Python Data Types Lecture Notes PDF
Document Details
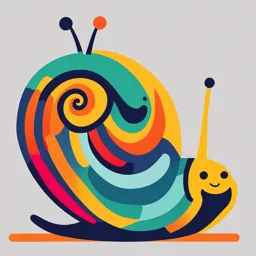
Uploaded by UnrealAutomatism
Al Salam University College
Tags
Related
- Ch-2 Python Introduction PDF
- Lecture 1 - MUH101 Introduction to Programming - PDF
- BSc (Hons) Electronics Ist Sem - Programming Fundamentals using Python PDF
- Computer Science Class XI Past Paper PDF 2023-24
- Meeting 2 - Fundamentals of Python Programming - s.en.ar.pptx PDF
- Cours Python - Programmations: Concepts Fondamentaux - Pratique (PDF)
Summary
This document is a lecture on Python data types. It provides an introduction and overview of different data types such as numeric, sequence, mapping, and set types, along with examples. The lecture likely covers various operations and characteristics of these data types.
Full Transcript
**Lecture Title: Understanding Python Data Types** **Introduction** Good \[morning/afternoon\], everyone! Today, we are diving into one of the most fundamental topics in Python programming: **Data Types**. Data types in Python define the kind of value a variable can hold and determine the operatio...
**Lecture Title: Understanding Python Data Types** **Introduction** Good \[morning/afternoon\], everyone! Today, we are diving into one of the most fundamental topics in Python programming: **Data Types**. Data types in Python define the kind of value a variable can hold and determine the operations that can be performed on them. Whether you\'re building a simple script or a complex application, understanding Python data types is critical for writing efficient and bug-free code. **Learning Objectives** By the end of this lecture, you should be able to: 1. Identify and classify the various data types in Python. 2. Understand the properties and operations associated with each data type. 3. Write Python programs that effectively use data types. **1. Why Are Data Types Important?** - They determine what kind of operations can be performed on data. - They help prevent errors and make code more readable. - Python is dynamically typed, meaning you don\'t need to declare a variable\'s type explicitly; the interpreter infers it at runtime. Example: x = 10 \# Integer y = \"Hello\" \# String z = 3.14 \# Float **2. Built-in Python Data Types** Python has several built-in data types that can be broadly categorized into the following groups: **A. Numeric Types** 1. **Integer (int)**: Whole numbers, e.g., 10, -5. 2. **Floating-point (float)**: Decimal numbers, e.g., 3.14, -0.01. 3. **Complex (complex)**: Numbers with a real and imaginary part, e.g., 1 + 2j. Examples: a = 42 \# int b = 3.14159 \# float c = 2 + 3j \# complex Key operations: - Addition, subtraction, multiplication, division - int(), float(), complex() for type conversion **B. Sequence Types** 1. **String (str)**: A collection of characters enclosed in single (\') or double (\") quotes. 2. **List (list)**: An ordered, mutable collection of items. 3. **Tuple (tuple)**: An ordered, immutable collection of items. Examples: \# String name = \"Python\" \# List numbers = \[1, 2, 3, 4\] \# Tuple coordinates = (10, 20) Key operations: - Indexing: name\[0\], numbers\[1\] - Slicing: name\[:3\], numbers\[1:3\] - Methods:.append(),.remove() for lists, but tuples are immutable. **C. Mapping Type** 1. **Dictionary (dict)**: A collection of key-value pairs. Example: person = {\"name\": \"Alice\", \"age\": 25, \"city\": \"New York\"} Key operations: - Access values: person\[\"name\"\] - Add new key-value pairs: person\[\"job\"\] = \"Engineer\" **D. Set Types** 1. **Set (set)**: An unordered collection of unique elements. 2. **Frozen Set (frozenset)**: An immutable version of a set. Examples: fruits = {\"apple\", \"banana\", \"cherry\"} unique\_numbers = frozenset(\[1, 2, 3, 3, 2\]) Key operations: - Union: set1 \| set2 - Intersection: set1 & set2 **E. Boolean Type** 1. **Boolean (bool)**: Represents True or False. Examples: is\_python\_fun = True is\_cold = False Key operations: - Logical operations: and, or, not **F. None Type** 1. **NoneType (None)**: Represents the absence of a value. Example: value = None **3. Mutable vs. Immutable Data Types** - **Mutable**: Can be changed after creation (e.g., lists, dictionaries, sets). - **Immutable**: Cannot be changed after creation (e.g., strings, tuples, numbers). Example of mutability: \# Mutable my\_list = \[1, 2, 3\] my\_list.append(4) \# \[1, 2, 3, 4\] \# Immutable my\_tuple = (1, 2, 3) \# my\_tuple\[0\] = 0 \# This will raise an error **4. Type Conversion** Python allows you to convert between data types: - Implicit: Done automatically by Python. - Explicit: Done using built-in functions like int(), float(), str(). Example: \# Implicit conversion x = 10 y = 2.5 z = x + y \# z becomes float (12.5) \# Explicit conversion a = \"123\" b = int(a) \# b is now an integer **5. Checking Data Types** You can use the type() function to check the type of a variable: x = 42 print(type(x)) \# Output: \ **6. Common Mistakes to Avoid** 1. Mixing incompatible types in operations: 2. x = 10 3. y = \"20\" 4. z = x + y \# This will raise a TypeError 5. Forgetting that some types are immutable: 6. my\_string = \"hello\" 7. my\_string\[0\] = \"H\" \# This will raise a TypeError **7. Practical Exercises** 1. Create a program that takes user input, identifies its type, and converts it to another type. 2. Write a script to perform set operations (union, intersection) on two user-defined sets. 3. Create a dictionary to store details about a person and add, modify, and delete keys. **8. Summary** - Python provides a rich set of data types to handle diverse programming needs. - Understanding the properties and behavior of each data type is crucial for writing effective code. - Practice working with different data types to become more confident in Python programming. **Questions and Discussion** Feel free to ask questions or share your thoughts about today\'s topic. Let\'s discuss any doubts you may have about Python data types! **Homework** 1. Write a Python program to demonstrate type conversion. 2. Explore Python\'s isinstance() function and test it with various data types. That concludes our lecture on Python Data Types. Thank you, and happy coding! **Common errors in using python data types** Here are some **common errors** programmers encounter when working with Python data types, along with examples and explanations to help avoid them: **1. Mixing Incompatible Data Types** Attempting to perform operations on variables of incompatible types without proper type conversion leads to errors. **Example:** x = 10 y = \"20\" z = x + y \# Raises TypeError: unsupported operand type(s) for +: \'int\' and \'str\' **Solution:** Use explicit type conversion: z = x + int(y) \# Now works, z = 30 **2. Indexing or Slicing Errors** Accessing invalid indices in sequences like strings, lists, or tuples raises an IndexError. **Example:** my\_list = \[1, 2, 3\] print(my\_list\[5\]) \# Raises IndexError: list index out of range **Solution:** Check the length of the sequence using len() before accessing indices: if 5 \< len(my\_list): print(my\_list\[5\]) **3. Modifying Immutable Data Types** Immutable types (e.g., strings, tuples) cannot be changed after creation. Attempts to modify them result in errors. **Example:** my\_tuple = (1, 2, 3) my\_tuple\[0\] = 10 \# Raises TypeError: \'tuple\' object does not support item assignment **Solution:** Create a new object instead: new\_tuple = (10,) + my\_tuple\[1:\] \# New tuple: (10, 2, 3) **4. Mutable Default Arguments in Functions** Using mutable data types (e.g., lists, dictionaries) as default arguments in functions can lead to unexpected behavior. **Example:** def add\_to\_list(item, my\_list=\[\]): my\_list.append(item) return my\_list print(add\_to\_list(1)) \# Output: \[1\] print(add\_to\_list(2)) \# Output: \[1, 2\] (unexpected behavior!) **Solution:** Use None as the default value and initialize inside the function: def add\_to\_list(item, my\_list=None): if my\_list is None: my\_list = \[\] my\_list.append(item) return my\_list **5. Key Errors in Dictionaries** Trying to access a non-existent key in a dictionary raises a KeyError. **Example:** person = {\"name\": \"Alice\", \"age\": 25} print(person\[\"city\"\]) \# Raises KeyError: \'city\' **Solution:** - Use the get() method to provide a default value: - print(person.get(\"city\", \"Unknown\")) \# Output: Unknown - Check if the key exists using in: - if \"city\" in person: - print(person\[\"city\"\]) **6. Using a Mutable Object as a Set Element** Sets require their elements to be immutable. Adding mutable objects like lists to a set raises a TypeError. **Example:** my\_set = {1, 2, \[3, 4\]} \# Raises TypeError: unhashable type: \'list\' **Solution:** Use immutable types like tuples: my\_set = {1, 2, (3, 4)} \# Works fine **7. Division by Zero** Attempting to divide a number by zero raises a ZeroDivisionError. **Example:** x = 10 / 0 \# Raises ZeroDivisionError: division by zero **Solution:** Check for zero before performing division: if y != 0: result = x / y else: print(\"Division by zero is not allowed.\") **8. Forgetting Type Conversion with Input** User input via input() is always a string, which can lead to unexpected behavior if you forget to convert it. **Example:** age = input(\"Enter your age: \") \# User enters \"25\" if age \> 18: \# Raises TypeError: \'\>\' not supported between instances of \'str\' and \'int\' print(\"You are an adult.\") **Solution:** Convert input to the correct type: age = int(input(\"Enter your age: \")) if age \> 18: print(\"You are an adult.\") **9. Modifying a Dictionary During Iteration** Modifying a dictionary while iterating over it raises a RuntimeError. **Example:** my\_dict = {\"a\": 1, \"b\": 2, \"c\": 3} for key in my\_dict: del my\_dict\[key\] \# Raises RuntimeError: dictionary changed size during iteration **Solution:** Use a copy of the keys for iteration: for key in list(my\_dict.keys()): del my\_dict\[key\] **10. Floating-Point Precision Issues** Floating-point arithmetic can lead to precision errors due to how numbers are stored in memory. **Example:** x = 0.1 + 0.2 print(x == 0.3) \# Output: False **Solution:** Use the decimal module for precise arithmetic: from decimal import Decimal x = Decimal(\"0.1\") + Decimal(\"0.2\") print(x == Decimal(\"0.3\")) \# Output: True **11. Forgetting to Handle NoneType** Operations on a NoneType variable can raise an AttributeError or TypeError. **Example:** x = None print(x.upper()) \# Raises AttributeError: \'NoneType\' object has no attribute \'upper\' **Solution:** Check for None before performing operations: if x is not None: print(x.upper()) else: print(\"x is None.\") **12. Using is Instead of == for Value Comparison** The is operator checks identity, not equality. This can cause unexpected results when comparing values. **Example:** x = 1000 y = 1000 print(x is y) \# Output: False (because they are different objects) **Solution:** Use == for value comparison: print(x == y) \# Output: True **Conclusion** Understanding these common errors and their solutions will help you avoid pitfalls when working with Python data types. Always test your code and use debugging tools to identify and resolve type-related issues.