JavaScript Basics PDF
Document Details
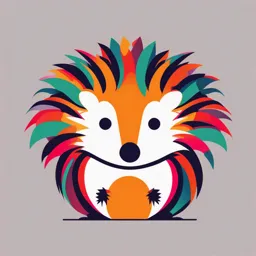
Uploaded by AdvantageousPurple8633
Tunis Business School
Tags
Summary
This document is a JavaScript tutorial. It covers basic concepts such as variables, data types, operators, conditional statements, and loops, showcasing examples in the context of web development. It includes introductions to JavaScript and useful resources such as the MDN JavaScript reference.
Full Transcript
JavaScript Basics CS200: Web Development Tunis Business School - 2024/2025 Outline Introduction Variables & Operators Dialog boxes to JavaScript data types Conditional and Loop Functions Arrays Objects Statements Introduction to JavaScript...
JavaScript Basics CS200: Web Development Tunis Business School - 2024/2025 Outline Introduction Variables & Operators Dialog boxes to JavaScript data types Conditional and Loop Functions Arrays Objects Statements Introduction to JavaScript What is JavaScript? Founded in 1995 by Brendan Eich What is JavaScript? Interpreted Language: "Bringing web pages Programs are plain text scripts to life" No compilation phase Executed on loading What is JavaScript? Name? LiveScript JavaScript What is JavaScript? It can run in: The Navigator The server(Node.JS) JavaScript engine: V8 (Chrome & Opera) SpiderMonkey (Firefox) How JavaScript engines work The Basics of the JS Engine 1. It reads the script 2. It compiles the script into machine language 3. The machine executes the code quickly What JavaScript can do in the browser The browser is a safe Fields of action: environment Manipulating Web Pages No direct access to User Interactions CPU, memory or HDD Web Server Interactions What JavaScript can do in the browser Add HTML, edit content, change style React to actions, clicks, gestures, key presses Send network requests, download, upload files Store client-side data (local storage) What can't JavaScript do in the browser? Access Disk (Read/Write) Recover files without user consent Interact with the camera and microphone without permission Interact with other tabs/domains Why is JavaScript unique? Full integration with HTML/CSS Frontend Backend Supported and enabled by default by all major browsers Cross-platform Mobile Apps Main tool for creating UIs applications Manuals & Specifications Specification The ECMA-262 specification The most detailed and formalized The ECMA-262 specification The ECMA-262 specification information about JavaScript. It defines language. https://tc39.es/ecma262/ https://tc39.es/ecma262/ A new version of the specification is published every year. Manuals MDN JavaScript reference MDN JavaScript reference Compatibility Charts Support tables by feature, e.g. to see which http://caniuse.com engines support modern cryptography http://caniuse.com functions: http://caniuse.com/#feat=cryptography. https://kangax.github.io/compat-table https://kangax.github.io/co A table with language features and engines that https://kangax.github.io/compat-table mpat-table support or don't support them. The Developer Console Browsers have built-in "developer tools". Most other browsers use F12/Ctrl+Shift+I to open the developer tools. Getting Started with JavaScript How to Insert JavaScript? Internal javascript: Inside the HTML page // JavaScript code here can be placed in the , or in the section of an HTML page, or in both. How to Insert JavaScript? External javascript: Outside the HTML page in a separate javascript file (.js) Practical when the same code is used in many different web pages Javascript statements JavaScript statements are composed of: let greeting = 'Hello'; const pi = 3.14; Values, Operators, var count = 10; Expressions, Keywords, and Comments Ending Statements With a Semicolon? Ending statements with semicolon is not required(optional), but highly recommended. Semicolons are required if you want to put more than one statement on a single line. JS Variables JS Variables Variables are used to store data. A variable is a "container" for information you want to store. The value of a variable can change during the script. You can refer to a variable by its identifier (name) to see its value or to change it. Rules for naming conventions Valid Characters: Variable names can contain letters (A-Z or a-z), digits (0-9), underscores (), or dollar signs ($). The name must begin with a letter, an underscore (), or a dollar sign ($). They cannot start with a digit. Rules for naming conventions Case Sensitivity: JavaScript is case-sensitive. myVariable, MyVariable, and myvariable are considered different variables.. Rules for naming conventions Reserved Keywords: Avoid using reserved keywords (like let, const, var, if, for, function, etc.) as variable names. These words have predefined meanings in JavaScript and cannot be used as identifiers for variables. Rules for naming conventions Meaningful Names: Use descriptive and meaningful names for variables to enhance code readability and maintainability. For instance, instead of x or temp, consider using names that describe the purpose of the variable, like userName, counter, totalAmount, etc. Rules for naming conventions Camel Case: The convention for naming variables in JavaScript is camelCase. This means that multi-word variable names should start with a lowercase letter, and each subsequent word should begin with a capital letter without any spaces or punctuation. For example: myVariableName totalAmount firstName numberOfStudents Valid/invalid variable names let userName; let 1stVariable; let totalAmount; // Cannot start with a digit let numberOfStudents; let my Variable; let _myVariable; // Contains a space let $specialVar; let let; // Reserved keyword let total-amount; // Contains a hyphen (not allowed) Variable declaration let const var var: « old school » Variables declared with var are function-scoped or Function Scoped globally scoped if declared outside any function. Variables declared with var are hoisted to the top of Hoisting their scope. This means that they are processed before the code execution begins. Reassignment Variables declared with var can be redeclared and reassigned. and Redefinition var: « old school » var x = 10; var x = 20; // Allowed - Variable redeclaration function example() { var y = 30; } console.log(y); // Throws an error - y is not accessible outside the function let Variables declared with let are block-scoped to the Block Scoped nearest enclosing curly braces {}. let variables are hoisted but not initialized. Accessing a Hoisting let variable before its declaration results in a ReferenceError. let variables can be reassigned but cannot be Reassignment redeclared in the same scope. let let a = 10; a = 20; // Allowed - Variable reassigned let b = 30; let b = 40; // Not allowed - Variable redeclaration in the same scope const Block Scoped: const also follows block scoping like let. Immutable Variables declared with const cannot be Binding: reassigned once they are initialized. Must be A const variable must be initialized Initialized: during declaration. const const pi = 3.14; pi = 3.14159; // Not allowed - Trying to reassign a const variable const greeting; // Throws an error - Const variables must be initialized during declaration Variable declaration Use var for traditional variable declarations. However, due to hoisting and scoping issues, it's often recommended to use let or const. Use let when variable reassignment is expected or needed within the same block scope. Use const when the value of the variable should not be reassigned and remains constant throughout the code. It's commonly used for constants or values that shouldn't change. Variable declaration Good practice: use const by default and only use let if variable reassignment is necessary. This promotes code readability and helps prevent unintentional reassignments or unexpected behavior. JS Data types Primitive data types Number Represents numeric values. String Represents textual data. Boolean Represents true or false values. Undefined Represents a variable that has been declared but not assigned a value. Null Represents the absence of any value. Composite Data Types Represents a collection of key-value Object pairs. Represents a list-like collection of Array elements. Function Represents reusable blocks of code. let num = 10; let calculatedValue = num * 5; let text = 'Hello, World!'; let greeting = text + ' Welcome!'; let isTrue = true; let isValid = false; let variable; console.log(variable); // Output: undefined let noValue = null; let person = { name: 'John', age: 30 }; console.log(person.name); // Output: John let numbers = [1, 2, 3, 4, 5]; console.log(numbers.length); // Output: 5 function greet(name) { return `Hello, ${name}!`; } console.log(greet('Alice')); // Output: Hello, Alice! JS Operators Operator Description Example Result x=2 + Addition y=2 4 x+y x=5 - Subtraction y=2 3 Aritmethic x-y x=5 operators * Multiplication y=4 x*y 20 15/5 3 / Division 5/2 2,5 5%2 1 % Modulus (division remainder) 10%8 2 10%2 0 x=5 ++ Increment x++ x=6 x=5 -- Decrement x-- x=4 Assignement Operators Operator Example Equivalent = x=y x=y += x+=y x=x+y -= x-=y x=x-y *= x*=y x=x*y /= x/=y x=x/y %= x%=y x=x%y Comparison Operators Operator Description Example == is equal to 5==8 returns false x=5 y="5" is equal to (checks for both value === and type) x==y returns true x===y returns false != is not equal 5!=8 returns true > is greater than 5>8 returns false < is less than 5= is greater than or equal to 5>=8 returns false 0) { of code if a console.log('Number is positive.'); specified } condition is true. If-Else Statement let num = -5; Executes one if (num > 0) { console.log('Number is block of code if a positive.'); condition is true } else { console.log('Number is and another non-positive.'); } block if it's false. Else-If Statement let num3 = 0; Allows if (num3 > 0) { console.log('Number is positive.'); multiple } else if (num3 < 0) { console.log('Number is negative.'); conditions to } else { console.log('Number is zero.'); be checked. } Switch Statement let day = 3; Evaluates an switch (day) { case 1: expression and console.log('Monday'); break; case 2: executes the console.log('Tuesday'); break; corresponding default: console.log('Unknown day'); case. } Loop Statements Repeats a block of code until a condition is met. Loop statements for iterating through arrays, handling repetitive tasks, etc. Types: for, while, do-while For Loop Executes a block of code a for (let i = 0; i < 5; i++) { console.log('Iteration', i); specified } number of times. While Loop let count = 0; Executes a block while (count < 3) { of code while a console.log('Count:', count); specified count++; } condition is true. Do-While Loop let x = 0; Executes a block do { of code at least console.log('Value of x:', x); once and x++; } while (x < 3); repeats while a condition is true. JavaScript Functions JavaScript Functions: Essential building blocks for reusable code. Used for performing specific tasks and calculations. Creating Functions function functionName(parameters) function greet(name) { { return `Hello, // Function body ${name}!`; // Code block to } execute return result; } Calling Functions Invoking a let message = greet(‘TBS'); console.log(message); function to // Output: Hello, TBS! execute its code block. Function Parameters and Arguments: function multiply(a, b) { Parameters: Variables return a * b; listed in a function's } declaration. let result = multiply(5, 3); // Arguments: 5 and 3 Arguments: Values console.log(result); // passed to a function Output: 15 when calling it. Return Statement: Returns a value from a function. Stops the execution of the function. JS Arrays JavaScript Arrays: Data structure used to store multiple values in a single variable. Indexed collections of elements. Creating Arrays Syntax: let arrayName = [value1, value2, value3]; Example: let fruits = ['Apple', 'Banana', 'Orange', 'Mango']; Accessing Array Elements: Elements are accessed using let fruit = fruits; // Accesses 'Banana' zero-based indexing. Array Methods - push() and pop() push(): pop(): fruits.push('Grapes’); Adds an Removes // Adds 'Grapes' element the last fruits.pop(); to the element // Removes 'Grapes' end of from the the array. array. Array Methods - shift() and unshift(): shift(): unshift(): fruits.shift(); Removes Adds an // Removes 'Apple' the first element fruits.unshift('Pineapple ’); element to the from the beginning // Adds 'Pineapple' array. of the array. Array Methods - slice() and splice(): let selectedFruits = slice(): splice(): fruits.slice(1, 3); // Returns ['Banana', Extracts a Adds or 'Orange'] section of removes fruits.splice(2, 0, an array elements 'Kiwi’); and from an // Inserts 'Kiwi' at returns a array. index 2 new array. Iterating Through Arrays - For Loop: Looping through elements using a for loop and array length. for (let i = 0; i < fruits.length; i++) { console.log(fruits[i]); } Iterating Through Arrays - forEach Method: Using forEach() method for concise array iteration. fruits.forEach(function (fruit) { console.log(fruit); }); JS Objects JavaScript Objects: Key-value pairs used to store various data types. Can hold properties and methods. Creating Objects: Syntax: Example: let objectName = { let person = { key1: value1, name: 'John', key2: value2, age: 30, //... occupation: }; 'Developer' }; Accessing Object Properties: Dot notation and bracket notation used to access properties. let personName = person.name; // Dot notation let personAge = person['age']; // Bracket notation Adding and Modifying Properties: Properties can be added or modified dynamically. person.location = 'New York'; // Adding a property person.age = 31; // Modifying a property Removing Properties: delete keyword used to remove properties. delete person.occupation; // Removes 'occupation' property Object Methods: Functions inside objects are known as methods. let person = { name: 'John', greet: function () { return `Hello, my name is ${this.name}.`; } }; console.log(person.greet()); // Output: Hello, my name is John. Object Iteration - for...in Loop: Looping through object properties using for...in loop. for (let key in person) { console.log(`${key}: ${person[key]}`); }