C Programming: Printing a Line of Text (PDF)
Document Details
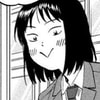
Uploaded by y88mi
2021
Tags
Summary
This document details the fundamentals of C programming, focusing on printing a line of text. It introduces concepts like comments, preprocessor directives, and escape sequences. It's a step-by-step guide.
Full Transcript
2.2 A Simple C Program: Printing a Line of Text (1 of 15) 1. // fig02_01.c 2. // A first program in C. 3. #include 4. Loading… 5. // function main begins program execution 6. int main(void) { 7. 8. printf("Welcome to C!\n"); } // end function main OUTPUT: – Welcome to C! Copyright © 2021 Pearson Edu...
2.2 A Simple C Program: Printing a Line of Text (1 of 15) 1. // fig02_01.c 2. // A first program in C. 3. #include 4. Loading… 5. // function main begins program execution 6. int main(void) { 7. 8. printf("Welcome to C!\n"); } // end function main OUTPUT: – Welcome to C! Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.2 A Simple C Program: Printing a Line of Text (2 of 15) Comments Begin with // Insert comments to document programs and improve program readability Comments do not cause the computer to perform actions Help other people read and understand your program Can also use multi-line comments – Everything between is a comment Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.2 A Simple C Program: Printing a Line of Text (3 of 15) #include Preprocessor Directive #include C preprocessor directive Preprocessor handles lines beginning with # before compilation This directive includes the contents of the standard input/output header () – Contains information the compiler uses to ensure that you correctly use standard input/output library functions such as printf Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.2 A Simple C Program: Printing a Line of Text (4 of 15) Blank Lines and White Space Blank lines, space characters and tab characters make programs easier to read Together, these are known as white space Generally ignored by the compiler Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.2 A Simple C Program: Printing a Line of Text (5 of 15) The main Function Begins execution of every C program Parentheses after main indicate that main is a function C programs consist of functions, one of which must be main Precede every function by a comment stating its purpose Functions can return information The keyword int to the left of main indicates that main “returns” an integer (whole number) value – for now simply mimic this Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.2 A Simple C Program: Printing a Line of Text (6 of 15) The main Function Functions can receive information – void in parentheses means main does not receive any information A left brace, {, begins each function’s body A corresponding right brace, }, ends each function’s body A program terminates upon reaching main’s closing right brace The braces form a block Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.2 A Simple C Program: Printing a Line of Text (7 of 15) An Output Statement string of Characters printf("Welcome to C!\n"); – “f” in printf stands for “formatted” Loading… Performs an action—displays the string in the quotes Str – A string is also called a character string, a message or a literal The entire line is called a statement Every statement ends with a semicolon statement terminator - Characters usually print as they appear between – Notice the characters \n were not displayed. Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.2 A Simple C Program: Printing a Line of Text (8 of 15) Escape Sequences In a string, backslash (\) is an escape character Compiler combines a backslash with the next character to form an escape sequence \n means newline When printf encounters a newline in a string, it positions the output cursor to the beginning of the next line Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.2 A Simple C Program: Printing a Line of Text (9 of 15) Escape Sequence be can \n \t blank 4 or on 8 the a : line Space or Description characters 2 depends Moves the cursor to the beginning of the next line. Moves the cursor to the next horizontal tab stop. system \a Produces a sound or visible alert without changing the current cursor position. \\ Because the backslash has special meaning in a string, \\ is required to insert a backslash character in a string. \” Because strings are enclosed in double quotes, \” is required to insert a double-quote character in a string. - Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.2 A Simple C Program: Printing a Line of Text (10 of 15) The Linker and Executables When compiling a printf statement, the compiler merely provides space in the object program for a “call” to the function The compiler does not know where the library functions are— the linker does When the linker runs, it locates the library functions and inserts the proper calls to these functions in the object program Now the object program is complete and ready to execute The linked program is called an executable Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.2 A Simple C Program: Printing a Line of Text (11 of 15) Indentation Conventions Indent the entire body of each function one level of indentation within the braces that define the function’s body Emphasizes a program’s functional structure and helps make them easier to read Set an indentation convention and uniformly apply that convention Style guides often recommend using spaces rather than tabs Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.2 A Simple C Program: Printing a Line of Text (12 of 15) Using Multiple printfs Fig. 2.2 uses two statements to produce the same output as Fig. 2.1 Works because each printf resumes printing where the previous one finished Line 7 displays Welcome followed by a space (but no newline) Line 8’s printf begins printing immediately following the space Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.2 A Simple C Program: Printing a Line of Text (13 of 15) 1. 2. 3. // fig02_02.c // Printing on one line with two printf statements. #include 4. 5. // function main begins program execution 6. int main(void) { 7. printf("Welcome "); 8. printf("to C!\n"); 9. } // end function main OUTPUT: – Welcome to C! Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.2 A Simple C Program: Printing a Line of Text (14 of 15) Displaying Multiple Lines with a Single printf One printf can display several lines Each \n moves the output cursor to the beginning of the next line Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.2 A Simple C Program: Printing a Line of Text (15 of 15) 1. // fig02_03.c 2. // Printing multiple lines with a single printf. 3. #include 4. 5. // function main begins program execution 6. int main(void) { 7. 8. printf("Welcome\nto\nC!\n"); } // end function main OUTPUT: Welcome to C! Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (1 of 16) scanf standard library function obtains information from the user at the keyboard Next program obtains two integers, then computes their sum and displays the result Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (2 of 16) 1. // fig02_04.c 2. // Addition program. 3. #include 4. // function main begins program execution 5. int main(void) { 6. int integer1 = 0; // will hold first number user enters 7. int integer2 = 0; // will hold second number user enters 8. 9. printf("Enter first integer: "); // prompt 10. scanf("%d", &integer1); // read an integer 11. Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (3 of 16) 1. printf("Enter second integer: "); // prompt 2. scanf("%d", &integer2); // read an integer 3. 4. int sum = 0; // variable in which sum will be stored 5. sum = integer1 + integer2; // assign total to sum 6. 7. 8. you argument have to this it is to write that clarify sum. L printf("Sum is %d\n", sum); // print sum & a - } // end function main 2 types of Print functions 1 :. Print that takes 2 Print. that talles a I argument arguments Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (4 of 16) OUTPUT: Enter first integer: 45 Enter second integer: 72 Sum is 117 Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (5 of 16) Variables and Variable Definitions Lines 7 and 8 are definitions. The names integer1 and integer2 are variables— locations in memory where the program can store values for later use integer1 and integer2 have type int—they’ll hold whole-number integer values Lines 7 and 8 initialize each variable to 0 Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (6 of 16) Define Variables Before They Are Used All variables must be defined with a name and a type before they can be used in a program You can place each variable definition anywhere in main before that variable’s first use in the code In general, you should define variables close to their first use Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (7 of 16) Identifiers and Case Sensitivity A variable name can be any valid identifier Each identifier may consist of letters, digits and underscores (_), but may not begin with a digit C is case sensitive, so a1 and A1 are different identifiers A variable name should start with a lowercase letter Choosing meaningful variable names helps make a program selfdocumenting Multiple-word variable names can make programs more readable – separate the words with underscores, as in total_commissions, or – run the words together and begin each subsequent word with a capital letter as in totalCommissions. Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (8 of 16) Prompting Messages Line 10 displays "Enter first integer: " This message is called a prompt – tells user to take a specific action Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (9 of 16) The scanf Function and Formatted Inputs Line 11 uses scanf to obtain a value from the user Reads from the standard input, usually the keyboard The "%d" is the format control string — indicates the type of data the user should enter (an integer) Second argument begins with an ampersand (&) followed by the variable name – Tells scanf the location (or address) in memory of the variable – scanf stores the value the user enters at that memory location Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (10 of 16) Prompting for and Inputting the Second Integer Line 13 prompts the user to enter the second integer Line 14 obtains a value for variable integer2 from the user. Loading… Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (11 of 16) Defining the sum Variable Line 16 defines the int variable sum and initializes it to 0 before we use sum in line 17. Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (12 of 16) Assignment Statement The assignment statement in line 17 calculates the total of integer1 and integer2, then assigns the result to variable sum using the assignment operator (=) Read as, “sum gets the value of the expression integer1 + integer2.” Most calculations are performed in assignments Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (13 of 16) Binary Operators The = operator and the + operator are binary operators— each has two operands Place spaces on either side of a binary operator to make the operator stand out and make the program more readable Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (14 of 16) Printing with a Format Control String The format control string "Sum is %d\n" in line 19 contains some literal characters to display ("Sum is ") and the conversion specification %d, which is a placeholder for an integer The sum is the value to insert in place of %d Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (15 of 16) Combining a Variable Definition and Assignment Statement You can initialize a variable in its definition For example, lines 16 and 17 can add the variables integer1 and integer2, then initialize the variable sum with the result: – int sum = integer1 + integer2; // assign total to sum Copyright © 2021 Pearson Education, Inc. All Rights Reserved 2.3 Another Simple C Program: Adding Two Integers (16 of 16) Calculations in printf Statements Actually, we do not need the variable sum, because we can perform the calculation in the printf statement Lines 16–19 can be replaced with – printf("Sum is %d\n", integer1 + integer2); Copyright © 2021 Pearson Education, Inc. All Rights Reserved