Programming and Flutter Assessment (30 Questions) PDF
Document Details
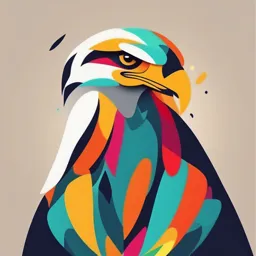
Uploaded by PurposefulConnemara96
Tags
Summary
This document contains 30 questions on Dart programming concepts and Flutter development. The questions cover topics such as syntax, data structures, functions, and error handling, with a specific emphasis on Flutter widgets and state management. It serves as a practice exam or assessment for students learning Dart and Flutter.
Full Transcript
Advanced Programming and Flutter Assessment Questions ===================================================== Q1. 1. What is the final output of the following code? - a\) 15 - b\) 510 - c\) Runtime Error - d\) Compilation Error Answer: c) Runtime Error Q2. 2. What is the difference betw...
Advanced Programming and Flutter Assessment Questions ===================================================== Q1. 1. What is the final output of the following code? - a\) 15 - b\) 510 - c\) Runtime Error - d\) Compilation Error Answer: c) Runtime Error Q2. 2. What is the difference between \`final\` and \`const\` in Dart? - a\) \`final\` is used for compile-time constants only - b\) \`const\` refers to runtime constants only - c\) \`const\` is for compile-time constants, and \`final\` values can change - d\) \`final\` is used for runtime constants, while \`const\` is for compile-time constants Answer: d) \`final\` is used for runtime constants, while \`const\` is for compile-time constants Q3. 3. What happens when the following code is executed? - a\) \[10, 2, 3\] - b\) \[1, 2, 3\] - c\) Compile-time Error - d\) Runtime Error Answer: d) Runtime Error Q4. 4. What is the output of the following code? - a\) 0 1 2 3 4 - b\) 0 1 2 - c\) 0 1 2 3 - d\) Nothing Answer: b) 0 1 2 Q5. 5. What does the following code do? - a\) 1 2 3 4 5 - b\) 1 2 4 5 - c\) 1 2 3 - d\) 1 3 4 5 Answer: b) 1 2 4 5 Q6. 6. What happens when the following code is executed? - a\) Prints \'Start\' and \'End\' - b\) Prints only \'Start\' - c\) Prints only \'End\' - d\) Prints nothing Answer: b) Prints only \'Start\' Q7. 7. Which of the following is the correct way to define a function with optional parameters? - a\) \`void greet(String name, \[int age\])\` - b\) \`void greet(String name, {int age})\` - c\) \`void greet(String name, {required int age})\` - d\) \`void greet(String name, optional int age)\` Answer: b) \`void greet(String name, {int age})\` Q8. 8. What does the following code do? - a\) 15 - b\) Compile-time Error - c\) Runtime Error - d\) Nothing Answer: a) 15 Q9. 9. What is the difference between a \`factory\` and a regular constructor? - a\) \`factory\` cannot be used to create a new object - b\) \`factory\` is used to dynamically create objects - c\) \`factory\` always returns a new object - d\) \`factory\` is used to create objects without a constructor Answer: b) \`factory\` is used to dynamically create objects Q10. 10. What is the output of the following code? - a\) Hello from Parent - b\) Hello from Child - c\) Compile-time Error - d\) Runtime Error Answer: b) Hello from Child Q11. 11. What is the output of the following code? - a\) Fetching\... Done Data Fetched - b\) Fetching\... Data Fetched Done - c\) Done Fetching\... Data Fetched - d\) Data Fetched Fetching\... Done Answer: a) Fetching\... Done Data Fetched Q12. 12. Which of the following is true about \`Stream\`? - a\) It can handle only one value - b\) It can handle multiple sequential values - c\) It must always be used with \`await\` - d\) It stops automatically when finished Answer: b) It can handle multiple sequential values Q13. 13. What is the difference between \`on\` and \`catch\` in error handling? - a\) \`on\` specifies the type of error, while \`catch\` handles any error - b\) \`catch\` specifies the type of error, while \`on\` handles general errors - c\) There is no difference between them - d\) They cannot be used together Answer: a) \`on\` specifies the type of error, while \`catch\` handles any error Q14. 14. What happens when the following code is executed? - a\) Cannot divide by zero - b\) Error: IntegerDivisionByZeroException - c\) Compile-time Error - d\) Nothing Answer: a) Cannot divide by zero Q15. 15. How do you handle nullable types in Dart? - a\) By adding \`?\` after the type - b\) By using \`late\` - c\) By adding \`\@nullable\` annotation - d\) By declaring the variable as \`dynamic\` Answer: a) By adding \`?\` after the type Q16. 16. What happens when you use \`late\` on a variable but never initialize it? - a\) It throws a compile-time error - b\) It remains uninitialized - c\) It throws a runtime error when accessed - d\) It gets initialized with a default value Answer: c) It throws a runtime error when accessed Q17. 17. How can you create an immutable list in Dart? - a\) Using \`const\` - b\) Using \`final\` - c\) Using \`immutable\` - d\) Using \`readonly\` Answer: a) Using \`const\` Q18. 18. What is the output of the following code? - a\) 3 - b\) 3.333\... - c\) 4 - d\) Compile-time Error Answer: a) 3 Q19. 19. Which Dart collection allows storing unique elements? - a\) List - b\) Map - c\) Set - d\) Queue Answer: c) Set Q20. 20. What is the purpose of \`typedef\` in Dart? - a\) To define custom data types - b\) To create type aliases for function signatures - c\) To create abstract classes - d\) To enable generics Answer: b) To create type aliases for function signatures Q21. 21. What is the purpose of the \`build\` method in a Flutter widget? - a\) To initialize state - b\) To build the widget tree - c\) To handle user input - d\) To manage navigation Answer: b) To build the widget tree Q22. 22. Which widget is used to create a scrollable list in Flutter? - a\) \`Column\` - b\) \`ListView\` - c\) \`Stack\` - d\) \`Row\` Answer: b) \`ListView\` Q23. 23. What is the difference between \`StatelessWidget\` and \`StatefulWidget\`? - a\) \`StatelessWidget\` manages state, \`StatefulWidget\` does not - b\) \`StatefulWidget\` manages state, \`StatelessWidget\` does not - c\) Both manage state - d\) None of the above Answer: b) \`StatefulWidget\` manages state, \`StatelessWidget\` does not Q24. 24. How can you pass data between screens in Flutter? - a\) Using a constructor - b\) Using a global variable - c\) Using Navigator arguments - d\) All of the above Answer: d) All of the above Q25. 25. What does the \`setState\` method do in Flutter? - a\) Rebuilds the widget tree - b\) Updates the state variable directly - c\) Navigates to a new screen - d\) None of the above Answer: a) Rebuilds the widget tree Q26. 26. Which of the following widgets is used to handle user gestures in Flutter? - a\) \`GestureDetector\` - b\) \`Container\` - c\) \`Scaffold\` - d\) \`Row\` Answer: a) \`GestureDetector\` Q27. 27. How do you make an API request in Flutter? - a\) Using the \`http\` package - b\) Using the \`Navigator\` package - c\) Using the \`async\` package - d\) Using the \`StreamBuilder\` widget Answer: a) Using the \`http\` package Q28. 28. What is the role of the \`Scaffold\` widget in Flutter? - a\) It provides a structure for the app\'s layout - b\) It handles navigation between screens - c\) It manages user inputs - d\) It defines app-wide themes Answer: a) It provides a structure for the app\'s layout Q29. 29. How do you add padding around a widget in Flutter? - a\) Using the \`Padding\` widget - b\) Using the \`Container\` widget - c\) Using the \`Row\` widget - d\) Using the \`ListView\` widget Answer: a) Using the \`Padding\` widget Q30. 30. What is the purpose of the \`FutureBuilder\` widget in Flutter? - a\) To display loading indicators - b\) To handle asynchronous data and update the UI accordingly - c\) To handle state management - d\) To manage navigation Answer: b) To handle asynchronous data and update the UI accordingly