Image Processing in Python: Canny Edge & Face Detection PDF
Document Details
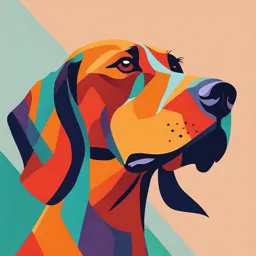
Uploaded by DeadOnCalculus9331
De La Salle University – Dasmariñas
Rebeca Gonzalez
Tags
Summary
This document is a tutorial on image processing in Python, covering techniques such as Canny edge detection, corner detection, and face detection using scikit-image. It also discusses real-world applications like privacy protection by blurring faces. The tutorial recommends OpenCV as a good next step.
Full Transcript
Finding the edges with Canny IMAGE PROCESSING IN PYTHON Rebeca Gonzalez Data Engineer Detecting edges Most of the shape information of an image is enclosed in edges. Detecting edges This image shows how th...
Finding the edges with Canny IMAGE PROCESSING IN PYTHON Rebeca Gonzalez Data Engineer Detecting edges Most of the shape information of an image is enclosed in edges. Detecting edges This image shows how the edges hold information about the domino's tokens. Representing an image by its edges has the advantage that the amount of data is reduced significantly while retaining most of the image information, like the shapes. Edge detection We have discussed how to detect edges using the Sobel filtering technique. Edge detection Another technique considered to be the standard method is Canny edge detection. This produces higher accuracy inin detecting edges compared with Sobel algorithm. Edge detection Tip: if you need a quick and general edge detection, Sobel is a good choice. If the task demands more precise and clean edge detection, especially in noisy images, Canny is the better option. Edge detection from skimage.feature import canny # Convert image to grayscale coins = color.rgb2gray(coins) # Apply Canny detector canny_edges = canny(coins) # Show resulted image with edges show_image(canny_edges, "Edges with Canny") Edge detection Canny edge detector # Apply Canny detector with a sigma of 0.5 canny_edges_0_5 = canny(coins, sigma=0.5) # Show resulted images with edges show_image(canny_edges, "Sigma of 1") show_image(canny_edges_0_5, "Sigma of 0.5") The first step in the Canny edge detection algorithm involves applying a Gaussian filter to remove noise from the image. This filter, similar to the one discussed in our course, can have its intensity adjusted through the sigma attribute in the Canny function. Canny edge detector Setting a lower sigma value in the Gaussian filter for the Canny edge detection algorithm, such as 0.5, applies less filtering, allowing more edges to be detected. Conversely, a higher sigma value removes more noise, resulting in fewer detected edges; the default value for sigma is 1. Questions? Right around the corner IMAGE PROCESSING IN PYTHON Data Engineer Corner detection Corner detection is an approach used to extract certain types of features and infer the contents of an image. It's frequently used in motion detection, image registration, video tracking, panorama stitching, 3D modelling, and object recognition. Corner detection Points of interest Features are points of interest in an image that are robust to changes in rotation, translation, brightness, and scale, providing valuable information about the image content. Corner detection specifically identifies these interest points, focusing on corners as a key type of feature alongside edges. Corners A corner can be defined as the intersection of two edges. Intuitively, it can also be a junction of contours. We can see some obvious corners in this checkerboard image. Or in this building image on the right. Matching corners By detecting corners as interest points, we can match objects across images taken from different perspectives. For example, corners identified in an original image can be matched to those in a downscaled version of the same image, demonstrating the technique's utility in recognizing and aligning features across varying scales. Matching corners Here is another example of corner matching, this time, in a rotated image. We see how the relevant points are still being matched. Harris corner detector The Harris Corner Detector is a widely used corner detection operator in computer vision that works by identifying regions in an image where the intensity shows significant changes in multiple directions, indicating the presence of corners. Harris corner detector Here, we see an original image of a building, and on the right we see the corners detected by the Harris algorithm, marked in red Harris corner detector Let's use this image of a Japanese gate to work with the Harris detector. Harris corner detector from skimage.feature import corner_harris # Convert image to grayscale image = rgb2gray(image) # Apply the Harris corner detector on the image measure_image = corner_harris(image) # Show the Harris response image show_image(measure_image) The corner_harris function computes the Harris response for each pixel, producing a 2D array (measure_image) where each value represents the "corner strength" at that pixel location. Higher values in measure_image indicate areas that are more likely to be corners. Harris corner detector Using show_image, we see how only some black lines are shown. These are the approximated points where the corners candidates are. Harris corner detector # Finds the coordinates of the corners coords = corner_peaks(corner_harris(image), min_distance=5) print("A total of", len(coords), "corners were detected.") A total of 122 corners were found from measure response image. The corner_peaks algorithm identifies points in the image that have strong corner responses and are at least min_distance pixels apart. The output is a 2D array where each row represents the coordinates of one detected corner point. Corners detected # Show image with marks in detected corners show_image_with_detected_corners(image, coords) Show image with contours Questions? Face detection IMAGE PROCESSING IN PYTHON Rebeca Gonzalez Data Engineer Face detection use cases Filters Auto focus Recommendations Blur for privacy protection To recognize emotions later on Detecting faces with scikit-image With scikit-image, we can detect faces using a machine learning classifier, with just a couple of lines! Detecting faces with scikit-image # Import the classifier class from skimage.feature import Cascade # Load the trained file from the module root. trained_file = data.lbp_frontal_face_cascade_filename() What’s up with trained_file ??? The trained_file object is a string that holds the file path to the xml file Detecting faces with scikit-image # Import the classifier class from skimage.feature import Cascade # Load the trained file from the module root. trained_file = data.lbp_frontal_face_cascade_filename() # Initialize the detector cascade. detector = Cascade(trained_file) Note: To use the face detector, we import the Cascade class from the feature module. This detection framework needs an xml file, from which the trained data can be read. Let's try it Note: It’s often called a cascade classifier because it uses a series of simple, sequential classifiers (or stages) arranged in a cascade structure. Each stage is a filter that decides whether a region of the image likely contains the object of interest (such as a face or a specific pattern). Detecting faces To detect faces, the detect_multi_scale method from the Cascade class can be used. This method moves a window across the image to find areas resembling a human face. Detecting faces Searching happens on multiple scales. The window will have a minimum size, to spot the small or far-away faces. And a maximum size to also find the larger faces in the image. Detecting faces The detect_multi_scale method for face detection adjusts the window size at each step by a scale factor and moves the window by a step ratio, where 1 indicates an exhaustive, though slow, search. Faster results but less accurate, can be achieved by increasing this ratio. Typically, values between 1 and 1.5 balance speed and accuracy well. Setting the min and max sizes for the search window to define the range of detection is required. Detected faces print(detected) Detected face: [{'r': 115, 'c': 210, 'width': 167, 'height': 167}] The detector will return the coordinates of the box that contains the face. When printing the result, we see that it's a dictionary, where r represents the row position of the top left corner of the detected window, c is the column position pixel, width is width of detected window, and height, the height of the detected window. Detected faces # Show image with detected face marked show_detected_face(image, detected) We'll use a function that shows the original image with the detected face marked with red lines, forming a box containing the face. This draw a rectangle around detected faces. Show detected faces Detected can look like this: [ {'r': 50, 'c': 100, 'width': 60, 'height': 60}, # First detected object {'r': 150, 'c': 200, 'width': 80, 'height': 80}, # Second detected object {'r': 250, 'c': 300, 'width': 70, 'height': 70} # Third detected object ] Questions? Real-world applications IMAGE PROCESSING IN PYTHON Rebeca Gonzalez Data engineer Applications Turning to grayscale before detecting edges/corners Reducing noise and restoring images Blurring faces detected Approximation of objects' sizes Privacy protection We would implement a privacy protection case by detecting faces and then anonymizing them. Privacy protection Privacy protection Remember: print(detected) Detected face: [{'r': 115, 'c': 210, 'width': 167, 'height': 167}] Privacy protection Privacy protection Privacy protection Privacy protection The result is an image that no longer contains people's faces in it and in this way, personal data is anonymized More cases Class Photo Great work! IMAGE PROCESSING IN PYTHON Rebeca Gonzalez Data Engineer Recap: What you have learned Improved contrast Restored images Applied filters Rotated, flipped and resized! Segmented: supervised and unsupervised Applied morphological operators Created and reduced noise Detected edges, corners and faces And mixed them up to solve problems! What's next? OpenCV (Open Source Computer Vision Library) is a comprehensive library primarily aimed at real-time computer vision. While scikit-image (or skimage) is also a powerful tool for image processing, OpenCV offers some additional functionalities and optimizations especially beneficial in certain domains. What's next? ✓ Real-Time Processing: ✓ Video Analysis ✓ Machine Learning and Deep Learning ✓ Camera Calibration and 3D Reconstruction Motion Analysis ✓ GUI Features ✓ Wide Range of Algorithms for Object Detection ✓ Integration with Other Technologies Scikit Image vs OpenCV Congrats! IMAGE PROCESSING IN PYTHON