Question On Python PDF
Document Details
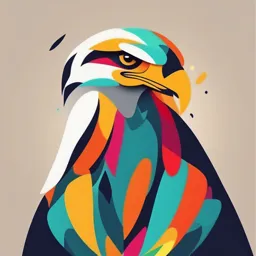
Uploaded by NiceEpic8588
Tags
Summary
This document contains questions and answers on Python programming, a high-level interpreted programming language widely used in various applications. The content covers topics such as the definition of Python, its creator, benefits of use, interpreted language, dynamic typing, and compiled languages. This is useful for learners who want to understand Python programming and its related concepts.
Full Transcript
❔ Question On Python Flashcard Tracker What is python? Python is high level interpretated programing language which is widely use for variety application due to its simplicity, versatility, vast library of package a...
❔ Question On Python Flashcard Tracker What is python? Python is high level interpretated programing language which is widely use for variety application due to its simplicity, versatility, vast library of package and modules. The one of the main advantage of python is ease of use and readability which makes it accessibility for beginner and allow faster development time. additionally python syntax is clear and concise which reduce likelihood of error and make it easy to maintain. Who create python? It is create by Guido Van Rossum in 1991 What is benefit of using of python ? 1. Object Oriented Language 2. High Level Language 3. Dynamically Type Language 4. Extensive support for Machine Learning Libraries Question On Python 1 5. Presence of third-party modules 6. Open source and community development 7. Portable and Interactive 8. Portable across Operating systems What is interpreted language? This relates to how the code is executed. Definition: In an interpreted language, code is executed line by line using an interpreter, rather than being compiled into machine code beforehand. Key Points: No separate compilation step is required. Errors are found during execution (runtime). Examples: Python, JavaScript, Ruby. Advantages: Easier debugging and testing. Code can be platform-independent (since the interpreter takes care of translating to machine code). Disadvantages: Slower execution compared to compiled languages (like C or C++) since translation happens at runtime. What is Dynamically Type Language? This relates to how variables are handled. Definition: In a dynamically typed language, variable types are determined at runtime, and you don't need to declare the variable's type explicitly. Key Points: No need to specify types (e.g., int , float ) when declaring variables. Variable types can change during execution. Question On Python 2 Examples: Python, Ruby, JavaScript. Advantages: Simplified syntax and flexibility. Faster prototyping and development. Disadvantages: Can lead to runtime errors if types are misused. Harder to enforce type consistency, which might reduce code reliability. What is Compile Language? A compiled language is a type of programming language in which the code written by the developer (source code) is transformed into machine code (binary) by a compiler before it is executed. The resulting machine code is a standalone file (like an executable) that can run directly on the target hardware without needing the original source code or a separate interpreter. Key Characteristics of Compiled Languages 1. Compilation Process: Source code is written by the developer. A compiler translates the source code into machine code (binary) in one go. The resulting file (e.g.,.exe on Windows) can be executed on the target machine. 2. Fast Execution: Since the program is already converted into machine code, it runs directly on the hardware, making compiled languages faster than interpreted ones. 3. Platform-Specific: Compiled machine code is specific to the operating system and hardware it was compiled for. For example, a binary compiled for Windows may not run on Linux. 4. Error Detection: Question On Python 3 Errors (e.g., syntax errors, type mismatches) are detected during the compilation process, preventing the program from running until it is error-free. 5. Examples: C, C++, Rust, Go, and Fortran. Advantages of Compiled Languages Performance: Compiled code is highly optimized for speed and efficiency. Error Checking: Many errors are caught during compilation, reducing runtime issues. Independent Execution: No need for a separate interpreter or source code at runtime. Disadvantages of Compiled Languages Slower Development Cycle: Compilation adds an extra step to the development process. Less Flexibility: The compiled code is platform-specific unless cross- compilation tools are used. Harder Debugging: Debugging can be more complex since errors are caught before execution. Comparison: Compiled vs Interpreted Aspect Compiled Languages Interpreted Languages Translated to machine code Translated line-by-line at Execution before running. runtime. Faster, as it directly runs on Slower due to real-time Performance hardware. interpretation. Platform-independent (requires Portability Platform-specific binary. an interpreter). Errors caught during Error Detection Errors caught during runtime. compilation. Examples C, C++, Rust, Go. Python, JavaScript, Ruby. Question On Python 4 What is opposite of Dynamic Type Language? Statically typed languages: In this type of language, the data type of a variable is known at the compile time which means the programmer has to specify the data type of a variable at the time of its declaration. How do you debug a Python program? By using this command we can debug a Python program: $ python -m pdb python-script.py Is Indentation Required in Python? Yes, indentation is required in Python. A Python interpreter can be informed that a group of statements belongs to a specific block of code by using Python indentation. Indentations make the code easy to read for developers in all programming languages but in Python, it is very important to indent the code in a specific order. What is the difference between a shallow copy and a deep copy? The difference between shallow copy and deep copy lies in how they duplicate objects and the level of nested structures they replicate. Shallow Copy A shallow copy creates a new object, but does not recursively copy nested objects (e.g., objects inside lists or dictionaries). Instead, it copies references to those nested objects. Effect: The outer object is copied, but the inner objects (nested structures) are shared between the original and the copy. Implementation: In Python, a shallow copy can be created using the copy() method or the copy module's copy() function. Example: import copy Question On Python 5 original = [[1, 2, 3], [4, 5, 6]] shallow = copy.copy(original) # Modify nested structure shallow = 99 print(original) # [[99, 2, 3], [4, 5, 6]] (Nested objec ts are shared) print(shallow) # [[99, 2, 3], [4, 5, 6]] Deep Copy A deep copy creates a new object and recursively copies all nested objects, so the new object is completely independent of the original. Effect: Both the outer object and all nested objects are entirely copied, and no references are shared between the original and the copy. Implementation: In Python, a deep copy can be created using the copy module's deepcopy() function. Example: import copy original = [[1, 2, 3], [4, 5, 6]] deep = copy.deepcopy(original) # Modify nested structure deep = 99 print(original) # [[1, 2, 3], [4, 5, 6]] (Nested object s are independent) print(deep) # [[99, 2, 3], [4, 5, 6]] Key Differences Feature Shallow Copy Deep Copy Outer Object Creates a new object. Creates a new object. Question On Python 6 Nested References are shared with the Completely independent Objects original. copy. Changes to nested objects affect Changes to nested objects do Independence both the original and the copy. not affect each other. When you want to copy the outer When you need a completely Use Case object but share inner objects. independent copy. When to Use Shallow Copy: When the nested objects are large or immutable, and you want to avoid duplicating them for performance reasons. Deep Copy: When you need full independence between the copied object and the original, especially when dealing with mutable nested objects. Which sorting technique is used by sort() and sorted() functions of python? The sort() and sorted() functions in Python use the Timsort algorithm. Timsort is a hybrid sorting algorithm derived from merge sort and insertion sort. It is designed to perform well on many kinds of real-world data by taking advantage of patterns like partially sorted sequences. Difference between sort() and sorted()? How sort() and sorted() Work list.sort() : A method that sorts a list in-place, modifying the original list. my_list = [5, 2, 9, 1] my_list.sort() print(my_list) # Output: [1, 2, 5, 9] sorted() : A built-in function that returns a new sorted list while leaving the original data unchanged. Question On Python 7 my_list = [5, 2, 9, 1] sorted_list = sorted(my_list) print(sorted_list) # Output: [1, 2, 5, 9] print(my_list) # Output: [5, 2, 9, 1] Question On Python 8