Chapter 9 - Interfaces and Polymorphism PDF
Document Details
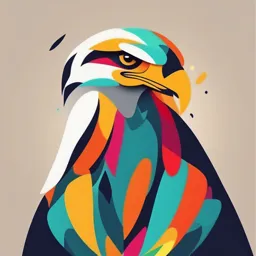
Uploaded by AdorableHeliotrope685
Fremont High School
2009
Cay Horstmann
Tags
Summary
This document is a chapter from a programming textbook, focusing on interfaces and polymorphism in Java. It explains the concept of interfaces and how they are used to decouple classes and provide polymorphic behavior. Topics include how to declare and use interface types, understand polymorphism, and implement helper classes as inner classes, as well as event listeners.
Full Transcript
Chapter 9 – Interfaces and Polymorphism Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Chapter Goals To be able to declare and use interface types To understand the concept of polymorphism To appreciate...
Chapter 9 – Interfaces and Polymorphism Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Chapter Goals To be able to declare and use interface types To understand the concept of polymorphism To appreciate how interfaces can be used to decouple classes To learn how to implement helper classes as inner classes G To implement event listeners in graphical applications Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Interface We’ve used the word "interface" to refer to the set of public methods (for example, getters and setters) through which we interact with an object. There's also a more formal use of the word interface in Java. A Java interface. This is a collection of constants and abstract method declarations. In other OO Programming Languages, a class can inherit more than one class (Chap 10). This is known as multiple inheritance. Java does not support this. Therefore they use a special type of coding element called interface. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Interface Very useful tool because it enables polymorphism and code sharing. Lessens coupling of higher and lower level classes As mentioned, Java uses a single inheritance. An interface is a work around which makes it possible to set up multiple inheritance relationships for classes to share characteristics. Also has several limitations Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. What is a Java Interface? An Interface is not a class, nor an object. It acts as a blueprint for a class but does not comprise of a class. An Interface contains a collection of constants and abstract methods, that if implemented by a class have to provide an implementation for all methods declared in the interface. An Interface specifies how one communicates with a method, but not how one implements the method. Abstract methods = no implementation, only the header and parameters, if any, followed by a “;” Interfaces are in a separate file and created: – BlueJ: new class interface – Eclipse: new interface Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. What is a Java Interface? public interface { public (type name, …, ); public ();... public (type name, …, ); } Note: The methods are not implemented (abstract) & they end w/ “;” Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. What is a Java Interface? public interface Complexity { void getComplexity(); int setComplexity(int complexity); int ACONSTNT = 12; } Both are correct public interface Complexity { public abstract void getComplexity(); public abstract int setComplexity(int complexity); public final int ACONSTNT = 12; // Note missing static, understood } Constants from interfaces can be used as if the class defined them. No need to redefined in the class. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. What is a Java Interface? Interfaces are like classes but cannot be instantiated. Their purpose is to specify sets of requirements for classes to implement. These requirements are sometimes regarded as contracts between the implementing class and any client that uses it. By stating in its header that it implements the interface, the class is guaranteeing that the required methods carry out the behavior specified by the interface. public class implements Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. What is a Java Interface? When a class implements an interface, think of the class as entering a contract to provide implementations for all the abstract methods in the interface. The compiler will check that this contract is adhered to. Otherwise, the class implementing the interface can contain anything else that a regular class contains. Again: do not try to instantiate an interface – this is meaningless! Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. What is a Java Interface? A class may implement any number of interfaces – Class Question implements A, B, C{ // where A,B,&C are intefaces … } A class definition can implement zero or more interfaces Since this class implements interfaces, the class body must provide a method definition for every method declared in the three interfaces. The class definition can use access modifiers as usual. Any number of classes can implement the same interface. The implementations of the methods listed in the interface can be different in each class. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Why Interfaces? Ok, you have a Java file that happens to be an interface that has no real working code in it. You're probably asking yourself, but should be asking me, what is the point? Why bother with the interface at all? Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Why Interfaces? Here is an example: public class Dog extends Animal{...} In Chap 10 you’ll learn that extends mean to inherit. This mean Dog is-a Animal Whatever an Animal can do, a Dog can do. public class FourLeggedObject extends Animal{...} This mean FourLeggedObject is-a Animal Whatever an Animal can do, a FourLeggedObject can do. What if want my Dog class to also extend FourLeggedObject. Can’t happen! Why not? Java cannot handle multiple inheritance, which is exactly what this situation would cause. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Visualizing Interfaces Depends/ Uses Flier Athlete Chap 8 void exercise(double hrs) String fly(); void train() Interface / IS-A Note the dotted lines Note the open arrows Bird Airplane SkiJumper int age() boolean flightPlan() int age() String color() double weightCap() double distance() Unified Modeling Language (UML) Diagram Consider the above UML Diagram: When a class implements an A Flier is one that flies. Interface, we say that it An Athlete is one that exercises. “realizes” the interface There are 3 classes Bird, Airplane, & SkiJumper. Each class can use and define fly() as it pleases. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. What they have in public interface Flier common { String fly(); //Notice no public or abstract identifier } public class Bird implements Flier { public String fly() { String brd = “wings”; return “Bird uses “ + brd + “ to fly”; } } public class Airplane implements Flier { public String fly() { String plane = “engines”; return “Airplane uses “ + plane + “ to fly” } } Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Java’s “solution” to multiple inheritance is allowing a class to implement more than one interface type. public interface Athlete { void exercise(double hours); } public class SkiJumper implements Flier, Athlete { public String fly() { String jumper = “skis”; return “SkiJumper uses “ + jumper + “ to get in the air”; } public void exercise(double time) { System.out.println(“I exercise “ + time + “ hours a day.” } } Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Using Interfaces You can use an interface when declaring local variables – Remember that a declaration is on the left side of the equals sign when initializing a variable Visualize it as just drawing a box & assigning it the value on the righthand side of the assignment operator – Syntax InterfaceName variableName = new ClassName(); – Example Flier robin = new Bird(30); //but…what can you access? Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Bird robin = new Bird(20); myWingVelocity = 30 Bird() myColor = Color.black mySpecies = “bird” Bird(int velocity) robin String toString() void fly() String speak() Can you do: YES robin.fly() robin.speak() YES robin.toString() YES Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Flier robin = new Bird(30); myWingVelocity = 30 Bird() myColor = Color.black mySpecies = “bird” Bird(int velocity) robin String toString() void fly() String speak() Can you do: robin.fly() YES robin.speak() NO robin.toString() YES Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Interfaces vs. Classes An interface type is similar to a class, but there are several important differences: All methods in an interface type are abstract; they don’t have an implementation All methods in an interface type are automatically public An interface type does not have instance fields Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. UML Diagram of DataSet and Related Classes Interfaces can reduce the coupling between classes UML notation: Interfaces are tagged with a “stereotype” indicator «interface» A dotted arrow with a triangular tip denotes the “is-a” relationship between a class and an interface A dotted line with an open v-shaped arrow tip denotes the “uses” relationship or dependency Note that DataSet is decoupled from Measureable DataSet BankAccount and Coin getMeasureable() BankAccount is-a Measureable Coin is-a Measureable DataSet uses/depends on Measureable BankAccount Coin Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Converting From Class to Interface Types You can convert from a class type to an interface type, provided the class implements the interface (i.e. using Measurable interface defined in the book) BankAccount account = new BankAccount(10000); Measurable x = account; //OK Coin dime = new Coin(0.1 “dime”); Measurable x = dime; //OK Measureable x = new Rectangle(5, 10, 20, 30); //Error Cannot convert between unrelated types. Rectangle does not implement Measureable Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Converting From interface to Class Type use Casts DataSet coinData = new DataSet(); coinData.add(new Coin(0.25, "quarter")); coinData.add(new Coin(0.1, "dime"));... Measurable max = coinData.getMaximum(); What can you do with it? It's not of type Coin String name = max.getName(); // ERROR Measureable does not have a getName method You need a cast to convert from an interface type to a class type Continued Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Casts (cont.) You know it's a coin, but the compiler doesn't. Apply a cast: Coin maxCoin = (Coin) max; String name = maxCoin.getName(); //now we can use methods in Coin Class If you are wrong and max isn't a coin, the compiler throws an exception Difference with casting numbers: When casting number types you agree to the information loss When casting object types you agree to that risk of causing an exception. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Converting between Class and Interface If you are converting from an Interface to Class you must use Cast className = interfaceName If you are converting from a Class to Interface No problem as long as it’s implemented! interfaceName = className STOP Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Interface Advantages : - Interfaces are mainly used to provide polymorphic behavior. - Interfaces function to break up the complex designs and clear the dependencies between objects. Disadvantages : - Java interfaces are slower and more limited than other ones. - Interface should be used multiple number of times else there is hardly any use of having them. -Need to chose interface methods very carefully at the time of designing your project because we can’t add of remove any methods from the interface at later point of time, it will lead compilation error for all the implementation classes. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Interfaces Reduces coupling (Dependencies). A class implements an interface by using the reserved word implements & providing the implementation for each abstract method defined in interface. A class is not limited to the abstract methods in the interface. You can add logic as usual. An interface can be implemented by many classes A class can implement multiple interfaces. Interfaces must all be defined as public Interfaces can act like class types If implemented, errors will occur if all methods are not implemented. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. POLYMORPHISM Big Java by Cay Horstmann Copyright © 2008 by John Wiley & Sons. All rights reserved. Polymorphism (Having many shapes and forms) Master this concept you master object-oriented programmimg Simply put, polymorphism is what allows actions to act differently based on the object performing the action or the action the object is performed on. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Polymorphism Example What sound does a cat make? Hopefully you’re thinking, meow Let’s call this action – makeSound() What sound does a dog make? Hopefully you’re thinking, woof Let’s call this action – makeSound() as well Oh, let’s just assume all animals can makeSound() This means, makeSound() does something different based on the object. Therefore should be in an interface not in the class Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Polymorphism Behavior can vary depending on the actual type of an object This is called dynamic/late binding: resolved at runtime Another type of binding is early binding overloaded methods: resolved at compile time System.out.print(12); System.out.print(“12”); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Polymorphism Flier Athlete void fly(); void train(); void takeOff(); boolean isJuiced(); void land(); Plane Bird SkiJumper CurlingPerson boolean flightplan(); int age(); double weight(); double maxDistance(); int maxPassengers(); String color(); double maxDistance(); int meetsWon(); String chirp(); double weightCap(); int yearsTrained(); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Some coding examples… Flier fred = new Plane(); //legal //but, what methods are available? Flier phil = new Flier(); //illegal //cannot construct an interface //”object” Bird bob = new Bird(); //legal Flier phil = bob; //legal //because all Birds are Fliers Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Some coding examples… Flier fred = new Plane(); //legal Plane temp = fred; //illegal //because not all Fliers are Planes The fix to this… Plane temp = (Plane)fred; //this a cast to a Plane object may be incorrect (“ClassCastException”) Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Some coding examples… Flier fred = new SkiJumper(); //legal Athlete temp = (Athlete) fred; // legal //all SkiJumpers implement Athlete Flier fred = new Plane(); //legal Athlete temp = (Athlete) fred; // illegal //Planes do not implement Athlete Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Some coding examples… Flier fred = new SkiJumper(); //legal SkiJumper temp = (SkiJumper) fred; // legal SkiJumper temp2 = (SkiJumper) temp; // legal //redundant, but legal… Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Polymorphism A major benefit of interfaces is that we can use them to achieve polymorphism. CONSIDER ArrayList airport = new ArrayList(); airport.add(new Bird()); airport.add(new AirPlane()); airport.add(new SkiJumper(20)); airport.add(new Bird()); for(int count=0; count 0 if the implicit is greater than other Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Comparable Interface Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Comparable Interface This can be carried out in two ways: pubic class Coin implements Comparable... public int compareTo (Object obj) { Coin temp = (Coin)obj; return this.value – temp.value; } Object is the "lowest common denominator" of all classes BUTTONS Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. Comparable Interface Another public class Coin implements Comparable... public int compareTo (Coin obj) { if (this.value < obj.value) return -2; if (this.value > temp.value) return 123; return 0; } Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved. HomeWork Google Form Read Unit 10 Big Java by Cay Horstmann Copyright © 2008 by John Wiley & Sons. All rights reserved.