Module 04 - Java Syntax and Grammar PDF
Document Details
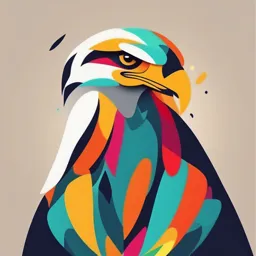
Uploaded by WellWishersWerewolf
Cavite State University
Tags
Summary
This document is a module on Java syntax and grammar, providing information on statements, comments, and identifiers in Java programming, with illustrative examples and guidelines.
Full Transcript
**Module 7: Syntax and Grammar** **Java program** - The items in angle brackets are placeholders. These must be replaced with whatever is appropriate for the program (without the angle brackets). - The is an identifier for the program which should be the same as the filena...
**Module 7: Syntax and Grammar** **Java program** - The items in angle brackets are placeholders. These must be replaced with whatever is appropriate for the program (without the angle brackets). - The is an identifier for the program which should be the same as the filename. Rules for identifiers will be discussed later on. - There is a minimum of 2 pairs of curly braces --- one after surrounding everything else, and another surrounding the. - args is a variable which may be renamed according to Java rules on variable names. At this point, just use it as is. **Sample Program:** Here, Hello is the class name. While System.out.println("Hello, World!"); is the statement. Statements will be discussed further later on. **Java Coding Guidelines** - Java program files must have the same name as public class and end with a.java extension - Use comments for documentation and readability - White spaces are ignored - Indent for readability **Java Statements** - A **Statement** specifies one or more actions to be performed during the execution of a program. In Java, a statement is one or more lines of code terminated by a semicolon. **Example:** System.out.print("Hello, World!"); - A **block** is formed by enclosing statements by curly braces. In Java, statements can be combined to form a statement block. A block is formed by enclosing statements by curly braces. These are treated as single units and may replace a statement anywhere a statement is valid. For readability, the statements of a block are indented. Common practice is to place the closing brace on its own line. Opening braces may be placed at the end of the previous line or on its own line. Doing these makes it easy to add/delete statements to/from blocks. **Example:**  - **Comment** is an optional statement used to describe what a program or a line of program is doing. Comments are used to add notes and documentation to source codes. Everything marked as comment is ignored by the compiler. Comments can appear anywhere in a Java source file except within character and string literals. - **Types of comments in Java** - A block comment is used to provide descriptions of files, methods, data structures, and algorithms. It starts with a forward slash and asterisk (/\*) and ends with an asterisk and forward slash (\*/). Anything between these delimiters is part of the comment and ignored, even if it spans multiple lines. - A line comment starts with two forward slashes (//) and ends at the end of the line the slashes appear on. If the slashes are the first two characters, then the whole line is considered a comment. If they appear towards the end of the line, after non-comment code, then only the characters following the slashes are ignored. This second use is called \"end-of-line comment\" which is often used to document variable declarations. - A JavaDoc comment is used specifically to document classes, attributes, and methods. It starts with a forward slash and two asterisks (/\*\*). It ends with one asterisk and a forward slash (\*/). The javadoc command can be used to convert a source file\'s JavaDoc comments into an HTML JavaDoc file. This HTML file can be packaged with the generated.class file to document the class\'s non-private API. - Note that a JavaDoc comment is just a special case of the block comment and can be used even if you do not intend to use JavaDoc. **Examples:** **Identifiers** Identifiers are words that are used by the programmer to properly identify or name a class, procedure, method, or variables. It should be noted that it is critical for the programmer to properly give identifiers so that it would be easy to know the purpose of variables, methods, or classes. In other words, choose identifier names that can be understood easily. For instance, if you require a variable to store the value of age, then name your variable as ―age.‖ **Rules on how to name an identifier** - Identifiers can use alphabetic characters, both lower and upper case (a--z and A--Z), numbers (0--9), underscores (\_), and dollar signs (\$). - Identifiers cannot start with a number. - Keywords cannot be used as identifiers (for this reason keywords are sometimes called reserved words). **Guidelines** - Name your identifiers close to its functionality - Method and variable names start in lowercase while classes start in uppercase. - For multi-word identifiers, either use underscores to separate the words, or capitalize the start of each word. - Avoid starting the identifiers using the underscore. Examples:  **Java Keywords** **Java Literals** Integer literals are used to represent specific integer values. Because integers can be expressed as octal (base 8), decimal (base 10), or hexadecimal (base 16) numbers in Java, each representation has its own form of literal. Also, integer literals can be expressed with an uppercase L (‗L') or owercase L (‗l') at the end to instruct the compiler to treat the number as a long (64-bit) integer. Java identifies any number that begins with a non-zero digit and does not contain a decimal point as a decimal integer literal (for example, any number between 1 and 9). To specify an octal literal, you must precede the number with a leading 0 (for example, 045 is the octal representation of 37 decimal). As with any octal representation, these literals can only contain the numerals 0 through 7. Hexadecimal integer literals are known by their distinctive \'zero-X\' at the beginning of the token. **Floating-Point Literal** The simplest way to understand what is allowed is to look at examples of valid float literals, as follows: 1e1f 2.f.3f 3.14f 6.02e+23f A suffix of \"F\" or \"f\" is always required on a float literal. A common mistake is to leave the suffix off the float literal, as follows: float cabbage = 6.5; Error: explicit cast needed to convert double to float. float cabbage = 6.5f; **Boolean Literal** The Boolean type has two values, false and true. It is used for evaluating logical conditions. You cannot convert between integers and Boolean values. **String Literals** The char primitive supports variables that represent a single character. Sequences of characters are not represented by a primitive type in Java, but by a reference type, the String class, instead. String literals contain zero or more characters enclosed in double quotation marks. These characters can include the escape sequences discussed previously. Both double quotation marks must appear on the same line of the source code, so strings cannot directly contain a new line character. To achieve the new line effect, you must use an escape sequence such as \\ n within the string. The doublequotation mark (\") and backslash (\\) characters must also be represented using escape sequences (\\ \" and \\) within a string literal. **Character Literal** Character literals are enclosed in single quotation marks. Any printable character, other than a backslash (\\), can be specified as the single character itself enclosed in single quotes. Some examples of these literals are \'a\', \'A\', \'9\', \'+\', \'\_\', and \'\~\'. Some characters, such as the backspace, cannot be written out like this, so these characters are represented by escape sequences. Escape sequences, like all character literals, are enclosed within single quotes. They consist of a backslash followed by one of the following: - A single character (b, t, n, f, r, \", \", or \\) - An octal number between 000 and 377 - A u followed by four hexadecimal digits specifying a Unicode character **Data Types** **Simple data types** are the built-in or primitive Java data types**. Composite data types** are created by the programmer using simple types, arrays, classes and interfaces. **Primitive Data Types in Java** Primitive data types are the building blocks and are an essential part of a program. Without these data types it would be impossible for a programmer to store pertinent data necessary in the completion of the program. There are eight primitive data types supported by the Java programming language and these are: - boolean (for logical) - char (for textual) - byte - short - int - long (integral) - double - float (floating-point) **Boolean** Java's Boolean data type is one-bit wide and takes on the values true or false. The initial value of a Boolean variable is false. A Boolean is not a number and cannot be converted (or cast) to a number or any other type. Nor can numeric operators be used with Boolean data. **Char Data Type** Java uses a 16-bit data type called char to represent text characters or Unicode characters. The data type can accommodate 216 or 65,536 bit combinations, so 65,536 characters can be represented. You must enclose a char literal in single quotes (‗'). For example, \'A\' is a character constant with value 65. It is different from \"A\", a string containing a single character. Unicode code units can be expressed as hexadecimal values that run from \\u0000 to \\uFFFF. For example, \\u2122 is the trademark symbol (™) and \\u03C0 is the Greek letter pi (p). The sequence must begin with the two characters \"\\u\". Following that opening sequence is a four-digit hexadecimal number that is the integer associated with that particular character according to the Unicode mapping. For example, the Unicode for the uppercase A is (hexadecimal) 0041, hence its Unicode escape sequence would be \'\\u0041\'. **Integer Data Type** The integer types are for numbers without fractional parts. Negative values are allowed.  In most situations, the int type is the most practical. If you want to represent the number of inhabitants of our planet, you\'ll need to resort to a long. The byte and short types are mainly intended for specialized applications, such as lowlevel file handling, or for large arrays when storage space is at a premium. Under Java, the ranges of the integer types do not depend on the machine on which you will be running the Java code. This alleviates a major pain for the programmer who wants to move software from one platform to another, or even between operating systems on the same platform. In contrast, C programs use the most efficient integer type for each processor. As a result, a C program that runs well on a 32-bit processor may exhibit integer overflow on a 16-bit system. Because Java programs must run with the same results on all machines, the ranges for the various types are fixed. Long integer numbers have a suffix L (for example, 4000000000L). Hexadecimal numbers have a prefix 0x (for example, 0xCAFE). Octal numbers have a prefix 0. For example, 010 is 8. Naturally, this can be confusing, and we recommend against the use of octal constants. **Floating Data Types** Another type of number that can be stored is a floating-point number, which has the type float or double. Floating-point numbers are numbers with a decimal point. The name double refers to the fact that these numbers have twice the precision of the float type. Here, the type to choose in most applications is double. The limited precision of float is simply not sufficient for many situations. Seven significant (decimal) digits may be enough to precisely express your annual salary in dollars and cents, but it won\'t be enough for your company president\'s salary. The only reasons to use float are in the rare situations in which the slightly faster processing of single-precision numbers is important or when you need to store a large number of them. Numbers of type float have a suffix F (for example, 3.402F). Floating-point numbers without an F suffix (such as 3.402) are always considered to be of type double. You can optionally supply the D suffix (for example, 3.402D). **Variable** Variables are places in memory that store values. They are used to refer to specific values that are generated in a program. Every variable has a data type and a name. The data type specifies the kind of data a variable can store. Its name is a label used to identify the variable and must follow the rules of identifiers. Some programming languages require that variables be declared before they are used. This means you need to explicitly list all the variables to be used by the program as well as the data type of each. **Declaration and initialization of Variables** In Java, variables are declared via statements of the form: \ \; and are initialized with values via statements of the form: \ \ = \[initial value\]; For example  **Guidelines in Declaring and Initializing Variables** Below are the guidelines in initializing variables: - Always initialize your variables as you declare them. - Use descriptive names for your variables. - Declare one variable per line of code. Example **Displaying Variable Values** In Java, we can output the value of a variable using the following commands:  The example below illustrates how these two commands are used in a program. The program will output the following text on screen: 10 The value of x = A System.out.print() System.out.print() does not append newline at the end of the data output. System.out.println()  **Types of Variables** Primitive variables are variables with primitive data types. These variables store data in the actual memory location of where the variable is. Reference variables are variables that store the address in the memory location. They point to another memory location where the actual data is. When you declare a variable of a certain class, you are actually declaring a reference variable to the object with that certain class. **Constant** Constants refer to those whose value does not change. In Java, the keyword final is used to modify a variable declaration to declare a constant.