CST8259 XML in PHP.pptx
Document Details
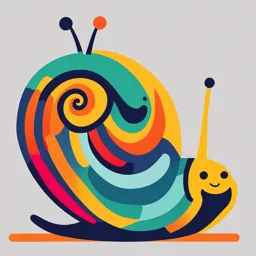
Uploaded by EndearingMeerkat4742
Tags
Full Transcript
CST8259 Web Programming Languages II Two Ways of Processing XML Document Object Model (DOM) – Build a hierarchical tree structure to which you can refer repeatedly. – DOM builds memory-intensive trees, but, once they’re built, the DOM provides plenty of tools to navigate, proc...
CST8259 Web Programming Languages II Two Ways of Processing XML Document Object Model (DOM) – Build a hierarchical tree structure to which you can refer repeatedly. – DOM builds memory-intensive trees, but, once they’re built, the DOM provides plenty of tools to navigate, process, and manipulate those tree structures. Forward-Only Read and Write (XmlReder, XmlWrite) – Treat XML documents as a character stream – Handling XML documents in a linear fashion, less flexibility, however, it is fast and resource efficient. – in C#/.NET, use the subclasses of abstract class XmlReader and XmlWriter provide functions to processing XML documents in this way. PHP XML Processing PHP installation contains packages for handling XML in both DOM and Forward-only approaches. See following links for details: http://www.php.net/xml/ http://www.php.net/dom/ PHP also provides a third approach to process XML documents – SimpleXML – SimpleXML is included and available by default in PHP 5 PHP SimpleXML Extension Like DOM, the SimpleXML PHP extension is another tree- based XML parser. – But SimpleXML takes a different approach to handling the tree; It has a much smaller API and handles elements and attributes more intuitively. – Contrasting to DOM approach, the SimpleXML extension has a single class type, three functions, and six class methods. Using SimpleXML The SimpleXMLElement class is the central class for all operations within this extension. $xml = "content"; $sxe = new SimpleXMLElement($xml); Or load and XML from a file: $sxe = simplexml_load_file("filename.xml"); Accessing Elements The document element is the object returned when a document is first loaded into SimpleXMLElement. All other elements of the tree by element name as properties of SimpleXMLElement objects. Accessing Elements If an element name contains characters not permitted under PHP's naming rule, you must encapsulating the element name within braces and the single quotation. Accessing Attributes In PHP SimpleXML, attributes of an element are kept in an associative array named after the element. – The keys of the array elements are the names of the attributes …… $xml=simplexml_load_file("book.xml"); echo (string)$xml->book['category’]; //SimpleXMLElement object echo (string)$xml->book->title['lang']; ?> PHP Programming John Simth Accessing Content To access the contents of an element, use the same SimpleXMLElement objects. When an element is a simple element (contains no child elements), the content of the element is the first element of SimpleXMLElment object. – When the print statement is used with the object, the content is returned as a string. – You may have explicitly cast the object to the right data type before using Access Repeating Element For repeating elements, the corresponding SimpleXMLElement object is an array and iterate-able. Access Repeating Element Repeating child elements can also be accessed using 0 based index – When object is accessed without index, the first element in the array is accessed. Reading Attributes To read from and write to an attributes of an element, use the name of the attribute as the index. Modify Content You can edit nodes and content natively using SimpleXML When working with repeating elements, you must ensure you are modifying the correct one. Modify Elements with Child Elements You can edit elements containing child elements, in the same way as with text content. – The child elements are removed from the document and replaced with the text content. – Even if the new text content contains XML elements, they will be replace with XML entities and used as strictly text content. <title>SimpleXML in PHP 5</title> Modify Elements with Child Elements To replace the subtree with another subtree, you can use the DOM extension SimpleXML in PHP 5 Removing Elements To remove elements from a tree using the PHP unset() function – The element must be referred as the property decent from root element. Study how to access elements of XML as properties … Writing Attributes To write to an attributes of an element, use the name of the attribute as the index. Create New Attributes New attributes can be created using SimpleXML … … … … … Removing Attributes Use PHP function unset( ) to remove an attribute – As with elements, the attribute has to be referred as a property decent from root element. Saving XML Data Using the asXML() method, to output a document or subtree to a string or a file. The actual output depends upon the node from which this method is called. – When called from the document element, which is the element returned from the initial load functions, the entire document is output. This includes the XML declaration, the prolog, the body. – When called from any other node within the tree, the entire node and any subtree are output. $xml = "content"; $sxe = new SimpleXMLElement($xml); print $sxe->asXML(); //output to HTML $sxe->asXML('filename.xml'); //save to a xml file content When to Use the Different Methods If you have a very simple but large XML document that needs only linear processing, and you don’t need to modify the document, Forward-Only Read is a good candidate. If you need to process a more complex XML document, and mostly extracting information from XML document, SimpleXML is a good choice. If you need to create XML documents from scratch, or make heavy modifications to existing documents, use DOM.