authenticated. The token’s payload includes: ** ** ** ` user_id (unique user identifier). ` ** * * ** ` role (e.g., manager, employee). ` ** * * * * ** ` exp (expiration time). ` ** * * ** Developer Responsibilities: ** Implement logic to check for the Authorization header and handle token validation ** ** ** ** efficiently. If no Authorization header exists: ** ** Write a query to fetch user data by username/email for validation. ** ** ** ** Implement secure password hashing for comparisons. ** ** ** Generate and sign JWT tokens securely using a cryptographic library. ** Return the token in the response, optionally setting it as a secure HTTP-only cookie. ** ** ** Step 3: Token Storage on the Client ** ** Flow Description: ** 1. The client receives the JWT from the backend after login. ** ** ** ** 2. The JWT is securely stored in an HTTP-only cookie or other secure storage mechanisms. ** ** ** ** ** Key Details: ** Use secure storage mechanisms to protect the token: ** ** Web: Store in an HTTP-only cookie. ** ** Mobile: Use platform-specific secure storage (e.g., Android Keystore, iOS Keychain). ** ** * * * * ** Developer Responsibilities: ** Ensure the frontend securely stores the token in a secure HTTP-only cookie. ** ** ** ** Implement measures to mitigate risks like Cross-Site Scripting (XSS) and token theft. ** ** ** ** ** Step 4: User Makes Authenticated Requests ** ** Flow Description: ** 1. For every API call requiring authentication, the client: ** ** Retrieves the JWT from its stored HTTP-only cookie or secure storage. ** ** ** ** Includes the JWT in the Authorization header. ** ** ` ` Example: Authorization: Bearer. ** ` ` ** ** Key Details: ** The JWT retrieval process ensures secure transmission without exposing the token to ** ** unauthorized scripts or users. ** Developer Responsibilities: ** Configure the frontend to automatically retrieve the JWT from the secure cookie. ** ** ** ** Attach the JWT to API requests via the Authorization header. ** ** ` ` ** Step 5: Backend Validates JWT and Authorizes Requests ** ** Flow Description: ** 1. The backend middleware intercepts the request and retrieves the JWT from the ** ** ** ** ` Authorization header. ` 2. The middleware performs token validation: ** ** ** Verifies the signature using the private key or shared secret. ** * * * * Checks token claims, such as exp (expiration) and iat (issued time). ** ** ` ` ` ` Decodes the token to extract user details (e.g., user_id , role ). ** ** ` ` ` ` 3. If the token is valid, the user is authorized, and the request proceeds. ** ** 4. If the token is invalid or expired, return an error response (e.g., 401 Unauthorized ). ** ** ** ** ` ` 5. Attach the user details to the request context for further processing by the backend. ** ** ** ** ** Key Details: ** Token validation ensures the request originates from an authenticated user. Attaching user details to the request enables role-based access control (RBAC). ** ** ** Developer Responsibilities: ** Implement middleware to: ** ** Extract the token from the Authorization header. ` ` Validate the token's signature and claims. ** ** ** ** Attach user details to the request context. ** ** ** ** Implement robust error handling for expired or invalid tokens. ** ** ** Step 6: Accessing the Black Box ** The approach for accessing the black box depends on the scale and traffic requirements of the ** ** system. Below are two options: synchronous (for small-scale systems) and asynchronous (for high- ** ** ** ** traffic, scalable systems). ** Option 1: Synchronous Approach ** ** Flow Description: ** 1. After the backend validates the request, it makes a synchronous API call to the black box. ** ** ** ** 2. The black box receives parameters such as: ** ** ** User availability (e.g., days of the week and time ranges). ** ** User credentials (if required). ** 3. The black box processes the request and returns a response in real-time. ** ** 4. The backend uses the response to complete the original user request and sends it back to the client. ** Use Case: ** Suitable for low-traffic applications, such as the given scenario of 100 restaurants with 100 ** customers each. ** The user traffic is small and manageable without risking timeouts. ** ** ** Key Considerations: ** The request-response cycle is simple and completed within a single transaction. If the black box is unavailable or takes too long to respond, the user may encounter ** ** ** timeout errors (e.g., HTTP 500 or 503 Service Unavailable). ** ** ** ** ** ** Developer Responsibilities: ** Implement a synchronous API integration with the black box. ** ** Handle errors gracefully: Return appropriate error messages to the client for timeout or unavailability. ** ** ** ** Log failures for monitoring and debugging. Ensure timeouts are configured for the API calls to prevent hanging requests. ** ** ** Option 2: Asynchronous Approach (High-Traffic Systems) ** ** Flow Description: ** 1. After the backend validates the request, it creates a task to invoke the black box with the ** ** ** ** following parameters: ** User availability (e.g., days of the week and time ranges). ** ** User credentials (if required). ** 2. The task is added to a task queue, such as: ** ** ** Kafka (for high durability and distributed messaging). ** ** AWS SQS, RabbitMQ, or IBM MQ (for simpler setups). ** ** ** ** ** 3. A worker process monitors the task queue and retrieves tasks as they are added. ** ** 4. The worker invokes the black box asynchronously and processes the response. ** ** 5. Once the worker receives a response from the black box: ** ** It updates the backend system with the results (e.g., stores it in a database or sends a ** ** notification to the user). ** Use Case: ** Ideal for high-traffic applications where synchronous calls may result in timeouts or ** ** ** ** overwhelm the black box. ** ** Enables load leveling by allowing tasks to be processed independently of user requests. ** Key Considerations: ** The user does not receive the response immediately; instead, the system must notify them when the process completes. Requires additional infrastructure for the task queue and worker processes. ** ** ** ** The black box can be invoked more flexibly, as tasks are queued and processed when ** ** resources are available. ** Developer Responsibilities: ** Implement the task creation logic in the backend: Enqueue the task with relevant parameters, such as user availability and user ** ** ** credentials. ** Set up a task queue system, such as: ** ** ** Kafka (for scalability and distributed durability). ** ** AWS SQS, RabbitMQ, or IBM MQ (for simpler integrations). ** ** ** ** ** Develop a worker process to: ** ** Poll the task queue. Invoke the black box asynchronously with the parameters. ** ** Handle the response and update the system (e.g., store results in a database or trigger a user notification). Monitor and maintain the task queue and worker processes to ensure scalability and fault ** ** ** ** tolerance. ** Key Differences Between the Approaches ** Feature Synchronous Approach Asynchronous Approach ** Use Case ** Low-traffic, small-scale systems High-traffic, large-scale systems ** Response Time ** Real-time Delayed ** Complexity ** Simple Higher (requires task queue setup) ** Timeout Risk ** Higher Lower (tasks queued for processing) ** Black Box Overload Risk ** Higher Lower (tasks processed asynchronously) ** Infrastructure ** Minimal Requires task queue and worker setup ** Appendix ** ** Key Terms ** **~ Schema: The structure of a database, defining tables, fields, and relationships. ~ ** **~ Data Model: A conceptual representation of how data is stored and organized. ~ ** **~ Database Table: A collection of related data in rows and columns (used in relational databases). ~ ** **~ Normalized Schema: A normalized schema in a database is a schema design that organizes ~ ** ** ** data into structured, related tables to reduce redundancy and improve data integrity. This approach follows the principles of database normalization, which involve dividing data into ** ** multiple tables and defining relationships between them using keys (primary keys and foreign keys). ℹ See [[ Understanding Normalized Schema: Definition, Characteristics, and Examples. ]] **~ Denormalized Schema: A denormalized schema in a database is a design that combines ~ ** ** ** related data into fewer tables or structures to optimize read performance by reducing the need for joins. In a denormalized schema, data redundancy is intentionally introduced to simplify queries and improve the efficiency of data retrieval, often at the cost of increased storage and potential data inconsistency. See [[ Understanding Denormalized Schema: Definition, Characteristics, and Use Cases. ]] ** Database Design ** ** Relational Database ** *** Normalized Schema: * ** ` users table: ` ` user_id (Primary Key), ` ` username , ` ` email , ` ` password_hash , ` ` role_id ` (Foreign Key → roles.role_id ). * * ** ** ` ` ` roles table: ` ` role_id (Primary Key), ` ` role_name , ` ` permissions ( JSON or related table ). ` ** ** ** ** ** NoSQL Database ** *** Denormalized Schema: * ** ℹ Embed role details directly in the user document: ` ` ```json { "user_id": "1", "username": "jdoe", "email": "[email protected]", "password_hash": "hashed_pwd_abc123", "role": { "role_name": "Manager", "permissions": { "view_shifts": true } } } ``` ** Shared Secret and Private Key Management ** See [[ Shared Secret and Private Key Management. ]]