IT-Web Development and Programming-Unit 19-PHP Student's Guide PDF
Document Details
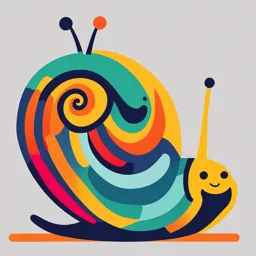
Uploaded by WinningNephrite5574
مدرسة WE للتكنولوجيا التطبيقية
2024
Tags
Summary
This student guide provides an introduction to PHP, covering various aspects of the programming language, including concepts, syntax, applications, and use cases.
Full Transcript
تطوير المواقع والبرمجيات الصف الثالث Unit 19 PHP IT Department Web Development and Programming Web Development and Programming STUDENT GUIDE 1st....
تطوير المواقع والبرمجيات الصف الثالث Unit 19 PHP IT Department Web Development and Programming Web Development and Programming STUDENT GUIDE 1st. 2024 - 2025 تطوير المواقع والبرمجيات Unit 19 PHP الثالث الصف Web Development and Programming STUDENT GUIDE 2 Unit 19 Name PHP Level 1 Goals / Remembering Outcomes 1. Recall the fundamental concepts of PHP and its role. 2. Remember the basic syntax for PHP variables and functions. 3. Identify PHP’s built-in functions and their usage. Understanding 1. Explain the core features of PHP and how it integrates with HTML. 2. Understand the role of PHP variables and functions. 3. Describe how PHP arrays, loops, and conditionals control program flow. 4. Explain the process of handling HTML forms using PHP. Applying 1. Write simple PHP scripts to demonstrate understanding of PHP syntax and basic functions. 2. Utilize PHP variables and functions in a practical script. 3. Implement PHP’s built-in functions to handle strings, arrays, and numbers. 4. Develop a PHP script that processes and validates form data from HTML forms. Analyzing 1. Compare PHP with other server-side scripting languages regarding syntax and functionality. 2. Assess the effectiveness of different PHP built-in functions. 3. Analyze how arrays, loops, and conditionals interact to control PHP script execution. 4. Evaluate different methods for handling and validating HTML form data in PHP. Evaluating 1. Evaluate the performance and efficiency of PHP scripts in different scenarios. 2. Judge the clarity and maintainability of PHP code that uses variables, functions, and control structures. 3. Assess the robustness of PHP form handling techniques 4. Select appropriate PHP functions and techniques for specific web development tasks. Creating 1. Develop a functional PHP application that demonstrates the use of variables, functions, and built-in functions. 3 2. Build a complete PHP project that includes handling arrays, loops, conditionals, and form data processing. 3. Create a set of PHP scripts that integrate with HTML forms 4. Construct a small web application using PHP. Code Description TPK20 writing asynchronous code using various techniques Knowledge TPK21 Basic routing and calling a controller method from a route TPK22 Analyze and solve common web applications tasks by writing PHP programs Code Description TPC5.1 Use database software tools to run queries and produce reports Skill TPC5.2 Write PHP scripts to handle HTML forms TPC5.3 Develop and use simple Dashboards and simple data visualization forms 4 Unit Preface 5 Lesson 1 Name Introducing To PHP Goals / By the end of this lesson, students should be able to: Outcomes Remembering 1. Recall the basic concepts and history of PHP. 2. Identify the uses and applications of PHP. 3. List the advantages and components of a PHP development environment. Understanding 1. Explain what PHP stands for and its evolution. 2. Describe how PHP integrates with HTML and web servers. 3. Understand PHP’s syntax, variable types, and data handling. Applying 1. Install PHP, Apache, and MySQL using XAMPP or similar tools. 2. Write and execute basic PHP scripts for dynamic web content. Analyzing 1. Analyze PHP’s role in server-side scripting 2. Compare PHP with other server-side languages Evaluating 1. Assess the performance and efficiency of PHP in web development. 2. Evaluate the suitability of PHP for different types of web applications. Creating 1. Develop and design basic PHP scripts to handle form data, generate dynamic content, and interact with databases. TPK20 writing asynchronous code using various techniques Knowledge Analyze and solve common web applications tasks by TPK22 writing PHP programs Use database software tools to run queries and produce Skill TPC5.1 reports 6 Lesson One: Introduction To PHP IN THIS LESSON WE LEARN: 1. Introduction to PHP 2. What is PHP Used For? 3. How PHP Works 4. Advantages of PHP 5. What is Apache? 6. Installing PHP, Apache, and MySQL 7. PHP Syntax 8. Variables in PHP 9. PHP Data Types 10. PHP Output Functions: echo and print 11. Constants in PHP 12. Scope in PHP 13. Comments in PHP 7 Section 1: PHP overview 1-1 Introduction To PHP PHP is an open-source, server-side programming language that can be used to create websites, applications, customer relationship management systems and more. It is a widely used general-purpose language that can be embedded into HTML. 1-2 What does PHP stand for? PHP Now stands for : ‘Hypertext Preprocessor’, But In The Past It Was stand for ‘Personal Home Page’. Since its release, there have been 8 versions of PHP, as of 2022, with version 8.1 currently a popular choice among those using the language on their websites. 1-3 What is PHP used for? PHP is primarily used for server-side web development. It enables the creation of dynamic web pages by embedding PHP code within HTML. PHP can perform various tasks, including handling form data, generating dynamic page content, managing databases, and interacting with servers. 8 1-4 How PHP Works? PHP runs on the Web server side. Your web browser at client end can’t execute PHP code. PHP script handles database and manages records at server end. Our PHP script at server end contains PHP code and other client-side codes. After execution of PHP code, the output is posted to client side. These outputs will contain HTML , JavaScript and style codes. 1-5 Advantages of PHP cross-platform. open source. large community. Easy Learn Great Performance. Security. Web servers Support. 9 Section 2: PHP setup 2-1 What Is Apache? Apache is a web server software that is responsible for accepting HTTP requests from visitors and sending them back the requested information in the form of web pages. Or in simpler terms, it allows visitors to view content on your website. 2-2 Installing PHP, Apache, and MySQL Setting up packages like XAMPP has simplified this process, making it easy for beginners to start coding quickly. XAMPP is popular for its ease of use and reliability, making it a great choice for new developers. Section 3: Working with PHP 3-1 PHP Syntax You can embed PHP code anywhere in a document. It starts with an opening tag of. All PHP statements end with a semicolon (;). A PHP file is always named with the file extension of.php for example, index.php or home.php. 10 3-2 Variables in PHP A variable is a container that stores data or values. In PHP, you create a variable with the dollar symbol ($) followed by the variable name. For a variable to be assigned to a value, we use the assignment operator (=). Here are a few important things to note about PHP variables: A variable is declared/executed with a dollar symbol $ then the variable name. Variable names are case sensitive. For example, $Ahmed is very different from $AHMED. A variable name shouldn’t and can’t start with a number, but rather a letter (Aa – Zz) or an underscore (_). 11 3-3 PHP Data types Data types specify the type of data a variable can hold. PHP supports several data types including: String: A sequence of characters ("Hello, World!") Integer: A non-decimal number (42) Float: A number with a decimal point (3.14) Boolean: Represents true or false values (true, false) Array: An ordered map of values (array("Apple", "Banana", "Cherry")) Object: An instance of a class NULL: Represents a variable with no value 12 3-4 PHP echo and print Statements echo and print are the same. They are both used to output data to the screen. The differences are small: 1-echo has no return value while print has a return value of 1 so it can be used in expressions. 2-echo can take multiple parameters , while print can take one argument. 3-echo is marginally faster than print. echo "PHP is Fun!"; echo "Hello world!"; echo "I'm about to learn PHP!"; echo "This ", "string ", "was ", "made ", "with multiple parameters."; print "PHP is Fun!"; print "Hello world!"; print "I'm about to learn PHP!"; 3-5 Constants Constants in PHP are like variables, except that once they are defined, they cannot be changed or undefined. Constants are useful for values that should remain constant throughout the script. Syntax: Constants are defined using the define() function. Notes: Constant names are typically written in uppercase. Constants do not use the $ symbol. 13 3-6 Comments in PHP As a developer, comments are crucial. Adding comments to your code makes it easier to read and understand. How to Write Single-Line Comments in PHP You can use the forward slash (/) or hash symbol (#) in PHP to denote a single line comment. For example: How to Write Multiple-Line Comments in PHP You can use the symbol to include a multi-line comment for example: 14 Terminology: PHP: an open-source, server-side programming language that can be used to create websites, applications, customer relationship management systems and more. Apache: a web server software that is responsible for accepting HTTP requests from visitors and sending them back the requested information in the form of web pages. Variable: is a container that stores data or values. In PHP, you create a variable with the dollar symbol ($) followed by the variable name. Constants: like variables, except that once they are defined, they cannot be changed or undefined. 15 Dear learner: Put "True" in front of the correct statement and "False" in front of the incorrect statement. No Question Answer 1 PHP is a client-side programming language. 2 PHP can be embedded into HTML. 3 The output of PHP code is sent to the server, not the client side. 4 PHP is not cross-platform. 5 Apache is a programming language. (Apache is a web server software.) 6 XAMPP simplifies the process of installing PHP, Apache, and MySQL. 7 PHP variables are case-sensitive. 8 A PHP file extension is always.php, for example, index.php. 9 In PHP, you can start a variable name with a number. 10 Constants in PHP can be changed after they are defined. Dear learner: Choose the correct answer. No. Question Answer 11 What does PHP stand for now? a) Personal Home Page b) Preprocessor Hypertext 16 c) Hypertext Preprocessor d) Page Hypertext Processor 12 Which of the following is a built-in function to output data in PHP? a) print() b) echo() c) display() d) Both a and b 13 What does Apache do? a) Acts as a database b) Is a programming language c) Manages web server requests d) Provides hosting services 14 Which tool simplifies the installation of PHP, Apache, and MySQL? a) WAMP b) XAMPP c) LAMP d) MAMP 15 In PHP, which of the following is used to declare a variable? a) & b) $ c) # d) @ 16 What is the correct file extension for a PHP file? a).html b).xml c).php d).js 17 17 Which function is used to define a constant in PHP? a) define() b) const() c) constant() d) def() 18 How can you write a single-line comment in PHP? a) // b) # c) d) Both a and b 19 Which of the following is a primary use of PHP? a) Client-side scripting b) Desktop application development c) Server-side web development d) Mobile application development 20 Which of the following statements about PHP variables is true? a) Variables are case-insensitive b) Variables can start with a number c) Variables start with a $ symbol d) Variables do not need to be declared 18 Lesson 2 Name PHP Variables and Introduction to Functions Goals / By the end of this lesson, students should be able to: Outcomes Remembering 4. Recall different types of PHP operators and their functions. 5. Identify various PHP control structures and their uses. 6. List different types of loops and their purposes in PHP. Understanding 4. Explain how PHP operators are grouped and used in expressions. 5. Describe the purpose and syntax of conditional statements and loops in PHP. 6. Understand how to use PHP functions and their basic syntax. Applying 3. Use PHP operators to perform arithmetic, comparison, and logical operations. 4. Implement conditional statements to control the flow of execution in PHP scripts. 5. Apply various loops to iterate over data and execute code multiple times. Analyzing 3. Analyze the effectiveness of different PHP control structures in various scenarios. 4. Compare the use of different loops and conditional statements for specific tasks. Evaluating 3. Assess the suitability of PHP operators and control structures for different coding requirements. 4. Evaluate the performance of PHP scripts utilizing different loops and conditions. Creating 2. Develop PHP scripts that incorporate variables, operators, conditional statements, and loops to solve problems and perform tasks. 19 TPK20 writing asynchronous code using various techniques Knowledge Analyze and solve common web applications tasks by TPK22 writing PHP programs Use database software tools to run queries and produce Skill TPC5.1 reports 20 IN THIS LESSON WE LEARN 1. PHP Operators Arithmetic Operators Assignment Operators Comparison Operators Increment/Decrement Operators Logical Operators String Operators Conditional Assignment Operators 2. PHP Conditional Operators if...else Statement if...elseif...else Statement switch Statement 3. Loops in PHP For vs Foreach Loop While vs Do...While Loop Break and Continue Statements Section 1:PHP Operators 21 1-1 PHP Operators Operators are symbols that instruct the PHP processor to carry out specific actions. For example, the addition (+) symbol instructs PHP to add two variables or values, whereas the greater-than (>) symbol instructs PHP to compare two values. PHP operators are grouped as follows: Arithmetic operators Assignment operators Comparison operators Increment/Decrement operators Logical operators String operators Conditional assignment operators 1-2 Arithmetic Operators The PHP arithmetic operators are used in conjunction with numeric values to perform common arithmetic operations such as addition, subtraction, multiplication, and so on. Example: 22 1-3 Assignment Operators Assignment operators are used to assign values to variables. Example: 1-4 Comparison Operators Comparison operators are used to compare two values in a logical way. Example: 24 1-5 Increment / Decrement Operators Increment operators are used to increment a variable's value while decrement operators are used to decrement. Example: 1-6 Logical Operators Logical operators are typically used to combine conditional statements. Exmple: 25 1-7 String Operators String operators are specifically designed for strings. Example: 26 1-8 Conditional Assignment Operators Conditional assignment operators are used to set a value depending on conditions. Example: Section 2: Conditional Statements 27 2-1 conditional statements in your code. PHP have the following conditional statements: if statement - executes some code if one condition is true. if...else statement - executes some code if a condition is true and another code if that condition is false. if...elseif...else statement - executes different codes for more than two conditions. switch statement - selects one of many blocks of code to be executed. 2-2 The if Statement The if statement is used to execute a block of code only if the specified condition evaluates to true. 2-3 The if...else Statement The if...else statement executes one block of code if the specified condition is evaluated as to true and another block of code if it is evaluated to be false. 28 2-4 The if...elseif...else Statement The if...elseif...else statement executes different codes for more than two conditions. 2-5 Switch...Case The switch statement is used to select one of many blocks of code to be executed. This is an alternative to the if...elseif...else statement. 29 30 Example: Section 3: Loops 3-1 For Loop The for loop repeatedly executes a block of code if a certain condition is met. It is used mostly to execute a block of code for certain number of times. The following is the syntax for the PHP for loop: for (init counter; test counter; increment counter) { code to be executed for each iteration. } Example: 3-2 Foreach Loop 31 The foreach loop works only on arrays and is used to loop through each key/value pair in an array. Increment is automatic. The syntax for the PHP foreach loop is: foreach ($array as $value) { code to be executed; } Example: 3-3 While Loop The while loop repeatedly executes a block of code as long as the specified condition is true. The syntax for the while loop is as follows: while (condition is true) { code to be executed; } Example: 3-4 Do...While Loop The do...while loop is a variant of while loop. It evaluates the condition at the end of each loop iteration. Thus, a do-while loop the block of code is executed once before the condition is evaluated (this is its difference from the while loop). The following is the syntax for the PHP do...while loop. do { code to be executed. 32 } while (condition is true); Example: 3-5 Break/Continue The break statement can be used to exit out of a loop ,but the continue statement skips one iteration of the loop. Terminology: Operators: symbols that instruct the PHP processor to carry out specific actions. Arithmetic operators are used in conjunction with numeric values to perform common arithmetic operations such as addition, subtraction, multiplication. 33 Assignment operators: are used to assign values to variables. Comparison operators: are used to compare two values in a logical way. Increment and decrement operators are used to increment a variable's value while decrement operators are used to decrement. Logical operators: are typically used to combine conditional statements. Conditional assignment: operators are used to set a value depending on conditions. If statement is used to execute a block of code only if the specified condition evaluates to true. Switch statement is used to select one of many blocks of code to be executed. This is an alternative to the if...elseif...else statement. For loop repeatedly executes a block of code if a certain condition is met. It is used mostly to execute a block of code for certain number of times. Foreach: is used to loop through each key/value pair in an array. Increment is automatic. while loop repeatedly executes a block of code as long as the specified condition is true. do...while loop is a variant of while loop. It evaluates the condition at the end of each loop iteration. break: can be used to exit out of a loop. continue: skips one iteration of the loop, if a specified condition occurs, and continues with the next iteration in the loop. 34 Dear learner: Put "True" in front of the correct statement and "False" in front of the incorrect statement. No Question Answer 21 Arithmetic operators in PHP include addition, subtraction, multiplication, and division. 22 Assignment operators are used to assign values to variables. 23 The assignment operator in PHP is ==. 24 Comparison operators in PHP are used to assign values to variables. 25 The comparison operator in PHP is !==. 26 Increment operators are used to increment a variable's value while decrement operators are used to decrement. 27 The increment operator (++) increases a variable's value by 1. 28 Logical operators in PHP can be used to combine multiple conditions. 29 String operators are specifically designed for numeric operations in PHP. 30 Conditional assignment operators are used to set a value based on a condition. Dear learner: Choose the correct answer. No. Question Answer 35 31 Which operator is used to compare two values in PHP? a) = b) == c) === d) Both b and c 32 Which of the following is a correct usage of the increment operator in PHP? a) $x + 1; b) $x++; c) ++$x; d) Both b and c 33 What will the following PHP code output? a) 10 b) 11 c) 9 d) Error 34 Which logical operator is used to combine two conditions that must both be true? a) && b) || 36 c) ! d) and 35 In PHP, what does the following code do? a) Assigns "World!" to $x b) Outputs "Hello World!" c) Concatenates " World!" to "Hello" d) Throws an error Dear learner: Complete the question. No. Question Answer 36 Arithmetic operators in PHP include addition, subtraction, multiplication, division, and _____. 37 The assignment operator in PHP is represented by the _____ symbol. 38 Comparison operators are used to compare two values and include operators like ==, !=, and _____. 39 The ++ operator is used to _____ a variable's value by 1. 40 Logical operators such as &&, ||, and ! are used to combine or invert _____. Lesson 3 37 Name PHP Built-in Functions Goals / By the end of this lesson, students should be able to: Outcomes Remembering 7. Recall the purpose and syntax of PHP functions. 8. Identify the categories of built-in PHP functions. Understanding 7. Explain the benefits of using functions in PHP. 8. Describe how to create and use user-defined functions. Applying 1. Implement user-defined functions to perform specific tasks. 2. Utilize built-in functions for string manipulation, numeric calculations, and date handling. Analyzing 1. Analyze how built-in functions simplify complex operations. 2. Evaluate the use of different function categories for various programming needs. Evaluating 1. Assess the effectiveness of using built-in versus user- defined functions in different scenarios. 2. Compare different functions for handling strings, numbers, and dates. Creating 1. Develop PHP scripts that leverage both built-in and user-defined functions to achieve desired functionality. Code Description TPK20 writing asynchronous code using various techniques Knowledge TPK21 Basic routing and calling a controller method from a route TPK22 Analyze and solve common web applications tasks by writing PHP programs Code Description Skill TPC5.1 Use database software tools to run queries and produce reports 38 IN THIS LESSON WE LEARN: 1. PHP Functions Functions User-Defined Functions Anonymous Functions Arrow Functions 2. Built-in Functions String Functions Numeric Functions Date Functions 3. Include Files include() require() include_once() require_once() 39 Section 1: Functions 1-1 What Is PHP Functions A Function in PHP is a reusable piece or block of code that performs a specific action. It takes input from the user in the form of parameters, performs certain actions, and gives the output. Functions can either return values when called or can simply perform an operation without returning any value. PHP has over 700 functions built in that perform different tasks. 1-2 Why use Functions? Better code organization Reusability Easy maintenance 1-3 User-Defined Functions in PHP A user-defined function is a function that is created by a programmer to perform a specific set of tasks or calculations. Unlike built-in functions, which are provided by the programming language or libraries, user-defined functions are customized to suit their specific needs. 40 To create a user-defined function, start by adding the function keyword, followed by the desired name for your function. Choose a meaningful and descriptive name that reflects the purpose of the function. For example, let's create a function called calculateSum(): If your function requires input from a user, you can define parameters within the parentheses after the function name. Parameters act as placeholders for values that are passed into the function when it’s called. To use the user-defined function, simply call it by its name followed by parentheses, providing any required arguments, like so: 41 1-4 PHP Function ArgumentsFunction Arguments are variables that are passed to a function when it’s called. These arguments provide input to the function and can be used within its code to perform specific actions or calculations. 1-5 How to Pass Variables to Functions To pass a variable to a function, start with defining the function and specifying its parameters – like so: Here, $name is the function argument. When greet() is called, its value, “Alice” is passed as an argument and assigned to the $name variable within the function. 1-6 Anonymous functions Anonymous functions, also known as closures, are functions without names. They can be assigned to variables or called directly, making them useful for creating functions on the fly. The general syntax for creating an anonymous function in PHP is as follows: 42 1-7 Arrow functions Arrow functions, also known as short closures, were implemented in PHP 7.4. They define anonymous functions in a more concise way. These functions enable you to create short, single-expression functions without the need for the function keyword or curly braces. The basic syntax for an arrow function is as follows: fn (argument_list) => expression Section 2: Built in Functions 2-1 PHP Built in Functions Built in functions are predefined functions in PHP that exist in the installation package. These PHP inbuilt functions are what make PHP a very efficient and productive scripting language. The built-in functions of PHP can be classified into many categories. Below is the list of the categories. 43 2-2 String Functions These are functions that manipulate string data. Here are some of the most important PHP built-in functions for working with strings: 1-strlen(): Returns the length of a string.