Problem Solving in Computing PDF
Document Details
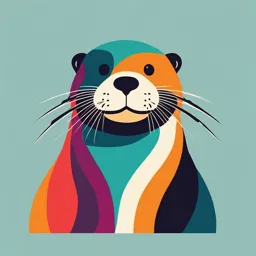
Uploaded by LuxuryRiemann5378
Year 9
Tags
Related
- GE8151- PROBLEM SOLVING AND PYTHON PROGRAMMING - Question Bank PDF
- Computer Science Problem Solving Concepts (2022) PDF
- Computer Science - Class XI - Problem Solving PDF
- Computer Science Algorithms and Problem Solving 2015 PDF
- Problem Solving Final Notes
- Lecture 2 - Foundations of Programming and Problem Solving
Summary
This document provides an overview of the problem-solving process. It highlights the importance of clearly defining a problem, analyzing it, developing a solution plan (e.g., using algorithms, pseudocode, or flowcharts), executing the plan efficiently, and evaluating the results. Examples of algorithms, such as finding the average of student grades or the largest of two numbers are illustrated.
Full Transcript
## Chapter 1: Introduction to Problem Solving ### 1.1) Defining a Problem A problem is a situation that needs to be resolved. We can identify a problem in many ways, for example, through observation, experience, or by someone defining it for us. Once identified, we can come up with a plan to solve...
## Chapter 1: Introduction to Problem Solving ### 1.1) Defining a Problem A problem is a situation that needs to be resolved. We can identify a problem in many ways, for example, through observation, experience, or by someone defining it for us. Once identified, we can come up with a plan to solve it. ### 1.2) The Problem-Solving Process A problem-solving process is the set of actions required to find solutions to a problem. The steps in a problem-solving process include the following : * **Clearly define the problem:** We understand the problem stated and outline the way(s) in which we expect to solve it. * **Analyze the problem:** We look at all the ways in which the problem can be solved and specify general operations that need to be carried out to solve it. We analyze all the ways to find the best, most efficient way of solving it. * **Sketch a solution plan:** After the problem has been analyzed, a model is developed to plan the solution. An algorithm, pseudocode, and/or flowchart is created to represent the solution plan as we will see in the following sections. * **Execute a plan:** The solution plan developed in the previous step is executed by transforming it into a computer program. * **Evaluate the results:** The program is tested by using sample data (input) to check that it is working as it should and producing the expected results (output). If the results are not as they should be, it means there is an error in the previous steps. ### 1.3) Algorithms An **algorithm** is a sequence of steps that describe how to solve a problem. An algorithm is not detailed, but it gives an outline of what needs to be done to solve the problem. An algorithm that finds the sum of two numbers may look like this: * **Step 1:** Ask the user to enter the first number. * **Step 2:** Ask the user to enter the second number. * **Step 3:** Add the two numbers and store the result in a variable called SUM. * **Step 4:** Display SUM. **Solved Examples** **Write an algorithm that finds the average of student grades that a user enters** * **Step 1:** Ask the user to enter grades. The program keeps accepting input grades until the user enters a negative number. * **Step 2:** Initialize variables SUM (that holds the total of all grades) and COUNT (that holds how many student grades were entered) to zero. * **Step 3:** With each grade entered, add the grade to the variable SUM. * **Step 4:** With each grade entered, increment the variable COUNT. * **Step 5:** After all grades are entered, find the average by dividing SUM by COUNT. Make sure not to divide by zero, in case no grades were entered. * **Step 6:** Display the average grade. **Activity** * Write an algorithm that finds the largest of two input numbers or indicates if they are equal. If we study the above problem, we can identify the following: **The problem:** Find the average of a class of students. **The input:** We input the grade of each student. Since the number of students in one class may vary from that in another, we input a negative number after entering all grades. This indicates that no more grades will be entered. **Processing:** * Calculate the sum of all grades. * Count how many students are in the class. * Calculate the average by dividing the sum by the number of students. **The output:** The average grade for the class. **Activity** * You need to write a program that finds the larger of two input numbers or indicates whether they are equal. To solve the problem, list the input, processing, and output. ### 1.4) Pseudocode **Pseudocode** is a way to represent an algorithm in regular English and in mathematical representations. Writing the pseudocode instructions is very similar to writing the actual program but without abiding by the rules or syntax of a specific programming language. The advantages of pseudocode are that it is easy to read, understand, and modify. For example, the pseudocode below asks the user for two numbers and displays their sum: * Display "Enter the first number:" * Read X * Display "Enter the second number:" * Read Y * SUM = X+Y * Display "The sum of both numbers is" SUM The following pseudocode represents the algorithm for finding the class average: * SUM = 0 * COUNT =0 * Average = 0 * Display "Enter the student grades, and a negative number after all grades are entered." * Read x * While x >= 0 then * Begin * SUM = SUM+x * COUNT = COUNT+1 * Read x * End * If COUNT > 0 then Average = SUM/COUNT * // make sure not to divide by zero * Display "The average is" Average **Activity** * Circle the correct answer: Which of the instructions below inputs a number from the user? * SUM = 0 * Begin * End * Read x * While x > 0 then ### 1.5) Flowcharts A flowchart is a graphical representation of an algorithm. A flowchart uses shapes and arrows to represent the steps of an algorithm and the flow of instructions. Each flowchart shape has a specific meaning and context in which it is used. Below is a list of flowchart shapes and what each means. Shape | Name | What it does ------- |:-------:|:-------- Rectangle | Terminator | This shows the start and end of the flowchart. Rounded Rectangle | Process | This shows operations. (For example, assigning values to variables or mathematical operations) Parallelogram | Data | This means input and output data (For example, print statements and read statements). Diamond | Decision | It branches to more than one option, depending on the result of a condition. The decision shape is usually used for conditional statements (such as if/else) and for loops (such as the while and repeat loops). Circle | Connector | This connects two parts of a flowchart, especially if a flowchart runs on to more than one page. Arrow | Flow line | This shows the direction of the flow of control in the program.