csc123_s24_Week4.pdf
Document Details
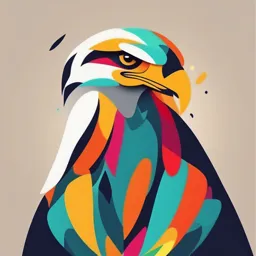
Uploaded by WarmerMemphis
Full Transcript
CSC123-01 (INTRO TO PROGRAMMING II) Week 4 (September 23) Usman Aslam | Computer Science RECAP In Object-Oriented programming, real-world problems are considered in terms of Objects, their Properties, and their interface or features Object-orientated programming offers greater re-use of software com...
CSC123-01 (INTRO TO PROGRAMMING II) Week 4 (September 23) Usman Aslam | Computer Science RECAP In Object-Oriented programming, real-world problems are considered in terms of Objects, their Properties, and their interface or features Object-orientated programming offers greater re-use of software components In normal life, an Object is material thing that can be see and touched. It has properties (e.g. color, size) and an interface (e.g. keyboard on a computer) through which objects can be interacted with. In programming an Object is a virtual construct that resembles a real-world object by having properties (data) and interfaces (methods) In real-world, every Object belongs to one or more categories and the object’s general behavior is defined by its category In programming every Object belongs to a class that defines its behavior Examples of classes in Java are java.util.Scanner and java.lang.String RECAP //imports import java.util.Scanner; //class declaration public class Person{ //fields/members private String firstName; //Constructor public Person(Sttring fname){ firstName=fname; } //Methods public void setFirstName(String s) { firstName = s; } } CLASSES IN JAVA This expression creates an object of class Scanner in memory Scanner keyboard = new Scanner(System.in); CLASSES IN JAVA This expression creates an object of class Scanner in memory Scanner keyboard = new Scanner(System.in); The object's memory address is assigned to the keyboard variable CLASSES IN JAVA This expression creates an object of class Scanner in memory Scanner keyboard = new Scanner(System.in); The object's memory address is assigned to the keyboard variable keyboard variable Scanner(class) object CONSTRUCTORS Classes can have special methods called constructors Constructors are used to performing operations at the time an object is created. Constructors typically initialize instance fields and perform other object initialization tasks A constructor is a method that is automatically called when an object is created. CONSTRUCTORS Constructors have a few special properties that set them apart from normal methods. 1. Constructors have the same name as the class 2. Constructors have no return type (not even void). 3. Constructors may not return any values 4. Constructors are typically public AccessSpecifier ClassName (Pararmeters…….) { …… } CONSTRUCTOR DECLARATION Access specifier public Same as the class name Parameters Car(…) If you no constructor is specified, then Java creates a default constructor with no parameters PRINTING OBJECTS By default if you print an object, you will see its unique reference assigned by Java The toString() method in Java is a special method that returns a string representation of an object The toString() method can be customized to print the object in a desired format public String toString() { return ”Customized string”; } REFERENCE VS VALUE Java process arguments and variable assignments for primitive types by value For objects, all arguments and variables are by reference The value of the reference variable is an address or reference to the object in memory A copy of the object is not passed, just a pointer to the object. When a method receives a reference variable as an argument, it is possible for the method to modify the contents of the object referenced by the variable COMPARING FOR EQUALITY You cannot compare two objects to each other by traditional means i.e. by using == operator. The equals() method in Java is used to compare two objects for equality. public boolean equals(Object obj) { //logic to compare objects } THE THIS REFERENCE The this reference is simply a name that an object can use to refer to itself. The this reference can be used to overcome shadowing and allow a parameter to have the same name as an instance field. public void setFeet(int feet) { this.feet = feet; //sets the this instance’s feet field //equal to the parameter feet. } SCOPE OF INSTANCE FIELDS Variables declared as instance fields, are known as global variables i.e. they can be accessed anywhere within the class Variables declared within a method, are only “visible” within that method. These variables are known as local variables Variables declared within a sub-structure within a method (e.g. a loop or try block) are only “visible” within that structure If an instance field (variable) is declared with the public access specifier, it can also be accessed by code outside the class, if an instance of the class exists SHADOWING Within a method, variable names must be unique A method may have a local variable with the same name as a global variable (instance field) This is called shadowing The local variable will hide the value of the global variable i.e. it will shadow it Shadowing is discouraged and local variable names should not be the same as instance field names To avoid the ambiguity of shadowing variables the global variables (instance fields) should can be prefixed by keyword this. NULL REFERENCE A null reference is a reference variable that points to nothing. If a reference is null, then no operations can be performed on it. References can be tested to see if they point to null prior to being used. if(name != null) { System.out.println("Name is: " + name.toUpperCase()); } GARBAGE COLLECTION When objects are no longer needed, they should be destroyed. This frees up the memory that they consumed. Java handles all of the memory operations for you. Simply set the reference to null and Java will reclaim the memory. GARBAGE COLLECTION The Java Virtual Machine has a process that runs in the background that reclaims memory from released objects The garbage collector will reclaim memory from any object that no longer has a valid reference pointing to it BankAccount account1 = new BankAccount(500.0); BankAccount account2 = account1; This sets account1 and account2 to point to the same object GARBAGE COLLECTION If a method with the signature: public void finalize(){…} is included in a class, it will run just prior to the garbage collector reclaiming its memory. The garbage collector is a background thread that runs periodically. It cannot be determined when the finalize method will actually be run. GARBAGE COLLECTION A BankAccount object account1 Address account2 Address Balance: 500.0 Here, both account1 and account2 point to the same instance of the BankAccount class. GARBAGE COLLECTION A BankAccount object account1 null account2 Address Balance: 500.0 However, by running the statement: account1 = null; only account2 will be pointing to the object. GARBAGE COLLECTION A BankAccount object account1 null account2 null Balance: 500.0 Since there are no valid references to this object, it is now available for the garbage collector to reclaim. If we now run the statement: account2 = null; neither account1 or account2 will be pointing to the object. GARBAGE COLLECTION account1 null account2 null Balance: 500.0 The garbage collector reclaims the memory the next time it runs in the background. EXERCISE Create a class Book with the following fields: Publishing Company should be static and pre-initialized as “Pearson” ISBN as String Number of Pages as int Author Name as String Amazon Rank as int Create following constructors: Constructor that takes author name and ISBN Constructor that takes author name, ISBN, and number of pages Create following Methods: getAuthor getAmazonRank getNumberOfPages setAmazonRank