Module 2 Strings - Learn C Programming
Document Details
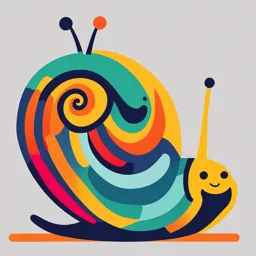
Uploaded by QuickerSynecdoche
Global Academy of Technology
Tags
Summary
This document provides an overview of strings in C programming. It covers string declaration, initialization, I/O operations, and various string-handling functions along with example programs. Topics include reading and writing strings, string manipulation and converting between strings and characters.
Full Transcript
Module-2 Strings Introduction A string is a sequence of characters terminated by a null character ‘\0’. ‘\0’ is automatically encountered at the end of the string. Declaration of string char ch ; Here, ch is a string which can store a maximum of 5 characte...
Module-2 Strings Introduction A string is a sequence of characters terminated by a null character ‘\0’. ‘\0’ is automatically encountered at the end of the string. Declaration of string char ch ; Here, ch is a string which can store a maximum of 5 characters and 1 character is reserved for ‘\0’. Initialization of string char ch = “HELLO” ; ch ch ch ch ch ch ‘H’ ‘E’ ‘L’ ‘L’ ‘O’ ‘\0’ char ch = “Good” ; ch ch ch ch ch ch ‘G’ ‘o’ ‘o’ ‘d’ ‘\0’ ‘\0’ char ch = { ‘P’, ‘r’, ‘e’, ‘m’, ‘\0’ } ; ch ch ch ch ch ch ‘P’ ‘r’ ‘e’ ‘m’ ‘\0’ ‘\0’ ch ch ch ch ch ch char ch = “Program” ; ‘P’ ‘r’ ‘o’ ‘g’ ‘r’ ‘a’ Initialization of string Invalid Initialization char str3; str3 = “GOOD”; I/O operations on strings scanf() printf() char address ; char address ; scanf(“%s”, address); scanf(“%s”, address); printf(“%s”, address); gets() puts() char address ; char address ; gets(address); gets(address); puts(address); Reading Text till ~ is encountered char line ; scanf("%[^~]", line); I/O operations on strings #include void main() { char line ; printf("Enter string\n"); gets(line); printf("The string is: "); puts(line); } I/O operations on strings #include #include void main() void main() { { char char a="GLO a="GLO BAL" ; BAL" ; int i,j; int i,j; for(i=0;i l) { int main() if (str[l++] != str[h--]) { { char str[] = { "abbba" }; printf("%s is not a palindrome\n", str); // Start from first and return 0; // last character of str // will return from here int l = 0; } int h = strlen(str) - 1; } printf("%s is a palindrome\n", str); return 0; } String/Data Conversion Morse Code