JavaScript Basics PDF - IT2301
Document Details
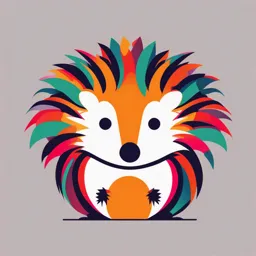
Uploaded by PalatialSousaphone8364
Carey, Vodnik
Tags
Summary
This document provides an introduction to JavaScript programming. It explains basic concepts like statements, objects, and output methods. The document focuses on internal and external JavaScript and includes examples.
Full Transcript
IT2301 THE JAVASCRIPT BASICS JavaScript Basics (Carey, Vodnik, 2022) As HTML is assigned for the structure of the website and CSS is for styling it, JavaScript (JS) makes the website interactive and complex through the implementation of intricate features such a...
IT2301 THE JAVASCRIPT BASICS JavaScript Basics (Carey, Vodnik, 2022) As HTML is assigned for the structure of the website and CSS is for styling it, JavaScript (JS) makes the website interactive and complex through the implementation of intricate features such as displaying timely content updates and determining what happens when users click, hover, or type within specific elements in the website. It is a case-sensitive scripting language for webpages and is considered the third standard for web technologies after HTML and CSS. As each language serves a different purpose, it is necessary to use a separate file for each, but it must be in the same directory, which can be a folder on the computer. For JavaScript, a.js file extension is used. This idea is called “separation of concerns,” meaning a program is separated into distinct sections. In context, the “program” refers to the website, while the “sections” refers to the separate files of HTML, CSS, and JavaScript. They might be separated, but they have a unique function needed for the website. Just like in CSS, JavaScript can be referred to internally and externally, such as: Internal: External: Internal External document.write("A sample script"); In internal or embedded JavaScript, the JS code is placed and can appear multiple times inside the or tags of an HTML document using tags that encloses the JavaScript codes. While in external JavaScript, the separated.js file is linked using tags with the src attribute are usually placed within the tags. It applies the JavaScript code to the webpage so that it can have an effect on the HTML document and anything else on it. As practiced, all codes can be in one HTML code, but it will lead to repetitive sets of code that might confuse as the website grows. JavaScript Comment It is the line of code that browsers ignore and is used on a specific line or block of codes to add notes such as the program name and date or notes for future programmers that might modify the program. A line comment is used by adding two (2) slashes ( // ) before the note or comment, while block comments are used to hide multiple lines of codes by adding after the last character in the block. 05 Handout 1 *Property of STI [email protected] Page 1 of 6 IT2301 JavaScript Statement It is an individual line of code that is enclosed within the tags. This sample statement writes the text “Best choices” without quotation marks on a webpage. It uses the write() document.write("Best choices"); method of the Document object, and each statement must end with a semicolon (;). JavaScript Object It is a programming code that can be treated as an individual unit or component. A JS object can be defined and created by programmers based on the program's need aside from available objects from the Document Object Model (DOM) and Browser Object Model (BOM). An object such as bankLoan is created to calculate the number of payments to pay off a bank loan. Statements such as this are often grouped into logical units called procedures that perform specific tasks. Additionally, procedures associated with an object are called methods which are actions that can be performed on it. In this sample, bankLoan.calcPayments(60); calcPayments(), which is defined to calculate the number of payments to pay off the loan is the associated method. Arguments are used and placed inside the parentheses beside it to provide more specific information about the method. In this case, (60) is the argument that specifies the number of months until the loan is paid off. JavaScript Output Methods Any text, graphics, and other information displayed on the webpage is part of the Document object, which is one of the most commonly used objects in DOM. The write() method of the Document object is used to write custom information such as username or address. Moreover, this also requires a text string as an argument, which is the text contained within double or single quotation marks. The combination of these concepts becomes document.write("Best choices"); console.log("Hello, World!"); console.log is a basic method used to write text to the console. A console.log(Hello, World); console is where messages are displayed that are invisible to users and are separated from the HTML/CSS environment. However, this console.log("Steve", can be visible in a browser’s developer tool console. "Martin", "0323"); console.log("Key" + "Quan" + It is possible to input text in the argument with or without quotation "0323"); marks and stack arguments separated by commas or + signs, The alert() method is used to display an alert box that has a message and an OK button. The text in the argument is the information function testFunction() { users are forced to see, so it is better not to overuse this method. alert("Hello, how are you?"); testFunction is the function name, and the parentheses here are } called parameters which are the variables used within the function separated by a comma. 05 Handout 1 *Property of STI [email protected] Page 2 of 6 IT2301 JavaScript Operator It is used to perform specific actions on arguments called operands, which can be both values and variables. Some of the common operator categories include the following: Assignment operators allow assigning of values to variables and constants. It commonly uses the equal sign (=). Arithmetic operators express mathematical operations and accept numerical values and variables. It includes addition (+), subtraction (-), multiplication (*), division (/) remainder (%), power (**), and increment (++) that adds one (1) in the operand and decrement (--) that subtracts one (1). Logical operators work with Boolean-type values such as true or false. It includes a conjunction that performs logical AND (&&), an alternative that performs logical OR (||), and a negation that performs logical NOT (!). Comparison operators compare two (2) variables or operands. It includes not equal or equal to the value of the variable or operand (!=, ==), equal value and equal type (===), less than and greater than (), and less than or equal to and greater than or equal to (=), JavaScript Variable It is the value that the program stores in a computer’s memory. It is used by writing a statement first that creates the variable and assigning a name for it. The assigned name to a variable is called an identifier which has the following conventions when naming a variable in JavaScript: It must begin with an uppercase or lowercase ASCII letter, a dollar sign ($), or an underscore (_). A number can be used but not as the first character. It cannot include spaces. Keywords or reserved words are not allowed as identifiers. Keywords or reserved words are unique words that are already part of the JavaScript language syntax. It includes these commonly used keywords such as abstract, boolean, char, class, const, debugger, double, else, FALSE, float, for, if, let, new, null, TRUE, var, void, while, and with. In JavaScript, five (5) primitive data types specify which type of value a variable contains. String – represents textual data. Boolean contains a logical entity that may return a TRUE or FALSE value. Number – any digit that is either an integer or a decimal. Null – an unknown or missing value. Undefined – refers to a variable with no value. The undefined keyword can be set in the variable. Declaring and Initializing Variables Before a variable is used, it must be declared first so it can store data and objects or initialized, which assigns it an initial value. Variables are declared and initialized using the let or var keywords. let variable = value; var variable = value; The variable is the variable name, while the value is its initial value. The value can be a text string enclosed within quotation marks or a numerical let taxRate = 0.05; value like the other set. var taxRate = “sales”; let salesTotal; Assigning the value of one variable to another is also possible. Here, the salesTotal variable is declared without an initial value, while the curOrder let curOrder = 47.58; has an initial value of 47.58. On the third statement, the value of curOrder is salesTotal = curOrder; assigned to the salesTotal variable, giving them the same value. An array is used to declare a set of data represented by a single variable name such as let months = ["Jan", "Feb", "Mar", "Apr", "May",]; 05 Handout 1 *Property of STI [email protected] Page 3 of 6 IT2301 Sample structure using Internal JavaScript. Sales Total let salesTotal = 3428.92; document.write("Your sales total is P" + salesTotal + ""); Sales Total with Shipping let shipping = 150; let totalCost = salesTotal + shipping; document.write("Your sales total plus shipping is P" + totalCost + ""); Control Flow (OpenEDG, 2023) Control flow statements, or conditional execution, break up the execution flow using decision-making, branching, and looping to enable programs to conditionally execute specific code blocks. if statement It is the simplest control flow instruction in JavaScript. It checks a given condition and, depending on its Boolean value, either executes a code block or skips it. The code block should be separated using curly brackets. The if keyword is followed by the expression in let isUserReady = confirm("Are you parentheses (isUserReady), and if the result is true, ready?"); meaning the user closes the dialog box asking Are you console.log(isUserReady); ready? by clicking the OK button, an alert message with User ready! will show. if (isUserReady) { alert("User is ready!"); If the expression evaluates to false, meaning the user } closes the dialog box without clicking OK, the alert message will never be shown. if… else statement let isUserReady = confirm("Are you The else keyword is an optional part of the if statement, ready?"); and it allows the addition of a second code block that will be executed only when the initial condition is NOT met. if (isUserReady) { Both blocks of code are separated using the else keyword. console.log("User is ready!"); } else { When the user closes the dialog box without clicking OK, console.log("User is not ready!"); the console displays User is not ready! Creating a } new output. 05 Handout 1 *Property of STI [email protected] Page 4 of 6 IT2301 else if statement let number = prompt("Enter any number from 0-100", 0); if (number < 20) { alert("Less than 20"); } else if (number < 40) { This statement branches the code execution flow until the alert("Less than 40"); inputted or available data meet the specific condition that } else if (number < 60) { corresponds to it. alert("Less than 60"); In this code, users are asked to Enter any number from } else if (number < 80) { 0-100 using the prompt method. If the entered number is alert("Less than 80"); 65, for example, it will execute the third else if condition, } else if (number < 100) { which shows an alert that the entered number is Less alert("Less than 100"); than 80. } else if (number == 100) { alert("100") } else { alert(">100") } Loops and Iterations (OpenEDG, 2023) Loops are the second form of control flow statement. While control flow statements can change code behavior, loops are an easy way to repeat any fragment of the code as many times as possible, or until a stopping condition is met. while loop In a while loop, a code block is executed while a given condition is true but stops once the condition is false. When a code block is repeated, it is referred to as an iteration. An infinite loop is avoided by including at least one statement that eventually results in a false value for the condition. A code block uses a counter, whose value changes with each iteration, and once that counter fails to match the condition, the loop ends. This sample includes a counter variable named j with an let j = 1; initial value of 1. With each iteration, the value of j while (j