JS Data Structures, Modern Operators and Strings - PDF
Document Details
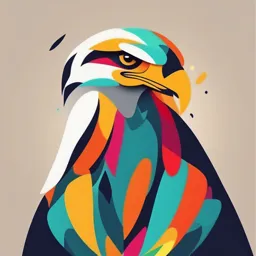
Uploaded by VirtuousSnowflakeObsidian
Tags
Summary
This document is a set of JavaScript notes on data structures, modern operators, and strings. It includes examples, explanations, and exercises for the topics.
Full Transcript
js Data Structures, Modern Operators and Strings Date @02/05/2025 Status To Review Subject 👾 js jonas chapter 9 array destructing: the EX6 feature...
js Data Structures, Modern Operators and Strings Date @02/05/2025 Status To Review Subject 👾 js jonas chapter 9 array destructing: the EX6 feature const arr = [1,2,3]; const [x,y,z]=arr; // Skip the second element const [a, , c] = arr; default values const numbers = ; // Provide default values const [a = 10, b = 20, c = 30] = numbers; const numbers = [1, 2, 3, 4, 5]; // Capture the first two elements and the rest const [a, b,...rest] = numbers; console.log(a); // 1 console.log(b); // 2 console.log(rest); // [3, 4, 5] js Data Structures, Modern Operators and Strings 1 for swapping 🍕(short answer!): let a = 1; let b = 2; // Swap values [a, b] = [b, a]; Returning Multiple Values from a Function function getMultipleValues() { return [10, 20, 30]; } // Destructure the returned array const [a, b, c] = getMultipleValues(); nested arrays const nested = [1, [2, 3], 4]; // Destructure nested array const [a, [b, c], d] = nested; destructing object swapping or mutating data // Mutating variables let a = 111; let b = 999; const obj = { a: 23, b: 7, c: 14 }; js Data Structures, Modern Operators and Strings 2 ({ a, b } = obj); //the () is nessesary unless it doesnt work console.log(a, b); const serie={ name:'merlin', type:'bromance', ship:['merlin','arthur'], year: [2008,2012], seasons:{ 1:{ episode:13, realesed:{First:'20 September 2008 ' ,Last:'13 December 2008'} }, 2:{ episode:13, realesed:{First:'19 September 2009 ' ,Last:'19 December 2009'} }, 3:{ episode:13, realesed:{First:'11 September 2010 ' ,Last:'4 December 2010'} }, 4:{ episode:13, realesed:{First:'1 October 2011 ' ,Last:'24 December 2011'} }, 5:{ episode:13, realesed:{First:'6 October 2012 ' ,Last:'24 December 2012 '} }, }, }; const getInfo =function({name:serieName='the best serie i know',type,ship:[fistS console.log(`it is ${serieName}.it has a ${type} story.the main copel are ${fist js Data Structures, Modern Operators and Strings 3 } getInfo(serie); spread operator: for passing separated data of array into the function 🍕(short answer!): const orders = ['salad','kebab','cola']; const restaurantOrder= function (a,b,c){ console.log(`here are your ${a} and ${b} with ${c} `); } restaurantOrder(...orders); const otherOrder = ['donat','pizza','abali']; const copyOfOtherOrder =[...otherOrder]; console.log(copyOfOtherOrder); const allorders= [...otherOrder ,...orders]; console.log(allorders); console.log(...allorders); const [ ,myFood, , , yourFood] = allorders; console.log(myFood,yourFood); const restaurant={ foundIn:'1998', founder:'poo', foods:[...copyOfOtherOrder], }; const copyRestaurant= {...restaurant}; copyRestaurant.founder='Fatherpoo'; console.log(restaurant); console.log(copyRestaurant); js Data Structures, Modern Operators and Strings 4 const name='harry'; const family= 'potter'; const letters =[...name, ,...family]; console.log(letters); for shallow copy of objects : iterables :arrays ,strings ,maps, sets.but not objects 🍩the spread operator(…) is work on all iterables. 🍪also work on object(the objects are not iterables) only use when functionName(…str) or build a new array const newArr=[…str,…sld]; the differents between rest pattern and the spread operation : spread:unpack the array rest:pack into array rest pattern and parameters : must be the last element const HP =['harry','hermion','ron',...['dambeldor','Snape','mackgolagan']]; console.log(HP); const [name,...rest] = HP; console.log(name,rest); const serie={ name:'merlin', type:'bromance', ship:['merlin','arthur'], year: [2008,2012], seasons:{ js Data Structures, Modern Operators and Strings 5 1:{ episode:13, realesed:{First:'20 September 2008 ' ,Last:'13 December 2008'} }, 2:{ episode:13, realesed:{First:'19 September 2009 ' ,Last:'19 December 2009'} }, 3:{ episode:13, realesed:{First:'11 September 2010 ' ,Last:'4 December 2010'} }, 4:{ episode:13, realesed:{First:'1 October 2011 ' ,Last:'24 December 2011'} }, 5:{ episode:13, realesed:{First:'6 October 2012 ' ,Last:'24 December 2012 '} }, }, }; const {name,type ,...rest}= serie; console.log(name,type,rest); rest parameter for the function: const shopingList = function(...concepts){ console.log(concepts); } shopingList('eggs','tomato','butter'); shopingList('noodels','chilly','sussages','parmejan','cola','ice'); js Data Structures, Modern Operators and Strings 6 const x=['gasming tea','poison '] shopingList(...x); operators : use any data type , return any data, short circuiting //return first truthy value console.log(2||'loise') //2 console.log('loise'||2) //loise console.log(0 || null||'hana') //hana console.log(0 || null);//null //return first falsy value console.log(0||'sherlock'); //0 console.log('loise'&& 2&&'hana')//hana we can change the if else statements to the statements with AND OR Nullish coalescing operator: //return first nullish value:null , undefined(NOT 0 '') const aurthorLovetoMerline =0; const loveNum = aurthorLovetoMerline ?? 100; console.log(loveNum); //0 logical assignment operators: const character1={ name:'Gandalf', // age:55000, job:'wizard', js Data Structures, Modern Operators and Strings 7 }; const character2={ name:'Frodo', age:33, // job:'ring keeper', }; //if the age : 0 it dont work character1.age||=55000; character2.age||=55000; console.log(character1.age); console.log(character2.age); character1.age??=55000; character2.age??=55000; console.log(character1.age); console.log(character2.age); for of loop continue and break ✅ index ❌ const arr= ['Newt','Tina','Jacob Kowalski','Queenie'] for(const item of arr) {console.log(item)} if we want the index?? for(const item of arr.entries()) {console.log(item)} //[0, 'Newt']] //(2) [1, 'Tina'] 0: 1,1: "Tina"length: 2[[Prototype]]: Array(0) js Data Structures, Modern Operators and Strings 8 //[2, 'Jacob Kowalski'] //[3, 'Queenie'] for(const [i,el] of arr.entries()) {console.log(`index ${i} has the value ${el}`)}; enhanced object literals const seasonNum =[1,2,3,4,5]; const seasons={ //compute property names [seasonNum]:{ episode:13, realesed:{First:'20 September 2008 ' ,Last:'13 December 2008'} }, [seasonNum]:{ episode:13, realesed:{First:'19 September 2009 ' ,Last:'19 December 2009'} }, [`the day${5-2}`]:{ episode:13, realesed:{First:'11 September 2010 ' ,Last:'4 December 2010'} }, 4:{ episode:13, realesed:{First:'1 October 2011 ' ,Last:'24 December 2011'} }, 5:{ episode:13, realesed:{First:'6 October 2012 ' ,Last:'24 December 2012 '} }, }; const serie={ js Data Structures, Modern Operators and Strings 9 name:'merlin', type:'bromance', ship:['merlin','arthur'], year: [2008,2012], //old way:seasons:seasons, //ES6 seasons, //ES6 getInfo(){ console.log(`it is ${this.name}.it has a ${this.type} story.the main copel are ${ } }; serie.getInfo(); optional chaining: const seasonNum =[1,2,3,4,5]; const seasons={ //compute property names [seasonNum]:{ episode:13, realesed:{First:'20 September 2008 ' ,Last:'13 December 2008'} }, [seasonNum]:{ episode:13, realesed:{First:'19 September 2009 ' ,Last:'19 December 2009'} }, [`the day${5-2}`]:{ episode:13, realesed:{First:'11 September 2010 ' ,Last:'4 December 2010'} }, 4:{ episode:13, realesed:{First:'1 October 2011 ' ,Last:'24 December 2011'} js Data Structures, Modern Operators and Strings 10 }, 5:{ episode:13, realesed:{First:'6 October 2012 ' ,Last:'24 December 2012 '} }, }; const serie={ name:'merlin', type:'bromance', ship:['merlin','arthur'], year: [2008,2012], //old way:seasons:seasons, //ES6 seasons, //ES6 getInfo(){ console.log(`it is ${this.name}.it has a ${this.type} story.the main copel are ${ } }; //dont want to get error? //chaining(if they are not null or undefind) console.log(serie.seasons['6']?.realesed.First); serie.getInfo?.(); serie.risoto?.(10,12)??console.log('the method does not exist'); //array const movies =[{director:'xavier dolan',age:22},{top1:'I Killed My Mother',top2:'m console.log(movies.tope??'there is no top1 movie'); looping objects ,object keys ,values ,entries: js Data Structures, Modern Operators and Strings 11 You can easily loop through iterables with the for...of loop(object are not iterables) looping over objects(property names,..): const yourBuletJornal ={texture:{size:10,colore:'lightGreen'},margins:{size:'25cm for(const k of Object.keys(yourBuletJornal)){ console.log(k) } //texture //margins //Binding const keyArr = Object.keys(yourBuletJornal);//keyArr:['','',''] const valuesArr = Object.values(yourBuletJornal);//valuesArr:[{},{},{}] const keyValues = Object.entries(yourBuletJornal);//keyValues:[{key,{value}},{ke an example: const openinghours = { sat:{ open:'10 am', close:'10 pm' }, sun:{open:'11 am', close:'9 pm'}, mon:{open:'6 am', close:'12 pm',shift:'harry'} }; // for(const day in Object.keys(openinghours)){ // console.log(day); // } js Data Structures, Modern Operators and Strings 12 const days = Object.keys(openinghours); console.log(days); const values =Object.values(Object.keys(Object.values(openinghours))) ; console.log(values); const enteries = Object.entries(openinghours); //day[key values] for(const [day,{open,close}] of enteries){ console.log(day,open,close); } sets : (pass the iterable) different from array:1)unique values 2)no order ❌(you cant use setName[0 ]) const singers = new Set(['NF','lady gaga','NF','eminume','NF','lady gaga','lady gag console.log(singers); singers.add('aslani'); singers.delete('eminumde');//dont get errore singers.delete('eminume'); console.log(singers); console.log(singers.size()); console.log(singers.has('NF')); // console.log(singers.clear());//delete all for(const singer of singers){ console.log(`i like the ${singer}`); } 🍪 // convert set→arr const topSingers=[...singers]; console.log(topSingers); js Data Structures, Modern Operators and Strings 13 //for finding different size dont creat new set console.log(new Set('Pneumonoultramicroscopicsilicovolcanoconiosis').size); maps: (map values to keys) the value and key have any type(difference to object) first way of creating map: const journey=new Map(); journey.set('paris','24 journey 2020'); //chaine(the set method return the map) journey.set('iran golestan',1991).set(true,'you didnt born').set(false,'you born'); console.log(journey); const year = 1999; console.log(journey.get(journey.get('iran golestan')>year)); console.log(journey.has('paris')); journey.delete('paris'); //getting the object or arrays defined out why? they are not the same place on he const objectOfJourney ={friend:['harry','louice','hemainii','ron','snape']}; journey.set(objectOfJourney,5); console.log(journey.get(objectOfJourney)); console.log(journey); //.clear() and.size dont need () ❌ second way of creating map: (similar to objcet.enteries(objectName) [ [ ],[ ],[ ],… ] 🍪 // convert object→map const chillMap =new Map( Object.enteries(chillObject)); // 🍪convert map→array js Data Structures, Modern Operators and Strings 14 const arrayQuize= [...quize]; console.log(arrayQuize); //they give the map operator but can convert to array(with [...name] console.log(quize.keys()); console.log(quize.values()); console.log(quize.entries()); const quize =new Map([ ['question','Where do Hobbits live?'], [1,'Tomato Town'], [2,'The Shire'], [3,'The Lake District'], [4,'Mordor'], ['correct',3 ], [true,'you get the point 😊'], [false,'not correct '] ☹️ ]); console.log(quize.get('question')); for(const [key,val] of quize){ if(typeof key === 'number') console.log(`${key}) ${val}`); } 🐱 const guess =Number(prompt("enter the number! ")) ; console.log(quize.get(guess===quize.get('correct'))); which data structure?? data from api in jason format what is the boxing prosses in the js and strings?? When you access a property or method on a primitive (like str.length or ), JavaScript temporarily converts it into an object using the str.toUpperCase() corresponding wrapper class ( String , Number , Boolean ). js Data Structures, Modern Operators and Strings 15 The method is executed on the temporary object. The object disappears, and the original primitive remains. strings: const airline = 'Mahan air Mamani'; const plain = 'AXB124'; console.log(airline); console.log('nanami'); console.log(airline.length); console.log(airline.indexOf('M')); console.log(airline.lastIndexOf('a')); console.log(airline.indexOf('Mamani')); console.log(airline.slice(7)); ❌ console.log(airline.slice(7,13));//dont include index 13 console.log(airline.slice(-1)); const checkSeat = function(seat){ console.log(seat[seat.length-1]==='B'||seat[seat.length-1]==='E'?'yeh you are } checkSeat('11B'); checkSeat('23C'); checkSeat('3E'); const namePassanger = 'nArGeS'; namepassanger = namePassanger.toLowerCase(); namepassanger=namepassanger.toUpperCase()+namepassanger.slice(1) console.log(namepassanger); there is another function trim() for deleting whit space around \n \t //trim js Data Structures, Modern Operators and Strings 16 // Comparing emails const email = '[email protected]'; const loginEmail = ' [email protected] \n'; // const lowerEmail = loginEmail.toLowerCase(); // const trimmedEmail = lowerEmail.trim(); const normalizedEmail = loginEmail.toLowerCase().trim(); console.log(normalizedEmail); console.log(email === normalizedEmail); // replacing const priceGB = '288,97£'; const priceUS = priceGB.replace('£', '$').replace(',', '.'); console.log(priceUS); const announcement = 'All passengers come to boarding door 23. Boarding door 23!'; console.log(announcement.replace('door', 'gate')); console.log(announcement.replaceAll('door', 'gate')); // Alternative solution to replaceAll with regular expression console.log(announcement.replace(/door/g, 'gate')); // Booleans const plane = 'Airbus A320neo'; console.log(plane.includes('A320')); console.log(plane.includes('Boeing')); console.log(plane.startsWith('Airb')); if (plane.startsWith('Airbus') && plane.endsWith('neo')) { console.log('Part of the NEW ARirbus family'); } // Practice exercise const checkBaggage = function (items) { js Data Structures, Modern Operators and Strings 17 const baggage = items.toLowerCase(); if (baggage.includes('knife') || baggage.includes('gun')) { console.log('You are NOT allowed on board'); } else { console.log('Welcome aboard!'); } }; checkBaggage('I have a laptop, some Food and a pocket Knife'); checkBaggage('Socks and camera'); checkBaggage('Got some snacks and a gun for protection'); split and join: let checkList = '1 wand+1 cauldron+1 set of glass+1 telescope+1 set of brass sca checkList =checkList.split('+'); console.log(['dear harry this is your checkList',...checkList].join(',')); //dear harry this is your checkList:,1 wand,1 cauldron,1 set of glass,1 telescope,1 se //padding a string const names=['Hermione Granger','Harry Potter','Minerva McGonagall','Snape']; for(const item of names){ console.log(item.padStart(30,'< ').padEnd(90,' >')); } //its not the actual output //< < < < < < < Hermione Granger //< < < < < < < < < Harry Potter //< < < < < < Minerva McGonagall //< < < < < < < < < < < < string String(num) or num+'' const maskCraditCard = function(num){ const strOfNum = num +''; const result =strOfNum.slice(-4); console.log(result.padStart(strOfNum.length-4,'*')); } maskCraditCard(6037804599342059); maskCraditCard('6037804599342435343435454434134333059'); //repeat const text = 'Will the real Slim Shady please stand up?\t'; console.log(text.repeat(5)); quiz 1: 17. What will this output? //index, value const arr = ["a", "b", "c"]; for (const [i, val] of arr.entries()) { console.log(i, val); } //0 a //1 b //2 c 18. What will console.log([...new Set("hello")]) print? console.log([...new Set("hello")]); //["h", "e", "l", "o"] Explanation: js Data Structures, Modern Operators and Strings 19 The Set object removes duplicate values because it only keeps unique elements. The string "hello" is treated as an iterable, meaning each character is processed separately. new Set("hello") creates a set with unique characters: {'h', 'e', 'l', 'o'}. The spread operator ( [...] ) converts this set back into an array. js Data Structures, Modern Operators and Strings 20