csc123_1_Week5.pdf
Document Details
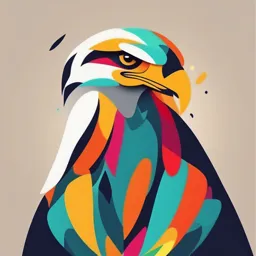
Uploaded by WarmerMemphis
Full Transcript
CSC123-01 (INTRO TO PROGRAMMING II) Week 5 (September 30) Book Chapter 8 Usman Aslam | Computer Science RECAP Classes can have special methods called constructors Constructors are used to performing operations at the time an object is created. Constructors typically initialize instance fields and pe...
CSC123-01 (INTRO TO PROGRAMMING II) Week 5 (September 30) Book Chapter 8 Usman Aslam | Computer Science RECAP Classes can have special methods called constructors Constructors are used to performing operations at the time an object is created. Constructors typically initialize instance fields and perform other object initialization tasks A constructor is a method that is automatically called when an object is created. RECAP Variables declared as instance fields, are known as global variables i.e. they can be accessed anywhere within the class Variables declared within a method, are only “visible” within that method. These variables are known as local variables Variables declared within a sub-structure within a method (e.g. a loop or try block) are only “visible” within that structure If an instance field (variable) is declared with the public access specifier, it can also be accessed by code outside the class, if an instance of the class exists RECAP equals() method is used to compare two objects for equality toString() method is used to print the internal state of an object this the reference is used to avoid ambiguity when referring to class fields Variables containing Java objects only contain their memory address. Therefore, you cannot compare two objects using == operator COMPOSITION The composition is the strong type of association An association is said to composition if an Object owns another object, and another object cannot exist without the owner object Consider the case of Human having a heart. Here Human object contains the heart and heart cannot exist without Human COMPOSITION //Car must have Engine public class Car { //engine is a mandatory part of the car private final Engine engine; public Car () { engine = new Engine(); } } //Engine Class class Engine {} AGGREGATION Aggregation is a weak association An association is said to be aggregation if both Objects can exist independently For example, a Team object and a Player object The team contains multiple players, but a player can exist without a team AGGREGATION //Team public class Team { //players can be 0 or more private List players; public Team () { players = new ArrayList(); } } //Player Object class Player {} UML UML, or Unified Modeling Language, is a standardized modeling language used primarily for software engineering Provides a set of graphic notation techniques to create visual models of object-oriented software systems Some of the diagrams included in UML notation are: Class Diagram: Displays classes, attributes, operations, and relationships. Object Diagram: Represents instances of classes and their relationships. Composite Structure Diagram: Represents internal structures of a class and collaborations between Deployment Diagram: Shows the configuration of runtime processing nodes and the components Use Case Diagram: Captures system functionalities from a user's perspective. Sequence Diagram: Represents object interactions arranged in a time sequence. Activity Diagram: Depicts workflows of activities and actions. State Machine Diagram: Illustrates states and transitions of an object. CLASS DIAGRAM Represents classes Rectangle shape with three sections Name of the Class Instance Fields Methods The + sign shows that the field or method is public The – sign shows that the field or method is private COMPOSITION IN UML Composition is Strong relationship between objects Composition is represented by a solid (filled) diamond shape attached the whole class of which the other classes is parts BI-DIRECTIONAL ASSOCIATION Bi-directional association exists between classes where objects of both classes know each other It should be possible obtain information about the other object by inquiring either objects in the relationship Example: You can tell from by looking at a sticker on a book which library the book belong to. You could also take the serial number of the sticker and ask the library if the belong to them. Both library and the book know about each other. JAVADOC Java Doc is Java Standard for documenting Java Classes Java is structured as: Modules Packages Classes Fields Methods Java Official Documentation is based on Java Doc standard https://docs.oracle.com/en/java/javase/17/docs/api/ JAVADOC JavaDoc is both a tool and a standard to document your Java Classes public class ExampleClass { //... } JAVADOC JavaDoc is both a tool and a standard to document your Java Classes public int add(int a, int b) { //... } JAVADOC JavaDoc is both a tool and a standard to document your Java Classes public static final int EXAMPLE_CONSTANT = 1; EXERCISE 1. Create a UML model of two classes: a Library and a Book 2. The information that the library should store: 1. Library Name 2. Address including City and State 3. The information that the Book should store : 1. Title, Author, Number of Pages, ISBN 4. The library and the Book have one-to-many relationship. Their relationship is bi-directional. 5. Implement the class in Java with whatever constructors and methods you consider important 6. Both classes should have toString() and equals() methods appropriately implemented. INHERITANCE A Java class can inherit its properties (fields and methods) from another class Vehicle Car Tesla Model 3 INHERITANCE A Java class inherits its parent class (known as a superclass) by using a special keyword “extends “ public class Car extends Vehicle By inheriting from a superclass, the Java class becomes a subclass A Java subclass inherits members (fields and methods) from the superclass without any of them being rewritten In addition to inheriting members from the superclass, the subclass can have its own additional members. This is known as specialization The subclass can “rewrite” the superclass’s methods to suit its needs, this is called method overriding. This is different from the method overloading A class refers to itself as this and its superclass as super. The default constructor for a class can be called this(), and superclasses as super() MEMBERS OF SUPERCLASS Members of the superclass that are marked private: – – – are not inherited by the subclass exist in memory when the object of the subclass is created may only be accessed from the subclass by public methods of the superclass Members of the superclass that are marked public: – – Are inherited by the subclass, and maybe directly accessed from the subclass CONSTRUCTORS OF SUPERCLASS Constructors are not inherited When a subclass is instantiated, the superclass default constructor is executed first The super keyword refers to an object’s superclass The superclass constructor can be explicitly called from the subclass by using the super() keyword Calls to a superclass constructor must be the first java statement in the subclass constructor OVERRIDING METHODS A subclass may have a method with the same signature as a superclass method The subclass method overrides the superclass method An object of the subclass invokes the subclass’s version of the method, not the superclass’s The @Override annotation should be used (but not compulsory) just before the subclass method declaration – This causes the compiler to display an error message if the method fails to correctly override a method in the superclass OVERRIDING METHODS A subclass method can call a superclass method via the super keyword super.getBalance(); The final modifier will prevent the overriding of a superclass method in a subclass public final void message() If a subclass attempts to override a final method, the compiler generates an error This ensures that a particular superclass method is used by subclasses rather than a modified version of it JAVA INHERITANCE TREE