Week-12 Assignment_July_2024.pdf
Document Details
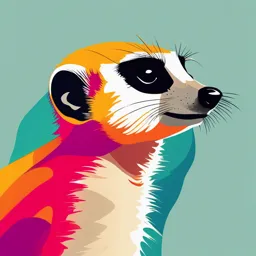
Uploaded by WellMadeGraph8884
IIT
Full Transcript
NPTEL Online Certification Courses Indian Institute of Technology Kharagpur DATA STRUCTURES AND ALGORITHMS USING JAVA Assignment-12 TYPE OF QUESTION: MCQ Numbe...
NPTEL Online Certification Courses Indian Institute of Technology Kharagpur DATA STRUCTURES AND ALGORITHMS USING JAVA Assignment-12 TYPE OF QUESTION: MCQ Number of questions: 10 Total marks: 10×1 = 10 ______________________________________________________________________________ Note: Assume all necessary imports are included in the code snippets wherever required. ______________________________________________________________________________ QUESTION 1: What will the given program print? public class createStringDemo { public static void main(String args[]) { char[] aSet = {'a', 'e', 'i', 'o', 'u', ' ', ' ', '5', '#'}; System.out.println(aSet); } } a. The program will throw a runtime exception because char[] cannot be printed directly using System.out.println(). b. The program will print each character on a new line. c. aeiou 5# d. aeiou null 5# Correct Answer: c Detailed Solution: run and check. Refer to slide #4 ___________________________________________________________________________ NPTEL Online Certification Courses Indian Institute of Technology Kharagpur QUESTION 2: What will be the output of the code snippet provided below? Note: assume all necessary imports. public class TrimStringDemo{ public static void main(String args[]){ String text = " Data Structure with Java "; String text2 = text.trim(); String text3 = text.toUpperCase(); String text4 = text.replace("C++" ,"Java"); char text5 = text.charAt(5); System.out.println(text2 ); System.out.println(text3 ); System.out.println(text4 ); System.out.println(text5 ); } } a. Data Structure with Java DATA STRUCTURE WITH JAVA Data Structure with Java t b. Data Structure with Java DATA STRUCTURE WITH JAVA Data Structure with Java t c. Data Structure with Java DATA STRUCTURE WITH JAVA Data Structure with C++ t NPTEL Online Certification Courses Indian Institute of Technology Kharagpur d. Data Structure with Java DATA STRUCTURE WITH JAVA Data Structure with Java t Correct Answer: d Detailed Solution: Run and try ___________________________________________________________________________ QUESTION 3: Consider the following Java program public class createStringDemo { public static void main(String args[]) { String text = "DATA STRUCTURES AND ALGORITHMS USING JAVA"; System.out.print(text); } } Which of the following is NOT true about the output or behavior of the program? a. The program will print the text exactly as it is: DATA STRUCTURES AND ALGORITHMS USING JAVA. b. The System.out.print() method prints the string without adding a newline character at the end. c. The string text is immutable, and any further operations on text would result in the creation of a new string. d. Changing System.out.print(text) to System.out.println(text) will cause the program to throw a NullPointerException. Correct Answer: d Detailed Solution: Option (a) is true: The program prints the string exactly as it is. Option (b) is true: System.out.print() does not add a newline character after printing. Option (c) is true: Strings are immutable in Java. NPTEL Online Certification Courses Indian Institute of Technology Kharagpur Option (d) is false: Changing System.out.print() to System.out.println() will not cause a NullPointerException. Both print and println work the same way, except println adds a newline after the string. There is no NullPointerException thrown here. ___________________________________________________________________________ QUESTION 4: What will be the output of code snippet given below? public class StringCompareCaseDemo{ public static void main(String args[]) { String text1 = "DATA STRUCTURE WITH JAVA"; String text2 = "DATA STRUCTURE WITH C++"; String text3 = "DATA STRUCTURE WITH JAVA"; String text4 = "data structure with c++"; String text5 = "data structure with java"; int output1 = text1.compareToIgnoreCase(text2); int output2 = text1.compareToIgnoreCase(text3); int output3 = text1.compareToIgnoreCase(text4); int output4 = text1.compareToIgnoreCase(text5); System.out.println(output1,output2,output3,output4); }} a. 7 0 7 0 b. 0 7 0 7 c. 7 0 7 0 d. error Correct Answer: d Detailed Solution: StringCompareCaseDemo.java:13: error: no suitable method found ___________________________________________________________________________ QUESTION 5: NPTEL Online Certification Courses Indian Institute of Technology Kharagpur Which of the following function definitions is/are Correct? a. String trim() Returns the copy of the String, by removing whitespaces at both ends. It does not affect whitespaces in the middle. b. String toUpperCase() Converts all the characters in the String to upper case. c. String replace(char old, char new) Returns new string by replacing all occurrences of old with new. d. String concat(String s) Concatenates specified string to the front of this string Correct Answer: a,b,c Detailed Solution: Option ‘d’ is wrong as it says “String concat(String s) Concatenates specified string to the front of this string” but the actual statement should be “String concat(String s) Concatenates specified string to the end of this string” ___________________________________________________________________________ QUESTION 6: Consider the code snippet given below: public class StringEqualsCaseDemo{ public static void main(String args[]){ String text1 = "DATA STRUCTURE WITH JAVA"; String text2 = "DATA STRUCTURE WITH C++"; String text3 = "DATA STRUCTURE WITH JAVA"; String text4 = "data structure with c++"; String text5 = "data structure with java"; Boolean output1 = text1.equalsIgnoreCase(text2); Boolean output2 = text1.equals(text3); int output3 = text1.compareTo(text4); int output4 = text1.compareToIgnoreCase(text5); System.out.println(output1); System.out.println(output2); System.out.println(output3); System.out.println(output4); } } a. false true NPTEL Online Certification Courses Indian Institute of Technology Kharagpur 0 0 b. true true -32 0 c. false true -32 -32 d. false true -32 0 Correct Answer: d Detailed Solution: Execute code and check ___________________________________________________________________________ QUESTION 7: Consider the code given below. NPTEL Online Certification Courses Indian Institute of Technology Kharagpur import java.lang.*; class StringBufferDemo { public static void main(String args[]) { StringBuffer strOb1 = new StringBuffer("First String"); StringBuffer strOb2 = new StringBuffer("Second String"); String strOb3 = strOb1 + " and "+strOb2; System.out.println(strOb1); System.out.println(strOb2); System.out.println(strOb3); StringBuffer sb1 = new StringBuffer(); StringBuffer sb2 = new StringBuffer(strOb3); StringBuffer aString = new StringBuffer("An example of string is" + sb2); StringBuffer text = new StringBuffer("Data Structure "); text.append(“C++"); System.out.println(text); text.insert(15,"with "); System.out.println(text); text.replace(20,23,"Java"); System.out.println(text); text.delete(14,19); text.reverse(); System.out.print(text); } } a. First String Second String First String and Second String Data Structure C++ Data Structure with C++ Data Structure with Java avaJtureC b. First String Second String First String and Second String Data Structure C++ Data Structure with C++ Data Structure with CJava Java Structure NPTEL Online Certification Courses Indian Institute of Technology Kharagpur c. First String Second String First String and Second String Data Structure C++ Data Structure with C++ Data Structure with Java avaJ erutcurtS ataD d. Compilation Error due to the expression `strOb1 + " and " + strOb2;` Correct Answer: c Detailed Solution: The code uses StringBuffer for strOb1 and strOb2. Concatenating strOb1 + " and " + strOb2 converts them to String type, resulting in "First String and Second String". Each System.out.println() prints the values accordingly. The operations on text manipulate the string buffer, appending, inserting, replacing, deleting, and reversing characters. _________________________________________________________________________ QUESTION 8: What will be the output of the code snippet given below- class StringBufferDemo { public static void main(String args[]) { StringBuffer sb = new StringBuffer("Hello"); System.out.println("buffer = " + sb); System.out.println("length = " + sb.length()); System.out.println("capacity = " + sb.capacity()); sb.setCharAt(1, 'i'); sb.setLength(2); System.out.println("buffer after = " + sb); System.out.println("charAt(1) after = " + sb.charAt(1)); } NPTEL Online Certification Courses Indian Institute of Technology Kharagpur } a. buffer = Hello length = 5 capacity = 5 buffer after = Hi charAt(1) after = i b. buffer = Hello length = 5 capacity = 21 buffer after = Hillo charAt(1) after = i c. buffer = Hello length = 5 capacity = 21 buffer after = Hi charAt(1) after = i d. buffer = Hello length = 5 capacity = 21 buffer after = Hi charAt(1) after = H Correct Answer: c Detailed Solution: buffer = Hello length = 5 capacity = 21 buffer after = Hi charAt(1) after = i ___________________________________________________________________________ QUESTION 9: NPTEL Online Certification Courses Indian Institute of Technology Kharagpur What will be the output of the code snippet given below? import java.lang.*; class BasicStringDemo { public static void main(String args[]) { String strOb1 = "First String"; String strOb2 = "Second String"; String strOb3 = strOb1 + " and " + strOb2; System.out.println(strOb1); System.out.println(strOb2); System.out.println(strOb3); String myString = "Joy with Java"; System.out.println(myString); System.out.println("Welcome"); System.out.println("Welcome" + " " + myString); String aString = "An example of string is " + myString; System.out.println(aString); } } Choose from the options given below- a. First String Second String First String and Second String Joy with Java Welcome Welcome An example of string is b. First String Second String First String and Second String Welcome Joy with Java Welcome Joy with Java An example of string is Joy with Java c. NPTEL Online Certification Courses Indian Institute of Technology Kharagpur First String Second String First String and Second String Joy with Java Welcome Welcome Joy with Java An example of string is Joy with Java d. First String Second String First String and Second String Joy with Java Welcome Welcome An example of string is Correct Answer: c Detailed Solution: Execute this code ___________________________________________________________________________ QUESTION 10: What will be the output of the code snippet given below? public class StringLengthDemo{ public static void main(String args[]){ String text = "DATA STRUCTURE WITH JAVA"; int stringLength = text.length(); System.out.print(stringLength); } } a. 24 b. 21 c. 25 d. 22 Correct Answer: a Detailed Solution: Run and check ___________________________________________________________________________ NPTEL Online Certification Courses Indian Institute of Technology Kharagpur ************END************