Android Kotlin Development PDF
Document Details
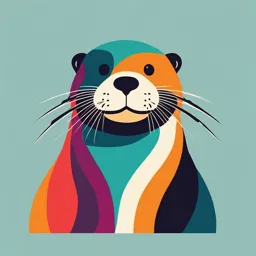
Uploaded by AdoredSanAntonio
Tags
Summary
This document provides an overview of Android Kotlin development. It covers topics from Android operating system fundamentals to creating projects and using the emulator. The guide explores key concepts and includes information on practical application and best practices for Android app development.
Full Transcript
Android Kotlin Development What is Android OS? Overview: Android is a mobile operating system based on a modified version of Linux, developed by Google primarily for touchscreen mobile devices like smartphones and tablets. Open Source: It’s open-source, which allows manufacturers, develop...
Android Kotlin Development What is Android OS? Overview: Android is a mobile operating system based on a modified version of Linux, developed by Google primarily for touchscreen mobile devices like smartphones and tablets. Open Source: It’s open-source, which allows manufacturers, developers, and enthusiasts to customize it freely. Popularity: Android holds a significant share of the global mobile OS market due to its flexibility, vast ecosystem of apps, and hardware compatibility. Ecosystem: Includes Google Play, Google Mobile Services, and various app distribution channels that support a wide range of apps and features. Platform Architecture Android’s architecture is organized into several layers, each with specific roles: Linux Kernel: The core foundation that interacts directly with hardware. It manages drivers, memory, process management, and other low-level functions. Hardware Abstraction Layer (HAL): Acts as an intermediary, providing standard interfaces for hardware components like the camera, Bluetooth, and sensors. Android Runtime (ART): Runs Android apps in a managed environment. The ART, which replaced the older Dalvik runtime, improves performance with Ahead-of-Time (AOT) compilation. Native C/C++ Libraries: Libraries for handling graphics, databases (SQLite), web rendering (WebKit), etc., supporting core Android functionality. Java API Framework: Provides core components that developers use to build Android apps. It includes system services and other API components. System Apps: These are pre-installed apps (like Phone, Messages) that give users core functionality and often serve as templates for third-party app development. API Levels Definition: An API level is an integer value that represents the version of the Android framework API provided by a version of the Android OS. Purpose: They ensure that apps can interact with specific system features available on devices with compatible OS versions. Backward Compatibility: Developers can specify a minimum API level required to run their app, ensuring it won’t be installed on devices lacking essential features. Evolution: Each new version of Android comes with new API levels, adding functionalities, fixing issues, and enhancing security. As of today, Android has reached API level 34 (Android 14). App Components Android apps are composed of four main components that define the structure and behavior of the app: Activities: Represent a single screen with a user interface. Each activity facilitates user interactions with the app, such as logging in or viewing a list. Services: Background processes that perform long-running tasks without a user interface. Examples include playing music in the background or fetching data from a server. Content Providers: Manage and share data across applications, such as contacts and media files, and facilitate database interactions. Broadcast Receivers: Respond to system-wide events, such as battery level changes or network connectivity changes, allowing apps to react to these events without running continuously. Android fundumntials Install android stuido Install kotlen Creating a Project in Android Studio Using Step-by-Step Implementation for Creating Projects in Android Studio with Kotlin: Step 1: Select the Layout for your Kotlin Application: Step 2: Here we write name of our application and select the language Kotlin f or the project. Then, click on the Finish button to launch the project. Run apps on the Android Emulator The Android Emulator simulates Android devices on your computer so that you can test your application on a variety of devices and Android API levels without needing to have each physical device. The emulator offers these advantages: Step Flexibility: In addition to being able to simulate a variety of devices and 3: Check on Android API levels, the emulator comes with predefined configurations for various Android phone, tablet, Wear OS, Android Automotive OS, and the Created Android TV devices. Project. High fidelity: The emulator provides almost all the capabilities of a real Android device. You can simulate incoming phone calls and text messages, specify the location of the device, simulate different network speeds, simulate rotation and other hardware sensors, access the Google Play Store, and much more. Speed: Testing your app on the emulator is in some ways faster and easier than doing so on a physical device. For example, you can transfer data faster to the emulator than to a device connected over USB. https://developer.android.com/studio/run/emulator Emulator system requirements For the best experience, you should use the emulator in Android Studio on a computer with at least the following specs: 16 GB RAM 64-bit Windows 10 or higher, MacOS 12 or higher, Linux, or ChromeOS operating system 16 GB disk space https://developer.android.com/studio/run/managing- avds Develop a UI with Views In Android, you can set up your app's layout in two main ways: XML Layouts: You can declare UI elements in XML files. Android offers a simple XML structure that matches different types of views (like buttons, text fields) and layouts (how elements are arranged). You can create these layouts by hand or use Android Studio’s drag-and-drop Layout Editor. Declaring layouts in XML keeps the app's look separate from its functionality, which is helpful for organizing code and creating layouts that adjust to various screen sizes and orientations. Programmatic Layouts: Alternatively, you can create and modify layout elements directly in your code (at runtime). This lets you build and adjust UI elements dynamically based on user interactions or app states. You can combine these two methods—using XML for your default layouts and making adjustments through code when needed. This flexibility helps you create adaptable, responsive interfaces. Types of Android Layout Android Linear Layout: LinearLayout is a ViewGroup subclass, Description: Arranges elements in a single row (horizontal) or column (vertical). Android Relative Layout: RelativeLayout is a ViewGroup subclass, Description: Positions elements relative to each other or the parent layout (e.g., above, below, aligned to the left/right). Android Constraint Layout: ConstraintLayout is a ViewGroup subclass, Description: Allows flexible positioning and sizing using constraints to other elements or parent layout; ideal for complex layouts. Types of Android Layout Android Frame Layout: FrameLayout is a ViewGroup subclass. Description: Stacks elements on top of each other; primarily used for single or overlaying elements. Android Table Layout: TableLayout is a ViewGroup subclass, Description: Arranges elements in rows and columns, similar to an HTML table. Android Web View: WebView is a browser that is used to display the web pages in our activity layout. Android ListView: ListView is a ViewGroup, used to display scrollable lists of items in a single column. Android Grid View: GridView is a ViewGroup that is used to display a scrollable list of items in a grid view of rows and columns. Using XML In Android XML, you design layouts similarly to HTML, using nested elements to define your screen's structure. Root Element: Each layout XML file must start with a single root element, either a View or ViewGroup, which organizes all other UI elements. Hierarchy: Inside the root, you add child elements (like layouts or widgets) to create a structure or hierarchy. Example: A LinearLayout is used as the root element to organize a TextView and a Button vertically: After you declare your layout in XML, save the file with the.xml extension in your Android project's res/layout/directory so it properly compiles. Load the XML resource When you compile your app, each XML layout file is compiled into a View resource. Load the layout resource in your app's Activity.onCreate() callback implementation. Do so by calling setContentView(), passing it the reference to your layout resource in the form: R.layout.layout_file_name. For example, if your XML layout is saved as main_layout.xml, load it for your Activity as follows: The Android framework calls the onCreate() callback method in your Activity when the Activity launches. For more information about activity lifecycles, see Introduction to activities. Attributes In Android XML layouts, several key attributes control the positioning, appearance, and behavior of elements. Every View and ViewGroup object supports its own variety of XML attributes. Some attributes are specific to a View object. For example, TextView supports the textSize attribute. However, these attributes are also inherited by any View objects that extend this class. Some are common to all View objects, because they are inherited from the root View class, like the id attribute. Other attributes are considered layout parameters, which are attributes that describe certain layout orientations of the View object, as defined by that object's parent ViewGroup object. Common Attributes for All Layouts android:layout_width and android:layout_height: Define the size of the view. Common values are: match_parent: Takes up the full space of the parent view. wrap_content: Adjusts to the size of the content inside the view. android:layout_margin: Defines space outside the view’s boundaries. You can use individual margins like android:layout_marginTop, android:layout_marginBottom, etc. android:padding: Sets space inside the view, between the content and the view’s boundaries (e.g., android:paddingTop, android:paddingLeft). android:background: Sets the background color or drawable (e.g., #FFFFFF for white or @drawable/image for a drawable resource). Layout-Specific Attributes Each layout has specific attributes that determine how its children are arranged. LinearLayout android:orientation: Sets direction for elements (horizontal or vertical). android:gravity: Aligns all children within the layout (center, start, end, top, etc.). android:layout_weight: Distributes space among child views proportionally. For example, if two views have layout_weight="1", each will take up half the available space. Layout-Specific Attributes RelativeLayout Positioning Attributes: Align elements relative to other elements or the parent. Examples include: android:layout_alignParentTop="true": Aligns the view with the top of the parent. android:layout_below="@id/anotherView": Positions the view below another view with ID anotherView. Alignment Attributes: Align a view's edges to another view's edges. Examples include layout_alignLeft, layout_toRightOf Layout-Specific Attributes ConstraintLayout Constraints: Position views relative to other views or the parent using attributes like: app:layout_constraintTop_toTopOf="parent" app:layout_constraintBottom_toBottomOf="@id/anotherView" Bias: Control positioning within constraints using attributes like app:layout_constraintHorizontal_bias, which accepts a value from 0 (start) to 1 (end). Layout-Specific Attributes FrameLayout android:layout_gravity: Specifies how each child is positioned within the FrameLayout. Options include center, top, bottom, left, right. TableLayout android:stretchColumns: Sets specific columns to stretch to fill the available space. android:shrinkColumns: Shrinks specific columns if needed to fit the screen. Layout-Specific Attributes Common View Attributes android:id: Unique identifier for the view, allowing you to reference it in Java/Kotlin code or other XML layouts. android:visibility: Controls visibility (visible, invisible, gone). android:text: Sets the text of a view (for TextView, Button, etc.). android:textSize: Sets the text size (e.g., 16sp). android:textColor: Sets the color of the text. XML attributes Description android:id Used to specify the id of the view. Used to declare the width of View and ViewGroup android:layout_width elements in the layout. Used to declare the height of View and ViewGroup android:layout_height elements in the layout. Used to declare the extra space used on the left android:layout_marginLeft side of View and ViewGroup elements. Used to declare the extra space used on the right android:layout_marginRight side of View and ViewGroup elements. Used to declare the extra space used in the top android:layout_marginTop side of View and ViewGroup elements. Used to declare the extra space used in the bottom android:layout_marginBottom side of View and ViewGroup elements. Used to define how child Views are positioned in android:layout_gravity the layout. UI Layouts The Layout Editor enables you to quickly build View-based layouts by dragging UI elements into a visual design editor instead of writing layout XML. The design editor can preview your layout on different Android devices and versions, and you can dynamically resize the layout to be sure it works properly on different screen sizes. The Layout Editor is especially powerful when building a layout with ConstraintLayout. Next lecture Next lectures