Revision Tour -1 Python Programming PDF
Document Details
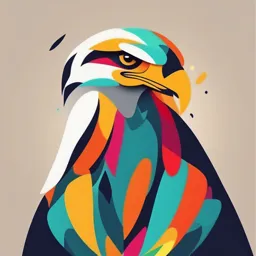
Uploaded by PerfectChiasmus5678
Kendriya Vidyalaya
Mihika Gupta
Tags
Summary
This document contains a revision tour on Python programming, outlining topics. It includes a list of questions and answers, separated by difficulty level (1, 2 and 3 marks). Examples and practical implementations of string manipulation are also observed.
Full Transcript
OUR PATRON Sh. D. P. Patel Deputy Commissioner Kendriya Vidyalaya Sangathan, Ranchi Region Chief Patron Smt. Sujata Mishra Assistant Commissioner Kendriya Vidyalaya Sangathan, Ranchi Region Pat...
OUR PATRON Sh. D. P. Patel Deputy Commissioner Kendriya Vidyalaya Sangathan, Ranchi Region Chief Patron Smt. Sujata Mishra Assistant Commissioner Kendriya Vidyalaya Sangathan, Ranchi Region Patron Sh. R C Gond Principal Kendriya Vidyalaya Patratu Convener CONTENT TEAM NAME OF TEACHER DESIGNATION NAME OF KV Mr. K Chakrovortty PGT CS K V GOMOH Mr. Amod Kumar PGT CS K V PATRATU Mr. Ravi Prakash PGT CS K V DIPATOLI Mr. Sanjay Kumar Sinha PGT CS K V CCL Mr. Lalit Kumar Jha PGT CS K V Bokaro No 1 Mr. R N P Sinha PGT CS K V HINOO 2ND SHIFT Mr. Rakesh Kumar Pathak PGT CS K V BOKARO THERMAL Mr. Aftab Alam Shahnwaz PGT CS K V RAMGARH Mr. Pravin Kumar Singh PGT CS K V DHANDAB NO 1 Mr. Mantosh Kumar PGT CS K V DEEPATOLI Mr. Murtaza Ali PGT CS K V GODDA Mr. Anand Prasad PGT CS K V JAMTARA INDEX SL NO. TOPIC PAGE 01 Revision of Python topics 1 02 Functions & Exception Handling 21 03 Introduction to files & Text Files 32 04 Binary Files 44 05 CSV files 57 06 Data Structures (Stack) 64 07 Computer Network 74 08 Database Management System 94 Interface of python with SQL 09 115 database: Revision Tour -1 Data Types: Data Type specifies which type of value a variable can store. type() function is used to determine a variable's type in Python Data Types In Python 1. Number 2. String 3. Boolean 4. List 5. Tuple 6. Set 7. Dictionary Python tokens : (1) keyword : Keywords are reserved words. Each keyword has a specific meaning to the Python interpreter, and we can use a keyword in our program only for the purpose for which it has been defined. As Python is case sensitive, keywords must be written exactly. (2) Identifier : Identifiers are names used to identify a variable, function, or other entities in a program. The rules for naming an identifier in Python are as follows: The name should begin with an uppercase or a lowercase alphabet or an underscore sign (_). This may be followed by any combination of characters a–z, A–Z, 0–9 or underscore (_). Thus, an identifier cannot start with a digit. It can be of any length. (However, it is preferred to keep it short and meaningful). It should not be a keyword or reserved word We cannot use special symbols like !, @, #, $, %, etc., in identifiers. (3) Variables: A variable in a program is uniquely identified by a name (identifier). Variable in Python refers to an object — an item or element that is stored in the memory. Comments: Comments are used to add a remark or a note in the source code. Comments are not executed by interpreter. a comment starts with # (hash sign). Everything following the # till the end of that line is treated as a comment and the interpreter simply ignores it while executing the statement. Mutable and immutable data types : Variables whose values can be changed after they are created and assigned are called mutable. Variables whose values cannot be changed after they are created and assigned are called immutable. (4) Operators: An operator is used to perform specific mathematical or logical operation on values. The values that the operators work on are called operands. Arithmetic operators :four basic arithmetic operations as well as modular division, floor division and exponentiation. (+, -, *, /) and (%, //, **) Relational operators : Relational operator compares the values of the operands on its either side and determines the relationship among them. ==, != , > , < , = Logical operators : There are three logical operators supported by Python. These operators (and, or, not) are to be written in lower case only. The logical operator 1 Page evaluates to either True or False based on the logical operands on either side. and , or , not Assignment operator : Assignment operator assigns or changes the value of the variable on its left. a=1+2 Augmented assignment operators : += , -= , /= *= , //= %= , **= Identity operators : is, is no Membership operators : in, not in Type Conversion: The process of converting the value of one data type (integer, string, float, etc.) to another data type is called type conversion.Python has two types of type conversion. Implicit Type Conversion /automatic type conversion Explicit Type Conversion CONTROL STATEMENTS Control statements are used to control the flow of execution depending upon the specified condition/logic. There are three types of control statements: 1. Decision Making Statements (if, elif, else) 2. Iteration Statements (while and for Loops) 3. Jump Statements (break, continue, pass) Questions and Answers 1 Mark questions Q1. Which of the following is not considered a valid identifier in Python: (i)three3 (ii)_main (iii)hello_kv1 (iv)2 thousand Q2.Which of the following is the mutable data type in python: (i)int (ii) string (iii)tuple (iv)list Q3. Which of the following statement converts a tuple into a list in Python: (i) len(string) (ii)list(tuple) (iii)tup(list) (iv)dict(string) Q4. Name of the process of arranging array elements in a specified order is termed as i)indexing ii)slicing iii)sorting iv) traversing Q5. What type of value is returned by input() function bydefault? i)int ii)float iii)string iv)list Q6. Which of the following operators cannot be used with string (i) + ii) * iii) - iv) All of these Q7. If L = [0.5 * x for x in range(0,4)] , L is i)[0,1,2,3] ii)[0,1,2,3,4] iii) [0.0,0.5,1.0,1.5] iv) [0.0,0.5,1.0,1.5,2.0] Q8. Write the output of the following python code: x = 123 for i in x: print(i) i)1 2 3 ii) 123 iii)infinite loop iv) error Q9. write the ouput of following code A = 10/2 B = 10//3 print(A,B) i)5,3.3 ii) 5.0 , 3.3 iii) 5.0 , 3 iv) 5,4 Q10. Name the built-in mathematical function / method that is used to return square root of a number. i) SQRT() ii)sqrt() iii) sqt() iv) sqte() 2 Page 2 MARK QUESTIONS Q1. Find the following python expressions: a) (3-10**2+99/11) b) not 12 > 6 and 7 < 17 or not 12 < 4 c) 2 ** 3 ** 2 d) 7 // 5 + 8 * 2 / 4 – 3 Q2. i) Convert the following for loop into while loop for i in range(10,20,5): print(i) ii) Evaluate:- not false and true or false and true Q3. What are advantages of using local and global variables ? Q4. Remove the errors from the following code Rewrite the code by underlining the errors. x = int((“enter the value”) for i in range [0,11]: if x = y print x+y else: print x-y Q5. Rewrite the following code in python after removing all syntax errors. Underline each correction done in the code: def func(x): for i in (0,x): if i%2 =0: p=p+1 else if i%5= =0 q=q+2 else: r=r+i print(p,q,r) func(15) Q6. Write the output of the following code:- String1="Coronavirus Disease" print(String1.lstrip("Covid")) print(String1.rstrip("sea")) Q7. Write the ouput of the following code:- Text = "gmail@com" L=len(Text) Ntext="" for i in range(0,L): if Text[i].isupper(): Ntext=Ntext+Text[i].lower() elif Text[i].isalpha(): Ntext= Ntext+Text[i].upper() else: Ntext=Ntext+'bb' print(Ntext) Q8. L = [“abc”,[6,7,8],3,”mouse”] Perform following operations on the above list L. i)L[3:] ii) L[: : 2] iii)L[1:2] iv) L Q9.Write the output of the following: word = 'green vegetables' print(word.find('g',2)) print(word.find('veg',2)) print(word.find('tab',4,15)) 3 Page 3 MARK QUESTIONS Q1. Write the python program to print the index of the character in a string. Example of string : “pythonProgram” Expected output: Current character p position at 0 Current character y position at 1 Current character t position at 2 Q2. Find and write the output of the following python code: string1 = "Augmented Reality" (i) print(string1[0:3]) (ii) print(string1[3:6]) (iii) print(string1[:7]) (iv) print(string1[-10:-3]) (v) print(string1[-7: :3]*3) (vi) print(string1[1:12:2]) Q3. Find the output of the give program : x = "abcdef" j = "a" for i in x: print(j, end = " ") Q4. Find output generated by the following code: i=3 while i >= 0: j=1 while j str = ‘ ‘ Accessing Values in Strings Each individual character in a string can be assessed using a technique called indexing. Python allows both positive and negative indexing. S = “Python Language” 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 P y t h o n L a n g u a g e -15 -14 -13 -12 -11 -10 -9 -8 -7 -6 -5 -4 -3 -2 -1 >>> S >>>S[-10] L n Deleting a String As we know, strings are immutable, so we cannot delete or remove the characters from the string but we can delete entire string using del keyword. >>> strl = " WELCOME " >>> del strl >>> print ( str1 ) String Slicing NameError : name ' strl ' is not defined. To access some part of a string or substring, we use a method called slicing. Syntax: string_name[start : stop] >>> str1 = " Python Program " >>> print ( str1[ 3: 8]) >>> print ( str1 [ : -4 ] ) >>> print ( strl [ 5 : ] ) hon P Python Pro n Program Strings are also provide slice steps which used to extract characters from string that are not consecutive. Syntax string_name [ start : stop : step ] >>> print ( stri [ 2 : 12 : 3 ] ) tnrr We can also print all characters of string in reverse order using [ ::-1 ] >>> print ( strl [ :: - 1 ] ) margorP nohtyP Traversing a String 1. Using ' for’ loop: for loop can iterate over the elements of a sequence or string. It is used when you want to traverse all characters of a string. eg. Output: >>> sub = " GOOD " G >>> for i in subs: O print ( i ) O D 2. Using ' for ' loop with range >>> sub = " COMPUTER " >>> for i in range ( len ( sub ) ) : print ( sub [ i ], ‘-’, end = ‘ ‘) Output: Output C - O - M - P - U - T - E - R String Operations Concatenation To concatenate means to join. Python allows us to join two strings using the concatenation operator plus which is denoted by symbol +. 8 Page >>> str1 = 'Hello' #First string >>> str2 = 'World!' #Second string >>> str1 + str2 #Concatenated strings 'HelloWorld!' String Replication Operator ( * ) Python allows us to repeat the given string using repetition operator which is denoted by symbol (*). >>> a = 2 * " Hello " >>> print ( a ) HelloHello Comparison Operators Python string comparison can be performed using comparison operators ( == , > , < , , < = , > = ). These operators are as follows >>> a = ' python ' < ' program ' >>> print ( a ) False >>> a = ' Python ' >>> b = ' PYTHON " >>> a > b True Membership Operators are used to find out whether a value is a member of a string or not. (i) in Operator: (ii) not in Operator: >>> a = "Python Programming Language" >>> a = "Python Programming Language" >>> "Programming" in a >>> "Java" not in a True True BUILT IN STRING METHODS Method Description len() Returns length of the given string title() Returns the string with first letter of every word in the string in uppercase and rest in lowercase lower() Returns the string with all uppercase letters converted to lowercase upper() Returns the string with all lowercase letters converted to uppercase count(str, start, end) Returns number of times substring str occurs in the given string. find(str,start, Returns the first occurrence of index of substring stroccurring in end) the given string. If the substring is not present in the given string, then the function returns -1 index(str, start, end) Same as find() but raises an exception if the substring is not present in the given string endswith() Returns True if the given string ends with the supplied substring otherwise returns False startswith() Returns True if the given string starts with the supplied substring otherwise returns False isalnum() Returns True if characters of the given string are either alphabets or numeric. If whitespace or special symbols are part of the given string or the string is empty it returns False islower() Returns True if the string is non-empty and has all lowercase alphabets, or has at least one character as lowercase alphabet and rest are non-alphabet characters 9 Page isupper() Returns True if the string is non-empty and has all uppercase alphabets, or has at least one character as uppercase character and rest are non-alphabet characters isspace() Returns True if the string is non-empty and all characters are white spaces (blank, tab, newline, carriage return) istitle() Returns True if the string is non-empty and title case, i.e., the first letter of every word in the string in uppercase and rest in lowercase lstrip() Returns the string after removing the spaces only on the left of the string rstrip() Returns the string after removing the spaces only on the right of the string strip() Returns the string after removing the spaces both on the left and the right of the string replace (oldstr, newstr) Replaces all occurrences of old string with the new string join() Returns a string in which the characters in the string have been joined by a separator partition ( ) Partitions the given string at the first occurrence of the substring (separator) and returns the string partitioned into three parts. 1. Substring before the separator 2. Separator 3. Substring after the separator If the separator is not found in the string, it returns the whole string itself and two empty strings split() Returns a list of words delimited by the specified substring. If no delimiter is given then words are separated by space. LIST List is an ordered sequence, which is used to store multiple data at the same time. List contains a sequence of heterogeneous elements. Each element of a list is assigned a number to its position or index. The first index is 0 (zero), the second index is 1 , the third index is 2 and so on. Creating a List In Python, a = [ 34 , 76 , 11,98 ] b=['s',3,6,'t'] d=[] Creating List From an Existing Sequence: list ( ) method is used to create list from an existing sequence. Syntax: new_list_name = list ( sequence / string ) You can also create an empty list. eg. a = list ( ). Similarity between List and String len ( ) function is used to return the number of items in both list and string. Membership operators as in and not in are same in list as well as string. Concatenation and replication operations are also same done in list and string. Difference between String and List Strings are immutable which means the values provided to them will not change in the program. Lists are mutable which means the values of list can be changed at any time. Accessing Lists To access the list's elements, index number is used. S = [12,4,66,7,8,97,”computer”,5.5,] 0 1 2 3 4 5 6 7 >>> S 97 12 4 66 7 8 97 computer 5.5 >>>S[-2] -8 -7 -6 -5 -4 -3 -2 -1 computer 10 Page Traversing a List Traversing a list is a technique to access an individual element of that list. 1. Using for loop for loop is used when you want to traverse each element of a list. >>> a = [‘p’,’r’,’o’,’g’,’r’,’a’,’m’] >>> fot x in a: print(x, end = ‘ ‘) output : p r o g r a m 2. Using for loop with range( ) >>> a = [‘p’,’r’,’o’,’g’,’r’,’a’,’m’] >>> fot x in range(len(a)): print(x, end = ‘ ‘) output : p r o g r a m List Operations 1. Concatenate Lists List concatenation is the technique of combining two lists. The use of + operator can easily add the whole of one list to other list. Syntax list list1 + list2 e.g. >>> L1 = [ 43, 56 , 34 ] >>> L2 = [ 22 , 34 , 98 ] 2. Replicating List >>> L = 11 + 12 Elements of the list can be replicated using * operator. >>> L [ 43, 56, 34, 22 , 34 , 98 ] Syntax list = listl * digit e.g. >>> L1 = [ 3 , 2 , 6 ] >>> L = 11 * 2 >>> L [ 3 , 2 , 6 , 3 , 2 , 6 ] 3. Slicing of a List: List slicing refers to access a specific portion or a subset of the list for some operation while the original list remains unaffected. Syntax:- list_name [ start: end ] Syntax: list_name [ start: stop : step ] >>> List1 = [ 4 , 3 , 7 , 6 , 4 , 9 ,5,0,3 , 2] >>> List1 = [ 4 , 3 , 7 , 6 , 4 , 9 ,5,0,3 , 2] >>> S = List1[ 2 : 5 ] >>> S = List1[ 1 : 9 : 3 ] >>> S >>> S [ 7, 6, 4 ] [ 3, 4, 0 ] List Manipulation Updating Elements in a List List can be modified after it created using slicing e.g. >>> l1= [ 2 , 4, " Try " , 54, " Again " ] >>> l1[ 0 : 2 ] = [ 34, " Hello " ] >>> l1 [34, ' Hello ', ' Try ', 54, ' Again '] >>> l1[ 4 ] = [ "World " ] >>> l1 [34, ' Hello ', ' Try ', 54, ['World ']] Deleting Elements from a List del keyword is used to delete the elements from the list. Syntax:- del list_name [ index ] # to delete individual element del 11st_name [ start : stop ] # to delete elements in list slice c.g. >>> list1 = [ 2.5 , 4 , 7 , 7 , 7 , 8 , 90 ] >>> del list1 >>> del list1[2:4] >>> list1 >>> list1 [2.5, 4, 7, 7, 8, 90] [2.5, 4, 8, 90] BUILT IN LIST METHODS Method Description len() Returns the length of the list passed as the argument list() Creates an empty list if no argument is passed Creates a list if a sequence is passed as an argument 11 Page append() Appends a single element passed as an argument at the end of the list extend() Appends each element of the list passed as argument to the end of the given list insert() Inserts an element at a particular index in the list count() Returns the number of times a given element appears in the list index() Returns index of the first occurrence of the element in the list. If the element is not present, ValueError is generated remove() Removes the given element from the list. If the element is present multiple times, only the first occurrence is removed. If the element is not present, then ValueError is generated pop() Returns the element whose index is passed as parameter to this function and also removes it from the list. If no parameter is given, then it returns and removes the last element of the list reverse() Reverses the order of elements in the given list sort() Sorts the elements of the given list in-place sorted() It takes a list as parameter and creates a new list consisting of the same elements arranged in sorted order min() Returns minimum or smallest element of the list max() Returns maximum or largest element of the list sum() Returns sum of the elements of the list TUPLES A tuple is an ordered sequence of elements of different data types. Tuple holds a sequence of heterogeneous elements, it store a fixed set of elements and do not allow changes Tuple vs List Elements of a tuple are immutable whereas elements of a list are mutable. Tuples are declared in parentheses ( ) while lists are declared in square brackets [ ]. Iterating over the elements of a tuple is faster compared to iterating over a list. Creating a Tuple To create a tuple in Python, the elements are kept in parentheses ( ), separated by commas. a = ( 34 , 76 , 12 , 90 ) b=('s',3,6,'a') Accessing tuple elements, Traversing a tuple, Concatenation of tuples, Replication of tuples and slicing of tuples works same as that of List BUILT IN TUPLE METHODS Method Description len() Returns the length or the number of elements of the tuple passed as Argument tuple() Creates an empty tuple if no argument is passed. Creates a tuple if a sequence is passed as argument count() Returns the number of times the given element appears in the tuple index() Returns the index of the first occurance of a given element in the tuple sorted() Takes elements in the tuple and returns a new sorted list. It should be noted that, sorted() does not make any change to the original tuple min() Returns minimum or smallest element of the tuple max() Returns maximum or largest element of the tuple sum() Returns sum of the elements of the tuple 12 Page DICTIONARY Dictionary is an unordered collection of data values that store the key : value pair instead of single value as an element. Keys of a dictionary must be unique and of immutable data types such as strings, tuples etc. Dictionaries are also called mappings or hashes or associative arrays Creating a Dictionary To create a dictionary in Python, key value pair is used. Dictionary is list in curly brackets , inside these curly brackets , keys and values are declared. Syntax dictionary_name = { key1 : valuel , key2 : value2... } Each key is separated from its value by a colon ( :) while each element is separated by commas. >>> Employees = { " Abhi " : " Manger " , " Manish " : " Project Manager " , " Aasha " : " Analyst " , " Deepak " : " Programmer " , " Ishika " : " Tester "} Accessing elements from a Dictionary Syntax: dictionary_name[keys] >>> Employees[' Aasha '] ' Analyst ' Traversing a Dictionary 1. Iterate through all keys 2. Iterate through all values >>> for i in Employees: >>> for i in Employees: print(i) print(Employees[i]) Output: Output: Abhi Manger Manish Project Manager Aasha Analyst Deepak Programmer Ishika Tester 3. Iterate through key and values 4. Iterate through key and values simultaneously >>> for i in Employees: >>> for a,b in Employees.items(): print(i, " : ", Employees[i]) print("Key = ",a," and respective value = ",b) Output: Output: Abhi : Manger Key = Abhi and respective value = Manger Manish : Project Manager Key = Manish and respective value = Project Manager Aasha : Analyst Key = Aasha and respective value = Analyst Deepak : Programmer Key = Deepak and respective value = Programmer Ishika : Tester Key = Ishika and respective value = Tester Adding elements to a Dictionary Syntax: dictionary_name[new_key] = value >>> Employees['Neha'] = "HR" >>> Employees {' Abhi ': ' Manger ', ' Manish ': ' Project Manager ', ' Aasha ': ' Analyst ', ' Deepak ': ' Programmer ', ' Ishika ': ' Tester ', 'Neha': 'HR'} Updating elements in a Dictionary Syntax: dictionary_name[existing_key] = value >>> Employees['Neha'] = " Progammer " >>> Employees {' Abhi ': ' Manger ', ' Manish ': ' Project Manager ', ' Aasha ': ' Analyst ', ' Deepak ': ' Programmer ', ' Ishika ': ' Tester ', 'Neha': ' Progammer '} Membership operators in Dictionary Two membership operators are in and not in. The membership operator inchecks if the key is present in the dictionary >>> " Ishika " in Employees >>> ' Analyst ' not in Employees True True 13 Page BUILT IN DICTIONARY METHODS Metho Description d len() Returns the length or number of key: value pairs of the dictionary dict() Creates a dictionary from a sequence of key-value pairs keys() Returns a list of keys in the dictionary values() Returns a list of values in the dictionary items() Returns a list of tuples(key – value) pair get() Returns the value corresponding to the key passed as the argument If the key is not present in the dictionary it will return None update() appends the key-value pair of the dictionary passed as the argument to the key- value pair of the given dictionary del() Deletes the item with the given key To delete the dictionary from the memory we write: del Dict_name clear() Deletes or clear all the items of the dictionary MIND MAP List is an ordered sequence Method of heterogeneous elements len() List is created using [ ] list() bracket append() Individual character in a list extend() can be assessed using index insert() Lists are mutable count() index() remove() List Osperations Concatination Operator (+) LIST pop() reverse() Replication Operators (*) sort() Comparison Operators ( == , sorted() > , < , < = , > = , !=) min() Membership Operators (in max() & not in sum() List supports slicing Tuple is an ordered sequence of heterogeneous elements Tuple is created using ( ) bracket Method Individual character in a tuple can be assessed using index len() Tuples are immutable tuple() count() TUPLE index() Tuple Operations sorted() Concatination Operator (+) min() Replication Operators (*) max() Comparison Operators ( == , > , < , sum() < = , > = , !=) Membership Operators (in & not in 14 Tuples supports slicing Page MIND MAP Dictionary is an unordered Method collection of data values that store len() the key : value pair dict() Keys of a dictionary must be keys() unique and of immutable data types Dictionary is created using { } DICTIONARY values() bracket items() Individual character in a dictionary get() can be assessed using keys update() Membership Operators (in & not in del() checks if the key is present clear() Enclosed by single, double or Method triple quotes len() Individual character in a string title() can be assessed using index lower() Strings are immutable upper() count(str, start, end) find(str,start, end) index(str, start, end) STRING endswith() startswith() String Operations isalnum() Concatination Operator islower() (+) isupper() Replication Operators isspace() (*) istitle() Comparison Operators ( lstrip() == , > , < , < = , > = , rstrip() !=) strip() Membership Operators replace(oldstr, newstr) (in & not in join() partition()) String supports slicing split() QUESTIONS: 1 MARK QUESTIONS 1. What will be the output of the following set of commands >>> str = "hello" >>> str[:2] a. lo b. he c. llo d. el 2. Which type of object is given below >>> L = 1,23,"hello",1 a. list b. dictionary c. array d. tuple 3. Which operator tells whether an element is present in a sequence or not a. exist b. in c. into d. inside 15 Page 4. Suppose a tuple T is declared as T = (10,12,43,39), which of the following is incorrect a. print(T) b. T = -2 c. print(max(T)) d. print(len(T)) 5. Which index number is used to represent the last character of a string a. -1 b. 1 c. n d. n – 1 6. Which function returns the occurrence of a given element in a list? a. len() b. sum() c. extend() d. count() 7. which type of slicing is used to print the elements of a tuple in reverse order a. [:-1] b. [: : -1] c. [1 : :] d. [: : 1] 8. Dictionaries are also called a. mapping b. hashes c. associative array d. all of these 9. Which function returns the value of a given key, if present, from a dictionary? a. items() b. get() c. clear() d. keys() 10. The return type of input() function is: a. list b. integer c. string d. tuple ANSWERS 1 B 6 d 2 D 7 b 3 B 8 d 4 B 9 b 5 A 10 c 2 MARKS QUESTIONS Q1. Rewrite the following code in python after removing all syntax error(s). Underline each correction done in the code. STRING=""WELCOME NOTE"" for S in range[0,8]: print (STRING(S)) Q2. Find output generated by the following code: Str=”Computer” Str=Str[-4:] print(Str*2) Q3. What will be the output of the following question L = [10,19,45,77,10,22,2] i) L.sort() ii) max(L) print(L) Q4. Find the output L = [10,19,45,77,10,22,2] i) L[3:5] ii) L[: : -2] Q5. Distinguish between list and tuple. Q6. Read the code given below and show the keys and values separately. D = {‘one’ : 1, ‘two’ : 2, ‘three’ : 3} Q7. Observe the given list and answer the question that follows. List1 = [23,45,63, ‘hello’, 20, ‘world’,15,18] i) list1[-3] ii) list1 Q8. Assertion (A) : s = [11, 12, 13, 14] s = 15 Reasoning (R) : List is immutable. (A) Both A and R are true and R is the correct explanation of assertion. (B) A and R both are true but R is not the correct explanation of A. (C) A is true, R is false. (D) A is false, R is true. 16 Page Q9. a=(1,2,3) a=4 Assertion: The above code will result in error Reason: Tuples are immutable. So we can’t change them. (A) Both Assertion and reason are true and reason is correct explanation of assertion. (B) Assertion and reason both are true but reason is not the correct explanation of assertion. (C) Assertion is true, reason is false. (D) Assertion is false, reason is true. ANSWERS Q1. CORRECTED CODE:- STRING= "WELCOME" NOTE=" " for S in range (0, 7) : print (STRING [S]) Also range(0,8) will give a runtime error as the index is out of range. It shouldbe range(0,7) Q2. Q3. Q4. uter [2, 10, 10, 19, 22, 45, 77] [77, 10] ‘ComputerComputer’ [2, 10, 45, 10] Q5. List Tuple Elements of a list are mutable Elements of tuple are immutable List is declared in square brackets [] Tuple is declared in parenthesis () Iterating over elements in list is slower as Iterating over elements of tuples is faster as compared to tuple compared to list e.g L1 = [1,2,3] e.g T1 = (1,2,3) Q6. Q7. Q8. (C) Q9. (A) Keys: ‘one’, ‘two’, ‘three’ ‘world’ Values: 1,2,3 ‘hello’ 3 MARKS QUESTIONS Q1. Which of the string built in methods are used in following conditions? ii) Returns the length of a string iii) Removes all leading whitespaces in string iv) Returns the minimum alphabetic character from a string Q2. Write a program to remove all the characters of odd index value in a string Q3. Write a python program to count the frequencies of each elements of a list using dictionary Q4. what will be the output of the following python code L = [10,20] L1 = [30,40] L2 = [50,60] L.append(L1) print(L) L.extend(L2) print(L) print(len(L) Q5. Find the output of the given question t = (4,0,’hello’,90,’two’,(‘one’,45),34,2) i) t ii) t[3:7] iii) t + t[-2] 17 Page ANSWERS Q1. i) len() ii) lstrip() iii)min() Q2. str = input(“Enter a string “) final = “ “ For i in range(len(str)): if (i%2 == 0): final = final + str[i] print(“The modified string is “,final) Q3. L1 = [] n = int(input(“Enter number of elements of the list “)) for i in range(0,n): ele = int(input()) L1.append(ele) print(“Original list = “,L1) print(“Elements of list with their frequencies : “) freq ={} for item in L1: if item in freq: freq[item] += 1 else: freq[item] = 1 for k,v in freq.item(): print(“Element”, k, “frequency”, v) Q4. Q5. [10, 20, [30, 40]] i) (‘one’,45) [10, 20, [30, 40],50,60] ii) (90,’two’,’(‘one’,45),34) iii) 34 4 MARKS QUESTIONS Q1. Find the output i) 'python'.capitalize() ii) max('12321') iii) 'python'.index('ho') iv) 'python'.endswith('thon') Q2. Consider the following code and answer the question that follows. book = {1:'Thriller',2:'Mystery',3:'Crime',4:'Children Stories'} library = {5:'Madras Diaries',6:'Malgudi Days'} v) Ramesh wants to change the book ‘Crime’ to ‘Crime Thriller’. He has written the following code: book['Crime'] = 'Crime Thriller' but he is not getting the answer. Help him to write the correct command. vi) Ramesh wants to merge the dictionary book with the dictionary library. Help him to write the command. Q3. Write the suitable method names for the conditions given below: i) Add an element at the end of the list ii) Return the index of first occurrence of an element iii) Add the content of list2 at the end of list1 iv) Arrange the elements of a list1 in descending order ANSWERS Q1. i) 'Python' ii) '3' iii) 3 iv) True 18 Page Q2. i) book = 'Crime Thriller' ii) library.update(book) Q3. i) append() ii) index() iii) list1.extend(list2) iv) list1.sort(reverse = True) 5 MARKS QUESTIONS Q1. Find the output of the following code: a = (5,(7,5,(1,2)),5,4) print(a.count(5)) print(a) print(a * 3) print(len(a)) b = (7,8,(4,5)) print(a + b) Q2. Following is a program to check a list is same if it is read from front or from back. Observe the program and answer the following questions: a = [1,2,3,3,2,1] i= # statement 1 mid = (len(a)) / 2 same = True while : # statement 2 if a[i] != : # statement 3 print(“NO”) same = False break # statement 4 if same == : # statement 5 print(“YES”) vii) Which value will be assigned to the variable I in statement 1? viii) Fill the blank line in statement 2. ix) Fill the blank line in statement 3. x) Fill the blank line in statement 4. xi) Fill the blank line in statement 5. Q3. Explain the following string functions with examples. i) title() ii) count( ) iii) find() iv) index() v) join() ANSWERS Q1. 2 (1, 2) (5, (7, 5, (1, 2)), 5, 4, 5, (7, 5, (1, 2)), 5, 4, 5, (7, 5, (1, 2)), 5, 4) 4 (5, (7, 5, (1, 2)), 5, 4, 7, 8, (4, 5)) Q2. i) 0 ii) i < mid iii) a[i] != a[len(a) – i – 1] iv) i = i + 1 v) True Q3. i) title() Returns the string with first letter of every word in the string in uppercase and rest in lowercase. 19 Page >>> str1 = 'hello WORLD!' >>> str1.title() 'Hello World!' ii) count( ) Returns number of times substring str occurs in the given string. If we do not give start index and end index then searching starts from index 0 and ends at length of the string. >>> str1 = 'Hello World! Hello Hello' >>> str1.count('Hello',12,25) 2 >>> str1.count('Hello') 3 iii) find() Returns the first occurrence of index of substring stroccurring in the given string. If we do not give start and end then searching starts from index 0 and ends at length of the string. If the substring is not present in the given string, then the function returns -1 >>> str1= 'Hello World! Hello Hello' >>> str1.find('Hello',10,20) 13 >>> str1.find('Hello',15,25) 19 >>> str1.find('Hello') 0 >>> str1.find('Hee') -1 iv) index( ) Same as find() but raises an exception if the substring is not present in the given string >>> str1 = 'Hello World! Hello Hello' >>> str1.index('Hello') 0 >>> str1.index('Hee') ValueError: substring not found v) join() Returns a string in which the characters in the string have been joined by a separator >>> str1 = ('HelloWorld!') >>> str2 = '-' #separator >>> str2.join(str1) 'H-e-l-l-o-W-o-r-l-d-!' 20 Page FUNCTION IN PYTHON Functions: types of function (built-in functions, functions defined in module, user defined functions), creating user defined function, arguments and parameters, default parameters, positional parameters, function returning value(s), flow of execution, scope of a variable (global scope, local scope) Let us revise A function is a block of code that performs a specific task. Advantages of function: Reusability of code, Reduce size of code, minimum number of statements, minimum storage, Easy to manage and maintain Types of functions: Built-in-functions, Functions defined in module, User defined function Built-in functions are the functions whose functionality is pre-defined in python like abs(), eval(), input(), print(), pow() Some functions are defined inside the module like load() and dump() function defined inside the pickle module. A function that can be defined by the user is known as user defined function. def keyword is used to define a function. There is a colon at the end of def line, meaning it requires block User Defined function involved two steps: defining calling Syntax for user defined function: def ( [parameter list ]): [””function’s doc string ””] [] Python supports three types of formal arguments/ parameters: Positional Arguments, Default parameters, Keyword (or named ) Arguments Positional Arguments: When the function call statement must match the number and order of arguments as defined in the function definition, this is called the positional argument matching. A parameter having default value in the function header is known as a default parameter. Keyword Arguments are the named arguments with assigned values being passed in the function call statement. A function may or may not return one or more values. A function that does not return a value is known as void function and returns legal empty value None. Functions returning value are also known as fruitful functions. The flow of execution refers to the order in which statements are executed during a program. A variable declared in a function body (block) is said to have local scope. i.e. it can be accessed within this function. A variable declared outside of all functions/top level of segment of a program is said to have global scope. i.e. it can be accessible in whole program and all blocks ( functions and the other blocks contained within program. 21 Page MIND MAP ON FUNCTION Function: A function is a group of statements that exists within a program for the purpose of performing a specific task. Types Scope: Scope of a variable is the area of the program where it may be referenced. Built-in-functions: User Defined Bulit-in functions are Function defined in module: A module is functions: A function the predefined is a block of code which functions that are a file containing functions and only runs when it is already available in called. In Python, a variables defined in Local: A variable the python. Ex- int(), function is defined separate files. created inside a using the def keyword. function belongs to the local scope of that function, and can only be used Global: A variable declared inside that function. outside of all functions/top Ex- level of segment of a program is said to have global scope. i.e. x = 300 it can be accessible in whole def myfunc(): program and all blocks. print(x) myfunc() Ex- print(x) def myfunc(): x = 300 print(x) myfunc() Types of Arguments/Parameters Default Arguments: A Keyword Arguments: Positional Arguments: default argument is an Value can be provided by using argument that assumes a their name instead of the position Arguments passed to a default value if a value is not function in correct positional (order) in function call statement. provided in the function call These are called keyword order. for that argument. arguments. 22 Page Multiple Choice Questions (1 Mark) 1. What is the default return value for a function that does not return any value explicitly? (a) None (b) int (c) double (d) null 2. Which of the following items are present in the function header? (a) Function name only (b) Parameter list only (c) Both function name and parameter list (d) return value 3. Which of the following keyword marks the beginning of the function block? (a) func (b) define (c) def (d) function 4. Pick one of the following statements to correctly complete the function body in the given code snippet. def f(number): # Missing function body print (f(5)) (a) return “number” (b) print(number) (c) print(“number”) (d) return number 5. Which of the following function header is correct? (a) def f(a=1,b): (b) def f(a=1,b,c=2): (c) def f(a=1, b=1, c=2): (d) def f(a=1,b=1,c=2,d); 6. Which of the following statements is not true for parameter passing to functions? (a) You can pass positional arguments in any order. (b) You can pass keyword arguments in any order. (c) You can call a function with positional and keyword arguments. (d) Positional arguments must be before keyword arguments in a function call 7. A variable defined outside all the functions referred to as……….. (a) A static variable (b)A global variable (c) A local variable (d) An automatic variable 8. What is the order of resolving scope of a name in a python program? (L: Local namespace, E: Enclosing namespace, B: Built-In namespace, G: Global namespace) (a) BGEL (b) LEGB (c) GEBL (d) LBEG 9. Assertion (A):- If the arguments in a function call statement match the number and order of arguments as defined in the function definition, such arguments are called positional arguments. Reasoning (R):- During a function call, the argument list first contains default argument(s) followed by positional argument(s). (a) Both A and R are true and R is the correct explanation for A (b) Both A and R are true and R is not the correct explanation for A (c) A is True but R is False (d) A is false but R is True 10. The......................... refers to the order in which statements are executed during a program run. (a) Token (b) Flow of execution (c) Iteration (d) All of the above 11. Choose the correct option: Statement1: Local Variables are accessible only within a function or block in which it is declared. Statement2: Global variables are accessible in the whole program. (a) Statement1 is correct but Statement2 is incorrect (b) Statement2 is correct but Statement1 is incorrect (c) Both Statements are Correct (d) Both Statements are incorrect 12. The............................ of a variable is the area of the program where it may be referenced a) external b) global c) scope d) local 23 Page Answers Questions Answers 1. (a) None 2. (c) Both function name and parameter list 3. (c) def 4. (d) return number 5. (c) def f(a=1, b=1, c=2): 6. (a) You can pass positional arguments in any order. 7. (b) A global variable 8. (b) LEGB 9. (c) A is True but R is False 10. (b) Flow of execution 11. (c) Both Statements are Correct 12. c) scope Short Questions (2 Marks) Q1. What do you mean by a function? How is it useful? Answer: A function is a block of code that performs a specific task. Functions are useful as they can be reused anywhere through their function call statements. Q2. Observe the following Python code very carefully and rewrite it after removing all syntactical errors with each correction underlined. def execmain(): x = input("Enter a number:") if (abs(x)= x): print("You entered a positive number") else: x=*-1 print("Number made positive : ",x) execmain() Answer: def execmain(): x = int(input("Enter a number:")) if (abs(x)== x): print("You entered a positive number") else: x*=-1 print("Number made positive : ",x) execmain() Q3. What is an argument? Give an example. Answer: An argument is data passed to a function through function call statement. It is also called actual argument or actual parameter. For example, in the statement print(math.sqrt(25)), the integer 25 is an argument. Q4. What is the output of the program given below? x = 75 def func (x) : x = 10 func (x) print ('x is now', x) Answer: x is now 75 Q5. What will be the output of the following code: 24 Page total=0 def add(a,b): global total total=a+b print(total) add(6,6) print(total) Answer: 12 12 Q6. Is return statement optional? Compare and comment on the following two return statements: (i) return (ii) return val Answer: The return statement is optional only when the function does not return a value. A function that returns a value must have at least one return statement. From the given two return statements, (i) The statement return is not returning any value. Rather it returns the control to caller along with empty value None. (ii) The statement return val is returning the control to caller along with the value contained in variable val. Q7. Divyansh, a python programmer, is working on a project which requires him to define a function with name CalculateInterest(). He defines it as: def CalculateInterest(Principal,Rate=.06, Time): # Code But this code is not working, Can you help Divyansh to identify the error in the above function and with the solution? Answer. Yes, here non-default argument is followed by default argument which is wrong as per python’s syntax. (while passing default arguments to a function ,all the arguments to its right must also have default values, otherwise it will result in an error.) Q8. Write a function that takes a positive integer and returns the one’s position digit of the integer. Answer: def getOnes(num): oneDigit=num%10 # return the ones digit of the integer num return oneDigit Q9. Anita has written a code to input a number and check whether it is prime or not. His code is having errors. Rewrite the correct code and underline the corrections made. def prime(): n=int(input("Enter number to check :: ") for i in range (2, n//2): if n%i=0: print("Number is not prime \n") break else: print("Number is prime \n’) Answer: def prime(): n=int(input("Enter number to check :: ")) #bracket missing for i in range (2, n//2): if n%i==0: # = missing print("Number is not prime \n") break #wrong indent else: 25 print("Number is prime \n”) # quote mismatch Page Q10. What is the difference between parameter and argument? Answer: Parameters are temporary variable names within functions. The argument can be thought of as the value that is assigned to that temporary variable. For instance, let’s consider the following simple function to calculate sum of two numbers. def sum(a,b): return a+b sum(10,20) Here a, b are the parameters for the function ‘sum’. Arguments are used in procedure calls, i.e., the values passed to the function at runtime.10, 20 are the arguments for the function sum. Q11. What is the significance of having functions in a program? Answer: Creating functions in programs is very useful. It offers the following advantages: (i) The program is easier to understand. (ii) Redundant code is at one place, so making changes is easier. (iii) Reusable functions can be put in a library in modules. Q12. What is the difference between local variable and global variable? Also give a suitable Python code to illustrate both. Answer: S.No. Local Variable Global Variable 1. It is a variable which is declared within a It is a variable which is declared function or within a block. outside all the functions or in a global space. 2. It cannot be accessed outside the function It is accessible throughout the but only within a function/block of a program in which it is declared. program. For example, in the following code x, xcubed are global variables and n and cn are local variables. def cube(n): # n and cn are local variables cn=n*n*n return cn x=10 # x is a global variable xcubed=cube(x) # xcubed is a global variable print(x, “Cubed 15”, xcubed) Short Questions (3 Marks) Q1. Consider the following function that takes two positive integer parameters a and b. Answer the following questions based on the code below: def funct1(a,b): if a>1: if a%b==0: print (b, end=’ ‘) funct1(int(a/b),b) else: funct1(a,b+1) (a) What will be printed by the fuction call funct1(24,2)? (b) What will be printed by the fuction call funct1(84,2)? 26 Page (c) State in one line what funct1()is trying to calculate. Answer (a) 2 2 2 3 (b)2 2 3 7 (c) finding factors of A which are greater than and equal to B. Q2. Write a user defined function to print the odd numbers from a given list passed as an argument. Sample list: [1,2,3,4,5,6,,7,8,9,10] Expected Result: [1,3,5,7,9] Answer: def odd_num(l): odd=[] for n in l: if n%2!=0: odd.append(n) return odd print(odd_num([1,2,3,4,5,6,7,8,9,10])) Q3. Which line in the given code(s) will not work and why? def interest(p,r,t=7): I=(p*r*t) print(interest(20000,.08,15)) #line 1 print(interest(t=10, 20000, 0.75)) #line 2 print(interest(50000, 0.7)) #line 3 print(interest(p=10000,r=.06,time=8)) #line 4 print(interest(80000, t=10)) #line 5 Answer: Line 2: positional argument must not be followed by keyword argument, i.e., positional argument must appear before a keyword argument. Line 4: There is no keyword argument with name ‘time’ Line 5: Missing value for positional arguments, R. Q4. Write a python function showlarge() that accepts a string as parameter and prints the words whose length is more than 4 characters. Eg: if the given string is “My life is for serving my Country” The output should be serving Country Answer: def showlarge(s): l = s.split() for x in l: if len(x)>4: print(x) s=" My life is for serving my Country " showlarge(s) Q5. Write a function Interchange (num) in Python, which accepts a list num of integers, and interchanges the adjacent elements of the list and print the modified list as shown below: (Number of elements in the list is assumed as even) Original List: num = [5,7,9,11,13,15] After Rearrangement num = [7,5,11,9,15,13] Answer: def Interchange(num): for i in range(0,n,2): 27 Page num[i], num[i+1] = num[i+1], num[i] print(num) num=[5,7,9,11,13,15] n=len(num) if n%2==0: Interchange(num) Q6. Write a function INDEX_LIST(L), where L is the list of elements passed as argument to the function. The function returns another list named ‘indexList’ that stores the indices of all Non-Zero Elements of L. For example: If L contains [12, 4, 0, 11,0, 56] The indexList will have - [0,1,3,5] Answer: def INDEX_LIST(L): indexList=[] for i in range(len(L)): if L[i]!=0: indexList.append(i) return indexList Long Questions/Case Study/Application Based (4 Marks) Q1. Krishnav is looking for his dream job but has some restrictions. He loves Delhi and would take a job there if he is paid over Rs.40,000 a month. He hates Chennai and demands at least Rs. 1,00,000 to work there. In any another location he is willing to work for Rs. 60,000 a month. The following code shows his basic strategy for evaluating a job offer. def DreamProject(): pay= location= if location == "Mumbai": print ("I’ll take it!") #Statement 1 elif location == "Chennai": if pay < 100000: print ("No way") #Statement 2 else: print("I am willing!") #Statement 3 elif location == "Delhi" and pay > 40000: print("I am happy to join") #Statement 4 elif pay > 60000: print("I accept the offer") #Statement 5 else: print("No thanks, I can find something better") #Statement 6 On the basis of the above code, choose the right statement which will be executed when different inputs for pay and location are given (i) Input: location = “Chennai”, pay = 50000 a. Statement 1 b. Statement 2 c. Statement 3 d. Statement 4 (ii) Input: location = “Surat” ,pay = 50000 a. Statement 2 b. Statement 4 c. Statement 5 d. Statement 6 (iii) Input- location = “Any Other City”, pay = 1 a Statement 1 b. Statement 2 c. Statement 4 d. Statement 6 28 Page (iv) Input location = “Delhi”, pay = 500000 a. Statement 6 b. Statement 5 c. Statement 4 d. Statement 3 Answer: (i) b. Statement2 (ii) d. Statement6 (iii) d. Statement6 (iv) c. Statement4 Q2. Kids Elementary is a Playway school that focuses on “Play and learn” strategy that helps toddlers understand concepts in a fun way. Being a senior programmer, you have taken responsibility to develop a program using user-defined functions to help children differentiate between upper case and lower case letters/ English alphabet in a given sequence. Make sure that you perform a careful analysis of the type of alphabets and sentences that can be included as per age and curriculum. Write a python program that accepts a string and calculates the number of upper case letters and lower case letters. Answer: def string_test(s): d={"UPPER_CASE":0,"LOWER_CASE":0} for c in s: if c.isupper(): d["UPPER_CASE"]+=1 elif c.islower(): d["LOWER_CASE"]+=1 else: pass print("Original String:",s) print("No. of Upper Case Characters:",d["UPPER_CASE"]) print("No. of Lower Case Characters:",d["LOWER_CASE"]) string_test("Play Learn and Grow") Case Based/ Source Based Integrated Questions (5 Marks) Q1. Traffic accidents occur due to various reasons. While problems with roads or inadequate safety facilities lead to some accidents, majority of the accidents are caused by driver’s carelessness and their failure to abide by traffic rules. ITS Roadwork is a company that deals with manufacturing and installation of traffic lights so as to minimize the risk of accidents. Keeping in view the requirements, traffic simulation is to be done. Write a program in python that simulates a traffic light. The program should perform the following: (a) A user defined function trafficLight() that accepts input from the user, displays an error message if the following is displayed depending upon return value from light(); (i) “STOP, Life is more important than speed”, if the value returned by light() is 0. (ii) “PLEASE GO SLOW”, if the value returned by light() is 1. (iii) “You may go now”, if the value returned by light() is 2. (b) A user defined function light() that accepts a string as input and returns 0 when the input is RED, 1 when the input is YELLOW and 2 when the input is GREEN. The input should be passed as an argument. (c) Display “BETTER LATE THAN NEVER” after the function trafficLight() is executed. Answer: (a) #Program to simulate a traffic light comprising of two user defined functions trafficLight() and light() def trafficLight(): signal=input("Enter the colour of the traffic light:") 29 Page if signal not in ["RED","YELLOW","GREEN"]: print("Please enter a valid traffic light colour in CAPITALS") else: value=light(signal) if value==0: print("STOP","Life is more important thanspeed") elif value==1: print("PLEASE GO SLOW") else: print("You may go now") (a) # function light() def light(colour): if colour=="RED": return 0 elif colour=="YELLOW": return 1 else: return 2 trafficLight() # Display BETTER LATE THAN NEVER print("BETTER LATE THAN NEVER") Q2. Observe the following code and select appropriate answers for the given questions: total=1 def multiply(l): # line1 for x in l: total # line2 total*=x return # line3 l=[2,3,4] print(multiply( ), end=” “) # line4 print(“ , Thank You”) (i) Identify the part of function in # line1 (a) Function header (b) Function calling (c) Return statement (d)Default argument (ii) Which of the keyword is used to fill in the blank for # line2 to run the program without error. (a) eval (b) def (c) global (d) return (iii) Which variable is going to be returned in # line3 (a) total (b) x (c) l (d) None (iv) Which variable is required in the # line4 (a) total (b) x (c) l (d) None (v) In the # line4 the multiple(l) is called (a) Caller (b) Called (c) Parameters (d) Arguments Answer: (i) (a) Function header (ii) (c) global (iii) (a) total (iv) (c) l (v) (a) Caller 30 Page Exception Handling Exception: Contradictory or Unexpected situation or unexpected error, during program execution, is known as Exception. Exception Handling: Way of handling anomalous situations in a program-run, is known as Exception Handling. Some common examples of Exceptions are: Divide by zero errors Hard disk crash Accessing the elements of an array beyond its range Opening a non-existent file Invalid input Heap memory exhausted Example: try : print ("result of 10/5 = ", (10/5)) print ("result of 10/0 = ", (10/0)) except : print ("Divide by Zero Error! Denominator must not be zero!") The output produced by above code is as shown below : result of 10 / 5 = 2 result of 10 / 0 = Divide by Zero Error! Denominator must not be zero! 1 Marks Question: 1. Errors resulting out of violation of programming language’s grammar rules are known as: (a) Compile time error (b) Logical error (c) Runtime error (d) Exception 2. An unexpected event that occurs during runtime and causes program disruption, is called: (a) Compile time error (b) Logical error (c) Runtime error (d) Exception 3. Which of the following keywords are not specific to exception handling ? (a) try (b) except (c) finally (d) else 4. Which of the following blocks is a ‘must-execute’ block ? (a) try (b) except (c) finally (d) else 5. Which keyword is used to force an exception ? (a) try (b) except (c) raise (d) finally Answers: 1. (a) 2. (d) 3. (d) 4. (c) 5. (c) Predict the output of the following code for these function calls: (a) divide(2, 1) (b) divide(2, 0) (c) divide(“2”, “1”) def divide(x, y): try: result = x/y except ZeroDivisionError: print ("division by zero!") else: print ("result is", result) finally: print ("executing finally clause") Solution. (a) divide(2, 1) result is 2 executing finally clause (b) divide(2, 0) division by zero! executing finally clause (c) divide(“2”, “1”) executing finally clause Traceback (most recent call last): TypeError: unsupported operand type(s) for /: 'str' and 'str' 31 Page FILE HANDLING – TEXT FILES I. INTRODUCTION: Files are named locations on disk to store related information. They are used to permanently store data in a non-volatile memory (e.g. hard disk). Since Random Access Memory (RAM) is volatile (which loses its data when the computer is turned off), we use files for future use of the data by permanently storing them. When we want to read from or write to a file, we need to open it first. When we are done, it needs to be closed so that the resources that are tied with the file are freed. Hence, in Python, a file operation takes place in the following order: – Open a file – Read or write (perform operation) – Close the file II Types of File in Python There are two types of files in Python and each of them are explained below in detail with examples for your easy understanding. They are: 1) Binary file 2) Text file II. Text files in Python A text file is usually considered as sequence of lines. Line is a sequence of characters (ASCII), stored on permanent storage media. Although default character coding in python is ASCII but supports Unicode as well. In text file, each line is terminated by a special character, known as End of Line (EOL). From strings we know that \n is newline character. At the lowest level, text file is collection of bytes. Text files are stored in human readable form. They can also be created using any text editor. Text files don’t have any specific encoding and it can be opened in normal text editor itself. Example of Text Files: Web standards: html, XML, CSS, Tabular data: csv, tsv etc. JSON Configuration: ini, cfg, reg etc Source code: c, app, js, py, java etc. Documents: txt, tex, RTF etc. III. OPENING OR CREATING A NEW FILE IN PYTHON The method open() is used to open an existing file or creating a new file. If the complete directory is not given then the file will be created in the directory in which the python file is stored. The syntax for using open() method is given below. – Syntax: – file_object = open( file_name, “Access Mode”, Buffering ) The open method returns file object which can be stored in the name file object (file-handle). File name is a unique name in a directory. The open() function will create the file with the specified name if it is not already exists otherwise it will open the already existing file. File Access Modes: The access mode: it is the string which tells in what mode the file should be opened for operations. There are three different access modes are available in python. Reading: Reading mode is crated only for reading the file. The pointer will be at the beginning of the file. Writing: Writing mode is used for overwriting the information on existing file. 32 Page Append: Append mode is same as the writing mode. Instead of over writing the information this mode append the information at the end. Below is the list of representation of various access modes in python. Access modes in Text Files ‘r' – Read Mode: Read mode is used only to read data from the file. ‘w' – Write Mode: This mode is used when you want to write data into the file or modify it. Remember write mode overwrites the data present in the file. ‘a' – Append Mode: Append mode is used to append data to the file. Remember data will be appended at the end of the file pointer. ‘r+' – Read or Write Mode: This mode is used when we want to write or read the data from the same file. ‘a+' – Append or Read Mode: This mode is used when we want to read data from the file or append the data into the same file. Buffering: Buffering is the process of storing a chunk of a file in a temporary memory until the file loads completely. In python there are different values can be given. If the buffering is set to 0 , then the buffering is off. The buffering will be set to 1 when we need to buffer the file. Examples of Opening TEXT Files in Python # open file in current directory f = open("test.txt“, “r”) # specifying full path – f = open(r“D:\temp\data.txt“, “r”) #–raw string f = open(“D:\\temp\\data.txt“, “r”) #-absolute path IV. CLOSING FILES IN PYTHON After processing the content in a file, the file must be saved and closed. To do this we can use another method close() for closing the file. This is an important method to be remembered while handling files in python. Syntax: file_object.close() string = "This is a String in Python" my_file = open(my_file_name.txt,"w+",1) my_file.write(string) my_file.close() print(my_file.closed) V. READING INFORMATION IN THE FILE In order to read a file in python, we must open the file in read mode. There are three ways in which we can read the files in python. – read([n]) – readline([n]) – readlines() – all lines returned to a list Here, n is the number of bytes to be read. Example 1: my_file = open(“C:/Documents/Python/test.txt”, “r”) print(my_file.read(5)) Output: Hello Here we are opening the file test.txt in a read-only mode and are reading only the first 5 characters of the file using the my_file.read(5) method. Example 2: my_file = open(“C:/Documents/Python/test.txt”, “r”) print(my_file.read()) 33 Page Output: Hello World Hello Python Good Morning #Here we have not provided any argument inside the read() function. #Hence it will read all the content present inside the file. Example 3: my_file = open(“C:/Documents/Python/test.txt”, “r”) print(my_file.readline(2)) Output: He This function returns the first 2 characters of the next line. Example 4: my_file = open(“C:/Documents/Python/test.txt”, “r”) print(my_file.readline()) Output: Hello World Using this function we can read the content of the file on a line by line basis. Example 5: my_file = open(“C:/Documents/Python/test.txt”, “r”) print(my_file.readlines()) Output: [‘Hello World\n’, ‘Hello Python\n’, ‘Good Morning’] Here we are reading all the lines present inside the text file including the newline characters. Example 5: Reading a specific line from a File Output: line_number = 4 How are You fo = open(“C:/Documents/Python/test.txt”, ’r’) #In this example, we are trying to read currentline = 1 only the 4th line from the ‘test.txt’ file for line in fo: using a “for loop”. if(currentline == line_number): print(line) break currentline = currentline +1 VI. WRITE TO A PYTHON TEXT FILE In order to write data into a file, we must open the file in write mode. We need to be very careful while writing data into the file as it overwrites the content present inside the file that we are writing, and all the previous data will be erased. We have two methods for writing data into a file as shown below. write(string) and writelines(list) Example 1: my_file = open(“C:/Documents/Python/test.txt”, “w”) my_file.write(“Hello World”) 34 Page The above code writes the String ‘Hello World’ into the ‘test.txt’ file. The first line will be ‘Hello World’ and my_file = as we have mentioned \n character, theo“w pe”n)(“C:/Documents/Python/test.txt”, cursor file andwill thenmove write to the next ‘Hello line of the Python’. my_file.write(“Hello World\n”) Remember if we don’t mention \n my_file.write(“Hello Python”) character, then the data will be written continuously in the text file like ‘Hello WorldHelloPython’ Example 3: fruits = [“Apple\n”, “Orange\n”, “Grapes\n”, “Watermelon”] my_file = open(“C:/Documents/Python/test.txt”, “w”) my_file.writelines(fruits) The above code writes a list of data into the ‘test.txt’ file simultaneously. Append in a Python Text File: To append data into a file we must open the file in ‘a+’ mode so that we will have access to both the append as well as write modes. Example 1: my_file = open(“C:/Documents/Python/test.txt”, “a+”) my_file.write (“Strawberry”) The above code appends the string ‘Strawberry’ at the end of the ‘test.txt’ file Example 2: my_file = open(“C:/Documents/Python/test.txt”, “a+”) my_file.write (“\nGuava”) The above code appends the string ‘Apple’ at the end of the ‘test.txt’ file in a new line flush() function When we write any data to file, python hold everything in buffer (temporary memory) and pushes it onto actual file later. If you want to force Python to write the content of buffer onto storage, you can use flush() function. Python automatically flushes the files when closing them i.e. it will be implicitly called by the close(), BUT if you want to flush before closing any file you can use flush() REMOVING WHITESPACES AFTER READING FROM TEXT FILE read() and readline() reads data from file and return it in the form of string and readlines() returns data in the form of list. All these read function also read leading and trailing whitespaces, new line characters. If we want to remove these characters you can use functions – strip() : removes the given character from both ends. – lstrip(): removes given character from left end – rstrip(): removes given character from right end File Pointer Every file maintains a file pointer which tells the current position in the file where reading and writing operation will take. When we perform any read/write operation two things happens: – The operation at the current position of file pointer – File pointer advances by the specified number of bytes. 35 Page 1- MARK QUESTIONS 1. To read three characters from a file object f, we use ………. (a) f.read(3) (b) f.read() (c) f.readline() (d) f.readlines() 2. The files that consists of human readable characters (a) binary file (b) text file (c) Both (a) and (b) (d) None of these 3. Which function is used to write a list of string in a file? (a) writeline() (b) writelines() (c) writestatement() (d) writefullline() 4. What will be the output of the following Python code? myFile = None for i in range (8): with open(“data.txt”, “w”) as myFile: if i > 5: break print(myFile.closed) (a) True (b) False (c) None (d) Error 5. What will be the output of the following Python code? myFile = open(“story.txt”, “wb”) print(“ Name of the file: ”, myFile.name) myFile.flush() myFile.close() Suppose the content of the file ‘‘story.txt’’ is Education Hub Learning is the key of success. (a) Compilation error (b) Runtime error (c) No output (d) Flushes the file when closing them 6. What is the output of following code? myfile=open(‘story.txt’,‘r’) s=myfile.read(10) print(s) s1=myfile.read(15) print(s1) myfile.close() a) Education b) Education c) Education d) Education Hub Learning Hub Hub Hub is Learning is Learning Learning is is