Comprog.pdf
Document Details
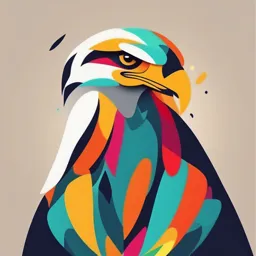
Uploaded by FlashyVariable3695
STI College
Full Transcript
# Data Types Data encoded to a program is stored in memory and can have and be in several types - for example, using a person's basic information such as name, age, and address. The name will be stored as characters, the age will be stored as a numeric value, and the address will be stored as an al...
# Data Types Data encoded to a program is stored in memory and can have and be in several types - for example, using a person's basic information such as name, age, and address. The name will be stored as characters, the age will be stored as a numeric value, and the address will be stored as an alphanumeric value. Data Types describe possible operations on the data and the storage method. The data types in Java are classified into two (2): primitive or standard data types and abstract or derived data types. ## Primitive Data Types (Agarwal & Bansal, 2023) Primitive Data Types are built into the Java language. These are data that are not objects and have no methods. The Java compiler has detailed instructions on the valid operations this data type supports. Here are the eight (8) Primitive types that Java defines: | Data Type | Description | Size | Default Value | Range | | :-------- | :------------------ | :-- | :------------ | :-------------------------------- | | Byte | Byte Length Integer | 8 bit | 0 | -128 to +127 signed | | Short | Short Integer | 16 bit | 0 | -32768 to +32767 | | Int | Integer (number) | 32 bit | 0 | - 2,147,483,648 to 2,147,484 | | Long | Long Integer | 64 bit | 0L | -2<sup>63</sup> to 2<sup>63</sup>-1 | | Float | Single Precision Floating Point | 32 bit | 0.0f | +1 - about 10<sup>39</sup> | | Double | Double-Precision Floating Point | 64 bit | 0.0d | 1 - about 10<sup>317</sup> | | Char | A simple character | 8 bit | \u0000 | 0 to 65535 | | Boolean | A Boolean (true or false) | 1 bit | false | 1 to 0 | **Table 1. Data types** ### Integer Types Java defines Byte, Short, Int, and Long as Integer data types. Since Java does not support unsigned integers, these four (4) are signed, positive, and negative values. Java does not support unsigned integers as these are mostly used to specify the behaviors of the high-order-bit. High-order bit defines the sign of an integer value, and Java manages it differently by adding a special "unsigned right shift" operation. * **Byte**: The smallest integer type that represents a small number. It is used to declare a variable holding a natural number between -128 and 127. Byte variables are declared by use of the byte keyword. Syntax: **byte b;** * **Short**: An integer is considered Short if its value is between -32768 and 32767. Short data types declare a variable that can hold numbers in that range. * **Syntax**: **short a;** **For example**: ```java public class ShortSample { public static void main(String[] args){ short total_stu = 1192; System.out.println("The total number of students are " + total_stu); } } ``` A hexadecimal number can also be initialized as a Short variable instead of a normal decimal integer. So, the 3001 integer can be written as **0x4A8**. **Ox** is used in Java to represent hexadecimal. * **Int**: This data type can handle larger numbers and is considered the best choice when an integer is needed because when Byte and Short values are used in an expression, they are promoted to Int automatically. **For example**: ```java public class IntSample { public static void main(String[] args) { int days = 279; System.out.println("The days are " + days); } } ``` * **Long**: This data type holds a number higher than a regular integer. The Long integer is a variable that can hold a very large number. A capital letter 'L' is placed right after the value. **For example**: ```java public class LongSample { public static void main(String[] args) { long distance = 84564L; System.out.println("The distance is " + distance); } } ``` ## Floating Point Types Floating points are also called "real numbers", and are used when evaluating an expression that needs fractional accuracy such as the computation of square root or a transcendental (sine and cosine). Float and Double are both floating-point types. * **Float** is described as a single precision floating point. A real number qualifies as a single precession when it only needs a limited number of bits. Single precision takes half the space of a double precision and is much faster on some processors. Floats are used to represent dollars and cents, so a small letter ‘f’ is placed to the right of the value when initializing the variable. **For example**: ```java public class FloatSample { public static void main(String[] args) { float pi = 3.14f; System.out.println("The value of pi is " + pi); } } ``` * **Double**: Double precision can be faster than single precision on modern processors that have been optimized for high-speed mathematical calculations. It can handle larger numbers than Float. **For example**: ```java public class DoubleSample { public static void main(String[] args) { double pi = 3.141590; // a d/D suffix is optional. System.out.println(pi); } } ``` ## Character Types Character types can be divided into two (2): (a) A Byte for a character (ASCII) and (b) a type in itself (Unicode). * **A character** is recognized by its ASCII (American Standard Code for Information Interchange - a code that assigns numbers to letters, digits, etc.) number. It allows Byte data types to be used to declare a variable that would hold a single character to initialize such variable or assign a single quoted character. **For example**: ```java public class CharByte { public static void main(String[] arg) { byte Gender = 'F'; System.out.println("Gender is " + Gender); } } ``` * **To support characters** that are not traditionally used in (US) English and do not work with compilers, the Unicode character format was created which includes Latin-based and non-Latin-based languages. The Char data type was created to support Unicode characters in Java. **To initialize, assign the character variable in single quotes.** **For example**: ```java public class CharUnicode{ public static void main(String a[]) { char gender = 'f'; System.out.println("Gender is" + gender); } } ``` ## Boolean This data type is used for logical values and can only hold true or false values. It is a type returned by all relational operators such as =, >, and <. **For example**: ```java public class BoolSample{ public static void main(String[] args) { boolean b; b = false; System.out.println("b is " + b); b = true; System.out.println("b is " + b); } } ``` ## Abstract Data Types These are based on primitive data types but with more functionality. For example, String is an abstract data type that can store letters, digits, and other characters such as a slash (/), comma (,), parentheses (()), semi-colon (;), dollar sign ($), and a hashtag (#). Performing calculations on a variable of the string data type is not allowed, even if the data stored in it has digits. String, however, provides methods for linking two (2) strings, searching for one string within another, and extracting a portion of a string. # Constants and Variables (Agarwal & Bansal, 2023) Java uses constants and variables for storing and manipulating data in a program. The values of variables can be changed in a program, but the value of constants, cannot. Unique names must be assigned to variables and constants. If the name "digit" refers to an area in memory where a numeric value is stored, then "digit" is a variable. A variable is defined by the combination of an identifier, a type, and an optional initialization depending on the task to be performed. Regardless of the data type, each variable used in a program must be declared. The "digit" variable must be declared with an Int data type since it refers to a memory storing an integer value. ## Variable Naming Conventions Just like identifiers, naming variables must follow specific rules and conventions. The program will not compile if the programming language rules are not followed. In general, here are the rules for naming variables: * The name of a variable must be meaningful, short, and without any embedded space or symbol like ?, !, @, #, %, ^, &, ( ), [, ], {, }, ., \, /, or c/. However, underscores (_) can be used wherever a space is required. For example, monthly_Salary. * Variable names must be unique. For example, to store five (5) different numbers, five (5) unique variable names need to be used for each. * A variable name must begin with a letter, a dollar sign, or an underscore, which may be followed by a sequence of letters (any case) or digits (0-9), `$', or an underscore. * Keywords cannot be used for variable names. For example, a variable called "switch" cannot be declared. For Java, here are the variable naming conventions: * Variable names should be meaningful. * The names must reflect the data that the variables contain. To store the gender of an employee, the variable name could be emp_gender. * Variable names are nouns and must begin with a lowercase letter. * If a variable name contains two or more words, begin each word with an uppercase letter. However, the first word will start with a lowercase letter. Here are some examples of valid and invalid variable names: * **Valid**: address, student_age, emp_name * **Invalid**: # phone, 1st Name. ## Declaring and Initializing a Variable As mentioned, all variables in Java must be declared first before they can be used. The basic format of a variable declaration is as follows: ```java Type identifier [= value] (, identifier] [= value); // The brackets are optional. ``` The type refers to the data type. The identifier is the name of the variable. Using an equal sign allows assigning a value to a variable. To declare more than one (1) variable of the same data type, a comma (,) separated list is used. By following this format, examples include **int a, b, c; int d=7; byte z = 22;** Variables cannot also be used without initializing them. Java assigns a default value to the primitive data types class variables when creating an object or a class. The following displays the default initial values that are used to initialize the data members of a class. | Data Type | Default Initial Value | | :-------- | :------------------ | | Byte | 0 | | Short | 0 | | Int | 0 | | Long | 0L | | Float | 0.0 | | Double | 0.0 | | Char | 'u0000' | | Boolean | false | | Abstract Data Type | Null | 0 is replaced by the actual value to be used. The values are only automatically assigned to the data members of a class that are of the primitive data types. Java returns an error if the variable declared in a method is not initialized. ## Literals In Java, variables can be assigned with constant values. The constant values to be assigned must have the data type of the variable. Literals are the values that may be assigned to primitive- or string-type variables and constants, such as in the following: 1. Boolean literals are true or false and cannot use numbers to represent them. 2. Integer literals are numeric data that can be represented as octal, hexadecimal, and binary literals. Octal is the number with a zero prefixed. Hexadecimal is the number prefixed with **Ox**. Binary Literal is the number that starts with **OB** or **Ob**. An underscore (_) can be used to improve the readability of the numeric data. **For example**: ```java int six=6; int x=0xffff; // Hexadecilmal literal int b1=0B100101; // Octal literal int b2=0b01010101; // Binary literal // Binary literal ``` A reminder that an underscore (_) at the start and end of a literal is not allowed, such as: ```java int i=_1_00_000; //illegal, begin with _ int j=2_00_4201_; //illegal, end with _ ``` 3. Floating-point literals are numbers that have a decimal fraction. A suffix letter F (lower or uppercase) to the number; otherwise, the compiler will flag about loss of precision. ```java float f=42.642658; //compiler error, possible loss of precision float g=6437.2395065F; //valid, has the suffix “F” ``` 4. Character literals must also be enclosed in single quotes (','). The Unicode value of the character is also allowed. ```java char varN='\u004E'; // The Letter N ``` A backslash (\) can also be used to represent a character that can be typed in as a literal, such as: ```java char c= '\"'; // A double quote char d= '\n'; // A newline char e= '\t'; // A tab ``` 5. On the other hand, string literals are enclosed in double quotes (","). Strings are included as literal as they can still be typed directly into the code, even though it is not a primitive type. ```java String s = "All emotions are valid"; ``` ## References: * Agarwal, S. & Bansal, H. (2023). Java in depth. BPB Publications. * DG Junior (2023). Basics of programming: A comprehensive guide for beginners. DG Junior.