Lecture 1.PDF
Document Details
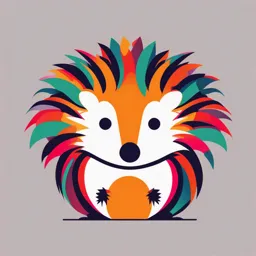
Uploaded by EntrancedSerendipity1806
Tags
Related
- Object Oriented Programming (2nd Year 1st Sem) PDF
- University of Zambia CSC 2000 Computer Programming Past Paper PDF 2021
- VILLASAN Object-Oriented Programming PDF
- Introduction To Object Oriented Programming PDF
- Object-Oriented Programming Prelim Workbook PDF (2025)
- Unit-1 Introduction to Object Oriented Programming and Basic Concepts PDF
Summary
This lecture introduces object-oriented programming (OOP) concepts and discusses the Java programming language. It covers topics such as the differences between human and machine communication, the origins and features of Java, and fundamental programming concepts. The lecture also touches upon compilers, interpreters and programming language structures.
Full Transcript
COMP 248: OBJECT ORIENTED PROGRAMMING I Chapter 1: A First Program Why do programming? Humans communicate in a natural language – Large vocabulary (10 000s words) – Complex syntax – Semantic ambiguity The chair’s leg is broken. The man saw the boy with the telescop...
COMP 248: OBJECT ORIENTED PROGRAMMING I Chapter 1: A First Program Why do programming? Humans communicate in a natural language – Large vocabulary (10 000s words) – Complex syntax – Semantic ambiguity The chair’s leg is broken. The man saw the boy with the telescope. Machines communicate in binary code / machine language – Small vocabulary (2 words… 1, 0) – Simple syntax – No semantic ambiguity 2 So? Humans -- natural language Machines -- binary language Large vocabulary Small vocabulary Complex syntax Simple syntax Semantic ambiguity No semantic ambiguity Programming Compiler + Interpreter (COMP 248 + COMP 249) Programming language Ex: ??? Vocabulary: restricted Syntax: small and restricted Semantic: no ambiguity (almost) 3 Origins of the Java Language Created by Sun Microsystems (1991) Originally designed for programming home appliances Introduced in 1995 and its popularity has grown quickly since Is an object-oriented programming (OOP) language 4 Compilers A compiler: – is a software tool which translates source code into another language Usually (ex. C, C++) – The compiler translates directly into machine language – But each type of CPU uses a different machine language – … so same executable file will not work on different platforms – … need to re-compile the original source code on different platforms Java is different… 5 Java Translation Java compiler: Java source code --> bytecode A machine language for a fictitious computer called the Java Virtual Machine Java interpreter: Executes the Java Virtual Machine (JVM) Java bytecode --> into machine language and executes it Translating byte-code into machine code is relatively easy compared to the initial compilation step So the Java compiler is not tied to any particular machine Once compiled to byte-code, a Java program can be used on any computer, making it very portable 6 Java Translation Java source Code MyProg.java Java Compiler Machine javac MyProg.java Code Java Bytecode Java Interpreter MyProg.class java MyProg 7 Some definitions Algorithm: A step-by-step process for solving a problem Expressed in natural language Pseudocode: An algorithm expressed in a more formal language Code-like, but does not necessarily follow a specific syntax Program: An algorithm expressed in a programming language Follows a specific syntax 8 Problem Solving The purpose of writing a program is to solve a problem The general steps in problem solving are: 1. Understand the problem 2. Design a solution (find an algorithm) 3. Implement the solution (write the program) 4. Test the program and fix any problems 9 Java Program Structure A java program: is made up of one or more classes (collection of actions) a class contains one or more methods (action) a method contains program statements/instructions A Java program always contains a method called main 10 Java Program Structure // comments about the class public class MyProgram class header { class body } MyProgram.java 11 Java Program Structure // comments about the class public class MyProgram { // comments about the method public static void main (String[] args) { method header method body } } MyProgram.java 12 A small Java program / 24 2 - Identifiers are the words a programmer uses/defines in a program to name variables, classes, methods, … Rules to create an identifier: can be made up of: - letters, - digits, - the underscore character (_), - and the dollar sign ($) cannot begin with a digit Cannot contain blank space cannot be a reserved word no limit on length 25 Valid Identifier? Which of the following is not a valid identifier? A) abc B) ABC C) Abc D) aBc E) a bc 26 Valid Identifier? Which of the following is not a valid identifier? A) a_bc B) A$BC C) _Abc D) 1AbC E) $abc 27 Identifiers Guidelines: give a significant name! avoid '$‘ by convention: class names --> use title case ex : MyClass, Lincoln constants --> use upper case ex : MAXIMUM variables, methods, … --> start with lowercase ex : aVar, a_var 28 Identifiers Avoid Predefined identifiers: Although they can be redefined, it is confusing and dangerous System String println Remember: Java is case sensitive 29 Java reserved words abstract double int strictfp assert else interface super boolean extends long switch break false native synchronized byte final new this case finally null throw catch float package throws char for private transient class goto protected true const if public try continue implements return void default import short volatile do instanceof static while 30 Examples Identifier Correct or not? GST PriceBeforeTax Student_3 student#3 Shipping&HandlingFee Class __123 the account 1floor 31 3 - Indentation Spaces, blank lines, and tabs are called white space White space is used to separate words and symbols in a program Extra white space is ignored Programs should be formatted to enhance readability, using consistent indentation 32 Example 1: bad indentation //****************************************************** // Lincoln2.java // Demonstrates a poorly formatted, though valid, // Program. //****************************************************** public class Lincoln2{public static void main(String[]args){ System.out.println("A quote by Abraham Lincoln:"); System.out.println("Whatever you are, be a good one.");}} 33 Example 2: bad indentation //******************************************************************** // Lincoln3.java // Demonstrates another valid program that is poorly formatted. //******************************************************************** public class Lincoln3 { public static void main ( String [] args ) { System.out.println ( "A quote by Abraham Lincoln:" ) ; System.out.println ( "Whatever you are, be a good one.“ ) ; } } 34 Example 3: good indentation //***************************************************** // Lincoln3.java // Demonstrates a properly formatted program. //***************************************************** public class Lincoln3 { public static void main(String[] args) { System.out.println("A quote by Abraham Lincoln:"); System.out.println("Whatever you are, be a good one."); } } 35 4 - Primitive Types 8 primitive data types in Java Numeric 4 types to represent integers (ex. 3, -5): byte, short, int, long 2 types to represent floating point numbers (ex. 3.5): float, double Characters (ex. 'a') char Boolean values (true/false) boolean 36 Numerical Types The difference between: byte, short, int, long AND float, double is their size (so the values they can store) 37 Numerical Types … The difference between: byte, short, int, long AND float, double is their size (so the values they can store) 38 Pitfall: Round-Off Errors in Floating-Point Numbers Floating point numbers are only approximate quantities Mathematically, the floating-point number 1.0/3.0 is equal to 0.3333333... A computer may store 1.0/3.0 as something like 0.3333333333 39 Characters A char stores a single character delimited by single quotes: 'a' 'X' '7' '$' ',' '\n' characters are ordered according to a character set each character corresponds to a unique number code Java uses the Unicode character set 16 bits per character, so 65,536 possible characters Unicode is an international character set, containing symbols and characters from languages with different alphabets 40 Characters The ASCII character set is older and smaller than Unicode, but is still popular The ASCII characters are a subset of the Unicode character set, including: uppercase letters ’A’, ’B’, ’C’, … lowercase letters ’a’, ’b’, ’c’, … punctuation ’. ’, ’; ’, … digits ’0’, ’1’, ’2’, … special symbols ’&’, ’|’, ’\’, … control characters ’\n’, ’\t’, … 41 Booleans A boolean value represents a true or false expression The reserved words true and false are the only valid values for a boolean type NOT… 0 and 1 42 5 - Variables a name for a location in memory used to store information (ex. price, size, …) must be declared before it is used indicate the variable's name indicate the type of information it will contain declaration can be anywhere in the program (but before its first access) data type variable name int total; Multiple variables can int count, temp, result; be created in one declaration 43 Tip: Initialize Variables A variable that has been declared but has not yet been given a value is said to be uninitialized In certain cases, an uninitialized variable is given a default value It is best not to rely on this Explicitly initialized variables have the added benefit of improving program clarity 44 Initialization at declaration A variable can be given an initial value in the declaration int sum = 0; int base = 32, max = 149; When a variable is used in a program, its current value is used 45 Example – PianoKeys.java //******************************************************** // PianoKeys.java // Demonstrates the declaration and initialization of an // integer variable. //******************************************************** public class PianoKeys { // Prints the number of keys on a piano. public static void main (String[] args) { int keys = 88; System.out.println("A piano has" + keys + "keys."); } } filename?? ??? Output 46 Constants Similar to a variable but can only hold one value while the program is active the compiler will issue an error if you try to change the value of a constant during execution use the final modifier final int MIN_AGE = 18; Constants: give names to otherwise unclear literal values facilitate updates of values used throughout a program prevent inadvertent attempts to change a value 47 6 - Output System.out.print System.out.println Displays what is in parenthesis Displays what is in parenthesis Advances to the next line Examples: System.out.print("hello"); System.out.print("you"); System.out.println("hello"); System.out.println("you"); System.out.println(); int price = 50; Output System.out.print(price); char initial = 'L'; System.out.println(initial); 48 Multiple output System.out.println("hello" + "you"); double price = 9.99; int nbItems = 5; System.out.println("total = " + price*nbItems + "$"); ??? Output in print and println, + is the concatenation… you need parenthesis for the + to be addition ??? int x = 1, y = 2; System.out.println("x+y="+x+y); System.out.println("x+y="+(x+y)); Output 49 Multiple output … cannot cut a string over several lines System.out.println("this is a long string"); // error! System.out.println("this is a" + "long string"); // ok 50 Escape sequences System.out.println ("I said "Hi" to her."); ??? Output to print a double quote character Use an escape sequence - sequence is a series of characters that represents a special character - begins with a backslash character (\) - considered as 1 single character System.out.println ("I said \"Hi\" to her."); ??? Output 51 Escape sequences Some Java escape sequences: Escape Sequence Meaning \b backspace \t tab \n newline \" double quote \' single quote \\ backslash 52 You try What will the following statement output? System.out.print("one\ntwo\nthree\n"); A) one two three B) one\ntwo\nthree\n C) "one\ntwo\nthree\n" D) one two three E) onetwothree 53 You try What statement will result in the following output? Read the file "c:\windows\readme.txt" System.out.print A) ("Read the file "c:\windows\readme.txt"); B) ("Read the file "c:\windows\readme.txt""); C) ("Read the file "c:\\windows\\readme.txt"); D) ("Read the file \"c:\\windows\\readme.txt\""); E) ("Read the file \"c:\windows\readme.txt\""); 54 7. Numeric Console Input Class Libraries & Packages A class library (ex: Java standard class library) is a collection of classes that we can use when developing programs The classes of the Java standard class library are organized into packages Package Purpose java.lang General support java.text Format text for output java.awt Graphics and graphical user interfaces java.util Utilities 55 The import Declaration to use a class from a package – you can import only the class DecimalFormat from the package java.text import java.text.DecimalFormat; – or import all classes from the package java.text import java.text.*; All classes of the java.lang package are imported automatically into all programs That's why we didn't have to import the System or String classes explicitly 56 Console Input since Java 5.0, use the Scanner class the keyboard is represented by the System.in object import java.util.Scanner; Scanner myKeyboard = new Scanner(System.in); 57 To read from a scanner to read tokens, use a nextSomething() method – nextBoolean(), – nextByte, tokens are delimited by – nextInt(), whitespaces (blank spaces, tabs, and line breaks) – nextFloat(), – nextDouble(), – next(), Will see later in the chapter – nextLine() Will see later in the chapter – … Scanner myKeyboard = new Scanner(System.in); System.out.println("Your name:"); String name = myKeyboard.next(); System.out.println("Welcome " + name + " Enter your age:"); int age = myKeyboard.nextInt(); 58 Example 1: ScannerDemo1.java //***************************************************** // Author: W. Savitch (modified by N. Acemian) // // This program demonstrates how to read numeric tokens from // the console with the Scanner class //***************************************************** import java.util.Scanner; // we need to import this class public class ScannerDemo1 { public static void main(String[] args) { // let's declare our scanner Scanner keyboard = new Scanner(System.in); 59 Example 1: ScannerDemo1.java // let's ask the user for some input System.out.println("Enter the number of pods followed by"); System.out.println("the number of peas in a pod:"); // let's read the user input (2 integers that we assign to 2 // variables) int numberOfPods = keyboard.nextInt( ); int peasPerPod = keyboard.nextInt( ); int totalNumberOfPeas = numberOfPods * peasPerPod; 60 Example 1: ScannerDemo1.java // let's display some output System.out.print(numberOfPods + " pods and "); System.out.println(peasPerPod + " peas per pod."); System.out.println("The total number of peas = " + totalNumberOfPeas); // close the Scanner keyboard.close(); } // end of main() } // end of class ScannerDemo1 61 Example 2: ScannerDemo2.java //******************************************************* // Author: W. Savitch (modified by N. Acemian) // // This program demonstrates how to read various types // of token with the Scanner class //******************************************************* import java.util.Scanner; public class ScannerDemo2 { public static void main(String[] args) { // let's try to read integers int n1, n2; // let's declare 2 variables for our tests // let's declare our scanner object Scanner scannerObject = new Scanner(System.in); 62 Example 2: ScannerDemo2.java // let’s read two whole numbers (integers) System.out.println("Enter two whole numbers "); System.out.println("separated by one or more spaces:"); // we read 1 integer and assign it to n1 n1 = scannerObject.nextInt( ); // we read another integer and assign it to n2 n2 = scannerObject.nextInt( ); System.out.println("You entered " + n1 + " and " + n2); 63 Example 2: ScannerDemo2.java System.out.println("Next enter two numbers with a decimal point."); System.out.println("Decimal points are allowed."); // let's try to read doubles now double d1, d2; d1 = scannerObject.nextDouble( ); d2 = scannerObject.nextDouble( ); System.out.println("You entered " + d1 + " and " + d2); // close the Scanner scannerObject.close(); } // end of main() } // end of class ScannerDemo2 64 close() method Good habit to close a Scanner object, at the end of your program Ex: Say created a Scanner object called keyIn as follows Scanner keyIn = new Scanner(System.in); Good habit to include the following statement just before the last } for the main method keyIn.close(); Will keep Eclipse happy! 8- Assignment Used to change the value of a variable The assignment operator is the = sign total = 55; Syntax: Variable = Expression; Semantics: 1. the expression on the right is evaluated 2. the result is stored in the variable on the left (overwrite any previous value) 3. The entire assignment expression is worth the value of the RHS 66 Example public class Geometry { // Prints the number of sides of several geometric shapes. public static void main (String[] args) { int sides = 7; // declaration with initialization System.out.println("A heptagon has " + sides + " sides."); sides = 10; // assignment statement System.out.println("A decagon has " + sides + " sides."); sides = 10+2; System.out.println("A dodecagon has " + sides + " sides."); } } filename??? ??? Output 67 Difference with the math = In Java, = is an operator In math, = is an equality relation In math… a+6 = 10 ok In Java… a+6 = 10; In math… a = a+1 always false In Java… a = a+1; In math… a = b and b = a same thing! In Java… a = b and b = a; 68 Examples Declarations: int x; int y = 10; char c1 = ’a’; char c2 = ’b’; Statements: x = 20+5; y = x; c1 = ’x’; c2 = c1; 69 Swap content of 2 variables Write a series of declarations & statements to swap the value of 2 variables… int x = 10; int y = 20; A) x = y; y = x; B) y = x; x = y; C) Both A) & B) will work D) Neither A) nor B) will work 70 9 - Arithmetic Expressions An expression is a combination of one or more operands and their operators Arithmetic operators: Addition + Subtraction - Multiplication * Division / Remainder % 71 Division and Remainder … the division operator (/) can be: Integer division if both operands are integers 10 / 8 equals? 1 8 / 12 equals? 0 Real division otherwise 10.0 / 8 equals? 1.25 8 / 12.0 equals? 0.6667 72 Division and Remainder The remainder operator (%) returns the remainder after the integer division 10 % 8 equals? 2 (10÷8 = 1 remainder 2) 8 % 12 equals? 8 73 Operator Precedence Operators can be combined into complex expressions result = total + count / max - offset; Precedence determines the order of evaluation – 1st: expressions in parenthesis – 2nd: unary + and - – 3rd: multiplication, division, and remainder – 4th: addition, subtraction, and string concatenation – 5th: assignment operator 74 Operator Associativity Unary operators of equal precedence are grouped right-to-left +-+rate is evaluated as +(-(+rate)) Binary operators of equal precedence are grouped left-to-right base + rate + hours is evaluated as (base + rate) + hours Exception: A string of assignment operators is grouped right-to-left n1 = n2 = n3; is evaluated as n1 = n2 = n3; 75 Example What is the order of evaluation in the following expressions? a + b + c + d + e a + b * c - d / e a / (b + c) - d % e a / (b * (c + (d - e))) 76 Assignment Revisited The assignment operator has a lower precedence than the arithmetic operators First the expression on the RHS is evaluated answer = sum / 4 + MAX * lowest; 4 1 3 2 Then the result is stored in the variable on the LHS 77 You try What is stored in the integer variable num1 after this statement? num1 = 2 + 3 * 5 - 5 * 2 / 5 + 10 ; A) 0 B) 18 C) 25 D) 10 78 Let’s put it all together Purpose: – Conversion of degrees Fahrenheit in degrees Celsius Algorithm: 1. Assign the temperature in Fahrenheit (ex. 100 degrees) 2. Calculate the temperature in Celsius (1 Celsius = 5/9 (Fahr – 32) 3. Display temperature in Celsius Variables and constants: Data Identifier Type var or const? Temperature in Fahrenheit fahr double 79 The Java program //********************************************************** // Temperature.java Author: your name // A program to convert degrees Fahrenheit in degrees Celsius. //********************************************************** public class Temperature { public static void main (String[] args) { // Declaration of variables and constants // step 1: Assign the temperature in Fahrenheit (100) // step2: Calculate the temperature in Celsius // step3: Display temperature in Celsius } } filename??? 80 10 - More assignment operators in addition to = Often, we perform an operation on a variable, and then store the result back into that variable Java has shortcut assignment operators: variable = variable operator expression; variable operator= expression; Operator Example Equivalent To += x += y x = x + y -= x -= y x = x - y *= x *= y x = x * y /= x /= y x = x / y %= x %= y x = x % y 81 Shorthand Assignment Statements Example: Equivalent To: count += 2; count = count + 2; sum -= discount; sum = sum – discount; bonus *= 2; bonus = bonus * 2; time /= rushFactor; time = time / rushFactor; change %= 100; change = change % 100; amount *= count1 + count2; amount = amount * (count1 + count2); 82 Assignment operators The behavior of some assignment operators depends on the types of the operands ex: the += If the operands are strings, += performs string concatenation The behavior of += is consistent with the behavior of the "regular" + 83 Example int amount = 10; amount += 5; System.out.println(amount); double temp = 10; temp *= 10; System.out.println(temp); String word = "hello"; word += "bye"; System.out.println(word); word *= "bye"; // ??? Output 84 Increment and Decrement In Java, we often add-one or subtract-one to a variable… 2 shortcut operators: The increment operator (++) adds one to its operand The decrement operator (--) subtracts one from its operand The statement: count++; is functionally equivalent to: count = count+1; The statement: count--; is functionally equivalent to: count = count-1; 85 Increment and Decrement The increment and decrement operators can be in: in prefix form - ex: ++count; 1. the variable is incremented/decremented by 1 2. the value of the entire expression is the new value of the variable (after the incrementation/decrementation) in postfix form: - ex: count++; 1. the variable is incremented/decremented by 1 2. the value of the entire expression is the old value of the variable (before the incrementation/decrementation) 86 Example int nb = 50; int nb = 50; ++nb; nb++; value of nb value of nb int nb = 50; int nb = 50; int x; int x; value of nb & x value of nb & x x = ++nb; x = nb++; int nb = 50; int x; value of nb & x x = nb++ + 10; 87 Example int nb = 50; int nb = 50; int x; int x; x = ++nb + nb; x = nb++ + nb; value of nb & x value of nb & x 88 You try What is stored in the integer variables num1, num2 and num3 after the following statements? int num1 = 1, num2 = 0; int num3 = 2 * num1++ + --num2 * 5; A) num1 = 1, num2 = 0, num3 = 2 B) num1 = 1, num2 = 0, num3 = -1 C) num1 = 2, num2 = -1, num3 = 2 D) num1 = 2, num2 = -1, num3 = -3 E) num1 = 2, num2 = -1, num3 = -1 89 You try What is stored in the integer q after the following statements? int x = 1, y = 10, z = 3; int q = ++x * y-- + z++; A) 13 B) 20 C) 23 D) No idea??? 90 You try What is stored in the integers a and c after the following statements? int a = 1; int c = a++ + a--; A) a = 1, c = 2 B) a = 1, c = 3 C) a = 3, c = 3 D) No idea??? 91 You try What is stored in the integer c after the following statements? int a = 1, b = 2; int c = a++ + a + 2*(-- b) + 3/b--; A) 7 B) 8 C) 8.5 D) No idea??? 92 Summary of ++ and - - Expression Operation Value Used in Expression count++ add 1 old value ++count add 1 new value count-- subtract 1 old value --count subtract 1 new value 93 11 - Assignment Compatibility In general, the value of one type cannot be stored in a variable of another type int intVariable = 2.99; //Illegal However, there are exceptions to this double doubleVariable = 2; For example, an int value can be stored in a double type 94 Assignment Compatibility an expression has a value and a type 2 / 4 (value = 0, type = int) 2 / 4.0 (value = 0.5, type = double) the type of the expression depends on the type of its operands In Java, type conversions can occur in 3 ways: arithmetic promotion assignment conversion casting 95 Arithmetic promotion happens automatically, if the operands of an expression are of different types aLong + anInt * aDouble operands are promoted so that they have the same type promotion rules: if 1 operand is of type… the others are promoted to… double double float float (double) long long short, byte and char are always converted to int 96 Examples (aByte + anotherByte) --> int (aLong + anInt * aDouble) --> ?? (aFloat - aBoolean) --> ?? value and type of these expressions? 2 / 4 int / int 2/4 int 0 97 Examples What is the value and type of this expression? 2 / 4 * 1.0 A) 0 (int) B) 0.0 (double) C) 0.5 (int) D) 0.5 (double) 98 Examples What is the value and type of this expression? 1.0 * 2 / 4 A) 0 (int) B) 0.0 (double) C) 0.5 (int) D) 0.5 (double) 99 Assignment conversions occurs when an expression of one type is assigned to a variable of another type var = expression; widening conversion if the variable has a wider type than the expression then, the expression is widened automatically long aVar; byte aByte; double aDouble; aVar = int anInt; int anInt = 10; 5+5; anInt = aByte; aDouble = anInt; integral & floating point types are compatible boolean are not compatible with any type 100 Assignment conversions narrowing conversion if the variable has a smaller type than the expression, then compilation error, because possible loss of information int aVar; aVar = 3.7; ok? int aVar; int aVar; aVar = 10/4; ok? aVar = 10.0/4; ok? 101 Casting the programmer can explicitly force a type conversion syntax: (desired_type) expression_to_convert int aVar; byte aByte; aVar = (int)3.7; int anInt = 75; (aVar is 3… not 4!) aByte = anInt; // ok? aByte = (byte)anInt; // ok? double d; d = 2/4; // d is 0 d = (double)2/4; // d is 0.5 // 2.0 / 4 d = (double)(2/4); // d is 0.0 Casting can be dangerous! you better know what you're doing… 102 Examples Which of the following assignment statements are valid? byte b1 = 1, b2 = 127, b3; b3 = b1 + b2; // statement a) b3 = 1 + b2 // statement b) b3 = (byte)1 + b2 // statement c) A) Statements a), b) and c) are valid B) Only statements a) and b) are valid C) Only statements b) and c) are valid D) Only statements a) and c) are valid E) None of the Java statements is valid 103 Examples Which of the following assignment statements are valid? byte b1 = 1, b2 = 127, b3; b3 = b1 + b2; // statement a) b3 = 1 + b2; // statement b) b3 = (byte)1 + b2; // statement c) A) Statements a), b) and c) are valid B) Only statements a) and b) are valid C) Only statements b) and c) are valid D) Only statements a) and c) are valid E) None of the Java statements is valid 104 12- Strings so far, we have seen only primitive types a variable can be either: a primitive type - ex: int, float, boolean, … or a reference to an object - ex: String, Array, … A character string: is an object defined by the String class delimited by double quotation marks ex: "hello", "a" - System.out.print("hello"); // string of characters - System.out.print('a'); // single character 105 Declaring Strings 1. declare a reference to a String object String title; 2. declare the object itself (the String itself) title = new String("content of the string"); This calls the String constructor, which is a special method that sets up the object 106 Declaring Strings Because strings are so common, we don't have to use the new operator to create a String object String title; title = new String("content of the string"); String title = new String("content of the string"); String title; title = "content of the string"; String title = "content of the string"; These special syntax works only for strings 107 Strings once a string is created, its value cannot be modified (the object is immutable) cannot lengthen/shorten it cannot modify its content the String class offers: the + operator (string concatenation) - ex: String solution = "The answer is " + "yes"; many methods to manipulate strings, a string variable calls … - length() // returns the nb of characters in a string - concat(str) // returns the concatenation of the string and str - toUpperCase() // returns the string all in uppercase - replace(oldChar, newChar) // returns a new string // where all occurrences of character oldChar have been replaced by character newChar - … see textbook 108 String indexes start at zero 109 Example – StringTest.java public class StringTest { public static void main (String[] args) { String string1 = new String ("This is a string"); String string2 = ""; ??? String string3, string4, string5; Output System.out.println("Content of string1: \"" + string1 + "\""); System.out.println("Length of string1: " + string1.length()); System.out.println("Content of string2: \"" + string2 + "\""); System.out.println("Length of string2: " + string2.length()); 110 Example … // String string1 = new String ("This is a string"); // String string2 = ""; string2 = string1.concat(" hello"); string3 = string2.toUpperCase(); string4 = string3.replace('E', 'X'); string5 = string4.substring(3, 10); System.out.println(string2); Output ??? System.out.println(string3); System.out.println(string4); System.out.println(string5); } } 111 Example 3: ScannerDemo3.java //************************************************************ // Author: W. Savitch (modified by N. Acemian) // // This program demonstrates how to read String tokens with // the Scanner class //************************************************************ import java.util.Scanner; public class ScannerDemo3 { public static void main(String[] args) { //Let's declare our Scanner object Scanner scannerObject = new Scanner(System.in); // let's try to read 2 "words" now System.out.println("Next enter two words:"); String word1 = scannerObject.next( ); String word2 = scannerObject.next( ); System.out.println("You entered \"" + word1 + "\" and \"" + word2 + "\""); 112 Example 3: ScannerDemo3.java //To get rid of '\n‘ String junk = scannerObject.nextLine( ); // let's try to read an entire line System.out.println("Next enter a line of text:"); String line = scannerObject.nextLine( ); System.out.println("You entered: \"" + line + "\""); // Close Scanner scannerObject.close(); } // end of main() }// end of class ScannerDemo3 113 A note on nextLine nextLine reads the remainder of a line of text starting where the last reading left off This can cause problems when combining it with different methods for reading from the keyboard such as nextInt ex: Scanner keyboard = new Scanner(System.in); int n = keyboard.nextInt(); String s1 = keyboard.nextLine(); String s2 = keyboard.nextLine(); input: need an extra invocation 2 of nextLine to get rid of Heads are better than the end of line character 1 head. after the 2 what are the values of n, s1, and s2? 114 close() method Good habit to close a Scanner object, at the end of your program Ex: Say created a Scanner object called keyIn as follows Scanner keyIn = new Scanner(System.in); Good habit to include the following statement just before the last } for the main method keyIn.close();