Digital Skills & Python Programming - Chapter 7 PDF
Document Details
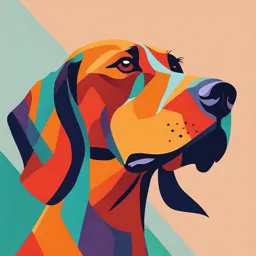
Uploaded by AmazingActionPainting2105
ENSA de Kénitra
Pr. Mehdia AJANA
Tags
Related
- CSC 213 Programming Language (2) Lecture Notes PDF
- Programming in Python for Business Analytics Lecture Notes PDF
- Programming 2 MEK3100 Lecture Notes PDF
- Programming with Python for Engineers PDF
- Introduction to Programming - STEM 1502 Lecture Notes PDF
- Starting Out with Python - Classes and Object-Oriented Programming PDF
Summary
This document is a chapter on Python object-oriented programming. It explains the core concepts, including classes, objects, attributes, and methods. It also touches on functional programming and their differences. The document is part of a larger course module about Digital Skills and Python Programming.
Full Transcript
Digital Skills and Python Programming Chapter 7 Python Object-Oriented Programming Pr. Mehdia AJANA Email: [email protected] Programming Paradigms in Python: PYTHON Functional vs. Object-Oriented: First steps Functional/Procedural Pro...
Digital Skills and Python Programming Chapter 7 Python Object-Oriented Programming Pr. Mehdia AJANA Email: [email protected] Programming Paradigms in Python: PYTHON Functional vs. Object-Oriented: First steps Functional/Procedural Programming: We break the code into a set of functions and these functions call each other with data passed between them. Object-Oriented Best for simple scripts and data processing. Programming Object-Oriented Programming: OOP We break the code into a set of Introduction entities/classes, and these entities interact with each other. These entities have data and methods. Ideal for complex, organized systems that model real-world entities. Pr. Mehdia AJANA 2 What is Object-Oriented Programming? PYTHON OOP is a programming paradigm based on First steps objects and classes. OOP helps in structuring programs into reusable pieces of code. OOP Key concepts: Object-Oriented Classes, Objects, Attributes, Methods Programming Encapsulation (OOP) Inheritance Polymorphism Almost everything in Python is an object, with its properties (attributes) and methods. Pr. Mehdia AJANA 3 Python Classes and Objects PYTHON Class: First steps Class is a user-defined data type A Class is like an object constructor, or a "blueprint" / “model” / “template” for creating objects (instances). Defines attributes and methods. Python class names are written Object-Oriented in CapitalizedWords / Camel Case Programming notation by convention To create a class, we use the keyword class: Example: Create an empty class named Person: class Person: pass # Empty class # having an empty class definition, would raise an error without the pass statement Pr. Mehdia AJANA 4 PYTHON Python Classes and Objects Create an Object First steps We can use the class named Person to create objects. An object is an instance of a class. While the class is the blueprint, an instance is an object that’s built from a Object-Oriented class and contains real data. Programming Example: Create an object named p1, and display its type: p1 = Person() print(type(p1)) # Output: 5 Pr. Mehdia AJANA PYTHON Python Classes and Objects Class Attributes and Methods First steps Object-Oriented Programming Pr. Mehdia AJANA 6 Python Classes and Objects PYTHON Class Attributes and Methods First steps Attributes are variables within a class that store data. Methods are functions defined inside a class that describe the behavior of an object. Object-Oriented Example: A Person class with attributes Programming (properties) like name, age, and a method greet(): class Person: def __init__(self, name, age): self.name = name self.age = age def greet(self): print(f"Hi, I'm {self.name}.") Pr. Mehdia AJANA 7 The Constructor (__init__ method) PYTHON The __init__ method is called automatically First steps when the class is being instantiated; when a new object is created. Used to initialize the object's attributes. Encapsulation: Bundling data (attributes) and methods (functions) that operate on the Object-Oriented data into a single unit (class). Programming Example: The __init__() method is called automatically when p1 and p2 are created. class Person: def __init__(self, name, age): self.name = name self.age = age # Creating objects p1 = Person("Ali", 25) p2 = Person("Ahmed", 30) Pr. Mehdia AJANA 8 The self Parameter PYTHON The self parameter is a reference to the current First steps object, and is used to access variables (attributes) that belong to the class. It does not have to be named self, but it has to be the first parameter of any method in the class: Object-Oriented Example: Programming Use the words myobject and abc instead of self: class Person: def __init__(myobject, name, age): myobject.name = name myobject.age = age def greet(abc): print(f"Hi, I'm {abc.name}.") p1 = Person("Ali", 36) p1.greet() Hi, I’m Ali Pr. Mehdia AJANA 9 Getter and Setter Methods PYTHON We can make attributes private and restrict direct First steps access to them. Instead, we use special methods (often called getters and setters) to interact with these attributes: In Python, a private attribute is prefixed with double underscore: __ class Person: Object-Oriented def __init__(self, name, age): Programming self.__name = name # Private attribute self.__age = age def get_name(self): return self.__name def set_name(self, name): self.__name = name def get_age(self): return self.__age 10 Getter and Setter Methods Example PYTHON (continued) First steps class Person: def set_age(self, age): if age > 0: self.__age = age else: Object-Oriented print("Age must be positive!") Programming Testing the getter and setter methods: p1 = Person("Ali", 25) print(p1.get_name()) # Output: Ali p1.set_age(26) print(p1.get_age()) # Output: 26 print(p1.__name) #AttributeError: 'Person' object has no attribute '__name' Pr. Mehdia AJANA 11 The __str__() Method PYTHON The __str__() method is a special class First steps method used to define the string representation of an object. It provides a human-readable string representation of the object. Called by print() or str(). Example: Object-Oriented class Person: Programming def __init__(self, name, age): self.name = name self.age = age def __str__(self): return f"{self.name}({self.age})" p1 = Person("John", 36) print(p1) # We can also use str(p1) John(36) # Printing the object will implicitly trigger the __str__ method. Pr. Mehdia AJANA 12 The __str__() Method PYTHON __init__() and __str__() are called dunder First steps methods / magic methods / special methods because they begin and end with double underscores (__). These methods are typically invoked implicitly by Python, not directly by the programmer. Object-Oriented __init__() is called implicitly when the Programming object is instantiated. __str__() is implicitly called when we use print(p1). Methods Without __: are regular methods defined by the programmer for specific functionality within the class. They are explicitly called on objects of the class and define custom behaviors or operations. E.g. p1.greet() Pr. Mehdia AJANA 13 Object Methods PYTHON Objects can also contain other methods. First steps Example: Add a method that displays the name and age: class Person: def __init__(self, name, age): self.name = name Object-Oriented self.age = age Programming def display_info(self): print(f"Name: {self.name}, Age: {self.age}") p1 = Person("Ali", 36) p1. display_info() Name: Ali, Age: 36 Pr. Mehdia AJANA 14 Modify & Delete Object Properties PYTHON You can modify properties of objects like this: First steps Example: Set the age of p1 to 40: p1.age = 40 #If age is a public attribute p1.set_age(40) #If __age is a private attribute You can delete properties on objects by using the del keyword for public attributes. Object-Oriented For private attributes you can define a class method to delete them: Programming del p1.age # For age as a public attribute p1.display_info() AttributeError: 'Person' object has no attribute 'age‘ #Method to add to the Peron class to delete __age as private attribute def delete_age(self): del self.__age 15 Delete Objects PYTHON You can delete objects by using the del First steps keyword: Example: Delete the p1 object: class Person: def __init__(self, name, age): self.name = name Object-Oriented self.age = age Programming def display_info(self): print(f"Name: {self.name}, Age: {self.age}") p1 = Person("John", 36) del p1 print(p1) NameError: 'p1' is not defined 16 Pr. Mehdia AJANA Python Inheritance PYTHON Inheritance is a mechanism where one class First steps inherits attributes and methods from another class: Parent class is the class being inherited from, also called base class. Child class is the class that inherits from Object-Oriented another class, also called derived class. Programming Inheritance allows code reuse. Create a Parent Class: Any class can be a parent class, so the syntax is the same as creating any other class. Let’s consider the person class that we created before as the parent class. Pr. Mehdia AJANA 17 Python Inheritance PYTHON First steps Create a Parent Class: class Person: def __init__(self, name, age): Object-Oriented self.name = name Programming self.age = age def display_info(self): print(f"Name: {self.name}, Age: {self.age}") Pr. Mehdia AJANA 18 Python Inheritance PYTHON Create a Child Class First steps To create a class that inherits the functionality from another class, send the parent class as a parameter when creating the child class: class Student(Person): def __init__(self, name, age, student_id): Object-Oriented Programming # Call the parent class constructor super().__init__(name, age) # you can use Person instead of super() # student_id is an attribute of the student class self.student_id = student_id Pr. Mehdia AJANA 19 Python Inheritance PYTHON Create a Child Class (cont.) First steps class Student(Person): def __init__(self, name, age, student_id): super().__init__(name, age) self.student_id = student_id Object-Oriented # Method overriding: modifying the Programming behavior of the parent class method def display_info(self): super().display_info() print(f"Student ID: {self.student_id}") Pr. Mehdia AJANA 20 Python Inheritance PYTHON Create a Child Object First steps Example: Use the Student class to create an object, and then execute the display_info method: s1 = Student("Ali", 25, "S12345") s1.display_info() Object-Oriented print(type(s1)) Programming print(isinstance(s1,Person)) Name: Ali, Age: 25 Student ID: S12345 True Note: All objects created from a child class are instances of the parent class 21 Pr. Mehdia AJANA Inheritance Levels Pr. Mehdia AJANA 22 PYTHON Inheritance Levels Single Inheritance: A class inherits from one First steps parent class: class Student(Person): pass Multiple Inheritance: A class inherits from Object-Oriented more than one parent class: Programming class Artist: def play(self): print("Playing music") class StudentArtist(Student, Artist): pass Pr. Mehdia AJANA 23 PYTHON Inheritance Levels Hierarchical Inheritance: Multiple derived First steps (child) classes inherit from a single base (parent) class. The parent class’s attributes and methods are shared across multiple child classes. Example: both Student and Teacher classes Object-Oriented inherit from the common Person class: Programming class Person: def __init__(self, name, age): self.name = name self.age = age def display(self): return f"Name: {self.name}, Age: {self.age}“ Pr. Mehdia AJANA 24 PYTHON Inheritance Levels Hierarchical Inheritance: Multiple derived First steps (child) classes inherit from a single base (parent) class. The parent class’s attributes and methods are shared across multiple child classes. Example: both Student and Teacher classes Object-Oriented inherit from the common Person class: Programming # Derived class 1: Student class Student(Person): pass # Derived class 2: Teacher class Teacher(Person): pass Pr. Mehdia AJANA 25 PYTHON Inheritance Levels Multilevel Inheritance: A class inherits from a First steps child class, which in turn inherits from another parent class. Example: Graduate class inherits from Student which itself inherits from Person class Graduate(Student): Object-Oriented def __init__(self): Programming super().__init__(self.name,self.age, self.student_id) def display_info(self): super().display_info() g1=Graduate("Ali", 23, 123456) g1.display_info() Name: Ali, Age: 23 Pr. Mehdia AJANA Student ID: 123456 26 Python Polymorphism PYTHON The word "polymorphism" means "many First steps forms", and in programming it refers to methods/functions/operators with the same name that can be executed on many objects or classes. Function Polymorphism Object-Oriented An example of a Python function that can be used on different objects is the len() function. Programming For strings: len() returns the number of characters For tuples: len() returns the number of items in the tuple For dictionaries: len() returns the number of key/value pairs in the dictionary Pr. Mehdia AJANA 27 Python Polymorphism PYTHON Function Polymorphism First steps Example: x = "Hello World!“ mytuple = ("apple", "banana", "cherry") thisdict = { "brand": "Ford", Object-Oriented "model": "Mustang", "year": 1964 Programming } print(len(x)) print(len(mytuple)) print(len(thisdict)) 12 3 3 Pr. Mehdia AJANA 28 Python Polymorphism PYTHON Class Polymorphism First steps Polymorphism is often used in Class methods, where we can have multiple classes with the same method name. Example: we have 3 classes: Car, Boat, and Plane, and they all have a method called move(): class Car: Object-Oriented def __init__(self, brand, model): self.brand = brand Programming self.model = model def move(self): print("Drive!") class Boat: def __init__(self, brand, model): self.brand = brand self.model = model def move(self): print("Sail!") Pr. Mehdia AJANA 29 Python Polymorphism PYTHON Class Polymorphism First steps class Plane: def __init__(self, brand, model): self.brand = brand self.model = model def move(self): Object-Oriented print("Fly!") Programming car1 = Car("C1", "CM1") boat1 = Boat("B1", "BM1") Drive! plane1 = Plane("P1", "PM1") Sail! for x in (car1, boat1, plane1): Fly! x.move() 30 Pr. Mehdia AJANA Python Polymorphism PYTHON Inheritance Class Polymorphism First steps Child classes can have the same method name as the parent class. Method overriding: when a child class provides its own specific implementation of a method that is already defined in its parent class, to change or extend the behavior of the inherited method. Object-Oriented Example: Let’s make the previous Vehicle class a parent class, and make Car, Boat, Plane child Programming classes of Vehicle: Parent class: class Vehicle: def __init__(self, brand, model): self.brand = brand self.model = model def move(self): print("Move!") Pr. Mehdia AJANA 31 Python Polymorphism PYTHON Inheritance Class Polymorphism Child classes: First steps class Car(Vehicle): pass # we do not override the methods of Vehicle class Boat(Vehicle): def move(self): C1 print("Sail!") Object-Oriented CM1 class Plane(Vehicle): Programming def move(self): Move! print("Fly!") B1 car1=Car("C1","CM1") BM1 boat1=Boat("B1","BM1") Sail! plane1=Plane("P1","PM1") P1 for x in (car1, boat1, plane1): PM1 print(x.brand) print(x.model) Fly! x.move() Pr. Mehdia AJANA 32 Exercise: Create a "Book" Class PYTHON Create a class called Book with the following First steps attributes: title (string) author (string) year_published (integer) The class should have the following methods: Object-Oriented __init__() to initialize the attributes. Programming get_info() that returns a formatted string showing the book’s title, author, and year of publication. Create an instance of the Book class and print the book information using the get_info() method. Pr. Mehdia AJANA 33 Solution: Title: Python Programming, Author: Kevin Smith, Year: 2018 Pr. Mehdia AJANA 34 PYTHON References: First steps You can read more about Object- Oriented Programming: https://realpython.com/quizzes/python3 -object-oriented-programming/ https://www.geeksforgeeks.org/python- Object-Oriented oops-concepts/ Programming https://docs.python.org/3.13/tutorial/cl asses.html# https://docs.python.org/fr/3/tutorial/cla sses.html# Pr. Mehdia AJANA 35