PolyLSOsem3.pdf
Document Details
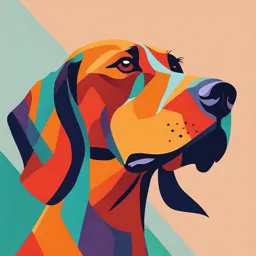
Uploaded by AgileIndianArt
Université Paris-Dauphine
Full Transcript
# 3. Boucle répétitive while (cours) ## 3.1 Definition - A repetitive loop is an instruction allowing to repeat a set of instructions. - The repetitive loop is introduced by the keyword while and allows to repeat a block of instructions as long as a condition is met. - It translates into Python a...
# 3. Boucle répétitive while (cours) ## 3.1 Definition - A repetitive loop is an instruction allowing to repeat a set of instructions. - The repetitive loop is introduced by the keyword while and allows to repeat a block of instructions as long as a condition is met. - It translates into Python as follows: ```python while condition: BlocInstructions ``` - The condition is a logical expression. - By entering the while loop, the condition is evaluated. - If the evaluation of the condition returns False, the instructions of the block associated with the loop are not executed, and we move on to the instruction following the loop (next instruction located at the same indentation level as the while). - If the evaluation of the condition returns True, the instructions of the block associated with the loop are executed once, then the condition is evaluated again and the instructions of the loop are executed again as long as the evaluation of the condition returns True. - The execution of the instructions in the loop stops when the evaluation of the instructions in the loop returns False. - The execution of all the instructions in the block constitutes an iteration of the loop. - As a reminder, the beginning of the instruction block associated with the loop is specified by the ':' character placed after the condition of the while. - The instructions of the block must be indented relative to the while and the end of the instructions block of the loop is specified by the first instruction located at the same indentation level as the while. ## 3.2 Examples ### Example 1 Python program displaying the 3 iterations of a loop. ```python n = 1 while n <= 3: print('Iteration', n) n = n + 1 ``` Simulation in a table: | n | n <= 3 | Writing | |---|---|---| | 1 | True | Iteration 1 | | 2 | True | Iteration 2 | | 3 | True | Iteration 3 | | 4 | False | | - At the beginning, the condition n <= 3 is verified, therefore we enter the loop, we display the value of the iteration and we increment the value of n, then we repeat these instructions as long as the condition n <= 3 is verified. - The program will therefore display the iterations from 1 to 3 (n has the value 4 at the end of the program). - A counter can be increased at each iteration by a value of any amount. - A counter can also be decreased at each iteration. See the example below: ### Example 2 What does this program do? ```python n = 3 while n > 0: print(n) n = n- 1 print('Partez !') ``` - Let's run the program step by step: | n | n> 0 | Writing | |---|---|---| | 3 | True | 3 | | 2 | True | 2 | | 1 | True | 1 | | 0 | False | Partez | - The program therefore displays the countdown from 3. - **Attention:** if we forget the instruction modifying n, the evaluation of the condition will always return True and the loop will repeat endlessly. We will also have an endless loop if we indent the instruction that modifies n incorrectly. ```python n = 3 while n > 0: print(n) n = n - 1 print('Partez !') ``` - To interrupt a program that runs infinitely in Pyzo, you need to go to the Menu Shell and select Interrupt, or press CRTL-I or press the second button on the toolbar. **Exercise:** Rewrite the previous program but using a counter that increments. ## 3.3 Nested while Loops When, in the block of instructions of a while loop, we find another while loop: we then speak of nested while loops. For example, the execution of the two nested loops below: ```python i= 0 while i < 3: j = 0 while j < 4: print(i*j, end=' ') j = j + 1 i = i + 1 print() ``` - results in the following display on the screen: ``` 0000 0 1 2 3 0 2 4 6 ``` What happens in the variation below? ```python i = 0 j = 0 while i < 3: while j < 4: print(i*j , end=' ') j = j + 1 i = i + 1 print() ``` ## 3.4 Use of while Loops ### 3.4.1 To count The while loop allows to repeat a specific calculation a certain number of times. For example, we want to write a program to calculate: $$ \sum_{i=1}^5 i^3 $$ - To do this, we will write a while loop using: - a variable i whose starting value is 1 (since we will vary i from 1 to 5), - a variable called resultat which will contain the result and which at the beginning of the program will be initialized to 0, - a while loop that will iterate over i: at each iteration, we add to the variable resultat the term i**3, then we increment i by 1. - We therefore obtain the program below: ```python resultat = 0 i = 1 while i <= 5: resultat = resultat + i**3 i = i + 1 print("Somme pour i allant de 1 à 5 =", resultat) ``` - Which, after execution, produces the following display: ``` Somme pour i allant de 1 à 5 = 225 ``` - Note that at the end, the value referenced by the variable i is 6. - If we want to display the value of the variable resultat at each iteration, we will move the writing instruction into the block of instructions of the loop (before the increment of i!): ```python resultat = 0 i = 1 while i <= 5: resultat = resultat + i**3 print("Somme pour i allant de 1 à", i, '=', resultat) i = i + 1 ``` - After executing this block of instructions, we obtain the following display: ``` Somme pour i allant de 1 à 1 = 1 Somme pour i allant de 1 à 2 = 9 Somme pour i allant de 1 à 3 = 36 Somme pour i allant de 1 à 4 = 100 Somme pour i allant de 1 à 5 = 225 ``` - There are many variations: - initialize resultat to 1 and i to 2 before the while loop, - initialize resultat to 1 and i to 1 but start by incrementing i; in this case, be careful about the exit test of the loop: ```python resultat = 1 i = 1 while i < 5: i = i + 1 resultat = resultat + i**3 print("Somme pour i allant de 1 à 5 =", resultat) ``` - In this case, the final value of i is 5. **Exercise:** The factorial of an integer n is denoted n! and is defined as follows: $$ n! = n * (n - 1) * (n - 2) * ... * 3 * 2 * 1 $$ - We also know that 0! = 1. - Write a program that allows you to calculate x!, x being a value entered by the user. ### 3.4.2 To control the validity of keyboard data For example, we want to read a positive integer and make sure that the user enters a positive value, therefore: ```python print('Saisir une valeur strictement positive : ', end='') val = input() val = int(val) while val <= 0: print('Saisir une valeur STRICTEMENT POSITIVE : ') val = input() val = int(val) ``` **Remarks:** - For the previous loop, it is not possible to say a priori what the number of iterations will be: it could be zero, - In this other version: ```python val = -1 while val <= 0: print('Saisir une valeur STRICTEMENT POSITIVE : ') val = input() val = int(val) ``` - The initialization of the variable val to -1 (we could have put any other negative value) ensures that we will perform at least one iteration of the while loop. **Exercise:** Write a program that allows you to read a grade entered by the user, ensuring that the value read is between 0 and 20. ### 3.4. 3 To search for values **Problem 1:** A farmer raises sheep and turkeys and, at the time of paying his taxes, curiously declares: I have 36 heads and 100 legs in my farm! Write a program in Python that allows you to determine the number of sheep and turkeys. - We can determine these two numbers by performing a simulation, with M the number of sheep, D the number of turkeys, and P the number of legs: - if M = 36 then D = 0 therefore P = 36x4 = 144 => False, - if M = 35 then D = 1 therefore P = 35x4 + 1x2 = 142 => False, - if M = 34 then D = 2 therefore P = 34x4 + 2x2 = 140 => False - .....until P is equal to 100. **Solution:** We must define three variables: M, D and P. At initialization, M references the value 36, D the value 0 and P the value resulting from the evaluation of the expression 4*M + 2*D. If P is equal to 100 then we can stop, otherwise we must explore other possibilities by decreasing the value of M by one unit and by increasing the value of D by one unit (to remain at 36 heads!) until P is different from 100. We then obtain the following program: ```python M = 36 D = 0 P = 4M + 2*D while P != 100: M = M-1 D = D+1 P = 4M + 2*D print('Nombre de moutons :', M) print('Nombre de dindons :', D) ``` - After executing this program, we obtain the following display: - Number of sheep: 14 - Number of turkeys: 22 **Problem 2:** Determine the smallest integer n such that 2^n is greater than or equal to 20. **Solution:** To solve this problem in an algorithmic way: - we use a variable cpt with an initial value of 1; - we use a variable res with an initial value of 2 because it stores 2**cpt - we increase the value of cpt as long as res is not greater than or equal to 20. - We obtain the following Python program: ```python n = 1 res = 2 while res < 20: n = n + 1 res = res*2 print("La plus petite valeur de n pour laquelle 2 puissance n " "est supérieur ou égal à 20 est ", n, "on a 2^",n,"=",res) ``` - Simulation: | Iteration | cpt | res | res < 20 | |---|---|---|---| | Initialization | 1 | 2 | True | | 1 | 2 | 4 | True | | 2 | 3 | 8 | True | | 3 | 4 | 16 | True | | 4 | 5 | 32 | False | - Writing of 5. - It is classic to have a variable that counts the number of iterations and another that stores the intermediate result. - The condition of the while loop can therefore be based on one or the other of these values. ### 3.4.4 Use a boolean variable in a while loop Let's say we're programming a game where we set a value between 0 and 30 and the user must find this unknown value. - To do this, we will use a while loop without knowing the number of iterations required: the user will be able to play again as long as she hasn't found it and wants to continue. - It will take a boolean variable to represent the fact that she hasn't found it and wants to play. - As long as this variable is True, she can make a suggestion. - We will therefore need to initialize this variable so that we can enter the loop, each iteration of which will allow the player to make a suggestion. ```python deviner = 15 jouer = True while jouer == True: proposition = int(input("Quelle valeur proposez-vous ?")) if proposition == deviner: print("Bravo") jouer = False else: rejouer = input("Voulez-vous rejouer?Oui/Non") if rejouer == "Non": jouer = False ``` - This type of algorithmic construction is extremely classic. Moreover, the line ```python while jouer == True: ``` - can simply be replaced with: ```python while jouer: ``` - Indeed, the variable jouer being a boolean variable, when evaluated, it returns either True or False and this is exactly what the while loop expects to know whether another iteration should be started. ==End of OCR for page 8==