Javascript Programming Language PDF
Document Details
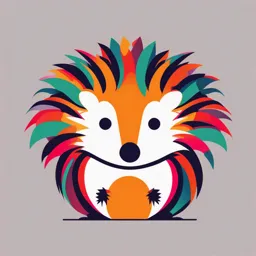
Uploaded by PurposefulPenguin9878
David Nii Armah Aryee
Tags
Summary
A JavaScript programming presentation. This presentation covers the introduction, variables, functions, operators, and control flow topics in JavaScript. It also includes simple examples and activities for learning JavaScript.
Full Transcript
JavaScript Programming language Presentation By David Nii Armah Aryee Table of contents 01 02 03 Introduction Problem & Variables & solution Datatypes 04 05 06 Operators& Functions Modern Control Flows...
JavaScript Programming language Presentation By David Nii Armah Aryee Table of contents 01 02 03 Introduction Problem & Variables & solution Datatypes 04 05 06 Operators& Functions Modern Control Flows JavaScript(ES6+) What is JavaScript? JavaScript is more than just a language for manipulating web pages. It's a versatile programming language that powers a wide range of applications, from web development and mobile apps to game development and even server-side scripting. However, its roots lie in the web, and its ability to add interactivity and dynamism to web pages remains one of its core strengths Why Study JavaScript? Ubiquitous & Essential Versatile Toolset Nearly every interactive element on modern Beyond websites, JavaScript is used in websites, from animations and sliders to mobile apps (React Native, Ionic), game dynamic forms and user logins, relies on development (Phaser, PixiJS), and even JavaScript. Learning it gives you the server-side scripting (Node.js). Mastering power to build modern, engaging web JavaScript opens doors to diverse career experiences that stand out. paths and projects. Why Study JavaScript? Beginner-friendly Its syntax is clear and concise, and numerous resources and tutorials are available to guide your learning journey. The Main Parts Of JavaScript ECMAScript DOM BOM (European Computer The Document Object The Browser Object Model Manufacturer’s Model (DOM) provides (BOM) provides the browser Association as a standard) interfaces for interacting provides the core with elements on web API for interacting with the functionality. pages web browser. Getting Started Linking HTML AND CSS To JavaScript Getting to know your web console 02 Problem & Solution What Is Problem- Solving Techniques? Problem-solving techniques are a set of strategies you can use to approach, analyze, and find solutions to challenges. These techniques can be applied in various aspects of life, including programming, engineering, design, and even everyday situations. Here are some common problem-solving techniques. Problem-Solving Techniques Breaking Down the Problem Logical Reasoning & Identifying Inputs and Outputs Algorithms Test Cases and Expected Results Problem-Solving Techniques Problem: Write a program that calculates the area and perimeter of a rectangle or square, given its width and height (or side length for a square). Techniques: Breaking Down: Separate the problem into calculating area (width * height) and perimeter (2 * width + 2 * height) Inputs/Outputs: Inputs are width and height (numbers). Outputs are area (number) and perimeter (number). Test Cases: Input: Width = 5, Height = 3. Expected Output: Area = 15, Perimeter = 16 Input: Side Length = 4. Expected Output: Area = 16, Perimeter = 16 Solution Techniques: Use variables to store width and height. Apply conditional statements (if/else) to check if it's a rectangle (different width and height) or a square (equal width and height). Use separate calculations for area and perimeter based on the shape. Activity ! Each group is to pick a problem and apply the problem-solving techniques to draw out a plan on how to solve the problem Sample problems Area & Planning a Height of a Circumference child Future human of a circle Education Write a program that Write a program that finds Write a program that determines the height of a the area and determines the principal a men and women using the circumference of a circle parent would have to length of their femur invest to get a certain amount of money for a number of years Text Basic Simple Unit Manipulator Calculator Converter Write a program that Develop a program that Develop a program that takes a sentence as input performs basics arithmetic converts between basic and reverses the order of operations (addition, units of measurement the words. subtraction, multiplication, (centimeter to meters , division) on two user- meters to inches) provided numbers. 03 Variables & Datatype What is a variable? A variable is a labeled container that can store data of various types. Keywords needed to declare a variable in JavaScript var, let and const. Syntax for declaring a variable var catAge = 5; let catName = “Rick”; const isEmpty = true; What is a Data types? Data types defines the kind of data in a variable. JavaScript is a dynamically typed language, meaning that the values stored in a variable determines the datatype of the variable JavaScript Data type Categories Data types Primitive Complex Datatypes Datatypes Object Array Number String Null Undefined Datatypes Datatypes Boolean Null & Undefined Data Types Undefined Data Null Data types types let evenNumber = null; Iet oddNumber; Boolean & Number Data Types Boolean data types Number data types let isLoggedIn = true; Iet wholeNum =23; let isAwake = false; let realNum = 2.56; String Data Types String let firstName = “Chris”; Objects & Arrays Data Types Objects Arrays const person ={ name: “John Smith”, age: 35, let myArray =[“apple”,25,true]; isEmployed: true, skills: [“programming”, “writing”] } Accessing values Objects & Arrays Data Types Objects Arrays console.log(person.name); console.log(myArray); console.log(person.age); console.log(myArray); 04 Operators & Contol Flow What is an operator? JavaScript operators are special symbols that tell the language how to perform actions on a value. JavaScript Operators Arithmetic Logical Assignment operators Operator Operator Addition(+), Subtraction(-), NOT(!) , AND(&&) & Equal to sign(=) Multiplication(*) & OR(||) Division(/) Remainder Exponential Comparison Operator Operator Operator Double equal to sign(==), Triple Percentage sign(%) Double the multiplication equal to sign(==), not sign(**) equal(!=), less than(), less than or equal to(=) Arithmetic Operators Addition Subtraction Operator (+) Operator (-) Eg1: let sum = 40 + 50; Eg1: let result = 90 - 10; console.log (sum); console.log(result); Eg2: let num1 = 60; Eg2: let numb1 = 80; let num2 = 40; let numb2 = 40 ; let secSum = num1 + num2 let result = num1 - num2; console.log (secSum); console.log(result); Arithmetic operators Multiplication Division Operator (*) Operator (/) Eg1: let result = 40*2; Eg1: let resultDivide = 20 / 10; console.log(result); console.log(resultDivide); Eg2: let num1 = 12; Eg2: let num1 = 40; let num2 = 12; let num2 = 2; let result = num1 * num2; const result = num1/num2; console.log(result); console.log( result); Logical Operators Logical AND (&&) Logical OR (||) Eg2: let isEmpty = false, Eg1: let isEmpty = false; let isComplete= true; let isComplete= true; console.log(isEmpty || console.log(isEmpty && isComplete); isComplete); Logical Operators Logical NOT (!) Eg1: let isEmpty = false; let isComplete= true; console.log(isEmpty && isComplete); Remainder & Assignment Operators Remainder Assignment Operator(%) Operator (=) Eg1: let a = 90; let b = a; console.log(b); Eg1: let remainder = 10%2; Eg2: let c = 40; console.log(remainder). let d += c; console.log(d) Comparison Operator Loose Strict Equality(==) Equality(===) Eg1: consol.log(5 === “5”) Eg1: console.log(5 == “5”); Comparison Operator Strict Inequality(!==) Eg1: console.log(5 !== “5”); Comparison Operator Less than() Eg1: consol.log(10 > 5) Eg1: console.log(10 < 5); Comparison Operator Greater Than or Less than or Equal equal to(>=) to (= 16) { Eg 1: let age = 16; console.log('You can sign up.’); if (age == 16) { } else { console.log('You can drive.’); console.log('You must be at least } 18 to sign up.'); } The if…elseif…else Statement if (condition){statement} elseif{ statement} else{statement} let month = 6; let monthName; if (month == 1) { monthName = 'Jan’; } elseif (month == 2) { monthName = 'Feb’; } else{ monthName = “invalid month”; } console.log(monthName); For Loop Control Statement for( initializer; condition; iterator){ statement} Eg 1: for (let i = 1; i < 5; i++) { console.log(i); } While Loop Control Statement initiator; while(condition){statement; iterator;} Eg1: let count = 1; while (count < 10) { console.log(count); count +=2; } JavaScript Functions functions are reusable blocks of code that perform a specific task. Syntax For Declaring Functions & Access function functionName(parameters, parameters2, …){ Statement; } console.log(functionName(argument1, argument2,...); Function Sample code function calculateArea(width, height){ let area = width * height; return area; } let width = parseFloat(prompt(“Enter the width of the rectangle: ”)); let height = parseFloat(prompt(“Enter the height of the rectangle: ”)); let rectangleArea = calculateArea(width, height); console.log(“The area of the rectangle is: “, rectangleArea); Reading Assignments Research and read on the types of functions. Research and read on inbuilt functions or methods. Alternative resources Here’s an assortment of resources to help you learn more on JavaScript. MDN : https://developer.mozilla.org/en-US/docs/Web/JavaScript Videos : https://www.youtube.com/watch?v=zJSY8tbf_ys https://www.youtube.com/watch?v=W6NZfCO5SIk&t=16s&pp=ygUhamF2YXNjcmlwdCB0dX RvcmlhbCBmb3IgYmVnaW5uZXJz Thanks! Do you have any questions? [email protected] 0555661029