Chapter 1: Basic Principles: Which Object for Computer Science? PDF
Document Details
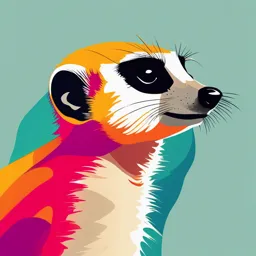
Uploaded by RealisticDeStijl
Badji Mokhtar University - Annaba
Dr. Sarra Namane
Tags
Related
- Introduction to Object-Oriented Programming (OOP) PDF
- CSC435 Object-Oriented Programming Introduction PDF
- Object-Oriented Programming - Lecture Notes PDF
- Introduction To Object Oriented Programming PDF
- Object-Oriented Programming Introduction PDF
- Chapter 1: Basic Principles: Object for Computer Science 2024/2025 PDF
Summary
This document is an introduction to object-oriented programming (OOP) for computer science students at Badji Mokhtar University, Annaba. It covers basic principles, entities, and attributes.
Full Transcript
Chapter 1: Basic Principles: Which Object for Computer Science? Dr. Sarra Namane Department of Computer Science Badji Mokhtar University, 1 Annaba Introduction Software works with data. To use data...
Chapter 1: Basic Principles: Which Object for Computer Science? Dr. Sarra Namane Department of Computer Science Badji Mokhtar University, 1 Annaba Introduction Software works with data. To use data effectively, you need to understand, analyze, update, and store it. How can you manage all this data? With Object- Oriented Programming (OOP)! OOP lets you organize data and the actions that work on it in a clear, understandable way. Once you've set up the necessary data classes and objects, you can work with the data in a logical 2 Introduction (2) Perception of the Environment: When we look out the window, we notice specific objects like cars, people, trees, and buildings. Cognitive Mechanisms: This selective perception highlights subtle cognitive mechanisms that are fundamental to understanding object-oriented programming. Selective Attention: We do not mention ambient elements like air, temperature, or light, despite also perceiving them. Object Attributes: The noticeable objects have distinct structures, shapes, dimensions, and colors, characterized by specific "attributes.“ 3 The trio Trio : We describe the world using the trio An entity: is anything that can be distinctly identified or recognized. It could be a physical object like a car, a person, or a tree, or it could be an abstract concept like a company, a project, or a particular event. Entity vs. Attribute: Entities (like cars) are perceived because they are characterized by multiple attributes (color, age, size) that converge to form a coherent object. Role of Attributes: Attributes themselves are not objects but serve to define and make objects perceivable. Common Attributes Across Objects: Attributes can apply to many different objects (e.g., both trees and cars have a color). 4 An example of the trio 19 years Valu Labiod Annaba e imene 06582…….. 1254252 35 Attribut e Entit y https://www.tutorialandexample.com/generalization- in-dbms 5 What is Object Oriented Programming? Object Oriented Programming is a coding paradigm that organizes code around data, known as “objects”, rather than functions and procedures. OOP simplifies software development by enabling us to model any data that we need and work with that data in a logical way. OOP uses classes to define the data model and provide simple, reusable blueprints for creating objects. By allowing us to model the world 6 What is an Object An object is a self-contained unit that combines both data and behavior. State: The data inside an object, represented by variables called fields or attributes. For example, if you have an object representing a car, its state could include its color, brand, and speed. Methods: The behavior of an object, represented by functions that operate on the object's state. These methods define what the object can do. For example, a car object might have methods like accelerate(), brake(), and honk(). https://codingnomads.com/blog/what- Identity: The identity of an object is is-object-oriented-programming-oop- what makes it unique, no matter its concepts-in-java 7 Storage of Objects in Memory Object Memory Allocation: Each object created in a program is allocated a specific memory space upon its creation. Primitive Data Types: To simplify memory management, programmers use primitive data types with known, fixed sizes (e.g., integers, floats, characters). Size of Primitive Types: Integers typically occupy 32 bits. Floating-point numbers (reals) occupy 64 bits. Unicode characters occupy 16 bits. Object Attributes: Attributes of an object, such as dimensions, colors, or brands, are stored using these primitive types, which allows for efficient memory usage. 8 Example of object memory size calculation Let's calculate the memory size required for an example of object that has the following attributes: Attribute 1: `int age` (32 bits) Attribute 2: `float height` (64 bits) Attribute 3: `char gender` (16 bits) Attribute 4: `String name` (let's assume the name is stored as a sequence of Unicode characters, and it has 10 characters; each character is 16 bits) Total Size of the Object: Total size= size of `age` + size of `height` + size of `gender` + size of `name` Total size = 32 bits + 64 bits + 16 bits + 160 bits 9 Total size = 272 bits The Referent of an Object Unique Object Naming: Each object in a program is given a unique name that serves as its identifier. Object Name as Memory Address: The name of the object corresponds uniquely to its physical memory address. Memory Address Uniqueness: The memory address is a unique identifier, ensuring no two objects can occupy the same memory space. Referent as a Variable: The object's name is a special variable called a referent, which stores the memory address of the object. Referent Storage: The referent is stored in a dedicated memory space for symbolic names. 32-bit Addressing: Typically, a memory address is 32 bits long, allowing for up to \(2^{32}\) unique addresses. Referent Function: A referent is a 32-bit variable that holds the 10 physical address of an object, enabling efficient memory access The Referent of an Object (2) Stack Memory: Stack memory is like a short-term storage space where Java keeps temporary data for methods. When a method runs, its data is stored here, and when the method finishes, the data is removed. Heap Memory: Heap memory is a larger storage space where Java keeps objects. When you create an object, it’s stored in the heap. The heap is managed by Java, which cleans up unused objects automatically. https://www.scientecheasy.com/2020/06/how-to- create-object-in-java.html/ 11 Stack memory example In this example, each time the add method is called, a new stack is created to store the local variables a, b, and result. When the method completes, these variables are removed from the stack. 12 Heap memory example In this example, the person object is created in heap memory. It stays there until it's no longer used, after which the Garbage Collector removes it from memory. 13 Indirect Addressing Multiple Referents for One Object: A single object in memory can be accessed by multiple referents, each containing the same physical memory address. Different Names, Same Object: Just as a book can be referred to by different names or descriptions by different people, in programming, an object can be referred to by different variables or names. Independent Contexts: These different referents can be used in separate contexts or parts of a program that are unaware of each other. Indirect Addressing: This concept, known as indirect addressing, allows multiple references to the same object in memory without creating duplicates. Object-Oriented Programming: In object-oriented 14 programming, this mechanism facilitates flexible and modular Multiple Referents Example In this example, person1 and person2 are two references pointing to the same Person object in memory. When the setName method is called using person2 to change the name to "Bob", the change is reflected for both person1 and person2 because they both refer to the same memory location. This is because person1 and person2 are not separate objects; they are 15 The Object in Its Passive Version Perception of Objects: We often perceive and refer to objects as wholes (e.g., "the car") rather than focusing on their individual components (e.g., "the wheel" or "the door"). Components of Objects: Objects are made up of various parts or sub-objects. For example, a car consists of wheels, an engine, etc. Object-Oriented Distinction: Public Interface: The aspects of an object that other objects use to interact with it (e.g., methods like `start()` or `stop()`). Internal Functioning: The internal details and components necessary for the object’s own operations (e.g., wheels and engine for a car). Physical Separation: It is important to separate what is exposed to other objects from what is necessary for the object’s internal 16 functioning to ensure better code organization and modularity. Object Composition Composition Relationship: Involves a primary object (composite) containing other objects (components). Dependency: Component objects cannot exist independently; they rely entirely on the composite object. Access: Components are accessed only through the composite object. Real-World Example: A house (composite object) contains rooms (component objects), Composition of which dois objects not exist independently of a design the house.principle where complex objects are created by combining simpler objects. This approach reflects real-world relationships and dependencies, where complex systems are made up of smaller components that interactwith each other. 17 Dependence Relationship Dependence Relationship: Involves objects that are connected, but where one object’s existence is not fully dependent on the other. Example: Passengers in a car—passengers do not depend on the car for their existence and can exist independently of the car. Types of Relations: Includes references or associations where In a objects use each library system, a Bookother but object hasdo not haveto a strict a reference composite an Authorrelationship. object, indicating an association between Modeling the two. Flexibility: The Book usesAllows for more the Author, butflexible and realistic the Author is not of modeling a part of the Book's interactions internal structure between objects andwhere strict can exist containment is independently. not required. This relationship is an example of an association rather than composition, where the objects interact but do not have a strict 18 The object in its active version Dynamic Nature: Objects are not static; they can move, change, and react to events. Interactions and Reactions: Objects respond to signals and interact with other objects (e.g., a car stopping at a red light). State Changes: Objects can transit between various states, affecting their attributes or behavior. Significance of Active Objects: Active objects are more interesting and important as 19 Object State Object state: refers to the current condition of an object, determined by its attributes and their values. State changes occur when an object's attributes are modified, but the object's identity remains constant despite these changes. State Changes: Objects can continuously change their state by altering their attributes. Identity Preservation: Despite changes, an object retains its fundamental identity and remains the same object. Attribute Modification: Changing an attribute involves updating its value in memory without altering the object's address. Frequent Operation: Updating attributes is a common and simple operation for computers. Lifecycle: An object maintains its identity until it is deleted from memory, reflecting a lifecycle of creation, state changes, and eventual deletion. Life Metaphor: An object’s time in memory is like a life story, 20 Object Lifecycle Object Lifecycle: An object’s life cycle involves a series of state changes from creation to deletion. State Transitions: Includes creation, usage, modification of attributes, and destruction. Creation: Memory is allocated, and attributes are initialized. Usage and Modification: The object’s state changes based on interactions and program conditions. Destruction: The object is removed from memory when no longer needed. Dynamic Representation: State changes enable 21 State changes Responsibility: Object state changes are driven by operations or methods defined for the object. Example: For a traffic light, an operation called `change` modifies the color attribute. Operations: These operations (e.g., `change`) update attribute values 22 Exercise How does the composition relationship between objects influence memory management in an object-oriented program? Provide a concrete example. Answer The composition relationship means that an object is entirely dependent on the existence of the object that contains it. For example, in a House class containing a Room object, the destruction of House will also result in the destruction of Room. This implies that the 23 QCM 1. How does the existence of one object depend on another object in a composition relationship? A. The object depends on the existence of the first object. B. The objects can exist independently. C. The object is automatically updated. D. The object changes type. 24 QCM (2) 2. What is the typical lifecycle of an object in object-oriented programming? A) Creation, use, modification, destruction B) Creation, modification, deletion, restart C) Creation, duplication, use, destruction D) Instantiation, interaction, saving, deletion 25 QCM (3) 3. How do an object's attributes change in object-oriented programming? A) By directly modifying the memory area B) By using methods to update the values C) By copying the values into new memory D) By using global variables 26 QCM (4) 4. What is the relationship between methods and attributes in object-oriented programming? A) Methods are independent of attributes B) Methods access and modify the object's attributes C) Methods and attributes are stored separately in memory D) Attributes modify methods 27 Introduction to the concept of a Class A Class is a template – or a blueprint – that defines the form of an object. It specifies the data and the code that will work with that data. Objects are created from this blueprint, and the data inside them is called instance variables. Classes define two main parts of an object: the data and the actions (methods). The data, stored as instance variables, describes the object, while the methods define what the object can do. Instance variables are declared outside of methods and belong to the class. Each object created from the class has its own copy of these instance 28 Introduction to the concept of a Class (2) In the code above, we created a basic class called Vehicle, which can tell us the number of passengers and fuel capacity. 29 Class Constructor A constructor is a special method in a class that is called when an object of the class is created. It initializes the object's attributes. The constructor has the same name as the class. In Java, the “this” keyword is used to refer to the current object. It helps to access the instance variables and methods of the 30 Getter A getter is a method used to retrieve the value of an object's attribute. It follows the convention `getAttributeName()`. 31 Setter A setter is a method used to set or update the value of an object's attribute. It follows the convention `setAttributeName()`. 32 Object instance In Java, an object instance refers to a specific realization of a class. When you create an object from a class using the keyword “new”, you are creating an instance of that class. 33 Example of Object instance 34 Instance variables and class variables In Java, instance variables and class variables are two types of variables used in object-oriented programming, but they differ in their scope and behavior. Instance Variables Definition Instance variables are declared within a class but outside any method, constructor, or block. Characteristics They are associated with a specific instance of the class. Each object (instance of the class) has its own copy of the instance variables. 35 Instance variables and class variables (2) 36 Instance variables and class variables (3) Static Variables definition Static variables, also known as class variables, are declared with the static keyword in a class but outside any method, constructor, or block. Characteristics: They are associated with the class itself rather than any instance. A single copy of the variable is shared among all instances of the class. They can be accessed directly by the class name, without needing to create an instance. They are initialized once when the class is loaded 37 Instance variables and class variables (4) 38 QCM What is the purpose of a constructor in Java? - A) Declare instance variables - B) Initialize objects - C) Create static methods - D) Define constants What is the function of the `this` keyword? - A) Access class variables - B) Call the default constructor - C) Reference the current object - D) Create a static method 39 QCM (2) What is the purpose of `getter` and `setter` methods? - A) They allow modifying only class variables - B) They allow controlled access to and modification of instance variables - C) They are used to create constructors - D) They define static methods What is the scope of a variable declared with the `static` keyword? - A) It is limited to the specific instance of the class - B) It is accessible only within the constructor - C) It is shared among all instances of the class - D) It is not accessible from the class methods 40 QCM (3) What is the main difference between instance variables and class variables? - A) Instance variables are accessible only within the class where they are defined, while class variables are accessible everywhere. - B) Instance variables are tied to a specific instance of the class, while class variables are shared among all instances of the class. - C) Instance variables can be static, but class variables cannot be. - D) There is no difference. 41 Object-Oriented Programming (OOP) Languages vs. Languages Manipulating Objects Object-Oriented Programming (OOP) Languages: In OOP languages, like Java or C++, the programmer creates and defines their own classes. The programmer not only uses existing classes and objects but also creates new classes, defines methods, and specifies the attributes that the objects will have. For example, if you wanted to represent a `Car`, you would define a `Car` class with attributes like `color` and `speed`, and methods like `accelerate()` and `brake()`. You can then create `Car` objects from this class and manipulate them in your program. 42 Object-Oriented Programming (OOP) Languages vs. Languages Manipulating Objects (2) Languages Manipulating Objects: These are languages where the primary interaction with objects is limited to using predefined classes and objects, rather than creating new ones. Scripting languages like JavaScript often fall into this category. In these languages, you typically don't create new classes. Instead, you work with objects that are instances of classes already defined by the language or its libraries. For example, when working on a web page, you might want to change the size of a font. You would use an existing `Font` object and call its method `setSize()` with a specific value, like `f.setSize(16)`. However, you never define the 43 Executing the Method on a Specific Object Let's take a simpler example: imagine a `Lamp` class that represents a lamp. The `turnOn()` method turns the lamp on by changing its state. Here's44 Executing the Method on a Specific Object (2) The `turnOn()` method needs to know which specific object it applies to. The dot (`.`) in the instruction connects the method to the object it should operate on. It allows the method to access only the attributes of that specific object. 45 How Objects Communicate In object-oriented programming (OOP), objects interact with each other to perform useful tasks. Imagine a teacher and a student: the teacher gives instructions, and the student follows them. Here, the Teacher and Student are objects that belong to their own classes. Each object has specific attributes, like the teacher having a subject and the student having a grade. In OOP, methods are like actions these objects can perform. For example, a teacher might have a method to "teach a lesson," and the student might have a method to "learn." When the teacher teaches, it sends a message to the student to start learning. This interaction between objects—where one object starts an action in another—is what makes the program work effectively. 46 Finding Message Recipients In object-oriented programming (OOP), imagine we have a Car class that represents a car, with attributes like color and brand, and a Parking class that represents a parking that can hold cars. Steps to Check if a Car is in the Parking : Identify the Car Type: First, we need to make sure the Parking object knows that what it’s working with is indeed a Car. This ensures that the parking doesn’t mistakenly handle something else, like a bicycle, as a car. Store the Car in Parking: Instead of always passing the car as an argument to methods whenever we need to check something about it, we can make the Parking object keep a reference to the car as part of its own data (like an attribute). This way, the Parking object knows which car it has, and we don't need to provide the car's details every time we perform an action. 47 Finding Message Recipients (2) Example: Car Class: This represents the car, with details like its color and brand. Parking Class: This represents the parking lot, which can hold a reference to the car. By having the Parking class keep a reference to the car, the parking lot can easily check if the car is present without needing to receive the car information repeatedly. The parking lot already knows which car it’s dealing with, making the interaction simpler and more efficient. 48 Inheritance What is Inheritance: A fundamental concept in object- oriented programming (OOP) where a new class (subclass or child class) derives from an existing class (superclass or parent class). This allows the subclass to inherit attributes and methods from the superclass, promoting code reuse and a hierarchical organization of classes. 49 Hierarchy of Objects Think about objects like "phone," When you say "phone," it could be more specific, like an "iPhone 14" or a "flip phone." In programming, objects can be categorized in a hierarchy from general to specific. In object-oriented programming (OOP), we organize classes in a hierarchy. This is called inheritance. For example, "phone" is more general, while "iPhone 14" is more specific. Phon e 50 Contextual Dependence on the Right Taxonomic Level Context-Specific Terminology: In everyday conversations, we often use general terms like "car" because they usually convey enough meaning for the situation. When you tell a friend, "I saw a car parked outside," they understand what you mean without needing more detail. The term "car" is sufficiently specific for most casual interactions. Generalization Issues: However, using even broader terms, like "means of transportation," can create confusion because it includes a wide range of vehicles, such as cars, trains, planes, and bicycles. If someone says, "I arrived by means of transportation," it’s unclear what type of vehicle they used, making the communication less effective. 51 Contextual Dependence on the Right Taxonomic Level (2) Context Matters: The level of detail required in communication depends on the context. For example, if you’re talking to a mechanic about fixing your vehicle, you might need to be more specific and say, "My Toyota Corolla is making a strange noise." Here, the term "car" wouldn’t provide enough detail for the mechanic to understand the issue and offer help. The context dictates that a more specific term is necessary. 52 Polymorphism Polymorphism is a fundamental concept in object-oriented programming that allows different objects to respond to the same message or method call in a way that is specific to their type. Polymorphism is the ability to create methods or reference variables that behave differently in different programming contexts. Polymorphism complements inheritance. 53 Polymorphism example In the computer screen example, when you click with your mouse, all the objects on the screen (windows, icons, etc.) receive the same click, but each reacts differently according to its nature. This is another illustration of polymorphism: a single event (the click) triggers various behaviors depending on the objects involved. 54 References BERSINI, Hugues. La programmation orientée objet. Editions Eyrolles. 2010. Head First Java, Second Edition, By Kathy Sierra, Bert Bates, O'Reilly Media. Programmer en JAVA, 4ème édition, Deitel et Deitel, Les éditions Reynal Goulet. http://java.sun.com Le Programmeur JAVA 2, Lemay L, Campus Press. Au cœur de Java 2 Volume I - Notions fondamentales, Horstmann et Cornell, The Sun Microsystems Press Java Series. Programmer en Java, Claude Delannoy, Eyrolles. 55