CS STUDY GUIDE .pdf
Document Details
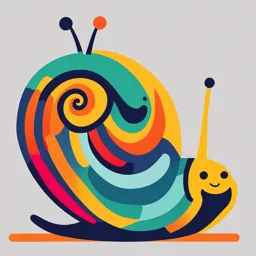
Uploaded by RedeemingDwarf
Tags
Full Transcript
CS EXAM 1 STUDY GUIDE Chapter 4(WHILE LOOPS: While Loop: A control flow statement that repeatedly executes a block of code as long as a given condition is true. - Indefinite iteration Condition: A boolean expression that is evaluated before each iterati...
CS EXAM 1 STUDY GUIDE Chapter 4(WHILE LOOPS: While Loop: A control flow statement that repeatedly executes a block of code as long as a given condition is true. - Indefinite iteration Condition: A boolean expression that is evaluated before each iteration of the loop. If the condition is true, the loop continues; if false, the loop terminates. Iteration: when you go through each value of a number, list,etc Iterable : object that can be iterated over Iterator: the object that allows things to be iterable Loop Body: The block of code inside the while loop that is executed repeatedly as long as the condition is true. - A single execution of the loop body. Each pass through the loop is one iteration. Infinite Loop: A loop that runs indefinitely because the termination condition is never met. This often occurs when the loop condition is always true or the condition is not updated correctly within the loop. Termination: The point at which a loop stops executing. This happens when the condition evaluates to false. Initialization: The process of setting up variables before the while loop starts. Proper initialization is crucial to ensure that the loop functions correctly. Increment/Decrement: The process of updating a variable that affects the loop condition. This is usually done within the loop body to eventually terminate the loop. - += / -= Break: Alternatives for break: “and not” and “flag” Assertion- checks if a condition is true and if false raises and assertion error - assert(condition) - assert(condition)(error message) Chapter 3(FOR LOOPS): for loop: a count-controlled loop and is used to execute a loop body a predictable number of times - Definite iteration loop variable: A variable defined in the header of a for loop. - (start,end) , (end) , (start,end,step) - Step naturally is 1 but if you want to go backwards use -1 iteration: The process of looping through the objects or items in a collection iterable: An object (or the adjective used to describe an object) that can be iterated over iterator: the object that produces successive items or values from its associated iterable iter(): The built-in function used to obtain an iterator from an iterable nested loop: a loop inside a loop An adjective: An object may be described as iterable. A noun: An object may be characterized as an iterable. Nested for loops:When writing two for loops whatever u want initialized should be above the for loop. Whatever we do once stays outside the loop and multiple is inside the loop. Chapter 2(CONDITIONALS) If statement- if statement is true do something Elif statement- if previous statement is false do something else Else statement- if none of the previous statement are true do this -no limit to elif statements Nested conditions- allows us to check multiple conditions function definition: A statement that creates a function. header: The first line of a function definition. body: The sequence of statements inside a function definition. function object: A value created by a function definition. The name of the function is a variable that refers to a function object. parameter: A name used inside a function to refer to the value passed as an argument. local variable: A variable defined inside a function, and which can only be accessed inside the function. docstring: A string that appears at the top of a function definition to document the function’s interface. multiline string: A string enclosed in triple quotes that can span more than one line of a program. precondition: A requirement that should be satisfied by the caller before a function starts; true before the function starts postcondition: A requirement that should be satisfied by the function before it ends. Module: file that contains a collection of related functions. - use import to get access to a module - use dot notation to get access to the function Ex: height = math.sin(radians) Call Stack: allows us to call different functions to perform different tasks(keeps track of variables that can be used) - Push : push something onto the top - Pop: remove something off the top Call frames: created when a function is called then put unto the stack Stack frames: holds local variables/parameters Chapter 1(VARIABLES/EXPRESSIONS/STATEMENTS) Know Arithmetic Operators,Relational Operators Literal : data-type value - the expression 10 ** 4 / 2 has the literal integers 2, 4, and 10. Know the different types(str,floats,bool,int) Str- sequence of letters held by quotation marks Bool- True(if not 0 then true) or false(data is 0) Integer- positive or negative non-decimal number Float- decimal number Name Class Type Integers int Float float Strings str Booleans bool Comments- line of text placed within the code that provides extra context to whoever is reading it. - Use # or “” to comment something out Debugging- allows us to see eros in our code - Syntax Error: “Syntax” refers to the structure of a program and the rules about that structure. If there is a syntax error anywhere in your program, Python does not run the program. It displays an error message immediately. - Runtime Error: If there are no syntax errors in your program, it can start running. But if something goes wrong, Python displays an error message and stops. This type of error is called a runtime error. It is also called an exception because it indicates that something exceptional has happened. - Semantic Error: If there is a semantic error in your program, it runs without generating error messages, but it does not do what you intended. Short circuiting- Stops before finishing/evaluating another condition