CMPE-103-Module-4-Introduction-to-Object-oriented-Programming.pdf
Document Details
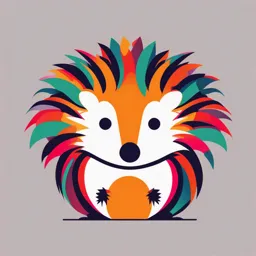
Uploaded by ConfidentBasilisk
Tags
Full Transcript
CMPE 103 OBJECT-ORIENTED PROGRAMMING MODULE 4 OBJECT-ORIENTED PROGRAMMING What is a Class and Objects in Python? Class: The class is a user-defined data structure that binds the data members and methods into a single unit. Class is a blueprint or code template for obje...
CMPE 103 OBJECT-ORIENTED PROGRAMMING MODULE 4 OBJECT-ORIENTED PROGRAMMING What is a Class and Objects in Python? Class: The class is a user-defined data structure that binds the data members and methods into a single unit. Class is a blueprint or code template for object creation. Using a class, you can create as many objects as you want. Object: An object is an instance of a class. It is a collection of attributes (variables) and methods. We use the object of a class to perform actions. In short, Every object has the following property. Identity: Every object must be uniquely identified. State: An object has an attribute that represents a state of an object, and it also reflects the property of an object. Behavior: An object has methods that represent its behavior. Python is an Object-Oriented Programming language, so everything in Python is treated as an object. An object is a real-life entity. It is the collection of various data and functions that operate on those data. Create a Class in Python In Python, class is defined by using the class keyword. The syntax to create a class is given below. Syntax: class Person: def __init__(self, name, sex, profession): # data members (instance variables) self.name = name self.sex = sex self.profession = profession # Behavior (instance methods) def show(self): print('Name:', self.name, 'Sex:', self.sex, 'Profession:', self.profession) # Behavior (instance methods) def work(self): print(self.name, 'working as a', self.profession) # create object of a class jessa = Person('Jessa', 'Female', 'Software Engineer') # call methods jessa.show() jessa.work() Objects do not share instance attributes. Instead, every object has its copy of the instance attribute and is unique to each object. All instances of a class share the class variables. However, unlike instance variables, the value of a class variable is not varied from object to object. Only one copy of the static variable will be created and shared between all objects of the class. Accessing properties and assigning values An instance attribute can be accessed or modified by using the dot notation: instance_name.attribute_name. A class variable is accessed or modified using the class name Class Methods In Object-oriented programming, Inside a Class, we can define the following three types of methods. What is Constructor in Python? In object-oriented programming, A constructor is a special method used to create and initialize an object of a class. This method is defined in the class. The constructor is executed automatically at the time of object creation. The primary use of a constructor is to declare and initialize data member/ instance variables of a class. The constructor contains a collection of statements (i.e., instructions) that executes at the time of object creation to initialize the attributes of an object. In Python, Object creation is divided into two parts in Object Creation and Object initialization Default Constructor Python will provide a default constructor if no constructor is defined. Python adds a default constructor when we do not include the constructor in the class or forget to declare it. It does not perform any task but initializes the objects. It is an empty constructor without a body. If you do not implement any constructor in your class or forget to declare it, the Python inserts a default constructor into your code on your behalf. This constructor is known as the default constructor. It does not perform any task but initializes the objects. It is an empty constructor without a body. Non-Parametrized Constructor A constructor without any arguments is called a non-parameterized constructor. This type of constructor is used to initialize each object with default values. This constructor doesn’t accept the arguments during object creation. Instead, it initializes every object with the same set of values. Parameterized Constructor A constructor with defined parameters or arguments is called a parameterized constructor. We can pass different values to each object at the time of creation using a parameterized constructor. The first parameter to constructor is self that is a reference to the being constructed, and the rest of the arguments are provided by the programmer. A parameterized constructor can have any number of arguments. Constructor With Default Values Python allows us to define a constructor with default values. The default value will be used if we do not pass arguments to the constructor at the time of object creation. Self Keyword in Python Circle.py 1 import math 2 3 class Circle: 4 # Construct a circle object 5 def _ _init_ _(self, radius = 1): 6 self.radius = radius 7 8 def getPerimeter(self): 9 return 2 * self.radius * math.pi 10 11 def getArea(self): 12 return self.radius * self.radius * math.pi 13 14 def setRadius(self, radius): 15 self.radius = radius 1 from Circle import Circle 2 TestCircle.py 3 def main(): 4 # Create a circle with radius 1 5 circle1 = Circle() 6 print("The area of the circle of radius", 7 circle1.radius,"is",circle1.getArea()) 8 9 # Create a circle with radius 25 10 circle2 = Circle(25) 11 print("The area of the circle of radius", 12 circle2.radius, "is",circle2.getArea() ) 13 14 # Create a circle with radius 125 15 circle3 = Circle(125) 16 print("The area of the circle of radius" 17 , circle3.radius, "is",circle3.getArea() ) 18 19 #Modify circle radius 20 circle2.radius =100 # or circle2.setRadius(100) 21 print("The area of the circle radius", 22 circle2.radius. "is", circle2.getArea()) 23 24 main() # Call the main function Exercise: Given: A UML Class Diagram below: Required: Create a Python Code for creating the Class named TV and a Test Driver program named TestTV that will create two objects from Class TV and will produce the following output: