Introduction to Computers - Lec 5 PDF
Document Details
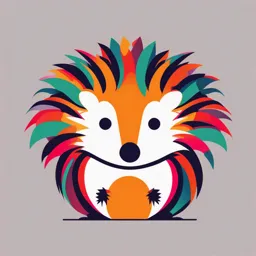
Uploaded by InestimableHeliotrope2806
Dr Islam Alkabbany
Tags
Related
- Lecture 1: Subject Introduction and Overview on Structured Programming Approach PDF
- Software Development Basics PDF
- Computer Science Algorithms and Problem Solving 2015 PDF
- Unit 02: Computational Thinking and Algorithms PDF
- Software Programming Chapter 10 PDF
- Software Development Unit 1 - Introduction to Algorithm PDF
Summary
This document provides a lecture on the basics of algorithms, including examples and control structures. The lecture also introduces the software development life cycle, pseudocode, and flowcharts.
Full Transcript
Introduction to Computer Systems Module 5: Algorithm Development Dr Islam Alkabbany Objectives Recognize the program development life cycle. Select appropriate computer-based methods for Modeling and analyzing problems. Differentiate between algorithm, pseudo code, and...
Introduction to Computer Systems Module 5: Algorithm Development Dr Islam Alkabbany Objectives Recognize the program development life cycle. Select appropriate computer-based methods for Modeling and analyzing problems. Differentiate between algorithm, pseudo code, and flowchart. Software development life cycle Software development life cycle 1- Planning (Problem Definition) In this phase, we define the problem statement and we decide the boundaries of the problem. In this phase we need to understand the problem statement, what is our requirement, what should be the output of the problem solution. These are defined in this first phase of the program development life cycle. Software development life cycle 2- Problem Analysis Precisely define the problem to be solved, and write program specifications – descriptions of the program’s inputs, processing, outputs, and user interface. Software development life cycle 3- Design Develop a detailed logic plan using a tool such as pseudo-code, flowcharts, object structure diagrams, or event diagrams to group the program’s activities into modules; devise a method of solution or algorithm for each module and test the solution algorithms. Software development life cycle 4- Implementation Translate the design into an application using a programming language or application development tool by creating the user interface and writing code; include internal documentation – comments and remarks within the code that explain the purpose of code statements. Software development life cycle 5- Testing and Integration Test the program, finding and correcting errors (debugging) until it is error-free and contains enough safeguards to ensure the desired results. Software development life cycle 6- Maintenance Provide education and support to end- users; correct any unanticipated errors that emerge and identify user-requested modifications (enhancements). Once errors or enhancements are identified, the program development life cycle begins again at Step 1. Software development life cycle Retirement The unofficial eighth step of the SDLC is retirement. No software lives forever. As it ages, stakeholders will find problems that need to be corrected, usually in a new version of the code. Or, they will decide to create an entirely new replacement application. Software development life cycle Documentation The document is to put together all of the materials that have been generated throughout the PDLC process. All of the flowcharts, messages, algorithms, lines of code, and the user manuals are part of this documentation. Internal documentation: is used by other programmers to help them know why you did something a certain way or tell them how you wrote a program. External documentation: includes user manuals and anything that is not the actual code or is part of the listing. This should also include materials that are placed on a website such as FAQs (frequently asked questions) and help areas. Algorithm Algorithm is an ordered sequence of finite, well defined, unambiguous instructions for completing a task. The term Algorithm derives from the name of Muhammad ibn Musa al’Khwarizmi Algorithm (Example) Problem: Find the area of a circle of radius r. Inputs to the algorithm Radius r of the circle. Expected output: Area of the circle Algorithm (Example) Algorithm: Step1: Read/input the Radius r of the circle Step2: Compute Area = 3.14*r*r Step3: Print Area Algorithm (Example) Algorithm to find the greatest among three numbers ALGORITHM 1. Step 1: Read the three numbers A, B, C. Step 2: Compare A and B. If A is greater perform step 3 else perform step 4. Step 3: Compare A and C. If A is greater, output “A is greatest” else output “C is greatest”. Step 4: Compare B and C. If B is greater, output “B is greatest” else output “C is greatest”. Algorithm (Example) Algorithm to find the greatest among three numbers ALGORITHM 2. Step 1: Read the three numbers A, B, C. Step 2: Compare A and B. If A is greater, store A in MAX, else store B in MAX. Step 3: Compare MAX and C. If MAX is greater, output “MAX is greatest” else output “C is greatest”. Algorithm ( Control structure) Sequential instructions are executed in linear order Step1: Read/input the Radius r of the circle Step2: Compute Area = 3.14*r*r Step3: Print Area Algorithm ( Control structure) Selection (branch or conditional): it asks a true/false question and then selects the next instruction based on the answer instructions are executed in linear order Step 1: Read the three numbers A, B, C. Step 2: Compare A and B. If A is greater perform step 3 else perform step 4. Step 3: Compare A and C. If A is greater, output “A is greatest” else output “C is greatest”. Step 4: Compare B and C. If B is greater, output “B is greatest” else output “C is greatest”. Algorithm ( Control structure) Iterative (loop) it repeats the execution of a block of instructions Step1: Read/input the Radius r of the circle Step2: Compute Area = 3.14*r*r Step3: Print Area Step4: Goto step 1 Flowchart is a diagrammatic representation of the logic for solving a task / diagrammatic representation of the Algorithm. Flowchart (Control Structures) Flowchart (Example) 1. Flowchart of a program that computes product of any two numbers Flowchart (Example) 2. Flowchart of a program that computes three numbers and finds the maximum Flowchart (Example) 3. Flowchart of a program that finds the sum of first 100 integers Pseudo Code Pseudo code consists of short, readable and formally-styled English language used for explaining an algorithm. In a pseudo code, some terms are commonly used to represent the various actions. For example: – for inputting data the terms may be (INPUT, GET, READ) – for outputting data (OUTPUT, PRINT, DISPLAY) – for calculations (COMPUTE, CALCULATE) – for incrementing (INCREMENT), initialization (INITIALIZE) Pseudo Code (Control Structures) Pseudo Code Pseudo Code