Programming in Business Analytics (BMAN73701) Lecture 1
Document Details
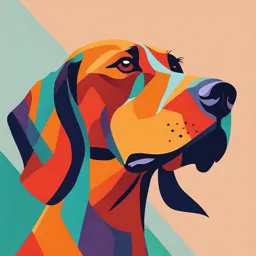
Uploaded by FastGrowingLute8905
University of Manchester
Dr. Xian Yang
Tags
Summary
This lecture introduces programming in Business Analytics (BMAN73701) with an overview of Python basics. Topics include the Integrated Analysis Environment, Python essentials, using Python for calculations, and handling numeric and string data types.
Full Transcript
Programming in for Business Analytics (BMAN73701) Lecture 1 - Introduction Dr. Xian Yang [email protected] https://research.manchester.ac.uk/en/persons/x ian.yang Outline for today General info about the course Integrated...
Programming in for Business Analytics (BMAN73701) Lecture 1 - Introduction Dr. Xian Yang [email protected] https://research.manchester.ac.uk/en/persons/x ian.yang Outline for today General info about the course Integrated Analysis Environment Python essentials – 1. Your first program – 2. Using Python as a Calculator – 3. Numeric data types – 4. String operations – 5. Type conversion – 6. Variables 2 Contact details For course-related questions: Post your question on the Discussion Board. For personal questions: [email protected] Allow 1-3 working days for a reply + please include BMAN73701 in Subject line Room: 3.077 AMBS Office Hours: Friday 12.00 – 1pm in office/zoom (Please email for appointment) 3 Who is in the room? MSc Business Analytics MSc Data Science MSc students from other programs MSc and PhD students auditing the module 4 Previous programming experience … A. None B. Intermediate Programming: A series of instructions that will automate performing a specific C. Expert task of solving a given problem. 5 Why Python? Used by e.g. Very attractive for Agile Software Development Very attractive for use as a scripting language (similar to Unix shell script or Windows batch files) but much more powerful! applicable for GUI applications, games, and more Open-source, simple and easy to learn syntax, short codes No compilation step fast edit-test-debug cycle increased productivity Debugging is easy error raises an exception Huge user community leading to loads of supporting online material, tutorials, discussion groups, blogs, books, etc 6 Course aims An introduction to the fundamentals of Python, a general-purpose programming language Application of Python to Data Science Use Python to facilitate decision making 7 Learning outcomes At the end of the course, you should be able to: Read and write Python code and understand how to use basic Python packages. Implement algorithms of moderate complexity in Python. Understand the fundamentals of object-oriented programming using Python. Understand how to implement simple data analysis from the literature to tackle business applications. Develop your own algorithms to solve basic data science problems. Use Python packages to solve complex data science, visualisation and optimisation problems in business and management (e.g., customer segmentation, and analysis of financial data). 8 Course assessment Formative assessment: – Online quizzes Summative assessment: 70% coursework and 30% online tests Coursework due on 13th Dec, Friday, 3 pm – Group report: 4000 words – Executable Python script Online tests – 2 online tests (15% each) on 7th Nov and 28th Nov 9 Basics of Python (and many other languages) Use existing Write own sophisticat routines from ed scratch modules Apply Apply to artificial modules or easy problems to real 10 Online Information https://www.python.org/doc/ essays/comparisons/ and many more comparisons available Supporting online information online… Python manual https://docs.python.org/3.12/inde x.html Learn Python http://www.learnpython.org/ http://www.tutorialspoint.com/ codingground.htm https://leetcode.com/ 11 Outline for today General info about the course Integrated Analysis Environment Python essentials – 1. Your first program – 2. Using Python as a Calculator – 3. Numeric data types – 4. String operations – 5. Type conversion – 6. Variables 12 Programming environment Anaconda: Freemium open source distribution of the Python and R programming languages for large-scale data processing, predictive analytics, and scientific computing, that aims to simplify package management and deployment. More than 30 other editors available… 13 Spyder Object inspector Documentation viewer Variable explorer File explorer Editor Interactive console Editor with function/class browser, code Instantly evaluate the code written analysis, code completion in the Editor 14 Spyder 15 Python source files have an Spyder extension of.py 16 Jupyter 17 Jupyter 18 Terminal 19 Terminal 20 Outline for today General info about the course Integrated Analysis Environment Python essentials – 1. Your first program – 2. Using Python as a Calculator – 3. Numeric data types – 4. String operations – 5. Type conversion – 6. Variables 21 1. Your first program In the terminal/console, type in “print(“Hello world!”)” and press enter. You should see the following Congratulations! You have written your first program 22 Your first program Type in “print(“Hello world!”)” and press enter. You should see the following Congratulations! You have written your first program Similar result: What happens if we write in the editor? 23 24 2. Using Python as a Calculator Python has the capability of carrying out calculations Standard operators, +, -, * and / work as you would expect; parenthesis () can be used for grouping 25 Using Python as a Calculator Other useful operators: // : floor division % : remainder ** : powers Assign a value to a variable using “=“ Last printed expression assigned to the 26 Using Python as a Calculator If you want to do more sophisticated calculations then import the math module. (Do not worry about what it means right now, we will cover this later during the course.) For example, take the square root of a number: 27 3. Built-in numeric data types int: Integer numbers (e.g. 4, 6, -4) float: Decimal numbers (e.g. 5.0, -3.1) Addition (+) , Subtraction (-) Multiplication (*), Floor Division (//) and Modulus (%): If both operands are int, the result will be an int. If either operand is a float, the result will be a float. Division (/): The division operation (/) always returns a float, even if both operands are int. 28 Quiz 1 1. Which option is the output of this code? >>> (4 + 8) / 2 ( becuz division always will be a flt) a) 6.0 b) 6 2. What is the result of this code? >>> 2 + 3 + 4 + 5.0 + 6 (if one got dec, then all dec) c) 20.0 d) 20 3. What is result of this code? >>> 7 % (13 // 5) e) 1 f) 1.0 4. The result of the following code is 0.1. >>> print(10 + 0.1 - 10) (due to floating-point precision, could be 0.10000) g) Yes h) No 29 4. Strings Use a string if you want to use text in Python Strings can start/end with ‘ or “ A string is generally displayed using single quotes ‘ - Anything put in str() like str(-3) will be 3. - string is not a numeric data type (but a sequence type 30 Strings Some characters cannot be directly included in a string Escape such special characters using a backslash before them Section 2.4.1.1 31 Output: The print() function The print() function produces a more readable output, by processing the escaped sequence 32 Input: The input() function The input() function prompts the user for input, and returns what they enter as a string -If you need the input to be an integer, float, or another type, you must explicitly convert it using functions like int(), float(), etc 33 String operations: Concatenation Concatenation is process of adding up strings in Python. It does not matter if strings were created with “ or ‘ Strings containing numbers are added up as strings and not integers Error when adding up string to a number 34 String operations: Concatenation However, strings can be multiplied by integers (but not by other strings or floats) 35 String operations: Concatenation Within the print()function, concatenation can be achieved via the “+” operator or via “,” >>> print(“Hello ”+”world!”) OR >>> print(“Hello“, “world!”) 36 5. Type conversion Another example: >>> x = 5 >>> x = 5 OR >>> print(“x = >>> print(“x = ”, x) ”+x) >>> x = 5 >>> print(“x = ”+str(x)) 37 Quiz 2 2. What is the correct output of this 1. What is the output of this code? code? >>> print(4 * “2”) >>> x = 5 a) ‘2222’ >>> print(“x” + “ y z”) (becuz not x, b) 8 its ‘x’) c) Error a) x y z b) 5yz c) Error: can’t add string to 3. The following code asks the user forinteger their year of birth. If you enter 2009, what would be the output? a) “You will be 21 years old in 2030”. b) “You will be 2030-YoB years old in 2030”. c) Error (Type conversion convert 2009 flt to str) 38 Quiz 3 What is the output of this code? >>> float(“111” * int(input(“Enter a number:” ))) Enter a number: 2 a) 222.0 b) 222 c) 111111.0 (becuz flt) d) 111111 e) Error 39 6. Variables A variable allows you to store a value by assigning it to a name (using one equals sign), which can be used to refer to the value later in the program. 40 Variables Variables can be reassigned arbitrarily often to change their value Variables do not have specific types! Variable names dynamic semantics can consist only of letters, numbers, and underscores (_), and they can’t start with numbers Python is case 41 Variables Trying to reference a variable you have not assigned to causes an error Use del statement to remove a variable (i.e. reference from the name to the value is deleted); deleted variables can be reassigned later as usual del is rarely used. The case where you might want to use del would be if you want to make it explicity clear that this variable cannot be used 42 In-place operators In-place operators allow you to write arithmetic operations, such as “x = x + 4”, more concisely as “x += 4” Whatever is on the right hand side of the assignment “=“, gets computed first. Only after that, the result is assigned to the variable on the left hand side. In-line operations, e.g. x Identical result += 1 equivalent to x = x >>> x = x + 3 + 1. Python cannot do 43 In-place operators Also possible with other operators, such as -, /, *, %. Operators can also be applied to types other than numbers, e.g. strings 44 Recap Integrated Analysis Environment Python essentials Your first program print() Using Python as a Calculator 5**2, 5%3, math.sqrt(5), etc Numeric data types int, float String operations input(), concatenation + Type conversion int(“4”), float(“8”) Variables var1 = 4, var1 += 3 45 Lecture 2 Preparation Prior to lecture, have a look at built-in types (sequences, mappings) control flow statements (for, if, while loops) comparison and Boolean operations BB: Additional materials Multiple choice questions Videos to support you in understanding how to determine and interpret line equations 46