PL-L1.pdf
Document Details
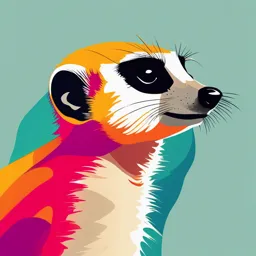
Uploaded by TopnotchImagery
Curtin University
2022
Tags
Full Transcript
Lecture 1: Introduction Created by Arlen Brower. Updated 2022-07-29. Unit Learning Outcomes Evaluate and analyze the development of programming languages and paradigms Assess the issues that need to be considered when choosing a programming language Evaluate program...
Lecture 1: Introduction Created by Arlen Brower. Updated 2022-07-29. Unit Learning Outcomes Evaluate and analyze the development of programming languages and paradigms Assess the issues that need to be considered when choosing a programming language Evaluate programming principals in the context of programming language design Analyse and apply compiler design tools by using Lex and Yacc to build a primitive compiler Teaching Pattern 2-hour lecture 1-hour tutorial ○ Expect to work longer on the tutorials! Assessments: In-class test, Assignment, Final assessment How I Lecture These notes are a broad structure … they’re really more for me. Future lectures will be much more demonstrations In other words: watch the lecture, don’t just read notes! But what is this unit? Why do we need to learn different programming languages? What about compilers? Different paradigms? Paradigms Imperative ○ Explicit, algorithmic steps ○ How the program will accomplish the task Declarative ○ Specify the desired result ○ What rather than the how There are more… Imperative Paradigms You’re probably familiar! Procedural ○ Fortran, ALGOL, C, etc. Object-Oriented ○ Java, C#, Kotlin, etc. Imperative Paradigms Manipulating state is a big characteristic here Variables Assignment x = 0; x = x + 1; Declarative Paradigms We will be focusing on: ○ Logic ○ Functional (will be in the test!) SQL may be your first declarative-style language Logic Programming Primarily Prolog Facts Rules food(pizza). food(cheese). food(X) :- tasty(X). Functional Programming Mimics mathematical functions Avoid variables, side-effects Repetition through recursion fib 0 = 1 fib 1 = 1 fib (n + 2) = fib (n + 1) + fib n Paradigms Paradigms are not hard rules Many languages are multi-paradigm ○ Some OO languages can be used in a procedural way ○ JavaScript can do anything! Kind of. Paradigms Many others do exist, though we don’t look at them in this unit. Back to Learning Outcomes Evaluate programming principals in the context of programming language design Perhaps more than anything else, this unit should give you the means to discuss the age old question: ○ What is the best programming language? And, hopefully, you will learn that there’s no good answer. Programming Principles Aspects of a programming language are not an exact science ○ Frameworks to discuss them are not necessarily perfect ○ But we’ll use one to start as a solid frame of reference This is a good point to remind you about the book this unit uses! Properties We will consider three overarching properties: ○ Readability – Can you understand the program? ○ Writability – Can you solve the problem? ○ Reliability – Does your program work under all conditions? Characteristics These can be further discussed in terms of characteristics: Characteristics Simplicity Orthogonality Data types Syntax design Support for abstraction Expressivity Type checking Exception Handling Restricted Aliasing Orthogonality I’ve taught this unit enough to know that you don’t like the look of ‘Orthogonality’. You can think of this as well-defined the building blocks of a language are You want there to be few exceptions to your rules! Constructs should behave the same, regardless of context a + b Examples Using the Properties and Characteristics, you can probably already discuss a programming language a little better than you could at the start of this lecture. If nothing else, use it to voice you disdain for your least-favourite language. … or the celebrate the weirdness of your favourite. Properties & Characteristics It will be important for this unit that you learn to discuss languages in these terms It will be especially important that you articulate why you might choose one language over another We will be getting hands-on experience with many languages ○ Note down what you like, what you don’t like Summary Three properties Nine characteristics Many different paradigms Huge amount of languages Reading Chapter 1: ○ 1.1 – Reasons for Studying ○ 1.2 – Programming Domains ○ 1.3 – Language Evaluation Criteria ○ 1.6 – Language Design Trade-Offs