Common Software Vulnerabilities PDF
Document Details
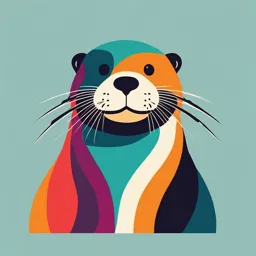
Uploaded by RejoicingGlacier
Tags
Summary
This document details common software vulnerabilities and provides practical examples, best practices, and coding techniques for secure programming. It includes discussions of key concepts such as buffer overflow, SQL injection, and cross-site scripting. The document also covers concepts like Encryption and remote desktop.
Full Transcript
COMMON SOFTWARE VULNERABILITIES 1. Buffer Overflow: Occurs when a program writes more data to a buffer than it can handle, allowing attackers to overwrite adjacent memory and execute malicious code. Mitigation: input validation and boundary checks, using safer programming languages that includ...
COMMON SOFTWARE VULNERABILITIES 1. Buffer Overflow: Occurs when a program writes more data to a buffer than it can handle, allowing attackers to overwrite adjacent memory and execute malicious code. Mitigation: input validation and boundary checks, using safer programming languages that include built-in safeguards, Regular security audits, code reviews, and penetration testing. 2. SQL Injection: Exploits improper handling of user input in SQL queries, enabling attackers to manipulate databases and gain unauthorized access to sensitive data. Coding Practices: Parameterized Queries (Prepared Statements), Input Validation and Sanitization, Least Privilege, Escaping Special Characters, Web Application Firewalls (WAF), and Regular Security Testing 3. Cross-Site Scripting (XSS): Attackers inject malicious scripts into web pages viewed by others, often leading to the theft of sensitive data or session hijacking. Coding Practices: Input Validation and Output Encoding, GTTP Only and Secure Cookies, Content Security Policy (CSP), HTTP Headers, Avoiding inline Scripts, and Regular Security Testing. 4. Cross-Site Request Forgery (CSRF): Allows an attacker to perform unauthorized actions on behalf of a victim user, tricking the browser into making unintended requests that the attacker can manipulate. [nanloloko] Security Measures: CSRF Tokens, SameSite Attribute, HTTP Referrer Header, Logout After Inactivity, HTTP Headers, and Regular Security Testing. 5. Privilege Escalation: Vulnerabilities that allow attackers to gain higher access levels within a system than they should have, leading to increased control over the system. a. Vertical Privilege Escalation: when a standard user attempts to gain the privileges of a higher-level user b. Horizontal Privilege Escalation: an authenticated user (standard/privileged) attempts to gain access to another user’s account within the same privilege level. Coding Practices: Principle of Least privilege, regular Updates and Patching, Secure Configuration, Strong Authentication and Authorization, Auditing and Monitoring, and Regular Security Testing. 6. Remote Code Execution (RCE): Enables attackers to execute arbitrary code on a remote system, often through network services. Best Practices: Input Validation and Sanitization, Secure Coding Practices, Least privilege, Use Sage APIs, Patch Management, Web Application Firewall (WAF), and Security Testing. 7. Denial of Service (DoS): Cyber-attack that aims to disrupt the availability of a computer system, network, or service. Attackers flood a system with excessive requests, rendering it inaccessible or significantly degrading its performance. Mitigating Strategies: Network and Infrastructure Hardening, DoS Mitigation Technologies, Traffic Filtering and Rate Limiting, Redundancy and Scalability, Incident Response Planning, and Monitoring and Alerting. 8. Authentication Bypass: Allows an attacker to gain unauthorized access to a system or resource without providing valid credentials or going through the intended authentication process. Prevention Techniques: Secure Coding Practices, Regular Software Updates, Security Audits and Penetration Testing, Use Secure Programming Languages, and Firewalls and Web Application Firewalls (WAFs). ENCRYPTION Step 1: Install cryptography using pip 1. Open Command Prompt (Windows) or Terminal (macOS/Linux): 2. Install the cryptography package: Run the following command to install the cryptography package: bash Copy code pip install cryptography Step 2: Using cryptography in IDLE 1. Open IDLE: Go to the Python installation folder and open IDLE, or find it through your system search. 2. Write and run your code: You can now write and execute Python code that uses the cryptography package. 3. Run the code: Press F5 in IDLE to run your script. Troubleshooting Ensure pip is installed: You can check if pip is installed by running: bash Copy code pip --version If it's not installed, you may need to install it or update your Python installation. Ensure Python is added to PATH: If you're having trouble using pip commands, make sure that Python and pip are added to your system’s PATH environment variable. You can reinstall Python and select the option to "Add Python to PATH" during installation. Caesar Cipher In cryptography, also known as Caesar’s cipher, the shift cipher, Caesar’s code, or Caesar shift, is one of the simplest and most widely known encryption techniques. A type of substitution cipher in which each letter in the plaintext is replaced by a letter some fixed number of positions down the alphabet. The method is named after Julius Caesar. Is often incorporated as part of more complex algorithm such as the Vigenere cipher, and still has modern application in the ROT13 system. It has no communication security and it can be easily broken even by hand. Mathematical Base: x = letter, n = shift Encryption En (x) = (x+n) mod 26 | Decryption Dn (x) = (x-n) mod 26 Weaknesses Can be easily broken even in a ciphertext-only scenario. Two situations can be considered: 1. An attacker knows (or guesses) that some sort of simple substitution cipher has been used. - the cipher can be broken using the same techniques as for a general simple substitution cipher, such as frequency analysis or pattern words 2. An attacker knows that a Caesar cipher is in use, but does not know the shift value. - breaking is even more straightforward. Since there are only a limited number of possible shifts (26 in English), they can each be tested in turn in a brute force attack. Solution: use multiple shift keys. Shift by 3, 5, and 7 Internet Infrastructure Internet a vast, global network of computers and devices that communicate using standardized protocols. The global system that enables data exchange between devices. Consists of physical components and protocols. Supports online services, websites, and communication. The building blocks of the Internet Fiber Optic Cables – high-speed cables made of glass fibers that transmit data as light. Form the physical backbone of the internet, transmitting large amounts of data at the speed of light over long distances with minimal signal loss. These cables are critical for intercontinental communications. How They Work: Data is transmitted as pulses of light through glass or plastic fibers. Each pulse represents a bit of data. The core of the cable carries the light, while cladding keeps the light inside through total internal reflection. Submarine Cables – fiber optic cables are often laid under oceans to connect continents. Specialized ships deploy these cables, ensuring they can withstand harsh underwater environments. Data Centers – are massive facilities filled with servers and networking equipment, they host websites, applications, and user data, allowing us to access information instantly. Cloud Computing – cloud services like AWS, Google Cloud, and Microsoft Azure revolutionized how we store and process data. Instead of relying on personal hardware, users and businesses rent computing power and storage space in these data centers. Satellites – provide internet access in remote and underserved regions, supplementing the physical infrastructure of fiber optic cables. Low-Earth Orbit (LEO) Satellites – companies like SpaceX’s Starlink are deploying constellations of LEO satellites to deliver high- speed internet globally, including areas where laying fiber optics is impractical. ISPs – provide internet access to individuals, businesses, and governments. They maintain the infrastructure that connects your devices to the broader internet. Types of ISPs – Mobile (4G, 5G), Broadband (cables, DSL), and Fiber. Routers – direct data packets between your local network and the internet. Switches – connect multiple devices within a local network, allowing them to communicate. Manage internal traffic. Modems – convert digital signals from your device into analog signals that travel over telephone lines or cable, and vice versa, enabling your connection to the internet. Servers – are computers designed to process requests and deliver data to other computers, known as clients. Web servers host websites, application servers run programs, and file servers store documents and media. Data Packets – data on the internet is transmitted in small chunks called packets. IP Addresses – are unique identifiers assigned to every device connected to the internet. These addresses allow devices to find each other and communicate. Types – IPv4 (4 groups of numbers | 192.168.0.1 | offers 4.3B addresses), IPv6 (longer, 8 groups by colon| offers 340 undecillion addresses) Public and Private - Public IP addresses are accessible from anywhere on the internet, while private IPs are used within local networks. DNS (Domain Name System) - translates human-readable domain names (like google.com) into IP addresses that computers use to locate each other. Network Protocols and Services - Network protocols are the set of rules that dictate how data is transmitted across networks. They ensure that devices, regardless of their operating systems or hardware, can communicate effectively. TCP/IP (Transmission Control Protocol/Internet Protocol) – is the fundamental suite of protocols that underpins the internet, enabling reliable communication between devices. TCP (Transmission Control Protocol) – ensures reliable data transmission by breaking data into smaller packets, sending them over the network, and reassembling them at the destination. IP (Internet Protocol) – handles the routing of packets across different networks to ensure they reach their destination. Addressing: Every device on a network has a unique IP address that identifies it. Layered Model (OSI vs. TCP/IP) OSI Model: 7 Layers: Application, Presentation, Session, Transport, Network, Data Link, Physical. TCP/IP Model: 4 Layers: Application, Transport, Internet, Network Interface. Packets and Encapsulation – data is encapsulated as it moves through the layers of the network model, which involves wrapping data with protocol-specific information HTTP/HTTPS (Hypertext Transfer Protocol/Secure) - HTTP is the protocol used for transferring web pages, while HTTPS is the secure version that encrypts the data transmitted between browsers and servers. FTP (File Transfer Protocol) – is used to transfer files between computers over the internet. SMTP (Simple Mail Transfer Protocol) – is the protocol used for sending emails. Port Numbers and Services – each network service operates on a specific port number, which helps identify the service that should handle incoming data. Common Port Numbers: Port 80: HTTP | Port 443: HTTPS | Port 25: SMTP Firewalls: Firewalls use port numbers to control traffic; they can block or allow data based on these numbers. World Wide Web (WWW) – is an application layer that operates on top of the internet, enabling users to browse, interact, and access information through websites. Web Browsers – are software applications that allow users to access and navigate websites. HTTP/HTTPS – protocol used by browsers to communicate with web servers and retrieve web pages. HTTP Status Codes: 200 OK: Request successful | 404 Not Found: Requested resource could not be found. | 500 Internal Server Error: The server encountered an error. The three core technologies that create web pages, enabling structure, style, and interactivity HTML (Hypertext Markup Language) – provides the structure of web pages using elements like headings, paragraphs, links, and images. CSS (Cascading Style Sheets) – controls the presentation and layout of web pages, including colors, fonts, and spacing. JavaScript – adds interactivity to web pages, enabling features like dynamic content, form validation, and animations. Web servers – are specialized computers that store, process, and deliver web pages to users via HTTP or HTTPS when requested. Popular Web Servers: Apache, Nginx, Microsoft IIS (Internet Information Services) Search Engines – help users find specific information on the web by indexing web pages & ranking them according to relevance. Caching – browsers store copies of web data (e.g., images, CSS files) in temporary storage, known as cache, to improve load times for repeat visits. Cookies – small text files stored on the user's computer by websites to remember session information or user preferences. What is Cybersecurity – is the practice of protecting systems, networks, and data from unauthorized access, attacks, or damage. Common Cyber Threats and Vulnerabilities 1. Malware – refers to malicious software that is designed to infiltrate, damage, or exploit a system without the user’s consent. a. Viruses – attach themselves to legitimate software and replicate when the infected software is run. They require a host program to propagate. b. Worms – standalone programs that replicate themselves and spread through networks without needing a host program, causing extensive damage quickly. c. Trojan Horses – disguised as legitimate software, these programs trick users into installing them, after which they exploit or compromise the system. d. Ransomware – locks users out of their systems or encrypts their data, demanding payment (usually in cryptocurrency) for restoration or access. e. Spyware – secretly monitors user activity, gathering sensitive data like passwords, credit card details, and browsing history, which is then sent to attackers. f. Adware – unwanted software that displays intrusive ads on devices, sometimes leading to other types of malware. 2. Phishing – attackers impersonate legitimate entities through deceptive emails, messages, or websites to trick users into revealing sensitive information such as passwords, financial data, or social security numbers. How to Identify if an email is fake 1. Check the sender's email address 2. Look for generic greetings 3. Watch for spelling and grammar mistakes 4. Beware of urgent language 5. Examine links carefully 6. Attachments from unknown sources (may contain malware) 3. Social Engineering - refers to the manipulation of people into divulging confidential information or performing actions that compromise security, often using psychological techniques. 4. Denial of Service (DoS – single machine) and Distributed Denial of Service (DDoS – multiple system) Attacks – flood a target (usually a website or service) with overwhelming traffic, rendering it slow or entirely unavailable to users. 5. Zero-Day Vulnerabilities – refer to security flaws that attackers exploit before the software vendor becomes aware or before a patch is available. Dangerous due to, giving the vendor zero days to fix the issue before it is exploited. 6. Insider Threats - Edward Snowden’s unauthorized leak of classified NSA documents. Cases of employees stealing trade secrets or sabotaging company systems. Vulnerabilities – are weaknesses in software, hardware, or security processes that can be exploited by cyber attackers. Common Vulnerabilities Buffer Overflow: When a program writes data beyond the limits of its allocated memory, potentially allowing attackers to execute arbitrary code. SQL Injection: Attackers insert malicious code into a database query, often leading to unauthorized access or data breaches. Unpatched Systems: Systems that have not received updates or security patches are vulnerable to exploitation. Attack vectors – refer to the paths or methods attackers use to infiltrate a system or network. Examples: Email: Phishing attempts or malware-laden attachments. Websites: Drive-by downloads or malicious code. USB Drives: Infected removable media devices. Unprotected Networks: Public Wi-Fi with weak or no encryption. Principles of Cybersecurity Cybersecurity is built upon fundamental principles that guide the protection of systems, networks, and data. These principles aim to ensure that information remains secure from unauthorized access, tampering, and disruptions. The core principles are represented by the CIA Triad, which focuses on Confidentiality, Integrity, and Availability. 1. Confidentiality – ensures that sensitive information is only accessible to authorized individuals. 2. Integrity – ensures that data remains accurate and reliable, preventing unauthorized changes or corruption. 3. Availability – ensures that systems, networks, and data are accessible when needed, minimizing downtime. Identification and Authorization Authentication: The process of verifying a user’s identity, typically using credentials such as passwords, biometrics (e.g., fingerprints or facial recognition), or security tokens. Authorization: Once a user is authenticated, authorization determines what they are allowed to do, specifying their level of access to resources (files, databases, etc.). Encryption – the process of encoding data to prevent unauthorized access, ensuring that only authorized parties with the correct decryption key can read it. Strong password – the first line of defense against unauthorized access. It should be long (at least 12 characters), complex (using uppercase and lowercase letters, numbers, and symbols), and unique for every account. Password Managers – tools like LastPass or Bitwarden help users store and manage complex passwords securely. Password managers generate and remember strong passwords, so users don’t have to. Multifactor Authentication (MFA) – adds an extra layer of security beyond just a password by requiring additional verification, like a fingerprint or a one-time code sent via SMS. Safe Browsing Identifying Secure Websites: recognize secure websites by looking for HTTPS (not just HTTP) and a padlock icon in the address bar, indicating that the website uses encryption to protect data. Avoiding Suspicious Links Browser Safety Practices: avoid downloading unknown files or clicking on unsolicited ads or links. Software Updates Why Regular Updates are Important? Outdated software often contains vulnerabilities that hackers can exploit. Keeping software and operating systems up-to-date ensures that known security flaws are patched. Attackers can exploit old versions of browsers or apps, but simple updates can prevent such risks Phishing Awareness Recognizing Phishing Attempts: Phishing attacks often use fake emails or websites that appear legitimate but are designed to steal sensitive information. Key Indicators of Phishing: Look for misspellings, unusual senders, urgent or threatening language, or suspicious URLs. Public Wi-fi Safety Risks of Public Wi-Fi: Public Wi-Fi networks are often unencrypted, making it easier for attackers to intercept data. This is especially risky when accessing sensitive accounts like banking or email on public networks. VPNs (Virtual Private Networks): encrypt internet traffic, providing a secure connection even on public Wi-Fi Remote Desktop Software Remote PC – a technology that allows you to access and control your computer from a different location using another device. This capability enables you to manage files, run applications, and perform tasks on your primary PC remotely, providing flexibility and convenience for both personal and professional use. Remote desktop software – captures a device's screen and mouse and keyboard inputs and transmits them to another device, where a user can view or control it remotely. Tech support professionals often use remote desktop connectivity to troubleshoot live fixes on a client's computer. What is the importance of remote desktop connection? Remote Desktop Services provide multiple advantages to businesses like increased productivity and efficiency, reduced travel costs, fast resolution to technical problems, etc. The significant benefit of RDS is that there's only one machine to manage for multiple users to access locally or remotely. Benefits of remote control software[MSEB] 1. More efficient troubleshooting. Troubleshooting IT 2. Streamlined remote working.... issues is much more efficient when tech support can 3. Easier collaboration.... use remote control software.... 4. Better network security. Software: TeamViewer, Splashtop, Quick Assist, GoToMyPC, RemotePC, Remote Desktop Protocol, Chrome Remote Desktop, Microsoft Remote Desktop, AnyViewer Remote Desktop, AnyDesk, DesktopNow Advantages of Remote Access [FFFCS] Disadvantages of Remote Access [SVHFC] 1. Flexible Access 1. Security Issues 2. Flexible Set-Up and Costs 2. Version Problems and Data Liabilities 3. Full Control on Authorization and Access 3. Hardware Issues Still Need On-Site Work 4. Centralized Storage and Backups 4. Difficulties with Work Culture 5. Shared Resources; Greater Efficiency and 5. Complex Software and Hardware Collaboration Disk Management (diskmgmt.msc) - is a tool in an operating system that helps users to manage and organize the hard disk and other storage devices. It allows users to create, delete, format, and resize partitions, as well as view and modify the attributes of disks, volumes, and partitions. Windows Computer Management Tool [TESPDDS] Windows Computer Management (compmgmt.msc) – a pack of Windows administrative tools Microsoft provides for users. You can use it to access to a series of administrative tools like Event Viewer, Task Scheduler, Device Manager, Disk Management, Services Manager, etc. [TESPDDS] 1. Task Scheduler 4. Performance 7. Services Manager 2. Event Viewer 5. Device Manager 3. Shared Folders 6. Disk Management Windows Registry Editor (regedit) – enables administrators and advanced users to keep the registry operational and make root- level and administrative-level changes, such as setting up access permissions or changing the hardware or software-level configurations. Is a database of configurations used by applications, services, and all other aspects of Windows. Always back up the Registry before editing it. control userpasswords2 – is used to access the user accounts user settings with advanced settings. You can use the list in user accounts to grant or deny users access to your computer and change passwords and other settings. Local Users and Groups ( lusrmgr.msc ) – regardless of how you choose to open Local Users and Groups (lusrmgr. msc), this is where you find all the user accounts and groups that are configured on your Windows computer or device, split into two folders: Users and Groups. Ways: Run, Cmd, Task Manager, Start Menu, Search box, Windows + X/Right-click on Start Best Data Recovery Software [DETRRSDD] 1. Disk Drill Data Recovery 5. R-Studio 2. EaseUS Data Recovery Wizard 6. Stellar Data Recovery Professional 3. TestDisk Data Recovery 7. DM Disk Editor and Data Recovery Software 4. Recuva 8. DiskInternals Uneraser