Lecture 6.1_ Strings.pdf
Document Details
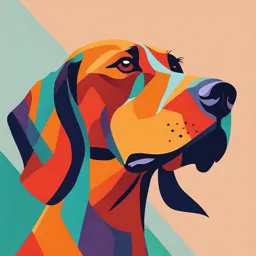
Uploaded by GenerousChrysoprase
La Trobe University
Tags
Full Transcript
Lecture 6.1 Strings Topic 6 Intended Learning Outcomes ◎ By the end of week 6 you should be able to: ○ Express text in Python using different kinds of string literals and escape sequences, ○ Build strings in a neater and more flexible way using fstrings, ○ Read and write data from text files, and...
Lecture 6.1 Strings Topic 6 Intended Learning Outcomes ◎ By the end of week 6 you should be able to: ○ Express text in Python using different kinds of string literals and escape sequences, ○ Build strings in a neater and more flexible way using fstrings, ○ Read and write data from text files, and ○ Perform various file system operations. 2 Lecture Overview 1. More String Literals 2. Manipulating Strings 3. f-strings and Formatting 3 1. More String Literals 4 What The Literal... ◎ Up until this point, we've been using single quotes around text to create string literals. ○ e.g. 'Programming’ ◎ But if a single quote indicates the end of the string, how do we include a single quote inside the string? ○ 'This doesn't work’ ◎ And what about other special characters, like newlines and tabs? 5 Escape Character ◎ In Python the backslash, \, is an escape character which changes how the character after it is interpreted inside a string. >>> print('Quotes aren\'t a problem') Quotes aren't a problem ◎ \' is an example of an escape sequence. ◎ Placing a backslash before a single quote tells Python "this isn't the end of the string, it's just a single quotation mark". 6 Common Escape Sequences OPERATOR NAME \\ One backslash (\) \' Single quote (') \" Double quote (") \n Newline \t Horizontal tab 7 Example: Escape Sequences >>> print('First line\nSecond line') First line Second line >>> print('\\\'o\'/') \'o’/ >>> print('"Free"') "Free“ >>> print('\"Free\"') "Free" 8 Alternative String Literal Syntax ◎ Although you can use escape sequences to express any string you can think of, it can start to look a bit ugly. ○ e.g. '\'\'\'\n\'\'\‘’ ◎ Python provides alternative ways of expressing string literals which you can choose between. 9 Double Quote Strings ◎ You can use double quotes instead of single quotes. ○ Changes which type of quotes need to be escaped. ◎ Useful when you need to include apostrophes. ○ e.g. "I don't need to escape" ◎ The trade-off is that double quotes now need to be escaped. ○ e.g. "It isn't the \"real\" you" 10 Multiline Strings ◎ You can write a string that spans multiple lines by using three single/double quotes. ◎ This avoids the need to use \n to separate lines. ○ You can still use \n inside multiline strings if you want, though. 11 Example: Multiline Strings >>> ... One Two >>> ... "1" "2" print("""One Two""") print('''"1"\n"2" "3"''') "3" 12 Indentation with Multiline Strings ◎ Note that the space included in the string matters, so things can look a bit weird when code is indented. ring = True if ring: print('''ding dong''') Output: ding dong 13 Indentation with Multiline Strings ◎ Python allows you to break the usual indentation rules inside multiline strings to work around this problem. ring = True if ring: print('''ding dong''') Output: ding dong 14 Check Your Understanding Q. Use a double-quoted, singleline string literal to express the following text: Is it cheap, Or "cheap"? 15 Check Your Understanding Q. Use a double-quoted, single- A. "Is it cheap,\nOr \"cheap\"?" line string literal to express the ◎ \n represents the newline. following text: ◎ \" represents double quotes. Is it cheap, Or "cheap"? 16 2. Manipulating Strings 17 Character Sequences ◎ A string is a sequence of characters. ◎ The length of a string is the number of characters in the string. ○ You can use the len built-in function to get this value. ○ e.g. len('Avocado') returns 7. ◎ An escape sequence counts as 1 character. ○ e.g. len('A\nB') returns 3. 18 Character Sequences ◎ The index of a character indicates its position in the string. ◎ Indices start at 0 (so the first character is at index 0). ◎ The last index is the length of the string minus 1. 19 Character Sequences 'Star Wars' S t a r 0 1 2 3 4 W a r s 5 6 7 8 ◎ The character at index 1 is t. ◎ The character at index 4 is the space character, . 20 Negative Indices 'Star Wars' S t a r -9 -8 -7 -6 -5 W a r s -4 -3 -2 -1 ◎ In Python, you can use negative indices to count backwards from the end of the string. ◎ The character at index -1 is the last character of the string (in this case, s). 21 Indexing a String ◎ You can use square brackets [] to retrieve a character by its index. ◎ This is called indexing a string. ◎ You can use normal or negative indices. >>> movie = 'Star Wars' >>> movie[0] 'S' >>> movie[-2] 'r' 22 Slicing a String ◎ You can also use square brackets to retrieve a segment of a string (a substring). >>> movie = 'Star Wars' >>> movie[0:3] 'Sta' >>> movie[-4:-1] ◎ This is called slicing a string. ◎ Notice that the character at the first index is included but the character at the last index is excluded. 'War' 23 Slicing a String ◎ If you omit the first index, all characters from the start of the string are returned. >>> movie = 'Star Wars' >>> movie[:4] 'Star' >>> movie[-4:] 'Wars' ◎ If you omit the second index while slicing, all characters to the end of the string are returned. 24 Looping Over Characters ◎ Since a string is a sequence of characters, it can be used in a for loop to iterate over each character. ◎ Each item is a string of length 1 containing a single character, starting with the first character. 25 Example: Looping Over Characters dna = 'GATTACA' for nucleotide in dna: if nucleotide == 'G' or nucleotide == 'A': print(nucleotide) Output: G A A A 26 Searching ◎ The find string method returns the position of the first occurrence of a smaller substring within a larger string. >>> s = "Where's Wally?" >>> s.find('W') 0 >>> s.find('Wally') 8 ◎ The position returned is the index of the first character of the matching part of the string. 27 Failing to Find ◎ If the string does not contain the substring, -1 is returned. >>> s = "Where's Wally?" >>> s.find('Odlaw') -1 >>> s.find('w') -1 28 Searching Backwards ◎ You can find the last instance of the substring (instead of the first) but using the rfind string method. >>> s = "Where's Wally?" >>> s.find('e') 2 >>> s.rfind('e') 4 29 Case and String Comparison ◎ It is important to keep in mind that the case of letters matters when comparing strings. >>> 'earth' == 'Earth' False >>> 'grand canyon'.find('canyon') 6 >>> 'Grand Canyon'.find('canyon') -1 ◎ An uppercase letter (e.g. 'A') is different to a lowercase letter (e.g. 'a'). 30 Case and String Comparison ◎ To ignore case, you can convert all of the letters to either lowercase or uppercase before comparing. ○ lower converts all letters to lowercase. ○ upper converts all letters to UPPERCASE. >>> 'earTH'.lower() == 'Earth'.lower() True >>> 'grand canyon'.upper().find('CANYON') 6 >>> 'grand canyon'.upper().find('canyon') -1 31 String Replacement ◎ The replace method can be used to replace parts of a string. It takes 2 arguments: ○ First: The substring to replace. ○ Second: The replacement. >>> s = "Where's Wally?" >>> s.replace('ly', 'do') "Where's Waldo?“ >>> s.replace('W', 'R') "Rhere's Rally?“ >>> s "Where's Wally?" ◎ A new string is returned (the original string is not modified). 32 String Replacement ◎ If the substring isn't found, the string is returned unchanged. ◎ The second argument (the replacement) can be an empty string. ○ Using an empty string will result in the found substrings being replaced with nothing (i.e. removed). 33 Example: Removing Vowels # File: remove_vowels.py vowels = 'aeiouAEIOU' s = input('Enter some text: ') for vowel in vowels: s = s.replace(vowel, '') print(s) Example run: $ python remove_vowels.py Enter some text: A fox is orange. fx s rng. 34 Check Your Understanding Q. What is the result of the following Python expression? 'No worries, Oliver'.rfind('o') 35 Check Your Understanding Q. What is the result of the following Python expression? 'No worries, Oliver'.rfind('o') A. 4. ◎ There are two instances of the substring 'o'. ○ Capital 'O' is different to lowercase 'o’. ◎ rfind searches backwards, so it finds the last 'o’. ◎ The index of the last 'o' is 4. 36 3. f-strings and Formatting 37 String Concatenation Can Be Ugly (and it's not even beautiful on the inside) ◎ So far we have built strings using the + operator. ◎ We used str() to convert other types of data into strings prior to concatenation. ◎ The code from this can become messy. acc = 1 bal = 23.0 print('Account ' + str(acc) + ' balance is $' + str(bal)) 38 f-strings ◎ Python provides f-strings as a convenient way of building strings. ◎ An f-string is a string literal prefixed with an f. ◎ When writing an f-string, you can include Python expressions by using curly brackets. ○ This part of an f-string is called a replacement field. ◎ f-strings reduce the need for + and str(). 39 f-strings # With string concatenation acc = 1 bal = 23.0 print('Account ' + str(acc) + ' balance is $' + str(bal)) # With an f-string acc = 1 bal = 23.0 print(f'Account {acc} balance is ${bal}') ◎ Note that the f-string has an f before the first quote. ◎ {acc} and {bal} are replacement fields. 40 Formatting Values ◎ There are multiple ways of expressing a number. ○ e.g. the number 5 can be written as 5, 5.0, 5.00, etc. ◎ A programmer should be able to choose how a number is displayed depending on the application. ○ For example, dollar amounts are usually expressed using two decimal places (e.g. $1.99). ○ Precise distance measurements might be expressed using many decimal places. 41 Format Specifiers ◎ You can specify how values are to be formatted in an fstring. ◎ This is achieved by including a format specifier in a replacement field. ◎ Format specifiers are actually quite in-depth and allow for lots of customisation. ◎ We will now cover some common kinds of format specifiers. 42 Format Specifiers ◎ A format specifier can be added to a replacement field. ◎ The format specifier is separated from the expression using a colon, :. 43 Number of Decimal Places ◎ You can specify the number of decimal places shown. ◎ Appropriate rounding will be applied. >>> x = 0.6666 >>> f'{x:.3f}' '0.667' >>> f'{x:.0f}' '1' >>> f'{x:.6f}' '0.666600' ◎ Trailing zeros will be added if necessary. 44 Number of Decimal Places ◎ The letter "f" in the format specifier indicates that the value should be presented as a number with a fixedlength fractional part. ◎ This is called the presentation type or format code. >>> x = 0.6666 >>> f'{x:.3f}' '0.667' >>> f'{x:.0f}' '1' >>> f'{x:.6f}' '0.666600' 45 Number of Decimal Places ◎ The ".#" in the format specifier indicates the number of decimal places. >>> x = 0.6666 >>> f'{x:.3f}' '0.667' >>> f'{x:.0f}' '1' >>> f'{x:.6f}' '0.666600' 46 Width ◎ The minimum space that the formatted value will occupy can be specified. ○ The "width" in terms of number of characters. >>> f'{123:6d}' ' 123' >>> f'{123:2d}' '123' >>> f'{0.11:6.2f}' ' 0.11' ◎ The extra room is filled with space characters. ◎ Useful for output. aligning program 47 Width ◎ Notice that spaces are added to the start to ensure that the minimum width is met. >>> f'{123:6d}' ' 123' >>> f'{123:2d}' '123' >>> f'{0.11:6.2f}' ' 0.11' ◎ Strings that are too long are not affected. 48 Width ◎ Using the "d" presentation type indicates that the value should be presented as a whole number without a fractional part. >>> f'{123:6d}' ' 123' >>> f'{123:2d}' '123' >>> f'{0.11:6.2f}' ◎ You can only use "d" when the value is an integer. >>> f'{12.3:6d}' ' 0.11' Traceback (most recent call last): File "<stdin>", line 1, in <module> ValueError: Unknown format code 'd' for object of type 'float' 49 Width ◎ Here’s a times table program that produces nicely aligned output using format specifiers. ◎ Can you see how the spaces automatically added to smaller numbers result in visually pleasing formatting? a = int(input('Times table: ')) for b in range(1, 13): print(f'{a:2d} x {b:2d} = {a * b:3d}') Times table: 11 11 x 1 = 11 11 x 2 = 22 11 x 3 = 33 11 x 4 = 44 11 x 5 = 55 11 x 6 = 66 11 x 7 = 77 11 x 8 = 88 11 x 9 = 99 11 x 10 = 110 11 x 11 = 121 11 x 12 = 132 50 Width for String Values ◎ String values can also be formatted with a minimum width. >>> f'{"hello":8s}' 'hello ‘ >>> f'{96:8s}' Traceback (most recent call last): File "<stdin>", line 1, in <module> ValueError: Unknown format code 's' for object of type 'int' ◎ In order to format a string, you should use the "s" presentation type instead of "d" or "f". ◎ You can only use "s" when the value is a string. 51 Alignment ◎ Notice that, unlike numbers, strings are left-aligned by default rather than right-aligned. ○ Spaces are added to the end, not the start. ◎ You can force a particular alignment using < for left alignment and > for right alignment. >>> f'{"hello":8s}' 'hello ' >>> f'{"hello":>8s}' ' hello' >>> f'{42:6d}' ' 42' >>> f'{42:<6d}' '42 ' 52 Check Your Understanding Q. What will the output of the shown program be? speed_kph = 40.0 time_h = 0.5 print(f'|{time_h * speed_kph:<6.2f}|') 53 Check Your Understanding Q. What will the output of the shown program be? speed_kph = 40.0 time_h = 0.5 A. |20.00 | (note the space between the 0 and the |). print(f'|{time_h * speed_kph:<6.2f}|') 54 Check Your Understanding Q. What will the output of the shown program be? speed_kph = 40.0 time_h = 0.5 print(f'|{time_h * speed_kph:<6.2f}|') A. |20.00 | (note the space between the 0 and the |). ◎ The result of the expression is 20.0. 55 Check Your Understanding Q. What will the output of the shown program be? speed_kph = 40.0 time_h = 0.5 print(f'|{time_h * speed_kph:<6.2f}|') A. |20.00 | (note the space between the 0 and the |). ◎ The .2f part of the format specifier specifies presentation with 2 decimal places (i.e. 20.00). 56 Check Your Understanding Q. What will the output of the shown program be? speed_kph = 40.0 time_h = 0.5 print(f'|{time_h * speed_kph:<6.2f}|') A. |20.00 | (note the space between the 0 and the |). ◎ The 6 part of the format specifier specifies that anything less than 6 characters wide will have spaces added to make it 6 characters wide. 57 Check Your Understanding Q. What will the output of the shown program be? speed_kph = 40.0 time_h = 0.5 print(f'|{time_h * speed_kph:<6.2f}|') A. |20.00 | (note the space between the 0 and the |). ◎ The < part specifies that the spaces should be added to the end (left-aligned). ◎ Since 20.00 is 5 characters wide, the result of <6 is that one space character will be added to the end. 58 Check Your Understanding Q. What will the output of the shown program be? speed_kph = 40.0 time_h = 0.5 print(f'|{time_h * speed_kph:<6.2f}|') A. |20.00 | (note the space between the 0 and the |). ◎ The pipe characters (|) don't have any special meaning, they are just part of the string. 59 Summary 60 In This Lecture We... ◎ Used escape sequences to include characters in strings that can't be written in a straightforward way. ◎ Explored different ways of writing string literals in Python. ◎ Manipulates strings in various ways. ◎ Used f-strings to build strings in a neater and more flexible way. 61 Next Lecture We Will... ◎ Learn how to read and write data in text files. 62 Thanks for your attention! The slides and lecture recording will be made available on LMS. The “Cordelia” presentation template by Jimena Catalina is licensed under CC BY 4.0. PPT Acknowledgement: Dr Aiden Nibali, CS&IT LTU. 63