Software Engineering Module 3 PDF
Document Details
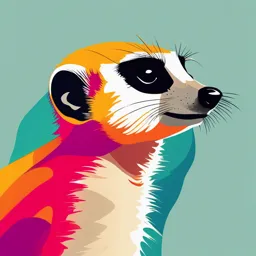
Uploaded by CheeryGrace8631
University of Kerala
Dr Lino A Tharakan
Tags
Summary
This document is a module on software engineering, covering various topics including software development methodology, procedural programming, and object-oriented programming. It outlines the key phases in software development and provides an overview of procedural and object-oriented approaches.
Full Transcript
MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan SOFTWARE ENGINEERING MODULE III Syllabus Why Object Orientation, Procedural Programming and Object Oriented Programming - Objec...
MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan SOFTWARE ENGINEERING MODULE III Syllabus Why Object Orientation, Procedural Programming and Object Oriented Programming - Object Oriented Systems development life Cycle. Object oriented Methodologies- Booch,Jacobson and Rumbaugh methodologies. SOFTWARE DEVELOPMENT METHODOLOGY A software development methodology is a structured approach or a series of processes used to develop software applications effectively. This methodology provides a framework that guides the planning, design, development, and delivery of software projects. Key phases in the development activity include: 1. System Analysis: This initial stage focuses on understanding and defining the requirements of the system. Analysts gather data on what the application should achieve, including end-user needs and system objectives. 2. Modeling: In this phase, the information collected during analysis is used to create models, like data flow diagrams or use-case diagrams, to visually represent the system and its functionalities. 3. Design: This step involves creating a blueprint for the software, defining the system architecture, components, interfaces, and data. 4. Implementation: Here, developers write code according to the design specifications. This is the actual building of the software. 5. Testing: Testing is essential to verify that the software functions as intended, is error-free, and meets the defined requirements. 6. Maintenance: After deployment, the software undergoes maintenance to address issues, add features, or make improvements based on user feedback. PROCEDURAL PROGRAMMING AND OBJECT ORIENTED PROGRAMMING – Procedural Programming Overview MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan Procedural programming is a traditional programming approach that structures code around procedures or routines. These routines perform specific tasks and are executed sequentially. Key characteristics include: 1. Focus on Functions and Procedures: Code is organized into functions, each carrying out specific operations. Functions are called as needed to perform tasks within the program. 2. Sequential Execution: Programs typically run in a top-down order, which makes it straightforward but can lead to complex dependencies as programs grow. 3. Global and Local Variables: Data is often stored in variables that can be accessed across functions (global) or restricted to a specific function (local), depending on their scope. 4. Data and Code Separation: Unlike Object-Oriented Programming (OOP), procedural programming keeps data separate from functions, which can make managing data flow more challenging as programs become larger. 5. Best for Simple Applications: Procedural programming works well for simple or smaller applications where tasks are straightforward and don’t require the complexity of OOP. OBJECT-ORIENTED SYSTEM DEVELOPMENT OVERVIEW The Object-Oriented (OO) approach organizes a system into objects that represent real- world entities, each containing both data (attributes) and functionality (methods). Key features include: 1. Data and Functionality Combined: Objects combine data with related functionality, making systems modular and intuitive. 2. Focus on Reusability: Emphasizes reusable classes and modules, reducing redundancy and simplifying updates. 3. Smooth Phase Transitions: Transitions between phases (e.g., design to implementation) are more streamlined, saving development time. 4. Lower Complexity: Encapsulation and abstraction reduce complexity, making the system easier to maintain and modify. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan OBJECT-ORIENTED APPROACH The Object-Oriented Approach (OOA) to systems development is a methodology focusing on creating systems as collections of interacting objects. This approach integrates analysis, design, and modeling steps in a way that views data and functions as a single entity, emphasizing reusability and modularity. Object-oriented systems development generally includes the following steps: 1. Object-Oriented Analysis (OOA): In this step, developers work to understand the problem by identifying the system’s requirements and the necessary objects that MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan will compose the system. Unlike traditional methods, OOA views data and procedures together within each object. 2. Object-Oriented Information Modeling: Here, developers create models to visually represent the relationships, properties, and functions of each object in the system. 3. Object-Oriented Design (OOD): During design, developers specify the details of the system's objects, including their attributes (properties) and methods (behaviors or operations). This process also involves creating hierarchical relationships, such as superclasses and subclasses, which organize and simplify object management. 4. Prototyping and Implementation: This phase involves creating working models of the system (prototypes) and coding the final software. The iterative nature of prototyping allows adjustments based on feedback. 5. Testing, Iteration, and Documentation: After implementation, the system is tested to ensure functionality. Documentation is also prepared, which describes the system’s design, operation, and maintenance. Core Principles of Object-Oriented Development Cooperative Objects: Objects within the system are seen as cooperative units that work together to achieve system functionality. Each object combines data (attributes) and the operations it can perform (methods). For example, an Employee object could manage payroll calculations, store information such as name and address, and print paychecks. Unified Data and Procedures: A major difference from structured methods is that objects do not separate data and functions; instead, they encapsulate both. This encapsulation helps keep each object independent and modular. Identifying Objects and Classes The first task in object-oriented analysis is identifying the classes and objects that will represent entities in the system. A good starting point is examining physical entities in the system to understand how they interact and contribute to system functionality. Common entities include: Persons: Individuals with roles in the system, like customers or employees. Places: Physical locations that hold relevant information, such as buildings or stores. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan Things/Events: Points in time or specific actions (like a customer placing an order). Attributes of these objects include details like who (customer ID), when (date), and what (item ordered). The analysis also defines hierarchical relationships. For example, an Employee might be a superclass with subclasses such as Manager, Production Worker, and Temporary Worker, each with its unique attributes and methods. Attributes and Methods Attributes: These are properties describing each object, such as cost, color, and manufacturer. For example, an Employee object may have attributes like name, address, and employee ID. Methods: These are the actions or behaviors an object can perform. For example, an Employee object might have methods like compute payroll, print paycheck, and update information. MODELING OBJECTS AND RELATIONSHIPS As in the structured approach, it’s essential to model objects and define relationships between them. For example, in a payroll system, objects like Employee, Manager, and Temporary Worker would each have methods to compute payroll, specific to their role. Example: Payroll System MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan In a payroll system, various employee classes (like Manager and Temporary Worker) exist as subclasses under a general Employee superclass. Each subclass would know how to calculate its payroll according to specific rules. The main program could loop through all employees, sending a message to each to calculate payroll without changing the main program's structure. Adding a new employee type would simply involve creating a new class without modifying existing code. SOFTWARE DEVELOPMENT PROCESS The Software Development Process is a systematic approach to creating or improving software products. It is structured as a series of transformations or phases, each producing outputs that serve as inputs to subsequent stages. This process emphasizes adaptability and modularity, meaning that each phase, or subprocess, can be modified independently as long as it maintains the required interfaces to interact effectively with other subprocesses. Key Characteristics of the Software Development Process 1. Independent Subprocesses: o Each subprocess is defined with clear input requirements, a detailed description, and specific outputs. This modularity allows flexibility in replacing subprocesses if needed while maintaining overall functionality. 2. Sequential Transformation Phases: o The development process consists of transformations that systematically refine the software, often divided into three major transformations: analysis, design, and implementation. Primary Transformations in Software Development 1. Transformation 1: Analysis o Analysis translates users' needs into system requirements. Understanding user requirements is crucial, often achieved by observing how they intend to use the system. For example, if the system requires a payroll feature, this becomes part of the requirements. 2. Transformation 2: Design o This phase uses the requirements to create a comprehensive design. It includes specifying how the software will be built, the necessary programs, MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan testing materials, and a structured testing plan. This phase ends with a fully detailed design that can be turned into a functioning system. 3. Transformation 3: Implementation o Implementation transforms the design into a deployed system, including hardware, software, procedures, and personnel. This phase embeds the system within its operational environment and considers resources and procedures to meet user needs. For example, implementing new payroll methods and producing new reports are part of this phase. BUILDING HIGH-QUALITY SOFTWARE Building high-quality software involves a focus on creating software that effectively meets user needs, maintains minimal defects, and achieves high user satisfaction. The emphasis is on proactively improving product quality during development, reducing the need for post-delivery corrections. Key Objectives for High-Quality Software 1. User Satisfaction: o The primary goal is to satisfy users by meeting their expectations, ensuring the software is bug-free and performs as required. 2. Quality Assessment Questions: o To assess software readiness, developers evaluate: ▪ Is the system ready for delivery? ▪ Does it operate as expected, meeting user needs? ▪ Does it pass necessary evaluation criteria? MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan Approaches to Software Testing Two main approaches are used for testing systems: 1. Functional Testing: Evaluates based on what the software is supposed to do. 2. Structural Testing: Evaluates based on how the software was built. These testing approaches align with Blum’s Four Quality Measures: 1. Correspondence: o Measures how well the software matches the original requirements and the operational environment, only fully determinable once the software is in use. 2. Correctness: o Checks if the product’s requirements match the design specification, ensuring that the product is precisely built to its specifications, such as accurately computing payrolls. 3. Verification: o Centers on correctness and asks, “Am I building the product right?” This ensures adherence to specifications and confirms the product’s intended functions. 4. Validation: o Looks at appropriateness, asking, “Am I building the right product?” It assesses whether the product fulfills actual user needs. Validation is more subjective and can evolve as real-world demands change. Relationship Between Verification and Validation Independence of Verification and Validation: MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan o Verification and validation are independent. A product may align with its specification but still miss the mark on user needs if the initial specifications were incomplete or outdated. o Example: A system might be technically correct but fail to satisfy current user practices due to an outdated specification. OBJECT-ORIENTED SYSTEMS DEVELOPMENT AND USE CASES Identifying System Requirements through Actors and Use Cases Actors and System Interaction: Identifying actors (users or entities) helps clarify who will interact with the system and how. Scenarios are developed to illustrate these interactions. Use Case Concept: Introduced by Ivar Jacobson, a use case captures typical interactions between a user and the system, focusing on user goals and system responsibilities. Role in Object-Oriented Programming: Use cases define object collaboration needed to achieve user goals, covering both normal behaviors and exceptions for complete interaction insights. Use-Case Modeling Capturing User Requirements: Use-case modeling frames high-level processes and user interactions from a user-centric perspective. Iterative Development: The model evolves iteratively as understanding deepens, leading to class identification and relationship-building. Example: In a word processor, use cases like replacing a word with a synonym align system functionalities with user expectations. Scenarios and Use Cases with Actors MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan Scenarios: Scenarios are examples of real-life system usage that illustrate user actions and object interactions. Actors: An actor is any entity interacting with the system, whose goals and actions drive use case development. Use Cases: Use cases are structured scenarios representing specific user goals and system responses and have become foundational in object-oriented design. Collaboration in Object-Oriented Design Object Interactions: Objects collaborate to perform tasks, interacting through messages and functions to fulfill use case goals. Scenarios as Examples of Collaboration: Scenarios reveal collaboration patterns among objects, aiding in the design of flexible, reusable components. Iterative Development: Scenarios are refined to explore actor interactions, covering both standard and exceptional behaviors. Documentation Essential but Efficient: Documentation is crucial throughout development. Following the 80-20 rule, developers aim to achieve 80% of the effective work with only 20% of the documentation. This approach ensures that essential documentation is concise and accessible. Integration with Modeling: Effective documentation is closely tied to good modeling. Primary documentation should be easily accessible for users, while extended technical details are made available for those who need them. Documentation and modeling are not separate tasks; rather, good modeling inherently results in good documentation. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan IMPORTANCE OF SOFTWARE PROTOTYPING Value of Prototyping: A prototype offers a hands-on approach to exploring the usability, functionality, and implementation challenges of a system early in its lifecycle. It helps refine user needs, verify use cases, and align functional specifications with user expectations. Evolution of Prototyping: Traditionally, prototypes were quickly developed and discarded after gathering insights. However, with modern practices like Rapid Application Development (RAD), prototypes often evolve into final products. Horizontal Prototype: Overview: Simulates the entire user interface without backend functionality. Benefits: Quick to build and helps users evaluate the look and feel of the interface. It provides a holistic visual model for users to experience the design and provide feedback on navigational flow. Vertical Prototype: Overview: Focuses on a deep, functional subset of the system. Benefits: Allows thorough testing of key features and addresses areas of high risk. This type is useful for validating the functionality of core system components in isolation before scaling up. Analysis Prototype: Purpose: Helps explore the problem domain and demonstrates a proof of concept. Characteristics: It is discarded once its purpose is fulfilled, as its code is not used in the final system. By focusing on user needs and clarifying requirements, it ensures critical insights are gathered early. Domain Prototype: Purpose: Facilitates incremental development, enabling a gradual build-up towards the final product by integrating key domain elements. Benefits: Assists in aligning technical features with specific domain requirements, ensuring that development consistently addresses the application's core objectives and functionalities. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan USAGE EXAMPLES OF TYPES OF PROTOTYPES Horizontal Prototype: Example: A prototype of an e-commerce website that shows the layout, navigation, and product pages without enabling actual purchasing functions. Users can click through the pages to see how the site will look and feel. Vertical Prototype: Example: A banking app prototype that only implements a fully functional login and balance-checking feature. This allows developers to test and refine these core functions before adding others. Analysis Prototype: Example: A prototype of a logistics system that visualizes delivery routes based on user input but doesn’t have backend data processing. It helps stakeholders confirm the feasibility of route planning concepts before full development. Domain Prototype: Example: A healthcare management system prototype that initially focuses on handling patient information entry and retrieval. As development progresses, more features like appointment scheduling and billing are incrementally added based on real-world needs. COMPONENT-BASED DEVELOPMENT (CBD): Component-Based Development (CBD): Component-Based Development is a method where software is built by assembling pre-existing, tested, and reusable components, much like industrialized processes in other fields. This approach helps developers build applications faster by using components available in catalogs for general or specialized needs, making software development more efficient and flexible. Key Concepts in CBD: 1. Component Assembly: o Applications are constructed by combining components that provide specific functions. o Example: In building an e-commerce platform, developers can use a pre- built payment gateway component to handle transactions without coding this feature from scratch. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan 2. Visual and Code-Based Integration: o Components are linked using visual tools (like "wiring" components) or by writing actual code. o Example: Using IBM’s VisualAge to visually connect UI components with backend components, allowing the interface to directly interact with data- handling functions. 3. Independent Service Delivery: o Components provide services independently of each other, even if they interact, allowing flexibility in the system. o Example: In a customer relationship management (CRM) application, a component handling customer data can operate independently of a sales data component while still exchanging information when needed. Advantages CBD speeds up development cycles and improves flexibility and customization, as users see faster product updates and tailored features without affecting other parts of the application. Component-based development focuses on building software through reusability and integration. Google API Example as CBD Google APIs are a prime example of Component-Based Development (CBD) in action. They serve as ready-made, reusable components that developers can easily integrate into their applications to access Google’s services and data without needing to build these functionalities from scratch. Here’s how Google APIs exemplify CBD: 1. Pre-Built, Specialized Functionality Google APIs provide specialized services, such as Google Maps for location services, Google Drive for storage, and Google Translate for language translation. These APIs allow developers to add complex functionalities to their apps quickly. Example: An app needing geolocation features can use the Google Maps API rather than developing its own mapping system, saving time and effort. 2. Interoperability and Flexibility Google APIs are designed to be interoperable, meaning they work well within various platforms and applications. This modularity allows them to interact seamlessly with different systems. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan Example: A travel application can integrate both the Google Flights API and Google Maps API to provide users with travel booking options and location details in a unified experience. RAPID APPLICATION DEVELOPMENT (RAD) Rapid Application Development (RAD) is a methodology designed to speed up the software development process by using tools and techniques that facilitate rapid building, prototyping, and incremental improvements to the application. Key Aspects of RAD in Software Development 1. Implementation and Iterative Development o RAD focuses on quickly creating a working model of the application to validate the understanding of requirements. It doesn’t abandon the Software Development Life Cycle (SDLC) but instead uses iterative development cycles. o Tools like Delphi, VisualAge, Visual Basic, or PowerBuilder allow developers to create functional prototypes, gather user feedback, and continuously refine the design and functionality. 2. System Integration o Integration is managed incrementally in RAD, where each prototype is tested to ensure that it aligns with the system’s design and functional requirements. This incremental approach reduces the risk of extensive rework at the end of the project. 3. Testing and Feedback o Each RAD iteration allows for frequent testing and user feedback, which is essential for identifying and resolving potential issues early in the development cycle. This process leads to a higher chance of delivering a functional product that meets user expectations. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan METHODOLOGIES RUMBAUGH ET AL.'S OBJECT MODELING TECHNIQUE (OMT) Rumbaugh's Object Modeling Technique (OMT) offers a structured approach to analyze, design, and implement a system with object-oriented principles. This technique, developed by Jim Rumbaugh and colleagues, supports a clear, intuitive way to identify and model system objects and their interactions. Key Components of OMT 1. Object Model The Object Model focuses on the static structure of the system by representing the system’s objects, their relationships, attributes, and operations.\ Classes and Objects: A class represents a blueprint for objects with shared attributes and behaviors, while an object is an instance of a class. For example, in a banking system, a "Customer" class could define attributes like name and account number, and operations like deposit or withdraw. Attributes and Operations: Each class has attributes (data specific to the object) and operations (functions that objects can perform). In our banking example, the "Customer" class might have attributes like customerID and balance, with operations like checkBalance and transferFunds. Relationships and Associations: Relationships represent how classes connect or interact with each other. Associations, often shown as lines between classes in an object diagram, describe links like inheritance (e.g., “SavingsAccount” inherits from “Account”) or aggregation (e.g., a “Bank” contains multiple “Account” objects). The Object Model provides a foundational view of the system and captures all the static elements that don't change with time. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan 2. Dynamic Model The Dynamic Model is concerned with the behavior of the system over time, focusing on changes in the state of objects in response to events. This model uses state transition diagrams to illustrate states, events, actions, and transitions between states. States: A state represents a condition in the lifecycle of an object where it satisfies certain criteria or performs a specific role. For example, in an ATM system, a state could be "Idle," "Card Inserted," or "Transaction Processing." Events and Actions: Events trigger transitions between states. An event could be a user action (e.g., entering a PIN) or an internal signal (e.g., an error). Actions are tasks performed as a result of an event, like displaying a welcome message. Transitions: Transitions are the connections between states that show the movement from one state to another. For example , an ATM might transition from "Idle" to "Card Inserted" upon detecting a card insertion event. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan By modeling the dynamic aspects, OMT allows you to understand how the system behaves under different conditions and how it responds to inputs over time. 3. Functional Model The Functional Model illustrates the flow of data within the system and is typically represented with Data Flow Diagrams (DFDs). This model shows how data moves between different processes and entities, making it ideal for capturing the business processes of the system. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan Processes: These are activities or transformations performed on data. In a DFD, processes are usually represented by circles or ovals. For example, in an ATM system, "Verify PIN" could be a process where the entered PIN is matched against the stored value. Data Flows: Arrows in the DFD represent the direction in which data moves from one process to another. For exampele, an arrow labeled "PIN code" might show data flowing from the user input to the "Verify PIN" process. Data Stores: Data stores are repositories where data is held for use by processes. In an ATM, a data store might be "Account Database," which contains records of user account details. External Entities: These represent sources or destinations of data outside the system, like users or external systems. In an ATM system, the card reader might be an external entity that inputs card data into the system. The Functional Model helps break down the system’s processes into manageable parts, showing how data moves and is transformed, providing a high-level view of system functionality. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan THE BOOCH METHODOLOGY The Booch methodology is a comprehensive, object-oriented approach for the analysis, design, and implementation of systems, providing detailed diagrams and frameworks for both high-level and low-level development. Let’s break down its components. Diagrams in the Booch Methodology Booch uses a set of diagrams to document and design object-oriented systems comprehensively: 1. Class Diagrams: Represent the classes in the system, their attributes, and methods, showing the relationships among classes. 2. Object Diagrams: Depict instances of classes and the relationships between specific objects in various scenarios. 3. State Transition Diagrams: Show how objects move from one state to another in response to events, helpful in modeling the lifecycle of objects. 4. Module Diagrams: Map out the modular structure, detailing where classes and objects reside in the codebase. 5. Process Diagrams: Describe processes and processors, defining where each process will run within the system and managing concurrent processing. 6. Interaction Diagrams: Illustrate object interactions over time, capturing the sequence of messages exchanged between objects for particular functions. Fig: Object modelling using Booch notation MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan The Macro Development Process The macro process is a broader framework governing the high-level, technical management of the system and typically spans weeks or months, focusing on project requirements and delivery timelines rather than the details of object-oriented design. Its steps include: 1. Conceptualization: Define core requirements, establish project goals, and develop prototypes to validate the concept. 2. Analysis and Model Development: Create class and object diagrams, describe object roles and responsibilities, and use interaction diagrams to model system scenarios. 3. System Architecture Design: Use class and object diagrams to determine the class structure and relationships, module diagrams to map the modular layout, and process diagrams to assign processes to processors and schedule tasks. 4. Evolution (Implementation): Refine the system through iterative cycles, producing successive software releases, each improving on the last. 5. Maintenance: Make necessary adjustments and bug fixes, adapting the system to meet new requirements over time. The Micro Development Process The micro process involves day-to-day development activities, often managed by individual developers or small teams. These steps focus on refining the details of each class and object: 1. Identify Classes and Objects: Determine the key classes and objects in the system. 2. Define Class and Object Semantics: Specify the purpose, attributes, and methods for each class or object. 3. Establish Relationships: Define relationships between classes and objects, such as inheritance, association, and aggregation. 4. Define Interfaces and Implementations: Determine how classes and objects will interact (interfaces) and implement these designs. JACOBSON ET AL. METHODOLOGIES MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan The Jacobson et al. methodologies, which include Object-Oriented Business Engineering (OOBE), Object-Oriented Software Engineering (OOSE), and Objectory, provide a comprehensive framework that spans the entire software development life cycle. These methodologies emphasize traceability between various phases—both forward and backward—which facilitates the reuse of analysis and design work. This is often more impactful in reducing development time than simply reusing code. Central to these methodologies is the concept of use cases, which play a crucial role in capturing system requirements. USE CASES Use cases are defined as scenarios that illustrate how users interact with a system to achieve specific goals. They serve to clarify the responsibilities of the system to its users and help define requirements in a structured way. Key elements of use cases include: Structure of Use Cases: Use cases can be described in various formats during requirements analysis: o Nonformal Text: A general narrative without a clear flow of events. o Readable Text: A structured narrative that presents a clear flow of events, which is the recommended style for clarity. o Formal Style: Utilizing pseudo-code for precise definitions. Essential Components: A use case description must address several critical aspects: o Start and End Points: Clearly define when the use case begins and when it ends. o User Interaction: Describe the interactions between the use case and its actors (users or other systems), including timing and the nature of exchanged information. o Data Handling: Specify how and when the use case will utilize or store data within the system. o Exception Handling: Outline any deviations from the main flow of events. o Problem Domain Concepts: Detail how various concepts relevant to the problem domain are managed. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan Flow of Events: Each use case should primarily focus on one main flow of events. Additional or exceptional flows can be included: o Exceptional Use Cases: These extend another use case by adding extra functionality. They are similar to subclasses in object-oriented programming. o Uses Relationship: This relationship is used to capture common behaviors that can be reused across different use cases. Concrete vs. Abstract Use Cases: Use cases can be classified as concrete or abstract: o Concrete Use Cases: Fully defined use cases with specific actors initiating them. o Abstract Use Cases: Incomplete use cases that do not have initiating actors. They serve as templates for other use cases and can include relationships such as "uses" or "extends." OBJECT-ORIENTED SOFTWARE ENGINEERING (OOSE) / OBJECTORY Object-Oriented Software Engineering (OOSE) / Objectory is a method designed for developing large, real-time systems. It emphasizes a use-case driven approach, integrating use cases throughout all stages of development: analysis, design, validation, and testing. This helps ensure the software aligns closely with user needs. Key Components of Objectory 1. Use Case-Driven Development: o Use cases outline interactions between users and the system, providing a clear understanding of user requirements and how the system should behave. 2. Disciplined Process: o Objectory promotes a structured approach, focusing on user interactions to create software that is user-friendly and adaptable to changes. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan Models in Objectory 1. Use Case Model: o Defines external actors (users) and the use cases representing system behavior. 2. Domain Object Model: o Maps real-world entities into the software, outlining their attributes and relationships. 3. Analysis Object Model: o Provides a blueprint for developers on how the source code should be structured. 4. Implementation Model: o Details the actual coding and architecture of the system. 5. Test Model: o Outlines testing strategies, plans, and specifications to ensure quality. Advantages of Objectory User-Centric Focus: Prioritizes user needs, leading to higher satisfaction. Robustness and Adaptability: Results in systems that can easily adapt to changes. Comprehensive Framework: Covers the entire software development life cycle, ensuring consistency and traceability. PATTERNS IN SOFTWARE DEVELOPMENT Definition of Patterns: Patterns are established solutions to recurring problems in software design and architecture. They serve as templates that developers can reference to solve specific issues effectively. Purpose of Patterns: They help standardize solutions, making it easier for developers to communicate ideas and collaborate. Patterns provide a common language to describe design strategies. Peer Review: MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan It's essential for a pattern to undergo some level of peer scrutiny. This ensures that the solution has been validated by others in the field, contributing to its credibility. Criteria for a Good Pattern: Solves a Problem: Patterns should effectively address real-world problems, capturing practical solutions rather than just abstract ideas. Proven Concept: A pattern must have a track record of success, rather than being based on theories or untested speculation. Non-obvious Solutions: Good patterns provide indirect solutions to complex design challenges rather than straightforward answers. Describes Relationships: Patterns should articulate the relationships between components, detailing not just isolated modules but the deeper structures and mechanisms of the system. Significant Human Component: The best patterns cater to human needs and aesthetics, enhancing user comfort and quality of life. Generative vs. Nongenerative Patterns 1. Generative Patterns: o These patterns not only identify recurring problems but also provide methods for generating solutions. They can be observed in the system architectures they help shape. 2. Nongenerative Patterns: o These are more static and merely describe recurring phenomena without offering a way to reproduce them. While useful, they don’t contribute to the active generation of solutions. Pattern Template Name: A descriptive title that helps identify and refer to the pattern. Context: The specific situation or environment in which the pattern is applicable. This outlines when to use the pattern. Motivation: The rationale behind the pattern, explaining the problem it addresses and why a particular approach is beneficial. Solution: The core of the pattern that details the design structure and behavior. This section outlines how to implement the solution effectively. Examples: Real-world scenarios where the pattern has been applied, providing practical illustrations of its use. These can include code snippets or case studies. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan Resulting Context: The state of the system after the pattern has been applied. This includes any changes made, benefits gained, and potential new issues that might arise. Rationale: This explains why the solution works and how it aligns with the goals and principles of good software design. It helps developers understand the underlying mechanisms. Related Patterns: This shows connections to other patterns, indicating how they can complement each other or be used in tandem. This includes predecessor patterns that lead to this one and alternative patterns that provide different solutions. Known Uses: Examples of existing systems or projects where the pattern has been successfully implemented. This helps validate the pattern's effectiveness. Antipatterns 1. Definition of Antipatterns: Antipatterns are common mistakes or ineffective solutions that developers should avoid. They serve as lessons learned from previous failures or inefficiencies. 2. Purpose of Antipatterns: They highlight what not to do in software design, providing valuable insights into pitfalls that can lead to poor outcomes. Understanding antipatterns can help developers make better design choices. 3. Types of Antipatterns: Bad Solutions: These describe ineffective approaches that lead to negative consequences. Remediation Strategies: These outline how to rectify a bad situation and transition to a better solution, guiding developers on how to improve after encountering a problem. FRAMEWORKS Frameworks are structured tools that facilitate application development by promoting best practices and solutions that can be shared across various organizations. They help developers by providing a foundation that incorporates proven methodologies and patterns. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan Key Characteristics 1. Pre-built Components: o Developers often don’t start coding from scratch. Instead, they utilize existing code snippets, libraries, and templates to speed up development. 2. Generic Solutions: o A framework presents a generic solution to common problems, applicable at different levels of development. 3. Object-Oriented Software Framework: o According to Gamma et al., an object-oriented software framework consists of a collection of cooperating classes designed to provide reusable solutions for specific types of software. 4. Customization: o Developers can adapt frameworks to meet the needs of specific applications by subclassing (extending existing classes) and composing instances of the framework's classes. 5. Design Reuse: o Frameworks focus on reusing design patterns rather than just code. Examples Web Development Frameworks Django: A high-level Python web framework for rapid development and clean design. Angular: A JavaScript framework for creating dynamic single-page applications using HTML and TypeScript. Mobile Development Frameworks React Native: A framework for building native mobile apps using JavaScript and React. Flutter: A UI toolkit for building natively compiled applications across mobile, web, and desktop using Dart. UNIFIED APPROACH Unified Approach: This methodology integrates various software development processes, such as analysis, design, and implementation, into a cohesive framework. It emphasizes the use of standardized practices and tools to enhance communication and collaboration among team members, ensuring consistency across the development lifecycle. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan Use Case Driven Development Use Case Driven Development: This approach focuses on defining the system's functional requirements through use cases, which describe how users interact with the system. Object-Oriented Analysis (OOA) Object-Oriented Analysis (OOA): OOA involves studying the problem domain to identify key objects, their attributes, and relationships. By modeling real-world entities as objects, OOA facilitates a deeper understanding of system requirements and helps in creating a conceptual model that serves as a foundation for design. Object-Oriented Design (OOD) Object-Oriented Design (OOD): Building on OOA, OOD focuses on structuring the software architecture by defining classes, interfaces, and their interactions. MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan Incremental Development and Prototyping Incremental Development and Prototyping: This methodology involves developing a system in small, manageable parts, allowing for regular user feedback and testing. Continuous Testing Continuous Testing: This practice integrates automated testing throughout the software development lifecycle. By continuously testing code as it's written and modified, developers can identify defects early, ensuring that the software maintains a high level of quality. TECHNOLOGY INCLUDE in UML UML for Modelling: The Unified Modeling Language (UML) is a standardized way to visualize software designs. It includes various diagram types, such as class diagrams, use case diagrams, and sequence diagrams, to represent different aspects of a system. Layered Approach: This design strategy organizes software into distinct layers, each responsible for specific functionalities. The layered architecture promotes separation of concerns, making it easier to manage complexity, enhance maintainability, and facilitate testing. Repository for OOSD Patterns and Frameworks: A centralized repository stores object-oriented software development patterns and frameworks. This repository serves as a knowledge base that developers can reference for best practices and reusable components, promoting efficiency and consistency in software development. Component-Based Development (CBD): CBD focuses on building software systems from reusable, self-contained components. Each component encapsulates specific functionality and can be independently developed and tested. Layered Approach in Software Development 1. User Interface (View) Layer o Description: This layer is responsible for presenting data to the user and handling user interactions. It includes elements like buttons, forms, and other UI components. o Responsibilities: MIIT MCA -SOFTWARE ENGINEERING Module 3 Dr Lino A Tharakan ▪ Displaying information to the user. ▪ Capturing user input and actions. ▪ Communicating with the business layer to send or receive data. o Technologies: HTML, CSS, JavaScript, frameworks like React, Angular, or Vue.js. 2. Business Layer o Description: Also known as the domain layer, this layer contains the core business logic of the application. It processes data received from the UI layer, performs calculations, enforces business rules, and manages transactions. o Responsibilities: ▪ Implementing business rules and logic. ▪ Validating data and enforcing constraints. ▪ Coordinating data flow between the user interface and access layer. o Technologies: Java, C#, Python, or any programming language suitable for implementing business logic. 3. Access Layer o Description: This layer serves as an intermediary between the business layer and the data source. o Responsibilities: ▪ Interacting with databases or external services. ▪ Performing CRUD (Create, Read, Update, Delete) operations. ▪ Abstracting data access methods to provide a cleaner interface for the business layer. o Technologies: ORM (Object-Relational Mapping) frameworks like Hibernate, Entity Framework, or direct database access technologies like SQL, MongoDB.