JavaScript Lecture Week 3 PDF
Document Details
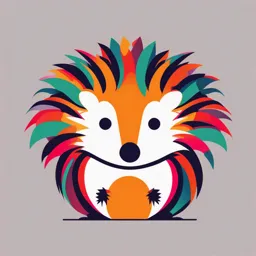
Uploaded by FormidableGyrolite3794
Herald College
Tags
Related
- Aula 6 – Programação web utilizando Javascript PDF
- IT 304 Web Systems and Technologies I - Javascript PDF
- Advanced Web Programming - Chapter 8 PDF
- Cours 4 Développement Web LST Génie Informatique 2024-2025 PDF
- Computer Programming 3 - Discussion 2 (1) PDF
- Fundamentals of Web Development Chapter 10 PDF
Summary
This document is a lecture on JavaScript, covering topics such as introduction, variables, conditional statements, loops, and objects, targeting a university level audience. The lecture is provided by Herald College in Kathmandu.
Full Transcript
4CS017 Internet Software Architecture 1 Web Technologies Lecture Week 3 Introduction to JavaScript 2 This week’s agenda Introduction to JavaScript Exploring the console Declaring variables and functions Conditional Statements JavaSc...
4CS017 Internet Software Architecture 1 Web Technologies Lecture Week 3 Introduction to JavaScript 2 This week’s agenda Introduction to JavaScript Exploring the console Declaring variables and functions Conditional Statements JavaScript Loops Understanding Objects, and Arrays 3 Introduction to JavaScript… JavaScript was originally made to make “webpages alive”. It was widely used to client-side interactivity. The programs in this language are called scripts. It is written as plain text and can be run when the page loads. They don’t need special preparation or compilation to run. Today, JavaScript can execute not only in the browser, but also on the server or any device that has a special program called the “JavaScript 4 Engine”. Introduction to JavaScript… The browser has an embedded engine sometimes called the “JavaScript Virtual Machine”. Different engines has different codenames: V8 – Google Chrome & Opera SpiderMonkey – Firefox ChakraCore – Microsoft Edge Nitro & SquirrelFish – Safari Remembering and understanding this engines is necessary, as most 5 of the developer articles uses this for reference. Introduction to JavaScript JavaScript can be written in the HTML document itself or can be written in a separate “.js” file. The latter is preferred. While writing JavaScript program into the HTML file itself, it needs to be wrapped inside the tag. console.log(“Hello World!!”); //This code prints “Hello World!!” in the browser console. 6 While writing in a separate file, the script tag is not needed. Exploring the console… Console is one of the most important in-browser tool while scripting in JavaScript. Console is a REPL, which stands for read, evaluate, print and loop. Meaning, it reads the JavaScript that you type into it, evaluates your code, prints out the result of your expression and then loops back to the first step. The console is also used for debugging purposes. And this is why it is important as a JavaScript programmer. 7 Exploring the console The console is considered as an object of the browser and can be used to access the browser’s debugging console. The console object only works in the browser’s console and not on the pages. Some of the most popular console methods are: console.log() console.table() console.clear() 8 Any Questions 9 10 Declaring Variables… Variables in JavaScript can be declared with three different keywords. Each with its own significant and use. The variables in JavaScript has its own scope. The scope meaning the area it can be accessed from and within. The variables are either Functional Scope or Block Scope. The three keywords to declare a variable are: var – The most common way of declaring a variable in JavaScript. let – “let” allows you to declare a block scoped variable. 11 const – It allows you define a variable with constant value. Declaring Variables… Using “var” keyword: - “var” is a global scoped variable keyword. - The values of the variable declared using “var” keyword can be changed or manipulated. var whatarewelearning = “JavaScript”; whatarewelearning = “Introduction to Web Technologies”; console.log(whatarewelearning); //Returns “Introduction to12Web Technologies” Declaring Variables… Using “let” keyword: - “let” is a block scoped variable keyword. - The values of the variable declared using “let” keyword can be changed or manipulated. let whatarewelearning = “JavaScript”; whatarewelearning = “Introduction to Web Technologies”; console.log(whatarewelearning); //Returns “Introduction to Web 13 Technologies” Declaring Variables (let vs var) for(let i=0; i 18){ console.log(“Qualified for driving”); } 20 If… else statement The if...else statement is the next form of control statement that allows JavaScript to execute statement in more controlled way. var age = 20; if(age > 18){ console.log(“Qualified for driving”); }else{ console.log(“Not qualified for driving”); } 21 If… else if… else statement The if...else if... statement is the one level advance form of control statement that allows JavaScript to make correct decision out of several conditions var book = “maths”; if(book == “history”){ console.log(“Your book is history book.”); }else if(book == “maths”){ console.log(“Your book is math book”); 22 }else if(book == “economics”){ console.log(“Your book is economics book”); }else{ console.log(“Your book is unknown”); } Switch statement… You can use multiple if...else if statements, as in the previous slides to perform a multiway branch. However, this is not always the best solution, especially when all of the branches depend on the value of a single variable. You can use a switch statement which handles exactly this situation, and it does so more efficiently than repeated if...else if statements. 23 Switch statement var grade = “A”; switch(grade){ case ‘A’: console.log(“Good Job ”); break; case ‘B’: console.log(“Pretty Good ”); break; case ‘C’: console.log(“Above Average ”); break; case ‘D’: console.log(“Average ”); break; case “E”: console.log(“Poor ”); 24 break; case “F”: console.log(“Failed”); break; default: console.log(“Marks not obtained”) } Any Questions 25 be back in 10 minutes… 26 27 JavaScript Loops… JavaScript performs several types of repetitive operations, called "looping". Loops are set of instructions used to repeat the same block of code till a specified condition returns false or true depending on how you need it. To control the loops you can use counter variable that increments or decrements with each repetition of the loop. JavaScript supports different types of loops. Some loops works best 28 for numbers, some for arrays and some for objects. JavaScript Loop: For The JavaScript for loop repeats a series of statements any number of times and includes and optional loop counter that can be used in the execution of the statements. var text; for(var i=0;i