Lecture 5.2_ Objects.pdf
Document Details
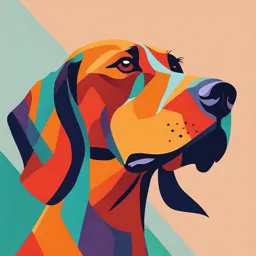
Uploaded by GenerousChrysoprase
La Trobe University
Tags
Full Transcript
Lecture 5.2 Objects Introduction ◎ So far the types of data we have worked with have been defined for us. ○ i.e. floats, integers, strings, etc. ◎ However, we might want to define our own types. ○ e.g. a "student" type that holds a name and ID. ◎ In this lecture we will learn about objects and ho...
Lecture 5.2 Objects Introduction ◎ So far the types of data we have worked with have been defined for us. ○ i.e. floats, integers, strings, etc. ◎ However, we might want to define our own types. ○ e.g. a "student" type that holds a name and ID. ◎ In this lecture we will learn about objects and how to create our own types using classes. 2 Lecture Overview 1. Objects 2. Creating Classes 3. References and Mutability 3 1. Objects 4 Objects Everywhere! ◎ An object consists of: ○ Related pieces of data (its value), and ○ Functions which use or manipulate that data (its methods). ◎ That is, an object knows stuff and can do stuff. ◎ In Python almost everything is an object. ○ Integers, strings, floats, and booleans are all objects. ○ If a variable can hold it, it's an object! 5 Date Objects ◎ We are going to use dates as an example to explore objects further. ◎ In Python, dates are part of the datetime module. ◎ The following line of code enables the creation of new date objects: ○ from datetime import date ◎ We'll learn more about importing modules in a future lecture. 6 Creating a Date Object ◎ A date has three key pieces of ◎ You could picture the date data: the year, the month, and object representing 21/7/2012 the day. like this: ○ It's a bit like three variables in one. ◎ The action of creating a new object is called instantiation. 7 Creating a Date Object ◎ The code for instantiating a new object is similar to a function call. ◎ The instantiated returned. object is >>> from datetime import date >>> my_date = date(2012, 7, 21) ◎ Here we are instantiating an object to represent the date 21/7/2012. ◎ For a date object, the arguments are the year, month, and day (respectively). 8 Creating a Date Object ◎ If we ask Python about the type of my_date, we'll see something interesting. >>> type(my_date) <class 'datetime.date'> ◎ my_date is not an int, str, or float---it's something else entirely! ◎ We say that my_date is an instance of the date class. 9 Accessing Object Data ◎ We can access the three pieces of data stored in my_date by name. >>> my_date.year 2012 >>> my_date.month 7 >>> my_date.day 21 ◎ We say that year, month, and day are attributes of my_date. ◎ Different types of objects have different attributes. 10 Calling Methods ◎ A method is a function attached to an object that has access to the object's value (data). ◎ The methods on my_date are accessed in a similar way to attributes. >>> my_date.isoweekday() 6 ◎ The isoweekday method determines which day of the week the date falls on. ○ Apparently 21/7/2012 was the sixth day of the week (Saturday). 11 What's The Point? ◎ Grouping related data (value) and behaviour (methods) makes code more manageable. ◎ Instead of keeping track of multiple different variables (year, month, day), we can bundle them together. ◎ We also have easy access to related behaviour (e.g. isoweekday). 12 2. Creating Classes 13 What is a Class? ◎ A class is a template from which objects are instantiated (created). ○ A date class describes what a date is (i.e. that it is a year, a month, and a day). ○ A date object describes a particular date, like 21/7/2012. ◎ One class is typically used to create many objects. 14 Class Vs Object 15 Example: Class - Object 16 Example: Class - Object 17 Creating a Custom Class 18 Creating a Custom Class ◎ By creating a class, we have a custom template which we can use to instantiate objects. ◎ Let's create an Ellipse class that has a width and a height. height width 19 Creating an Ellipse Class ◎ __init__ is a special function called a constructor. class Ellipse: def __init__(self): self.width = 2 self.height = 1 ◎ The code in this constructor will be run every time an Ellipse object is instantiated. ◎ Behind the scenes, Python will provide the object instance as the first parameter (self). 20 Creating an Ellipse Class ◎ When instantiating an Ellipse object, two variables called width and height will be attached to it. class Ellipse: def __init__(self): self.width = 2 self.height = 1 ◎ We call width and height instance variables. ◎ width and height will initially be 2 and 1, respectively. 21 Using Our Ellipse Class >>> ell = Ellipse() >>> type(ell) <class '__main__.Ellipse'> >>> ell.width 2 >>> ell.width = 3 >>> ell.width 3 ◎ Notice that we can assign to instance variables, just like regular variables. ◎ Here we change the width of the ellipse from 2 to 3. 22 Constructor Arguments ◎ Currently, if we want to create an ellipse with a particular width and height, we must: 1. Instantiate an Ellipse. ell = Ellipse() 2. Set the width. ell.width = 5 3. Set the height. ell.height = 7 ◎ To make things simpler, we can rewrite the constructor so that the width and height can be passed in as arguments when instantiating the object. 23 Constructor Arguments class Ellipse: def __init__(self, w, h): self.width = w self.height = h >>> ell = Ellipse(5, 7) >>> ell.width 5 >>> ell.height 7 ◎ Now 2 arguments are expected when instantiating an Ellipse. ◎ These arguments are used to set the initial values of the width and height instance variables. 24 What is a Method? ◎ A method is a function which is attached to an object. ◎ Behind the scenes, Python itself will provide the object instance as the first parameter (self). ○ Sound familiar? This is because the constructor, __init__, is a method! 25 Creating a Method class Ellipse: def __init__(self, w, h): self.width = w self.height = h def calculate_area(self): return 3.14159 * self.width * self.height ◎ Here we have defined a calculate_area method which returns the area of the ellipse. 26 Using a Method >>> ell = Ellipse(5, 7) >>> ell.calculate_area() 109.95565 ◎ Notice that we don't pass an argument for self, Python does that automatically. 27 Method Arguments ◎ A method can accept additional argument after self. ◎ A method can also modify instance variables. ◎ Let's add another method to the Ellipse class for scaling its size. 28 Method Arguments class Ellipse: def __init__(self, w, h): self.width = w self.height = h def calculate_area(self): return 3.14159 * self.width * self.height def scale(self, s): self.width = self.width * s self.height = self.height * s ◎ The argument, s, determines the scale factor. 29 Method Arguments >>> ell = Ellipse(3, 4) >>> ell.calculate_area() 37.699079999999995 >>> ell.scale(2) >>> ell.width 6 >>> ell.height 8 >>> ell.calculate_area() ◎ Notice that calling the scale method changes the width and height of our ellipse object. ○ This changes the value returned by calculate_area. 150.79631999999998 30 Check Your Understanding Q. What are the instance variables of the Box class? class Box: def __init__(self, width, height): self.area = width * height self.full = False 31 Check Your Understanding Q. What are the instance variables of the Box class? A. area and full. ◎ width and height are constructor parameters, not instance variables. ◎ The instance variables are attached to the object instance. class Box: def __init__(self, width, height): self.area = width * height self.full = False 32 3. References and Mutability 33 Memory ◎ Every computer has memory. ○ RAM = random access memory. ◎ Memory provides a temporary storage area for programs to store objects. ○ When the program finishes, the objects in memory are no longer accessible. 34 References ◎ So far we have considered variables to be like boxes which hold objects, but this is not strictly true. ◎ In reality, the objects are stored in memory and variables reference those objects. ◎ A variable can only reference one object. ◎ The same object can be referenced by many variables. ◎ Assigning an object to a variable causes the variable to reference that object. ○ It does not copy the object. 35 Example: References 1 2 3 4 a = 5 b = a c = 7 Objects (in memory) a = c References 36 Example: References 1 2 3 4 a = 5 b = a c = 7 Objects (in memory) 5 a = c a References ◎ The integer 5 is assigned to variable a. ○ a now references the integer 5 in memory. 37 Example: References 1 2 3 4 a = 5 b = a c = 7 Objects (in memory) 5 a = c a b References ◎ The object referenced by a (which is the integer 5) is assigned to variable b. ○ Now both b and a reference the integer 5. 38 Example: References 1 2 3 4 a = 5 b = a c = 7 Objects (in memory) 5 7 a = c a b c References ◎ The integer 7 is assigned to variable c. ○ c now references the integer 7 in memory. 39 Example: References 1 2 3 4 a = 5 b = a c = 7 Objects (in memory) 5 7 a = c a b c References ◎ The integer 7 is assigned to variable c. ○ c now references the integer 7 in memory. 40 Objects Without References ◎ An object can only be accessed if there is a reference to it. ○ That is, when there are no references to an object, that object is no longer accessible by the program. ◎ Python will automatically remove objects without references from memory. ○ This helps to free up space in memory. 41 Example: Objects Without References 1 b = 0 Objects (in memory) 5 a 7 b c References 42 Example: Objects Without References 1 b = 0 Objects (in memory) 5 a 7 b 0 c References ◎ b now references the integer 0 in memory. ◎ There are no references left to the integer 5. ○ That object can be automatically removed by Python. 43 Summary of References ◎ Objects are stored in memory. ◎ Variables reference objects. ○ A variable can only reference one object. ○ The same object can be referenced by many variables. ◎ Assignment can be used to change which object a variable references. ◎ Objects with no references are inaccessible. 44 Immutable Objects ◎ An immutable object cannot have its value changed. ◎ Before this lecture, all of the types we've dealt with have been mutable (int, float, etc.). ◎ When using immutable objects in a computation, a new object is created to represent the result. ○ The original objects do not change. 45 Example: Immutable Objects 1 2 3 a = 0 b = a Objects (in memory) b = b + 1 References 46 Example: Immutable Objects 1 2 3 a = 0 b = a Objects (in memory) 0 b = b + 1 a References ◎ The integer 0 is assigned to variable a. ○ a now references the integer 0 in memory. 47 Example: Immutable Objects 1 2 3 a = 0 b = a Objects (in memory) 0 b = b + 1 a b References ◎ The object reference by a (the integer 0) is assigned to variable b. ○ Now both b and a reference the integer 0. 48 Example: Immutable Objects 1 2 3 a = 0 b = a Objects (in memory) 0 b = b + 1 a 1 b References ◎ The new integer 1 (result of b + 1) is assigned to variable b. ○ b now references the integer 1 in memory. ○ a continues to reference the integer 0. ◉ i.e. the original object still has its original value, 0. 49 Mutable Objects ◎ Mutable objects can be changed. ○ We saw this earlier when we changed the width and height of our Ellipse object. ◎ Object instances created from custom classes are mutable. ◎ If multiple variables refer to the same object, mutating the object will affect all of them. 50 Example: Mutable Objects >>> >>> >>> >>> 6 >>> 6 a = Ellipse(3, 4) b = a a.scale(2) a.width ◎ a and b reference the same Ellipse object. b.width ◎ As long as they hold the same reference, a and b can be used interchangeably. 51 Example: Mutable Objects 1 2 3 a = Ellipse(3, 4) b = a a.scale(2) Objects (in memory) width=3 height=4 a References ◎ The Ellipse object is assigned to variable a. ○ a now references the ellipse object in memory. 52 Example: Mutable Objects 1 2 3 a = Ellipse(3, 4) b = a a.scale(2) Objects (in memory) width=3 height=4 a b References ◎ The object referenced by b is assigned to variable a. ○ Now both b and a reference the same Ellipse object. 53 Example: Mutable Objects 1 2 3 a = Ellipse(3, 4) b = a a.scale(2) Objects (in memory) width=6 height=8 a b References ◎ The scale method mutates the Ellipse object. ◎ The effect will be seen by both a and b. 54 Comparing References ◎ In Python, the is keyword can be used to check whether two variables reference the same object. ◎ This is equivalent to checking whether two arrows point to the same object in our diagrams. 55 Example: Comparing References >>> a >>> b >>> c >>> a True >>> a = Ellipse(3, 4) = a = Ellipse(7, 5) is b Objects (in memory) width=3 height=4 width=7 height=5 is c False a b c References 56 Multiple Instances ◎ Using a class to instantiate multiple objects will result in different object instances. ◎ They are different objects even if they have the same value. ○ That is, they will occupy different locations in memory. ○ Mutating one will not affect the other (in general). 57 Example: Multiple Instances >>> >>> >>> >>> 6 >>> 3 >>> a = Ellipse(3, 4) b = Ellipse(3, 4) a.scale(2) a.width ◎ a and b reference different Ellipse objects. b.width ◎ Mutating a does not affect b. a is b False 58 Example: Multiple Instances 1 2 3 a = Ellipse(3, 4) b = Ellipse(3, 4) Objects (in memory) a.scale(2) References 59 Example: Multiple Instances 1 2 3 a = Ellipse(3, 4) b = Ellipse(3, 4) a.scale(2) Objects (in memory) width=3 height=4 a References ◎ The Ellipse object is assigned to variable a. ○ a now references the ellipse object in memory. 60 Example: Multiple Instances 1 2 3 a = Ellipse(3, 4) b = Ellipse(3, 4) a.scale(2) Objects (in memory) width=3 height=4 a width=3 height=4 b References ◎ A different Ellipse object is instantiated and assigned to variable b. ○ b now references the second Ellipse object in memory. 61 Example: Multiple Instances 1 2 3 a = Ellipse(3, 4) b = Ellipse(3, 4) a.scale(2) Objects (in memory) width=6 height=8 a width=3 height=4 b References ◎ The scale method mutates the first Ellipse object. ◎ The effect will be seen by a only. 62 Check Your Understanding Q. Assuming that the Ellipse class has been defined, what will be the outputs of the shown program? 1 2 3 4 5 6 var1 = Ellipse(2, 2) var2 = Ellipse(3, 4) var1 = var2 var1.height = 5 print(var1.width) print(var1.height) 63 Check Your Understanding Q. Assuming that the Ellipse class has been defined, what will be the outputs of the shown program? A. 3 and 5. 1 2 3 4 5 6 var1 = Ellipse(2, 2) var2 = Ellipse(3, 4) var1 = var2 var1.height = 5 print(var1.width) print(var1.height) 64 Check Your Understanding 1 2 3 4 var1 = Ellipse(2, 2) var2 = Ellipse(3, 4) var1 = var2 var1.height = 5 Objects (in memory) References 65 Check Your Understanding 1 2 3 4 var1 = Ellipse(2, 2) var2 = Ellipse(3, 4) var1 = var2 var1.height = 5 Objects (in memory) width=2 height=2 var1 References 66 Check Your Understanding 1 2 3 4 var1 = Ellipse(2, 2) var2 = Ellipse(3, 4) var1 = var2 var1.height = 5 Objects (in memory) width=2 height=2 var1 width=3 height=4 var2 References 67 Check Your Understanding 1 2 3 4 var1 = Ellipse(2, 2) var2 = Ellipse(3, 4) var1 = var2 var1.height = 5 Objects (in memory) width=2 height=2 var1 width=3 height=4 var2 References 68 Check Your Understanding 1 2 3 4 var1 = Ellipse(2, 2) var2 = Ellipse(3, 4) var1 = var2 var1.height = 5 Objects (in memory) width=2 height=2 var1 width=3 height=5 var2 References ◎ So var1.width is 3 and var1.height is 5. 69 Example Summary 71 In This Lecture We... ◎ Learnt that objects combine data and behaviour. ◎ Defined our own types of objects using classes. ◎ Discovered how references work and the difference between mutable and immutable objects. 72 Next Lecture We Will... ◎ Take a closer look at how strings can be created and manipulated. 73 Thanks for your attention! The slides and lecture recording will be made available on LMS. The “Cordelia” presentation template by Jimena Catalina is licensed under CC BY 4.0. PPT Acknowledgement: Dr Aiden Nibali, CS&IT LTU. 74