Socket Programming PDF
Document Details
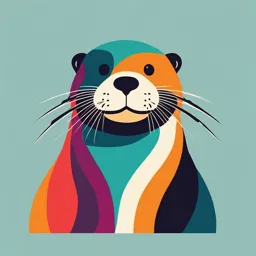
Uploaded by LuxuryAbundance
Algonquin College
Tags
Summary
This document provides an overview of socket programming, focusing on Java implementation. It details socket pairs, functionality, and comparisons between TCP and UDP. Examples of client and server implementations are included.
Full Transcript
SOCKET PROGRAMMING* Socket Pairs Definition: A combination of the source IP address and source port number, or the destination IP address and destination port number. Purpose: Used to identify the server and service being requested by the client. Socket Pair Example: (19...
SOCKET PROGRAMMING* Socket Pairs Definition: A combination of the source IP address and source port number, or the destination IP address and destination port number. Purpose: Used to identify the server and service being requested by the client. Socket Pair Example: (192.168.1.5:1099, 192.168.1.7:80). Functionality: Identification: Allows multiple processes on a client and multiple connections to a server to be distinguished. Return Address: The source port number serves as the return address for the requesting application. Tracking: The transport layer manages and keeps track of active sockets. In Java network programming, the two key classes from the java.net package used for creating server and client programs are: //INSERT THE TWO DIAGRAM HERE Purpose: : Used by the server to listen for incoming client connections on Usage: Create an instance of ServerSocket and call accept() to wait : for client connections. Purpose: Used by both clients and servers to establish a connection and Usage: For the client, create an instance of Socket to connect to the server's IP address and port. For the server, use the Socket instance obtained from the accept() method of ServerSocket to communicate with the client. Here's a summary of how to establish a server-client connection in Java using ServerSocket and Socket: //INSERT DIGRAM HERE For the Server: 1. Create a ServerSocket: This binds the server socket to a specific port number where it will listen for incoming connections. 2. Listen for Connections: The server waits for a client to connect. Once a connection request is received, it returns a Socket object representing the connection to the client. 3. Communicate with the Client: Use the clientSocket to get input and output streams for reading from and writing to the client: InputStream in = clientSocket.getInputStream(); OutputStream out = clientSocket.getOutputStream(); For the Client: 1. Request a Connection: Socket socket = new Socket(serverAddress, portNumber); The client creates a Socket object to connect to the server using the server's IP address (or hostname) and port number. 2. Use the Summary: Server Side: 1. Create a ServerSocket and bind it to a port. 2. Call accept() to wait for and accept client connections. 3. Use the Socket object obtained from accept() for communication. Client Side: 1. Create a Socket to connect to the server's address and port. 2. Use the Socket object for communication with the server. Make sure the server is running and listening before the client attempts to connect. Here’s a comparison between Stream Sockets and Datagram Sockets: Stream Socket: Protocol: Uses TCP (Transmission Control Protocol). Connection: Provides a dedicated point-to-point channel between a client and server. Reliability: Lossless and reliable; ensures data is delivered correctly and in order. Ordering: Data is received in the same order it was sent. Use Case: Suitable for applications requiring reliable, ordered communication (e.g., web browsing, file transfer). Datagram Socket: Protocol: Uses UDP (User Datagram Protocol). Connection: No dedicated point-to-point channel; connectionless. Reliability: May lose data; not guaranteed to be 100% reliable. Ordering: Data may arrive out of order or not at all. Use Case: Suitable for applications where speed is more critical than reliability (e.g., streaming, online gaming). Summary: Stream Sockets (TCP): Reliable, ordered delivery, connection- oriented. Datagram Sockets (UDP): Unreliable, unordered delivery, connectionless. In UDP socket programming using Java, the primary classes from the java.net package are: DatagramPacket: Represents a data packet in UDP communication. It is used to send and receive data over a network. Each DatagramPacket contains the data, its length, and the destination address. DatagramSocket: Manages the transmission and reception of DatagramPacket objects. It provides methods for sending and receiving datagrams. Basic Usage: 1. Server: Client: 2. Server (to receive data): ) 3. Handling Data: Server: 0 Client: 4. Closing the DatagramSocket: Key Points: DatagramSocket is used to create a socket and manage communication. DatagramPacket encapsulates the data to be sent or received and includes information such as the data bucer, length, and destination address. UDP is connectionless, so each packet is independent of others, and you must handle any necessary sequencing or error-checking in your application. DatagramPacket Class The DatagramPacket class is used in UDP communication for sending and receiving data packets. Here’s an overview of its key features: Constructors 1. Receiving Data: : : byte[] bucer = new byte; DatagramPacket packet = new DatagramPacket(bucer, bucer.length); socket.receive(packet); // Receives data into the bu>er 2. Sending Data: Key Methods 1. Returns the data buffer of the packet. Usage: 2. Description: Returns the length of the data contained in the packet. This could be the length of the data received or the length of the data to be sent. Usage: 3. Sets the data buffer for this packet. This method is often Usage: 4. Sets the length of the data in this packet. This is useful to define how much of the data buffer should be considered valid. Usage: Example Usage: Server Example: 0 Client Example: ) These constructors and methods allow you to eciciently handle UDP communication by creating packets, setting or getting data, and specifying packet properties. DatagramPacket Class Overview The DatagramPacket class in Java represents a datagram packet used for connectionless communication via UDP. Here’s a summary of its key aspects: Purpose: Represents a datagram packet in a connectionless packet delivery service. Each packet is routed independently from one machine to another based on the packet's header information. Key Characteristics: Connectionless: There is no persistent connection between sender and receiver. Routing: Messages are routed based on information in the packet itself, such as destination address and port. Constructors 1. Receiving Data: Purpose: : : The length of the buffer. 2. Sending Data: Purpose: and port. : The byte array containing the data to be sent. : The length of the data to be sent. : : The destination port number. Key Methods 1. byte[] getData() Description: Retrieves the byte array that holds the packet's data. Usage: Used to access the data received in the packet. 2. int getLength() Description: Returns the length of the data in the packet. Usage: Determines how much of the data bucer contains valid data. 3. void setData(byte[] buf) Description: Sets the data bucer for this packet. Usage: Prepares the packet with new data. 4. void setLength(int length) Description: Sets the length of the data in the packet. Usage: Defines how much of the bucer should be considered valid data. Example Usage Server Example: 0 Client Example: ) and received, encapsulating all necessary information for routing and handling packets. DatagramSocket Class Overview The DatagramSocket class in Java is used for sending and receiving datagram packets over UDP. It serves as the endpoint for packet-based communication, with each packet individually addressed and routed. Key Characteristics: Connectionless: No established connection between sender and receiver; each packet is sent independently. Routing: Packets may be routed dicerently and arrive in any order, as they are independently addressed. Multiple Packets: Packets sent from one machine may take dicerent routes and arrive at dicerent times. Constructors 1. Purpose: that is bound to a specific port on the local host. : used for receiving packets. Example: ); 2. Purpose: Parameters: No parameters; the port is assigned automatically. Example: Key Methods void send(DatagramPacket packet) Description: Sends a datagram packet through the socket. Usage: Used to transmit packets to a remote machine. void receive(DatagramPacket packet) Description: Receives a datagram packet from the socket. Usage: Used to receive packets from a remote machine. void close() Description: Closes the socket and releases any resources associated with it. Usage: Must be called when the socket is no longer needed. Example Usage Server Example: ) { ); 0 ; } The Client Example: ) { ; ), 0, } In summary, is used for both server and client implementations in UDP communication, handling the sending and receiving of instances. DatagramSocket class in Java provides methods for sending and receiving datagram packets over UDP. It is fundamental for implementing UDP-based communication. Constructors DatagramSocket() Purpose: Creates a client DatagramSocket that is bound to any available port on the local host. Throws: java.net.SocketException if the socket cannot be opened or bound. Purpose: that is bound to a specific : Throws: bound. Example: ); Key Methods void send(DatagramPacket p) Purpose: Sends a datagram packet from this socket to the specified address and port. Parameters: p: The DatagramPacket containing the data to be sent. Usage: Used to transmit data to a remote machine. Example: ); Purpose: : The filled with data upon return. Usage: Used to receive data from a remote machine. Example: Summary The DatagramSocket class is essential for UDP communication, handling datagram packet transmission and reception. It allows the creation of both client and server sockets with the ability to send and receive data packets. Sending and Receiving with DatagramSocket Here's a concise guide to sending and receiving data using DatagramSocket in Java. Sending Data 1. Create a DatagramPacket for the data: Initialize a DatagramPacket with the data to be sent, the length of the data, the receiver's address, and the port number. 2. Send the packet: Use the send(DatagramPacket p) method of DatagramSocket to transmit the packet. ) { ; ); } Receiving Data 1. Create an empty to receive data: 2. Receive the packet: Use the method of to fill the packet with data from the sender. Example: ) { ); 0 } Summary Sending Data: Create a DatagramPacket with the data, address, and port, then use send(). Receiving Data: Create an empty DatagramPacket, then use receive() to fill it with data. Datagram Programming Overview In datagram programming, both the client and server use DatagramSocket for sending and receiving packets, which is dicerent from stream socket programming. Here’s a brief overview: Key Points No ServerSocket: Unlike stream sockets (TCP), which use ServerSocket to listen for incoming connections, datagrams do not require a ServerSocket. Both client and server use DatagramSocket directly. Connectionless Protocol: Datagram programming uses UDP (User Datagram Protocol), which is connectionless. This means there is no need to establish a connection before sending or receiving data. Each datagram is an independent packet. Client and Server Similarities: Both client and server programs create and use DatagramSocket objects. The primary dicerence is in how they use the sockets: Server: Binds to a specific port and listens for incoming packets. Client: Sends packets to a specified port on a server. Example Workflow Server: 1. Create DatagramSocket: Bind to a port to listen for incoming datagrams. 2. Receive Data: Use receive() method to get datagrams from clients. 3. Process Data: Extract and handle data from received packets. Client: 1. Create DatagramSocket: Create a socket (can use any available port). 2. Send Data: Use send() method to send datagrams to the server. 3. Receive Data (Optional): Optionally, handle responses from the server. Example Code UDP Server Example: ) { ); ); 0 } UDP Client Example: ) { ; ); } Summary No ServerSocket: Datagram programming does not require ServerSocket. Both the client and server use DatagramSocket. Connectionless: Each packet is independent; no connection is maintained. Direct Communication: Both ends use DatagramSocket for sending and receiving datagrams.