SWEN-601 Software Construction PDF
Document Details
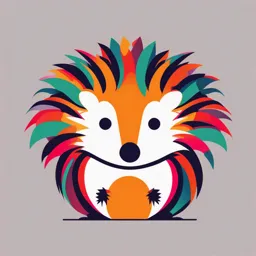
Uploaded by NonViolentThulium
Rochester Institute of Technology
SWEN-601
Tags
Related
- Week 3a - SWE202 Software Construction PDF
- SWEN-601 Software Construction PDF
- Introduction to Software Construction (CCSW 325) - University of Jeddah - PDF
- Projet de fin d’études (PFE) - Construction des outils autonomes pour le contrôle qualité - PDF
- 01_OO_Composition PDF - NC State University
- Software Entwicklung II: Reflection PDF
Summary
This document is a set of lecture notes on software construction, specifically covering variables, types, functions, and Java packages from SWEN-601. There are also activities and examples.
Full Transcript
SWEN-601 Software Construction Variables, Types, & Functions Java Packages 3 Activity: Accept the GitHub Classroom Assignment Your instructor has provided a GitHub classroom invitation. Yo...
SWEN-601 Software Construction Variables, Types, & Functions Java Packages 3 Activity: Accept the GitHub Classroom Assignment Your instructor has provided a GitHub classroom invitation. You should be able to find it under “Homework” on MyCourses. 1. Click the GitHub classroom invitation. You will be asked to accept a 2. Assuming that you have already linked your GitHub account with new assignment at the start of your name in the class roster, you should be prompted to accept nearly every class. the assignment. Do so. 3. Once the repository is created, copy the URL. You should get used to 4. Clone the repository to your local file system. As before, the accepting the assignment and starting your new project right repository will be empty. after you finish your quiz each 5. Create a new IntelliJ Project inside the repository, and push it to day. GitHub. 6. You are now ready to begin today’s activities! 4 Java Packages IntelliJ IDEA will flatten empty packages. Once Java programs very often include many more than one classes have been added, the packages will class. expand. Simply dumping all of the classes together in one big folder would quickly get very disorganized and difficult to manage. Thankfully, Java provides a mechanism for organizing your classes: the package. A package creates a namespace into which you can place Java classes that are closely related to each other. A package is named using words separated by dots, e.g. my.example.pkg. While packages may appear to be hierarchical, the namespaces are flat, i.e. There is no special relationship Packages are represented in the file system as between “my.example” and “my.example.pkg”. folders. Again, the folders may be hierarchical, but there is no relationship. 5 2 In the dialog, name your new package activities, and click OK. Activity: Creating a Package 3 This will create a new folder under your src 1 Right click on the src folder in your project folder. You should implement your solution to and select New → Package from the popup. all of today’s activities in this folder. 6 Statements, & The Head { Body } Pattern 7 Statements & Sequential Execution start A computer program comprises statements. ○ Also typically referred to as “lines of code.” Statement 1 ○ Statements contain instructions for what the computer should do when the statement is executed. ○ In Java, a typical statement begins at the start of a line and ends with a semicolon (;) on the same or a later line. Statement 2 Statements are executed in the order in which they are written into the program. ○ From top to bottom, beginning with the first line. ○ The program exits after the last statement is executed. Statement 3 The sequential execution of statements in a program is referred to as sequential flow of control. exit 8 The Head { Body } Pattern In Java the Head { Body } Pattern is used to structure computer programs. There is always some kind of Head, like a class or main method declaration. Head { The Body follows the Head and defines a block of 0 or more related statements. Body } The Body is almost always enclosed in a pair of curly braces ({ }) that indicate the start and end of the block of statements. As you write Java, you will see this pattern over and over again. 9 The Head { Body } Pattern public class HelloSwen601 { public static void main( String[] args ) { System.out.println(“Hello, SWEN-601!”); } The Body comprises the statements between the curly } braces. 10 The Head { Body } Pattern You will see that most Java programs repeat the pattern over The main method declaration is public class HelloSwen601 { another Head... public static void main( String[] args ) { System.out.println(“Hello, SWEN-601!”); }...and the statements inside of the main method are another Body. } 11 The Head { Body } Pattern Unlike some other languages, in Java, whitespace is insignificant. This means that the following blocks are equivalent to the compiler. Head { Head { Body Stmt 1; Body Stmt 2; Body Stmt 3; } Body Stmt 1; Body Stmt 2; Body Head { Stmt Body Stmt 1; Body Stmt 2; 3; Body Stmt 3; } } However, stylistic convention says that whitespace is used to improve readability of the code for humans. ○ This typically means that your code should look like the first example in most cases. 12 Types, Literals, Variables, Scope, & Expressions 13 Java Primitive Types Types Name Description Example(s) byte 8-bit signed integer. -128, 127 Very often a statement in a program needs to use some short 16-bit signed integer. 12876 values computed by or provided by the computer. Every value has a specific type. In Java, there are two int 32-bit signed integer. -21474836 broad categories of types: primitives and references. long 64-bit signed integer. 4284866286 Primitive Types are the basic building blocks of every other type. They include: 32-bit signed floating point. float 3.14159 ○ Numbers - integers and floating point numbers. ○ Characters - an individual letter, number, or symbol. double 64-bit signed floating point. -14657.241 ○ Booleans - values that are either true or false. Reference Types combine primitives and other 16-bit unsigned integer type; a char ‘a’, ‘b’, references to create more complex compound single Unicode character. ‘!’, ‘&’ structures. ○ Strings - So far these are the only reference type we have boolean true, used. false ○ We will talk about more soon! 14 Literals A literal is a value that is typed directly into code. ○ Tells the computer to “literally use this value.” No computation is required. For example: ○ Numbers: 1, 5, -127436, 3.14159, 98.6, etc. ○ Characters: 'a', 'Z', '3', '&', etc. ○ Strings: "Buttercup", "My Dear Aunt Sally", While Strings are reference types, they are "August 27th, 2019", etc. very special in Java. ○ Booleans: either true or false. Remember, a single character (char) is a primitive type and is enclosed in single quotes ('). They are afforded many of the same features as primitive types, including the ability to use a A String is a reference type and is enclosed in String literal in your code. double quotes ("). 15 Activity: Literally Printing Create a new Java class named Literally. Add a main method. Use System.out to print a few literals including at least the following: ○ One natural number. Make sure to create your new class ○ One floating point number. inside the activities package! ○ A character. ○ A String. ○ A boolean. If you accidentally create it in the wrong place, just drag it into the package. Run your program. Push your code to GitHub. 16 Variable Table Variables Name Type Address x int 0x1000 pi float 0x2000 A computer is capable of storing, retrieving, and manipulating information. A variable table (also called a symbol table) The information is stored in and retrieved from keeps track of the address in memory to which each variable refers. memory. A variable is a name for a location in memory. Memory ○ This is an alternative to requiring that the programmer keep track of the specific address of a Address Value needed value. 0x1000 27 The value stored in the variable’s location in memory can, and frequently will, change. 0x2000 3.14159 ○ When the variable name is used in code, the most The value for each variable is stored in the recently stored value is substituted in its place. corresponding address in memory. If the There are a few rules related to variables. variable is changed, the value in memory is overwritten. 17 (Some) Variable Rules // a variable cannot be used before // the value of the variable may // it is declared. // be changed at any time System.out.println(x); // error x = 22; // a variable is declared with a // trying to assign a value of the // type and a name. // wrong type is a syntax error int x; x = false; // a variable cannot be used before // a value has been assigned There are lots of other rules related to variables, System.out.println(x); // error but we aren’t ready to talk about them yet. We will add more as we continue to learn about Java. // a literal value of the correct // type may be assigned x = 17; 18 Scope public class Variables { public static void main(String[] args) { // x scope begins Scope refers to the parts of a program in which a int x = 5; variable may be used. Recall the Head { Body } pattern discussed System.out.println(x + 7); earlier. // y scope begins ○ A variable must be declared within the Body of char y = ‘O’; something, e.g. the Body of the main method. x ○ A variable’s scope begins when it is declared and ends // z scope begins at the curly brace (}) at the end of the Body. char z = ‘k’; Trying to use a variable that is out of scope will y cause a syntax error. System.out.print(y); z ○ Your program will not compile. System.out.println(z); Trying to declare a second variable with the same } // x, y, z scope ends name as another variable that is in scope will also } cause a syntax error. 19 Expressions // an expression can be used as the An expression tells the computer how to compute a // value assigned to a variable value. float x = 22.5 * 13.6 / 12; An expression combines one or more values together using operators to compute some new // the standard order of operations is resulting value. // used (PMDAS) There are different operators for different data int y = 12 + 10 / 2 * 6; types. ○ Numbers - arithmetic - add (+), subtract (-), multiply // variables may be used in expressions (*), divide (/), modulo (%) float z = x + y / 3.1; ○ Strings - concatenation - "foo" + "bar" ○ Booleans - logical operators - and (&&), or (||), not (!) Whenever a program requires a value, either an // in fact, a variable may be used to expression or a literal may be used. // change its own value ○ Provided that it is the right kind of value! x = x + 4; 20 Activity: Express Yourself Create a new Java class named Expressions. Add a main method. Declare three variables of any numeric type. ○ Use an expression to assign a value to each variable. ○ At least one expression must use one variable to assign a value to another, e.g. int z = x + y; Make sure to create your new class inside the activities package! Print the name and value of all three variables. Push your code to GitHub. If you accidentally create it in the wrong place, just drag it into the package. 21 Functions, Parameters, & Return Values 22 Functions It is often the case that you would like to execute the same Functions are the primary mechanism for reuse in programming languages. code more than once. You could copy and paste the code, but that has lots of Rather than duplicating code (by copying and drawbacks. pasting or retying), a function is called ○ Adds lots of extra code. wherever and whenever the code in its body needs to be executed. ○ Duplicates bugs. ○ Changes need to be made in multiple places. You’ve already written several variations of the Virtually all programming languages provide an alternative main function, which is automatically called to cut-and-paste coding: functions. whenever your program is executed. A function encapsulates a block of statements. ○ This block is contained within the Body of the function. Let’s take a look at writing other functions, and then calling them from main (or even each Each time you want to execute the statements in the other). function, you write a call the function. ○ The statements in the Body of the function are executed. 23 Anatomy of a Function You have already seen the main function, so most of this should be familiar to you by now. Like many other Java constructs, functions follow the Head { Body } pattern. public class Functions { The Head is the declaration which must include the name of the new function, e.g. sayMyName. public static void sayMyName() { System.out.println(“Bobby”); The Body of the function is enclosed in curly } braces ({}) and should include any statements that you would like to execute when the function is called. public static void main(String[] args) { sayMyName(); The function is called using its name. Each sayMyName(); time it is called, the statements in its Body will sayMyName(); be executed. } } 24 Activity: Printing Pets B u Create a new class in the activities package t called “PetNames.” t e Write a function that prints your favorite pet’s r name to standard output with one letter on each c line. u ○ If you don’t have any pets, you may use your own p B name or the name of a friend. u Write a main function, and call your new... function at least twice. When you are finished, push your code to The first several lines of your output should look something like this. GitHub. 25 Parameters It is often the case that a function needs one or more A parameter is a variable that is declared as input values in order to do its work. part of the method’s signature. ○ A function that adds two numbers and prints the result. ○ A function that converts temperatures from Celsius to Like any other variable, a parameter is Fahrenheit. declared with a type and a name and must be assigned a value before it can be used. ○ etc. A function may declare zero or more parameters as part The arguments to the function are used to of its declarations. assign a value to each parameter. ○ Parameters are declared between the parentheses after the function’s name. The scope of the parameter is the entire Body ○ Each parameter must be declared with a type and a name. of the function. When the function is called, a value of the correct type must be specified for each parameter. ○ These values are arguments to the function. 26 Function Parameters Parameters are declared as part of the method signature, between the parentheses. Each parameter must be declared with a type and a name. public static void sayHello(String name, int age) { System.out.println(“Hello, ” + name + The scope of the parameters is the Body of the function, and so they can be used “you are ” + age + “ years old!”); anywhere in the function. } sayHello(“Bobby”, 44); When the function is called, an argument of sayHello(“Buttercup”, 8); the correct type must be provided for each parameters. sayHello(“President Obama”, 58); 27 Activity: Arithmetic Create a new class in the activities package called x=4.0, y=2.0 “Calculator.” x + y = 6.0 Write a function that takes two floating point parameters x * y = 12.0 x and y. It should print the following: x - y = 2.0 ○ The values for both x and y x / y = 2.0 ○ x + y = ? ○ x * y = ? Your output each time that ○ x - y = ? you call the function should ○ x / y = ? look similar to this. Write a main function, and call three times with different values. When you are finished, push your code to GitHub. 28 Return Values All functions in Java must declare a return type. The return type indicates the type of value that will be returned when the function is called. A return type of void indicates that the function does not If a method declares a return type other than return a value. void and does not return a value, this will ○ So far, all of the functions that we have written have cause a compiler error. declared a void return type. Any code that follows a return statement is If the function declares a return type other than void, it unreachable. Any such code will also cause a must return a value of that type. compiler error. ○ This is done using a return statement. Finally, if a method returns a type that is not ○ The return statement must return a value that compatible with its declared return type, this matches the declared return type. will also cause a compiler error. ○ The return must be the last statement in the method. 29 Returning from Functions The return type is declared as part of the method declaration. All of our previous examples declared a void return type, and so a return statement was not necessary. public static float cubed(float base) { System.out.println(“Cubing: ” + base); This function declares a float return type, and return base * base * base; so must include a return statement that returns a floating point value. } When the function is called, the returned value cubed(5.7); may be ignored. float result = cubed(3.14159); Or it may be used, e.g. assigned to a variable System.out.println(cubed(2.5)); or printed to standard output. 30 Activity: Pounds to Kilograms pounds: 186.2 Create a new class in the activities package called kilos: 84.63636 “WeightConverter.” pounds: 207.0 Write a function that declares a floating point parameter kilos: 94.090904 for a weight in pounds: ○ Prints the weight in pounds. total: 178.72726 ○ Calculates the weight in kilograms (there are 2.2 pounds to a kilogram) and prints it. Your output should look ○ Returns the weight in kilograms. something like this. Write a main function that calls your function twice and prints the total weight returned. When you are finished, push your code to GitHub. 31 JavaDoc for Functions As mentioned last time, JavaDoc is a special kind of * JavaDoc can be automatically converted into HTML * @param x The first number to add. format to create a web-viewable document of your * @param y The second number to add. code. * JavaDoc is used to document Java functions, * @return The sum of the two numbers. including: */ public int adder(int x, int y) { ○ A description of the function. return x + y; ○ The name and purpose of each parameter. } ○ The return type (if any) and a description of the expected values. In IntelliJ IDEA, typing /** just before a function and From this point forward, you will be expected to write pressing enter will generate a stubbed JavaDoc that JavaDoc for all of your functions (including main). you can fill in. 32 Standard Input 33 // you will want to import the class Reading Standard import java.util.Scanner; Input With Scanner public class Input { public static void main(String[] args) { Just like System.out is used to send output to the // create a scanner to read from standard // input (System.in) terminal, System.in can be used to read input typed Scanner scanner = new Scanner(System.in); by the user into the terminal. ○ This is referred to as standard input. // read the next line However, System.in is fairly complicated and String line = scanner.nextLine(); difficult to use. A much easier alternative is the java.util.Scanner class. // read the next word It provides several useful methods, including: String word = scanner.next(); ○ next() - returns the first word that the user typed (up to the first space). // read the next word as an int ○ nextLine() - reads up to the end of the next line of int number = scanner.nextInt(); input (up until the user pressed the enter key). } ○ nextInt() - reads the next work and returns it as an } int. ○ nextFloat() - reads the next word and returns it as a A call to one of Scanner’s methods will block (your float. program will pause) until input is available. ○ etc. Refer to the online documentation for many more. 34 Activity: More Arithmetic Enter two numbers: 4.0 2.0 Modify your Calculator class so that the main x=4.0, y=2.0 method uses a Scanner to prompt the user to x + y = 6.0 enter the values for x and y. x * y = 12.0 x - y = 2.0 ○ Hint: Use System.out.print for the x / y = 2.0 prompt. Call your function to print the result of the 4 Your output should look something like arithmetic operations (+, *, -, /). this. When you are finished, push your code to GitHub. 35