Programming 2: File Handling - PDF
Document Details
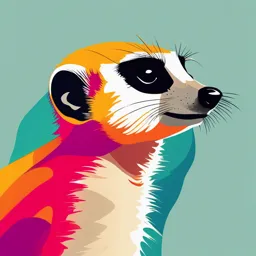
Uploaded by WellMadeAmber2007
Al Janad University
2025
Bilal Al-samaee
Tags
Summary
These lecture notes on Programming 2 cover the topic of file handling in Python. It explains how to open, read, write, and delete files, along with various methods for file manipulation. The notes are from Al-Janad University and dated 2025-2026.
Full Transcript
جامعة الجند كلية الهندسة وتقنيات المعلومات قسم الذكاء االصطناعي وعلم البيانات – مستوى 1 المحاضرة 6 د.بالل السامعي 2025-2026 Dr. Bilal Al-samaee 1 File Handling Dr. Bilal Al-samaee...
جامعة الجند كلية الهندسة وتقنيات المعلومات قسم الذكاء االصطناعي وعلم البيانات – مستوى 1 المحاضرة 6 د.بالل السامعي 2025-2026 Dr. Bilal Al-samaee 1 File Handling Dr. Bilal Al-samaee 2 Open File Python has several functions for creating, reading, updating, and deleting files. The key function for working with files in Python is the open() function. The open() function takes two parameters; filename, and mode. Dr. Bilal Al-samaee 3 There are four different methods (modes) for opening a file: 1. "r" - Read - Default value. Opens a file for reading, error if the file does not exist. Ex: : يحتوي علىmyfile.txt نفترض أن لدينا ملفًا اسمه Hello, world! file = open('myfile.txt', 'r') # أو فقطopen('myfile.txt') content = file.read() print(content) file.close() output: Hello, world! Dr. Bilal Al-samaee 4 2. "a" - Append - Opens a file for appending new content to end files without replace the previous content, creates the file if it does not exist. :مثال عملي المالحظة األولى: يحتوي على السطرnotes.txt نفترض أن لدينا ملف اسمه :ثم شغّلت هذا الكود file = open(notes.txt', 'a') # فتح الملف بوضع اإللحاق file.write(‘\المالحظة الثانيةn') # كتابة النص file.close() # إغالق الملف Output: المالحظة األولى المالحظة الثانية Dr. Bilal Al-samaee 5 3. "w" - Write - Opens a file in Python for writing, creates the file if it does not exist Ex: file = open('write_example.txt', 'w’) file.write(“The file has been overwritten”) file.close() 4. "x" - Create - To create a new file in Python, use the open() method, with "x" parameters, returns an error if the file exist Ex: file = open('create_example.txt', 'x') file.write(“the file has been created”) file.close() Dr. Bilal Al-samaee 6 To open a file for reading it is enough to specify the name of the file: f = open("demofile.txt") # for reading f = open("demofile.txt", ’rt’) Because "r" for read, and "t" for text are the default values, you do not need to specify them. Dr. Bilal Al-samaee 7 Open a File on the Server To open() the file, use the built-in open() function: The open() function returns a file object, which has a read() method for reading the content of the file: f = open("C:\\Users\bilal\demofile.txt" , "r") # C:\\Users\bilal\demofile.txt file path print(f.read()) #default the read() method returns the whole text, but you can also # specify how many characters you want to return. But how? See next Dr. Bilal Al-samaee 8 Close a file Close the file when you are finish with it: f = open ("c:\\demofile.txt", "r") print(f.readline()) f.close() Dr. Bilal Al-samaee 9 Open a File on the Server To read Only Parts of the File: f = open("demofile.txt", "r") print(f.read(5)) #Return the 5 first characters of the file f.close() To read a line: f = open("demofile.txt", "r") print(f.readline()) #Read one line of the file f.close() Dr. Bilal Al-samaee 10 Open a File on the Server To read two lines of the file: f = open("c:\\demofile.txt", "r") print(f.readline()) # قراءة السطر األول من الملف print(f.readline()) # قراءة السطر الثاني من الملف f.close() Loop through the file line by line: #Loop through the file line by line: f = open ("c:\\demofile.txt", "r") for x in f: print(x) # سيتم طباعة كل سطر من الملف f.close() Dr. Bilal Al-samaee 11 Overwrite Overwrite: Write to the file (writing with "w" means deleting all previous content): Ex: # will print all content file f = open("c:\\demofile.txt", "r") print(f.read()) f.close() f = open("c:\\demofile.txt", "w") f.write("Hoo! I have deleted the content!") f.close() # Open the file and read its contents after writing f = open("c:\\demofile.txt", "r") print(f.read()) f.close() Dr. Bilal Al-samaee 12 Delete a File To delete a file, you must import the OS module, and run its os.remove() function: import os os.remove("demofile.txt") To delete an entire folder, use the os.rmdir() method: import os os.rmdir("myfolder") #Remove Directory entire folder Dr. Bilal Al-samaee 13 The rename() Method The rename() method takes two arguments, the current filename and the new filename. import os os.rename('old_file.txt', 'new_file.txt’) Ex: Rename c:\demofile.txt to c:\my_new_file.txt import os os.rename('c:\\demofile.txt', 'c:\\new_file.txt’) Dr. Bilal Al-samaee 14 With Python provides a special syntax that auto-closes a file for you once you're finished using it. Syntax: with open('my_path/my_file.txt', 'r') as file: file_data = file.read() This with keyword allows you to open a file, do operations on it, and automatically close it after the code has been executed, in this case, reading from the file. Now, we don’t have to call f.close()! Dr. Bilal Al-samaee 15 With Ex: Writing text to a file using with: with open("c:\\demofile.txt", ", "w") as file: # Opens the file in write mode (and deletes its previous content, if any). file.write("Hello, this text is written using 'with' statement.\n") file.write("It handles file closing automatically!") Ex: # Read file content with open ("c:\\demofile.txt", "r") as file: content = file.read() print(content) Dr. Bilal Al-samaee 16