Python PCAP Mock Practice Questions PDF
Document Details
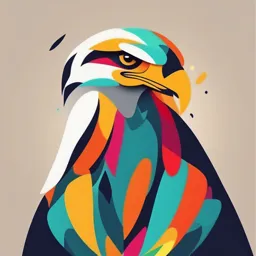
Uploaded by AuthoritativeBanjo2312
Tags
Summary
This document contains a series of practice questions designed to prepare individuals for the PCAP (Certified Associate in Python Programming) certification exam. The questions cover a range of Python programming topics and concepts, and is suitable for those preparing for a professional certification.
Full Transcript
Okay, here is the conversion of the images into a structured markdown format. ### Question 1/40 Q: The following code snippet is used to import and invoke the `showinfo()` function. Which statement is always true about the function and its environment? (Select two answers.) * The `messagebox()`...
Okay, here is the conversion of the images into a structured markdown format. ### Question 1/40 Q: The following code snippet is used to import and invoke the `showinfo()` function. Which statement is always true about the function and its environment? (Select two answers.) * The `messagebox()` function can be also invoked using the following syntax: `tkinter.messagebox()` * The `showinfo()` function is contained in `the messagebox` module. * `messagebox` is a module or a package. * The `messagebox()` function can be also invoked using the following syntax: `messagebox()` **Code Snippet:** ```python import tkinter.messagebox messagebox.showinfo("Hello") ``` ### Question 2/40 Q: Which of the following statements are false? (Select two answers.) * The extension `.pyc` marks files containing Python semi-compiled byte-code. * A programmer is obliged to manually create a directory/folder named `__pycache__` inside every package. * A source file named `__init__.py` is used to mark a directory/folder as containing a Python package and to initialize the package's state. * A variable named `__name__` is a string containing the module name. ### Question 3/40 Q: Which of the following statements are true? (Select two answers.) * Variables with names starting with two underscores are considered private inside their home module. * The directory from which the code is run is never searched through. * The directory from which the Python code is run is always searched through in order to find the necessary modules. * Variables with names ending with two underscores are considered private inside their home module. ### Question 4/40 Q: What is the expected output of the following code? ```python import math x = 1.7 print(-abs(math.floor(x) + math.ceil(x))) ``` * -2 * 3 * -3 * 2 ### Question 5/40 Q: Which function will you use to reveal a module's contents? * `dir()` * `_dir()` * `_dir_()` * `dir ()` ### Question 6/40 Q: You need to find out if a hardware platform utilizes the x86 or arm CPU. Which function can you use? (Select two answers.) * `node()` * `processor()` * `hardware()` * `platform()` ### Question 7/40 Q: Which of the following operations will not raise any exception? (Select two answers.) * Invoking the `float()` function. * Decrementing an integer variable by one. * Indexing a tuple. * Slicing a string. ### Question 8/40 Q: Which of the following messages will appear on the screen when the code is run? (Select two answers.) ```python class Failure (Exception): def _init_(self, message): self.message = message def _str (self): return "out of order" try: print("turn on") raise Failure ("crash") except Failure as problem: print (problem) else: print("success") ``` * success * out of order * turn on * crash ### Question 9/40 Q: What is the expected output of the following code? ```python data = [261, 321] try: print (data[-3]) except Exception as exception: print (exception.args) else: print("('success',)") ``` * `('list index out of range',)` * `('success',)` * 321 * 261 ### Question 10/40 Q: What is the expected output of the following code? ```python def attic(x): assert x != 0 return 1 / x def floor(x): try: attic(x) except: raise try: x = attic (0) except RuntimeError: x = -3 except: x = -2 else: x = -1 print(x) ``` * -3 * An error message appears on the screen. * -2 * -1 ### Question 11/40 Q: What is the expected output of the following code? ```python s = 'three' e = 0 try: e = int(s) except ArithmeticError: e = 1 except: e = 2 else: e = 3 print(e) ``` * 2 * 3 * 1 * 0 ### Question 12/40 Q: Which of the following statements are true? (Select two answers.) * `\n` can be used to encode a new-line character. * ASCII is a subset of UNICODE. * In ASCII, Latin upper-case letter codepoints are greater than their lower-case counterparts. * Python accepts UTF-8 encoded source files. ### Question 13/40 Q: Which of the following snippets output 123 to the screen? (Select two answers.) * `print(sorted("321"))` * \`\`\`tmp = "321".sort() print(str(tmp))\`\`\` * `print(''.join(sorted("321")))` * \`\`\`tmp = list("321") tmp.sort() print(''.join(tmp))\`\`\` ### Question 14/40 Q: What is the expected output of the following code? ```python strng = "John, Doe, 42" strng = "".join(strng.split(",")) print(strng[-2]) ``` * 4 * o * e * 2 ### Question 15/40 Q: What is the expected output of the following code? ```python header = "+-" * 2 + '+' plus = 0 for x in header: if not x in header: plus += 1 print (plus) ``` * 3 * 2 * 0 * 5 ### Question 16/40 Q: Which of the following expressions evaluates to `True` and raises no exception? * `'9' * 1 < 1` * `'9' * 3 > '9' * 9` * `'Al' * 2 ! = 2 * 'Al'` * `10 != '1' + '0'` ### Question 17/40 Q: Which of the following assignments will successfully produce a non-empty string? (Select two answers.) * \`\`\`S = "12"\ \`\`\` `S = s[-3:-5]` * \`\`\`S = "12"\ \`\`\` `S = s[1::2]` * \`\`\`S = 'rhyme'\ \`\`\` `S = s[9]` * \`\`\`S = "12"\ \`\`\` `S = s[-1]` ### Question 18/40 Q: Which of the following are not valid Python string literals? (Select two answers.) * `"\\"` * `'Whether 'tis nobler in the mind to suffer'` * `'''To be, or not to be, that is the question"""` * `"this is a quote: \""` ### Question 19/40 Q: Which of the following expressions evaluate to `True` and raise no exception? (Select two answers.) * `'?' in ""` * `'T' in 'de'` * `'de' not in 'abc'` * `not 'xyz' in 'uvwxyz'` ### Question 20/40 Q: What is, the expected behavior of the following code? ```python class Content: title = "None" def __init__(self, this): self.name = this + " than " + Content.title text1 = Content ("Paper") text2 = Content ("Article") print (text 1.title == text 2.name) ``` * It outputs `False` * It outputs `None` * The code is erroneous and will raise an exception. * It outputs `True` ### Question 21/40 Q: What is the expected output of the following code? ```python class Cat: Species = 1 def get_species (self): return 'kitty' class Tiger (Cat): def get_species (self): return 'tiggy' def set_species (self): pass creature = Tiger() print (hasattr (creature, "Species"), hasattr(Cat, "set_species")) ``` * True True * False True * True False * False False ### Question 22/40 Q: What is the expected output of the following code? ```python class Example: def __str__(self): return name thing = Example() print (thing) ``` * thing * Example * `__main__` * None ### Question 23/40 Q: Given the class below, indicate the method which will correctly provide the value of the rack variable. ```python class Storage: def __init__(self): self.rack = 1 # Insert the method here. stuff = Storage () print(stuff.get()) ``` \* def get(): return rack * def get(): return self.rack * def get (self): return self.rack * def get (self): return rack ### Question 24/40 Q: Given the code below, indicate the code lines correctly decrementing the `b` variable by one. (Select two answers.) ```python class A: b = 1 def init (self): self.c = 1 def action (self): pass object = A () ``` * `A.b -=1` * `object.b -= 1` * `object.A.b-= 1` * `A.A.b -= 1` ### Question 25/40 Q: Given the code below, which of the following expressions will evaluate to `True`? (Select two answers.) ```python class Un: value = "Ein" def say (self): return self.value.upper() class Deux (Un): value = "Zwei" class Troi (Un): def say (self): return self.value.lower() class Quatre (Troi, Deux): pass d = Quatre () b = Deux () ``` * `isinstance (d, Un)` * `d.say() == "ZWEI"` * `Troi in Quatre. __bases__` * `d.value == "uno"` ### Question 26/40 Q: Assuming that the following code has been successfully executed, indicate the expressions which evaluate to True and don't raise any exception. (Select two answers.) ```python class Volume: chapter = 1 def _init_(self, paragraph): self.paragraph = paragraph Volume.chapter += 1 def remove(self): del self.paragraph edition = Volume (1) edition.remove() ``` * `Volume. dict ['chapter'] != None` * `'paragraph' in Volume. dict` * `len (edition. __dict ) != len (Volume. __dict )` * `'paragraph' in edition. _ dict` ### Question 27/40 Q: What is the expected behavior of the following snippet? ```python class Taxi: def print_number(self): print(self.get_number()) def get number(self): return "unknown" class Yellow (Taxi): def get_number(self): return "261" cab = Yellow () cab.print_number() ``` * It outputs `261` * It outputs `unknown` * It raises an exception. * It outputs `None` ### Question 28/40 Q: Which statements are true about object-oriented programming? (Select two answers.) * A class may exist without its objects, but an object cannot exist without its class. * Polymorphism is a phenomenon allowing you to have many classes of the same name. * In the hierarchy diagram, a superclass of a class is located above the class. * Encapsulation is a phenomenon which allows you to hide some class traits from the outer world. ### Question 29/40 Q: Given the class hierarchy presented below, indicate which of the following class declarations are permissible and will not raise an exception? (Select two answers.) ```python class Diamond: pass class Adamant (Diamond): pass class Gem (Diamond): pass ``` * \`\`\`class Jewel (Diamond, Adamant): pass\`\`\` * \`\`\`class Jewel (Diamond, Diamond): pass\`\`\` * \`\`\`class Jewel (Adamant, Gem): pass\`\`\` * \`\`\`class Jewel (Adamant, Diamond): pass\`\`\` ### Question 30/40 Q: Which of the following classes contain a usable constructor? (Select two answers.) * \`\`\`class Lower: def init (self): self.color = "blue"\`\`\` * \`\`\`class Working: def init (self): raise KeyError\`\`\` * \`\`\`class Middle: def __init(): self.color = None\`\`\` * \`\`\` class Upper: def __init__(self): return False \`\`\` ### Question 31/40 Q: Given the code below, which of the expressions will evaluate to True? (Select two answers.) ```python class Control: my_ID = 1 def say (self): return self.my_ID class Button (Control): my_ID = 2 class Radio (Button): def say (self): return -self.my ID selection = Radio () element = Control() start = Button() ``` * `isinstance (start, Button)` * `selection.my ID == 2` * `start.my ID == -2` * `selection is element` ### Question 32/40 Q: What is the expected output of the following code? ```python def power(a): def internal(x): return x ** a return internal cube = power (3) print (cube(2)) ``` * 8 * 4 * 2 * 1 ### Question 33/40 Q: Which of the following lines contain valid Python code? (Select two answers.) * `lambda a,b = a if a < b else b` * `lambda a,b: a if a < b else b` * `lambda (a,b): return a if a < b else b` * `lambda a,b: True` ### Question 34/40 Q: The `errno.ENOENT` symbol refers to an error described as: * Operation not permitted * Permission denied * No child processes * No such file or directory ### Question 35/40 Q: Which function will you use to read one line from a text file? * `readlines()` * `readStr()` * `readln ()` * `readline()` ### Question 36/40 Q: What is the expected output of the following code? ```python source = [1, 2, 4, 8, 16] target = [x // 2 for x in source if x < 10] print (target[1]) ``` \* 8 * 4 * 1 * 2 ### Question 37/40 Q: What is the expected output of the following code? ```python pairs = [[2, 1], [-2, -1]] new_pairs = map (lambda p: sorted(p), pairs) print(list(new_pairs)[0][0]) ``` * 2 * -2 * 1 * -1 ### Question 38/40 Q: What is the expected output of the following code? ```python def f(a,b): return b (b) print (f(lambda x: x + 1, 0)) ``` * 1 * An error message will appear on the screen. * None * 0 ### Question 39/40 Q: Which of the following statements are true? (Select two answers.) * The `input()` function reads data from the stdin stream. * There are three pre-opened file streams. * The first argument of the `open ()` function is an integer value. * The `readlines ()` function returns a string. ### Question 40/40 Q: What is the expected output of the following code? ```python def boolean(op): return op (False, True) print (boolean (lambda x,y: x if x else y)) ``` * 1 * True * 0 * False